10 API Secrets Even Pro Developers Miss And How to Fix Them
Discover the 10 critical API secrets that even seasoned developers often overlook—and how to fix them.
You’ve built APIs that work. They return data, handle requests, and maybe even have decent documentation. But here’s the uncomfortable truth: your API might be a ticking time bomb.
Why? Because most developers focus on “it works” instead of “it works well.” The difference between a “good enough” API and a bulletproof one? Tiny, easily overlooked details that crash apps, frustrate users, and kill scalability.
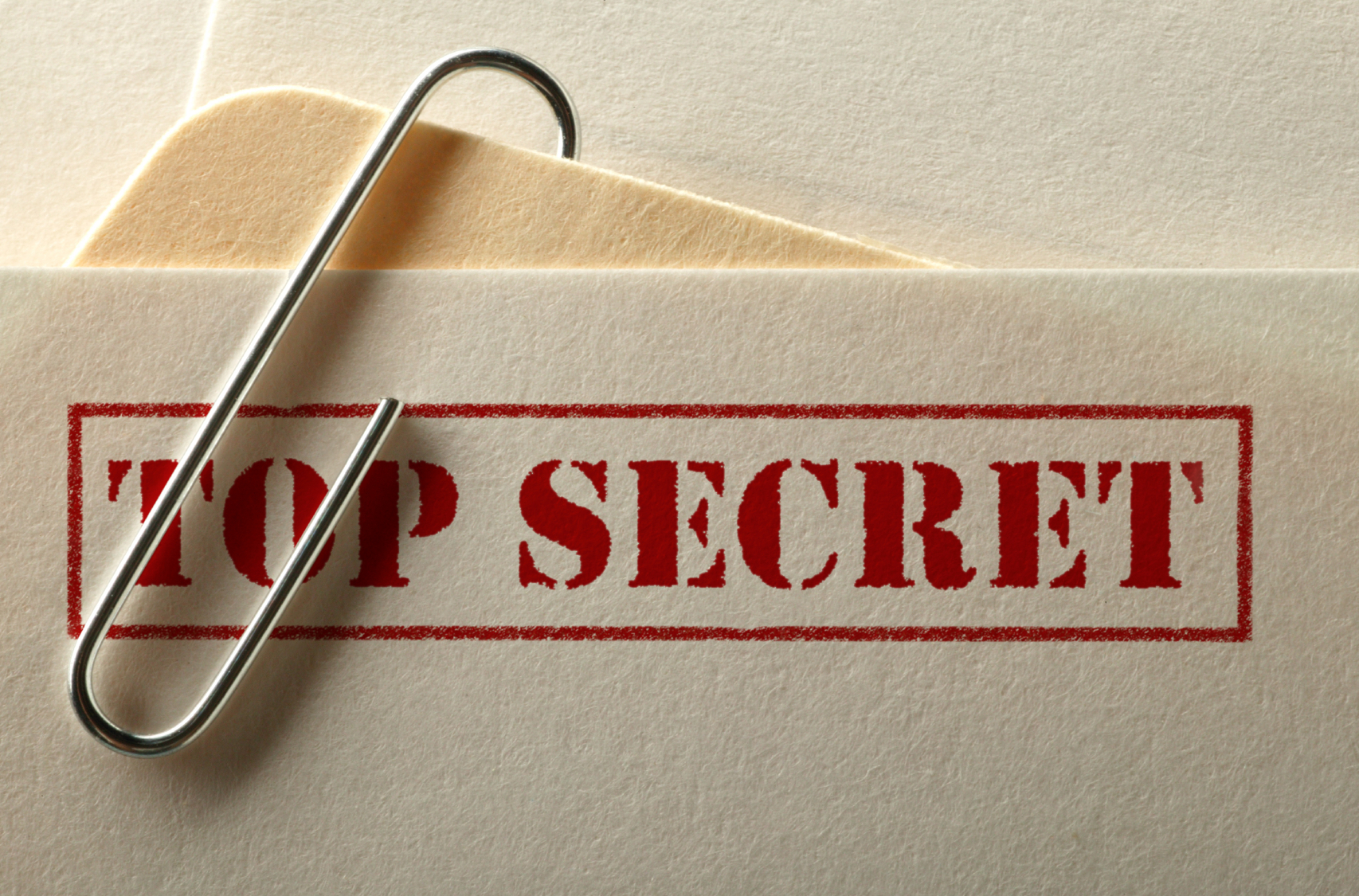
I’ve seen senior engineers make these mistakes. I’ve made them myself. But after debugging 500+ APIs, I’ve learned the 10 non-negotiable rules every API needs. Let’s fix your API before it fails.
1. Versioning: Stop Breaking Apps Overnight
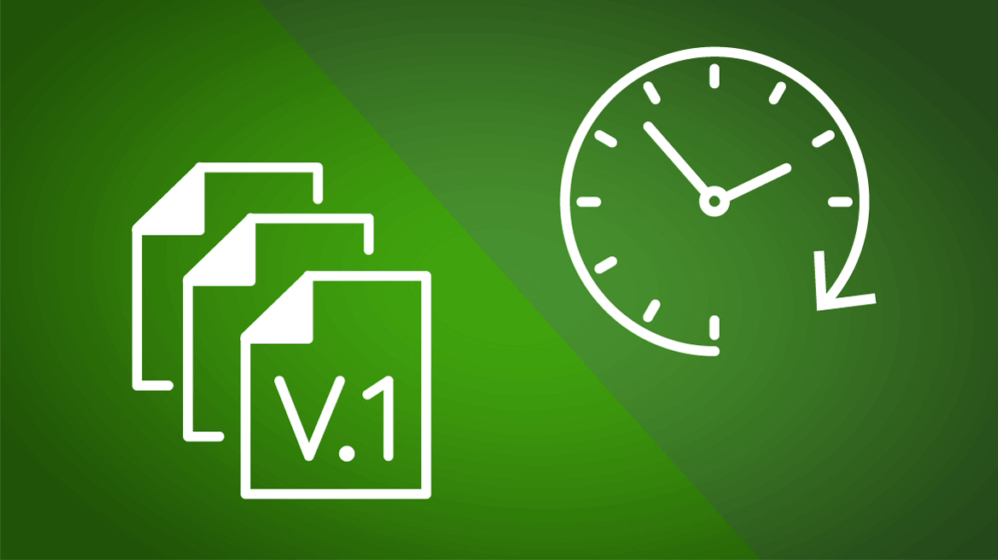
The Mistake: Changing your API and breaking every app that uses it.
The Fix: Version from Day 1.
How to Do It:
- Add a version to your URL:
https://api.yourservice.com/v1/users
- Use headers like
Accept: application/vnd.yourapi.v1+json
2. Authentication: Don’t Be the Next Data Leak
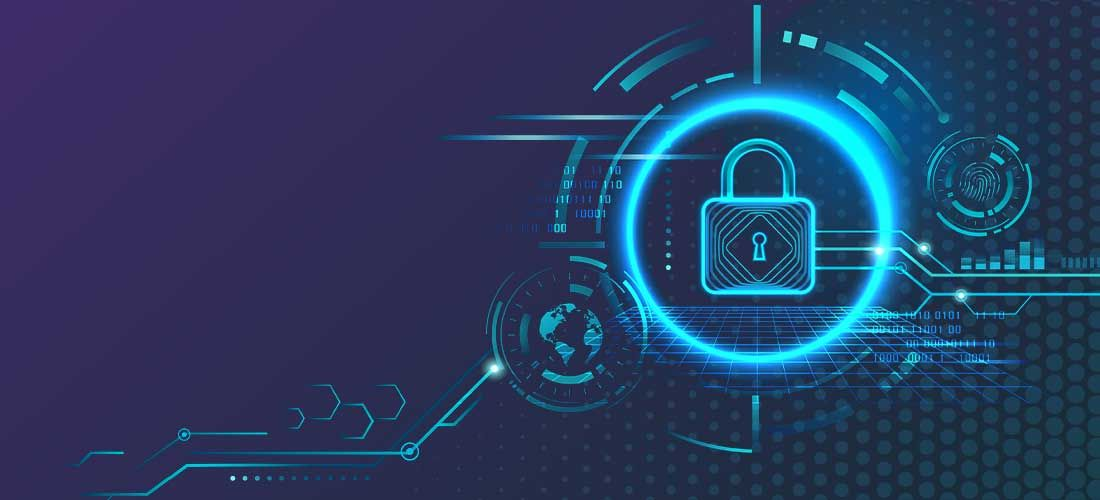
The Mistake: Rolling your own auth (spoiler: it’s insecure).
The Fix: Use OAuth 2.0 or API keys.
Step-by-Step:
- For API keys: Generate unique keys per user in your dashboard.
3. Error Handling: Speak Human, Not Code
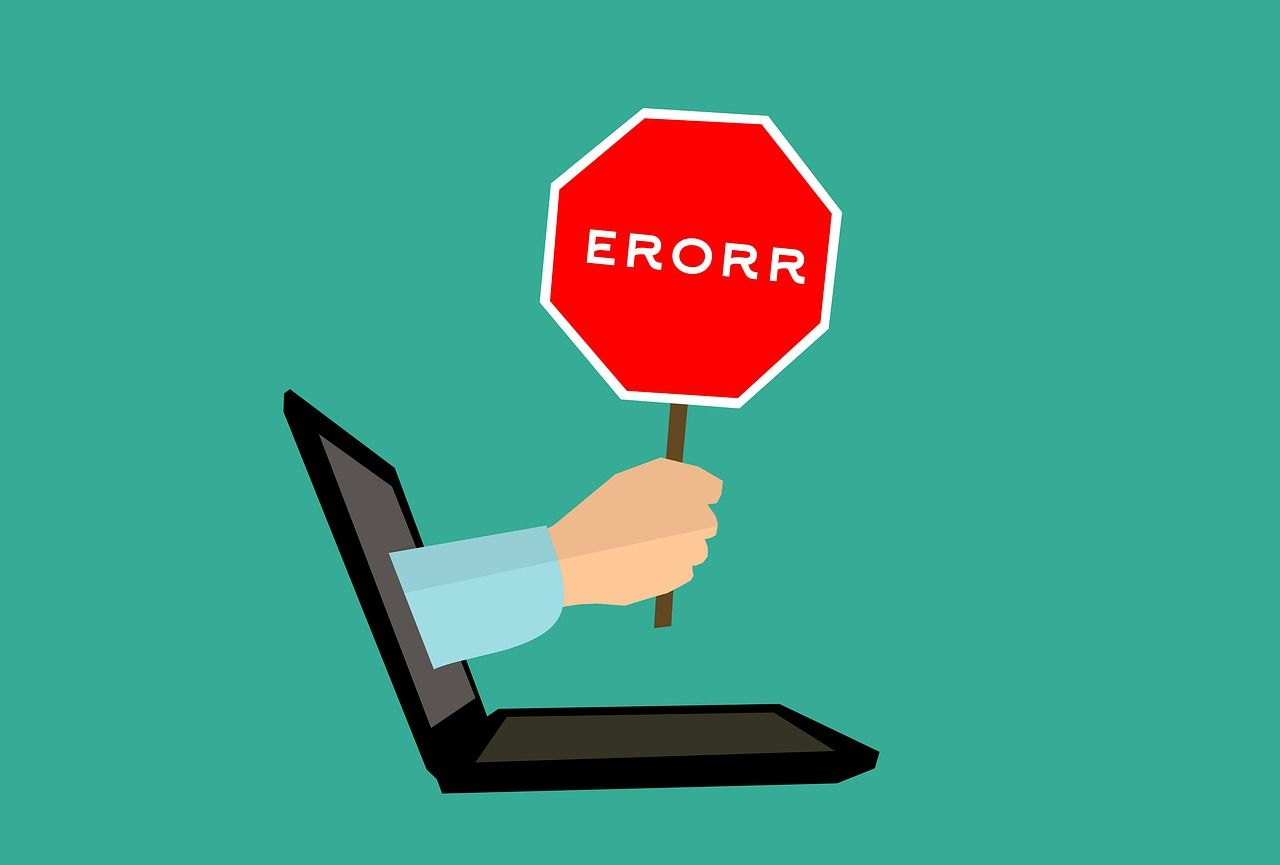
The Mistake: Returning {"error": "FAILED"}
.
The Fix: Explain errors clearly.
Example of a Good Error:
{
"error": {
"code": "invalid_email",
"message": "Email must include @ symbol",
"documentation": "https://api.yourservice.com/errors#invalid_email"
}
}
Test in EchoAPI: Force errors (e.g., send malformed data) and verify responses include actionable details.
4. Rate Limiting: Save Your Server from Being DDOS’d
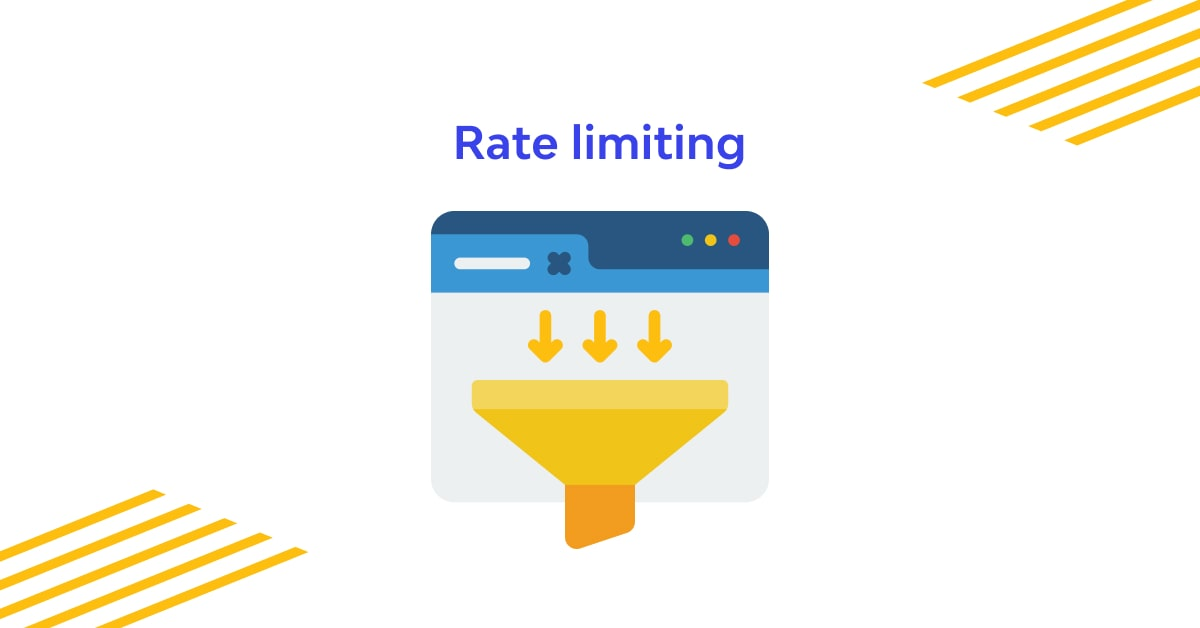
The Mistake: Letting one user crash your API with 10,000 requests/second.
The Fix: Add rate limits.
How to Implement:
- Use headers like
X-RateLimit-Limit: 1000
andX-RateLimit-Remaining: 999
. - EchoAPI Pro Tip: Use the “Load Testing” feature to simulate traffic spikes.
5. Pagination: Don’t Return 10,000 Records at Once
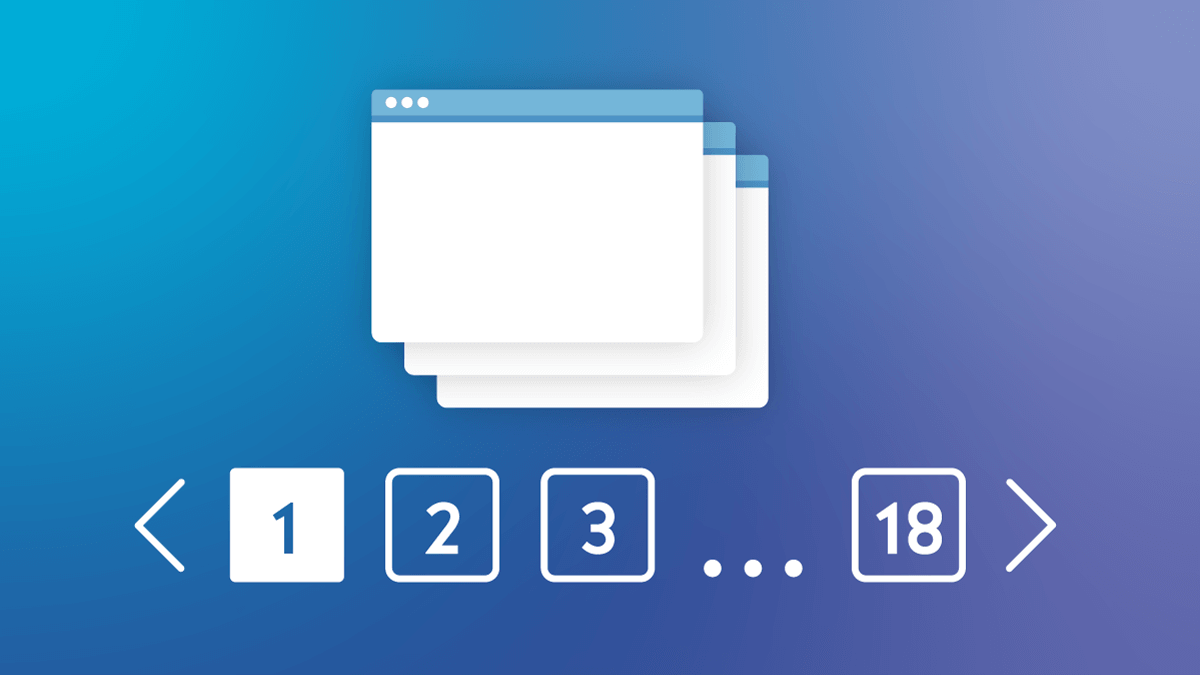
The Mistake: Sending massive datasets that slow down apps.
The Fix: Use limit
and offset
(or cursor-based pagination).
Example Request:GET /v1/products?limit=50&offset=100
6. Caching: Stop Wasting Server Resources
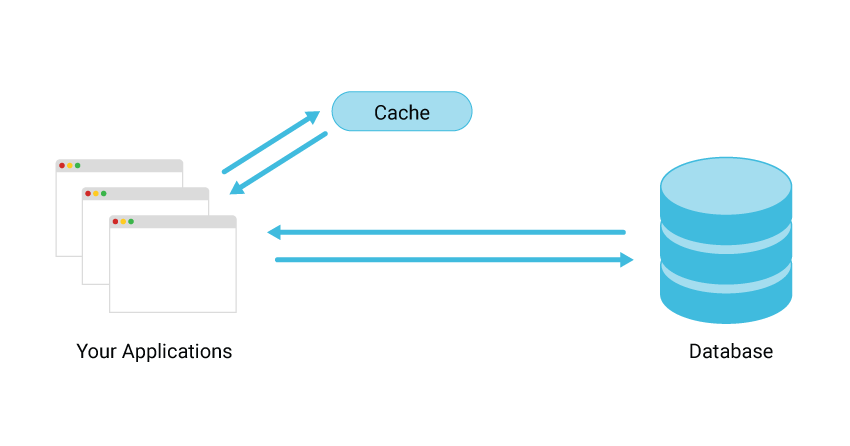
The Mistake: Processing the same request 1,000 times.
The Fix: Add Cache-Control
headers.
Simple Caching:Cache-Control: public, max-age=3600
(cache for 1 hour)
EchoAPI Hack: Use the “Response Inspection” tool to check if caching headers are present.
7. Documentation: Stop Getting 100 Support Emails
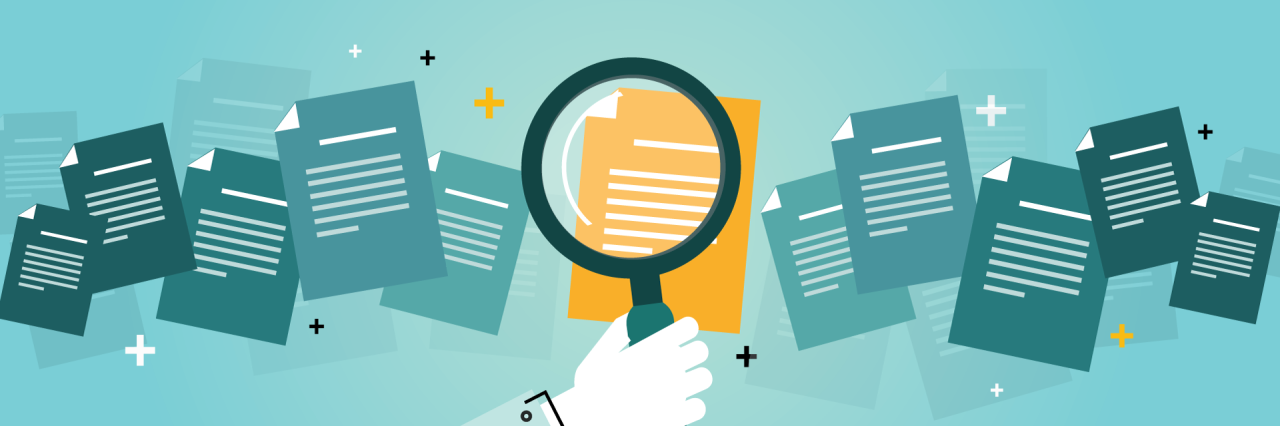
The Mistake: Writing docs that only you understand.
The Fix: Use OpenAPI (Swagger) and test your docs.
EchoAPI Integration: Auto-generate interactive docs from your API definitions.
8. Validate Inputs: Kill Bad Data at the Door
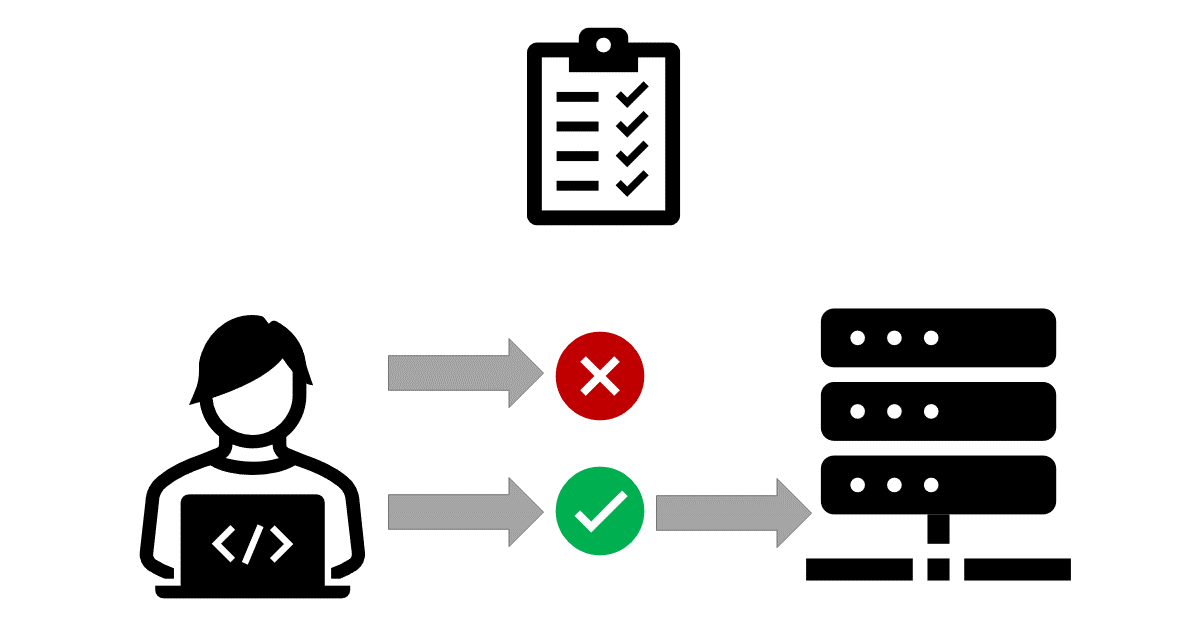
The Mistake: Trusting user input (hello, SQL injections!).
The Fix: Validate EVERY input.
Example Validation Rules for /users
endpoint:
- Email: Must match regex
/.+@.+\..+/
- Password: Min 8 chars, includes a number
Test in EchoAPI: Send invalid data and verify your API rejects it with specific errors.
9. Use HTTPS: No Excuses in 2025
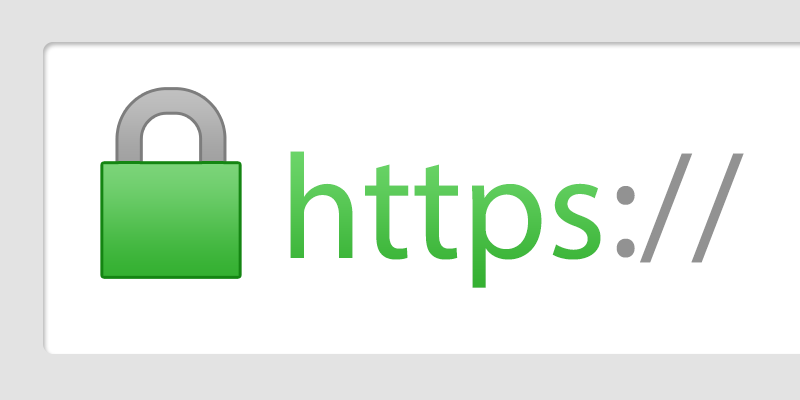
The Mistake: Sending sensitive data over HTTP.
The Fix: Force HTTPS everywhere.
How to Enforce:
- Redirect HTTP → HTTPS
- Use HSTS headers
10. Monitor Everything: Catch Issues Before Users Do
The Mistake: Assuming your API “just works.”
The Fix: Track uptime, latency, and errors.
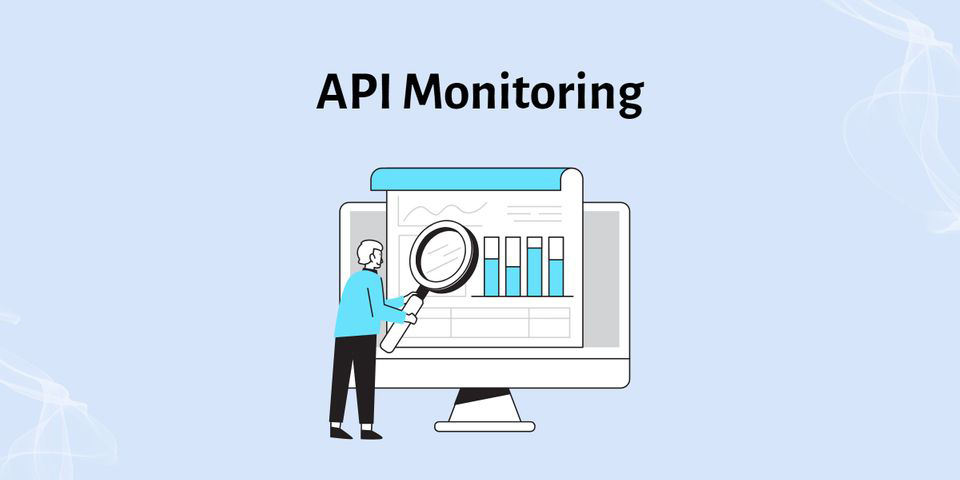
Conclusion
These 10 rules separate APIs that “work” from APIs that thrive. The best part? You don’t need to be a guru to implement them. Tools like EchoAPI automate 80% of the work:
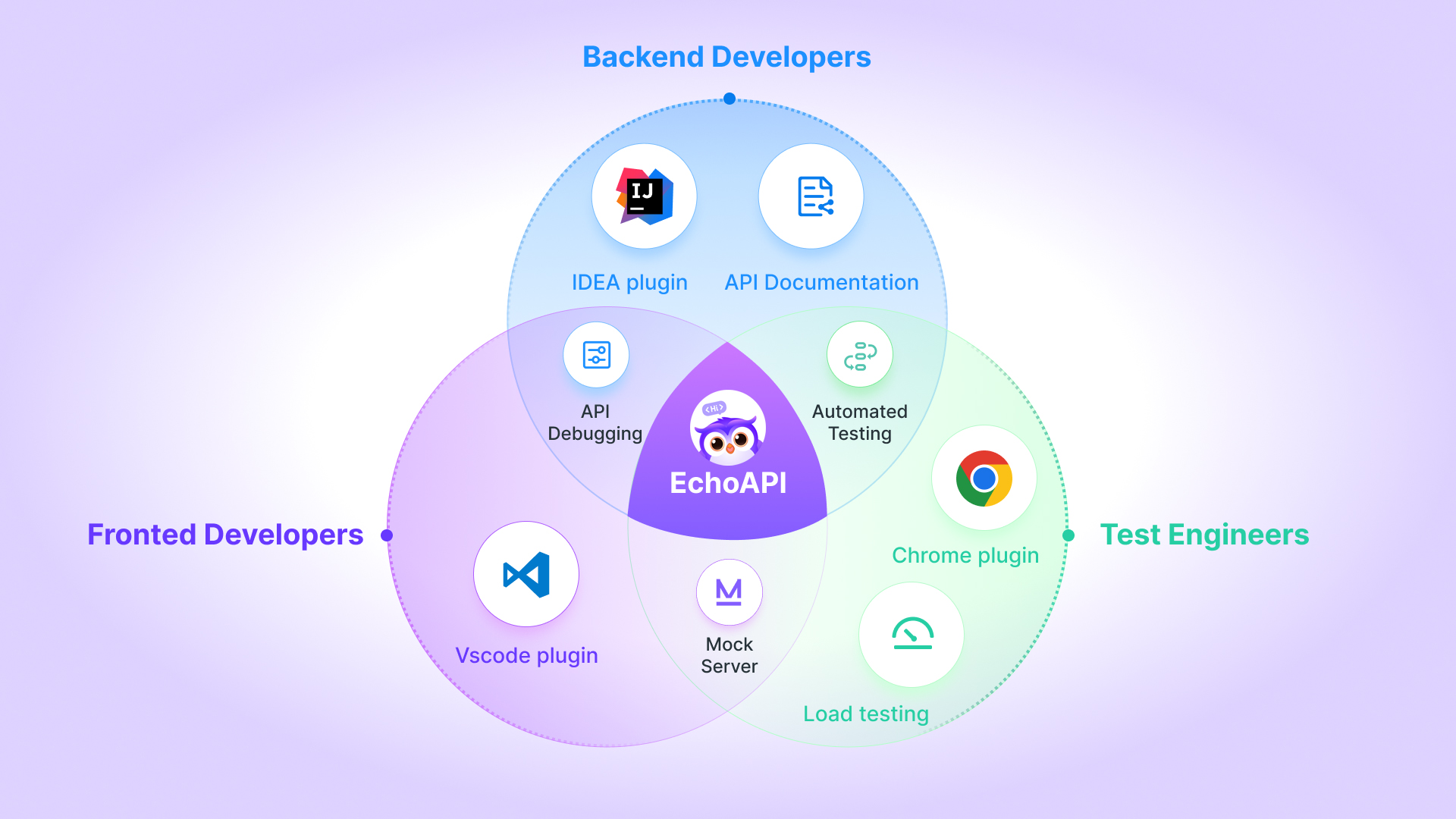
- Test endpoints with one click
- Auto-generate docs and client SDKs
- Monitor performance in real-time
Your move: Pick one practice from this list and implement it today. Then test it with EchoAPI’s free tier. Your future self will thank you.