(2) Practicing and Optimizing API Testing with Postman and EchoAPI - Interface Signature
In production environments, ensuring the integrity and security of API calls is paramount. Interface signatures are a commonly employed technique to achieve this. This article explores a typical signing rule and demonstrates the implementation process in Postman and EchoAPI, analyzing the advantages and shortcomings of each.
Signature Rules
Overview
- Parameter Sorting: All non-empty parameters in the request body must be sorted according to the ASCII dictionary order of their parameter names.
- String Concatenation: Concatenate these parameters into a string in the format
key1=value1&key2=value2
, creatingstringA
. - Signature Generation: Append a secret key to
stringA
, generating a temporary stringstringSignTemp
. Perform an MD5 hash on this string and convert it to uppercase to obtain the final signature. This signature should be added to the request URL's query parameters.
Important Considerations
- Empty value parameters and the
sign
parameter itself should not be included in the signature calculation. - Parameter names are case-sensitive, and case mismatches can lead to errors.
Postman Implementation: Testing Signature with Pros and Cons
Implementation Steps
To compute and debug the signature in Postman, you need to write the necessary code in the pre-request script. The basic steps include:
- Retrieve the current request's body parameters (using
pm.request.body.raw
). - Calculate the signature according to the rules.
- Add the computed signature to the URL's query parameters (using
pm.request.addQueryParams
).
Here's the code for implementation:
// Function to calculate the signature according to the rules
function calculateSign(params, secretKey) {
// Filter out empty value parameters
const filteredParams = Object.entries(params).filter(([key, value]) => value !== "");
// Sort by parameter names in ASCII order
filteredParams.sort(([key1], [key2]) => key1.localeCompare(key2));
// Concatenate into a string in key=value format
const stringA = filteredParams.map(([key, value]) => `${key}=${value}`).join("&");
// Append the secret key
const stringSignTemp = `${stringA}&${secretKey}`;
// Perform MD5 hashing and convert to uppercase
const sign = String(CryptoJS.MD5(stringSignTemp)).toUpperCase();
return sign;
}
// Process the raw request body data
const bodyRaw = JSON.parse(pm.request.body.raw);
const secretKey = "yourSecretKey"; // Replace with your secret key
const sign = calculateSign(bodyRaw, secretKey);
pm.request.addQueryParams([{
key: "sign",
value: sign
}]);
After executing the request, you'll see the signature parameter (sign
) successfully added to the URL:
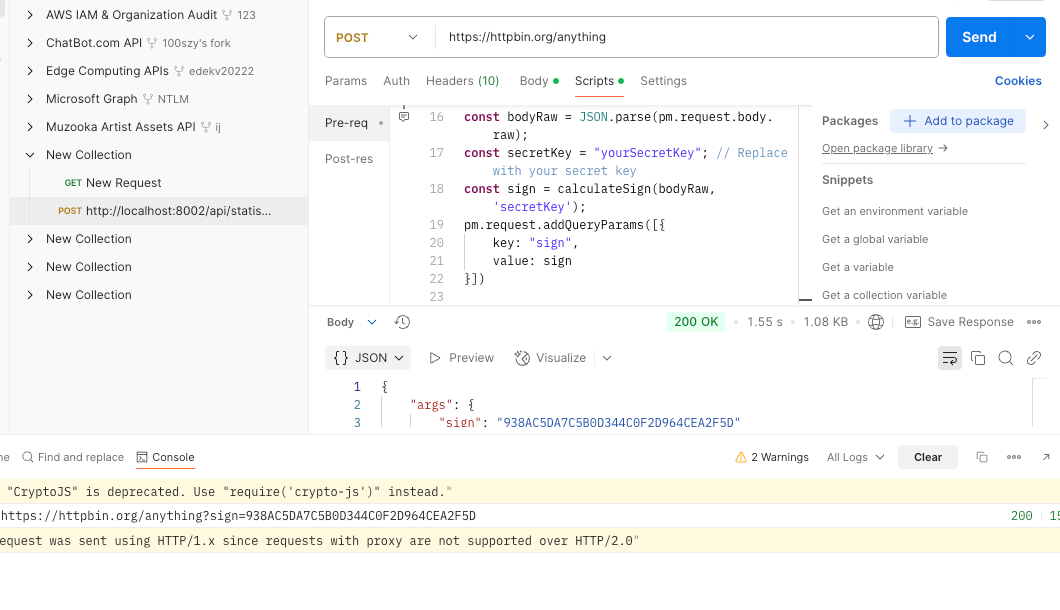
Analysis of Limitations
While this method works perfectly when there are no variables in the request body, it falls short when variables are used. In such cases, pm.request.body.raw
retrieves values before variable substitution, leading to incorrect signature calculations and frequent errors.
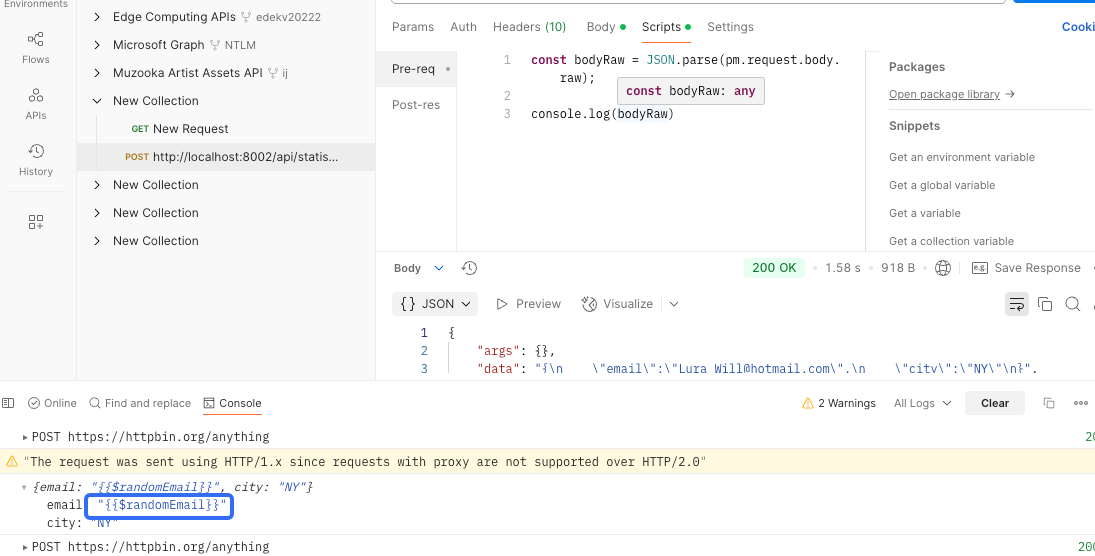
EchoAPI Implementation: Signature Testing with Advantages
Implementation Process
The process in EchoAPI mirrors that in Postman and ensures full compatibility with Postman code, providing developers with a consistent testing experience.
Overcoming Postman Limitations
EchoAPI extends the pm.getFinalRequestRawBody()
method to support retrieval of request bodies with substituted variables, addressing Postman’s limitation. Only a single line update is required, as shown below:
// Function to calculate the signature according to the rules
function calculateSign(params, secretKey) {
// Filter out empty value parameters
const filteredParams = Object.entries(params).filter(([key, value]) => value !== "");
// Sort by parameter names in ASCII order
filteredParams.sort(([key1], [key2]) => key1.localeCompare(key2));
// Concatenate into a string in key=value format
const stringA = filteredParams.map(([key, value]) => `${key}=${value}`).join("&");
// Append the secret key
const stringSignTemp = `${stringA}&${secretKey}`;
// Perform MD5 hashing and convert to uppercase
const sign = String(CryptoJS.MD5(stringSignTemp)).toUpperCase();
return sign;
}
// Process the final raw request body
const bodyRaw = pm.getFinalRequestRawBody(); // Only change needed here
const secretKey = "yourSecretKey"; // Replace with your secret key
const sign = calculateSign(bodyRaw, secretKey);
pm.request.addQueryParams([{
key: "sign",
value: sign
}]);
After sending the request, the signature parameter (sign
) is correctly appended to the URL:
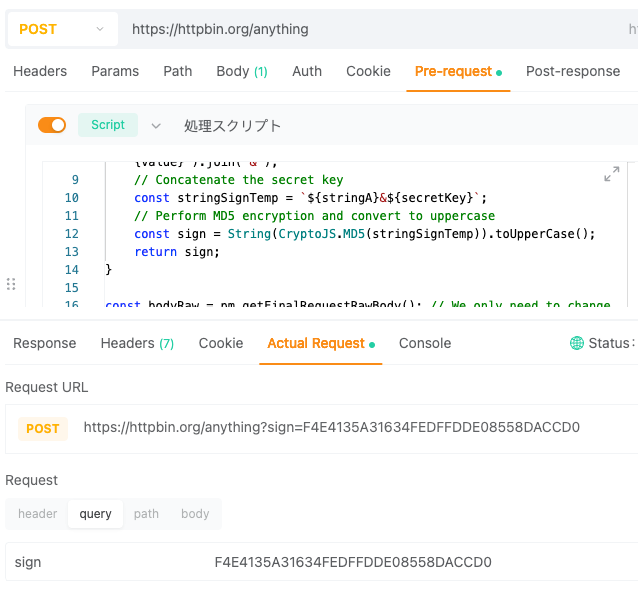
Conclusion
In API design and testing, interface signatures play a crucial role in ensuring data security and call integrity. A comparison between Postman and EchoAPI reveals that while Postman is feasible for implementing signatures, it has notable shortcomings when handling variable substitution. EchoAPI’s extensibility effectively resolves these issues, providing developers and testers with a convenient tool. For API interface testing, EchoAPI is recommended to enhance work efficiency and reduce errors.