API Authentication Demystified: Mastering OAuth, API Keys, and JWT Like a Pro
In this article, we simplify API authentication by mastering OAuth, API keys, and JWT.
In this article, we simplify API authentication by mastering OAuth, API keys, and JWT. Understanding these essential security measures will empower developers to create secure APIs that protect user data and system integrity.
The Silent Guardian of Your APIs (That You’re Probably Ignoring)
Let’s play a quick game. Imagine you’ve built a killer API. It’s fast, scalable, and packed with features. But here’s the twist: no one cares. Why? Because you forgot to lock the front door.
Surprised? Most developers obsess over functionality but treat security like an afterthought—until a breach happens. APIs are the backbone of modern apps, but they’re also hacker magnets. The difference between a secure API and a disaster? Authentication.
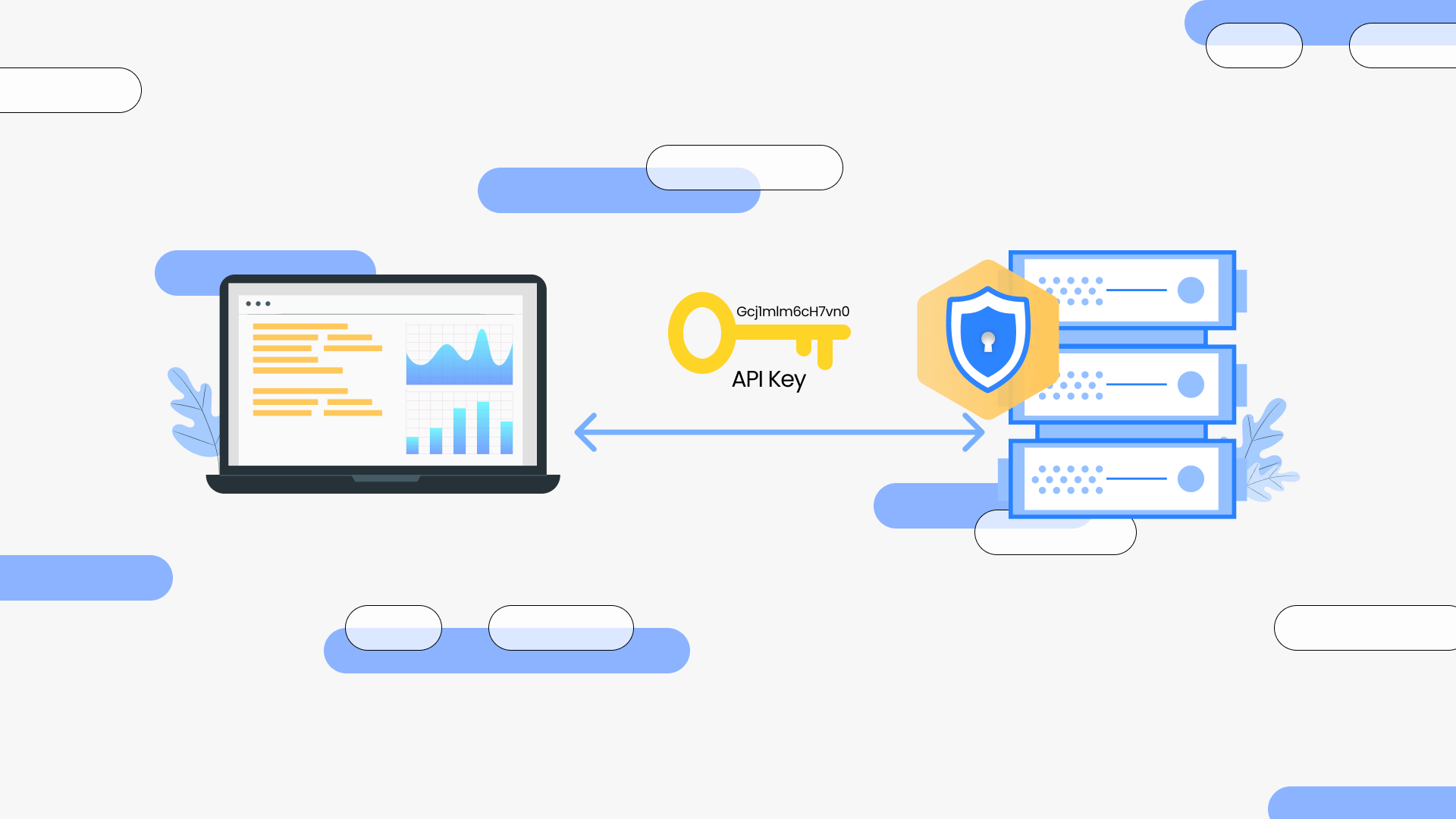
Here’s the kicker: authentication isn’t boring. It’s the secret sauce that decides who gets in, who gets blocked, and who accidentally DDoSed your server. Let’s break down the three heavyweights—OAuth, API Keys, and JWT—and turn you from “meh” to “master” in 15 minutes. Oh, and you’ll learn how to test them all with EchoAPI (spoiler: it’s ridiculously easy).
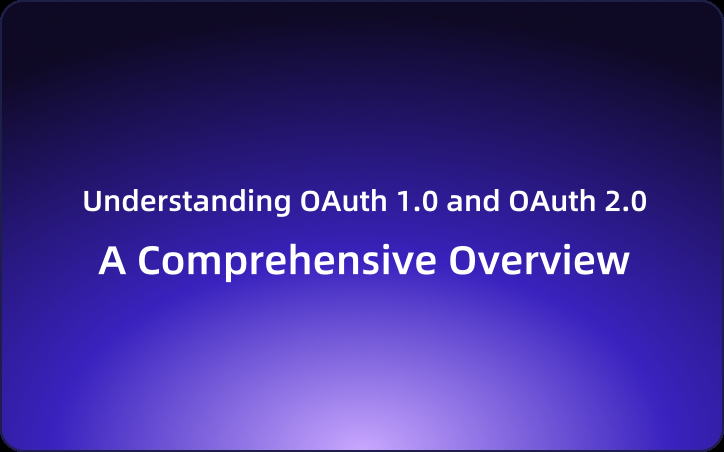
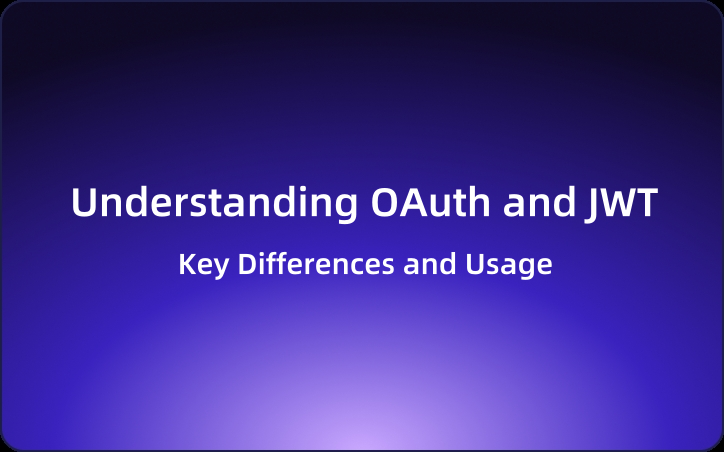
Middle: Let’s Get Our Hands Dirty
1. API Keys: The “Hello World” of Authentication
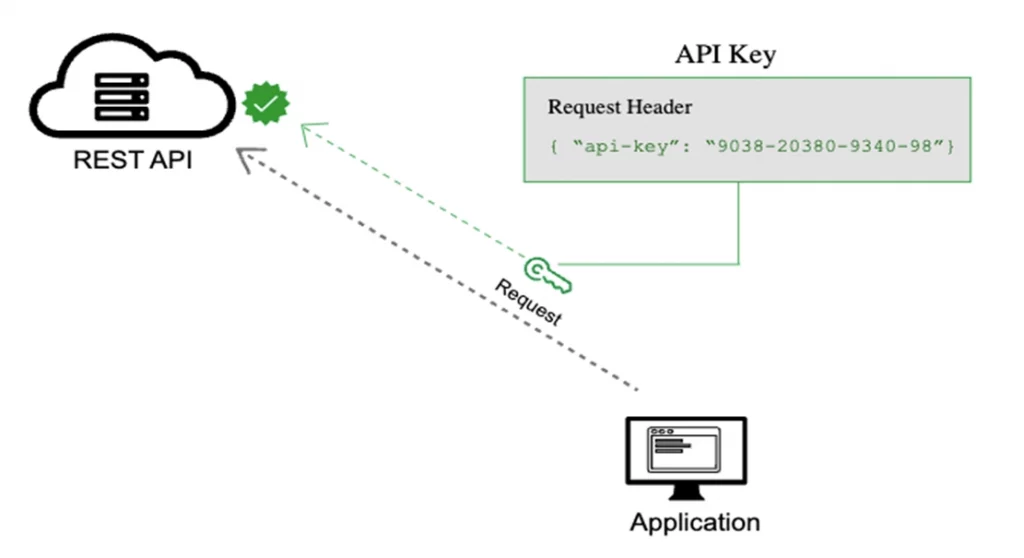
What it is: A simple string (like sk_1234abcd
) passed in headers or URLs to identify clients.
Use case: Quick, low-security scenarios (e.g., weather APIs, public data).
Step-by-Step:
- Generate a Key: In your API dashboard, create a new key.
- Test It:
Open EchoAPI, paste your endpoint, add the key to headers, and hit send. If you get a 200 OK, you’re golden!
Send It: Add it to your request header:
curl -H "X-API-Key: your_key_here" https://api.example.com/data
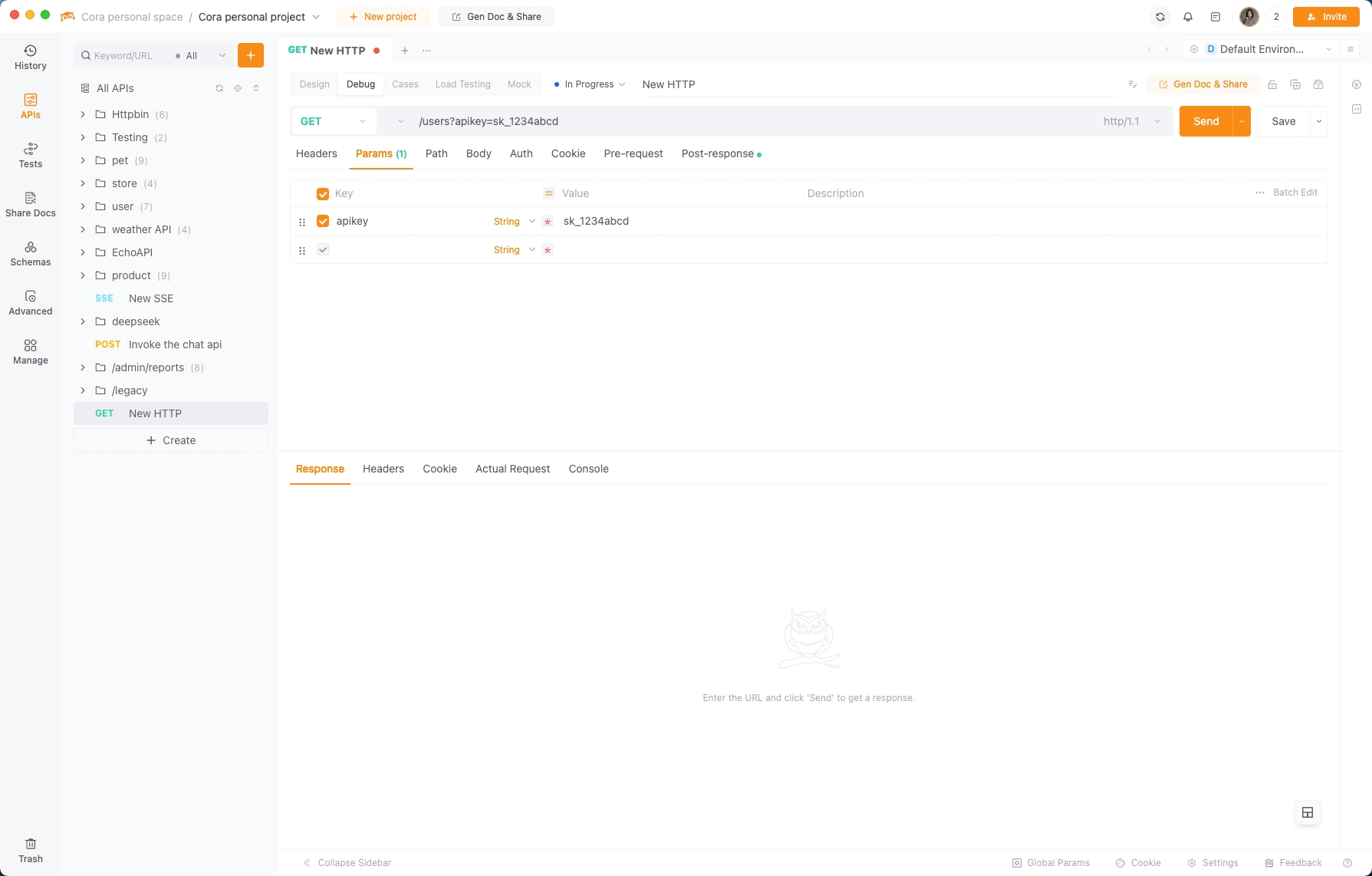
Gotchas:
- Never expose keys in client-side code (hello, GitHub leaks!).
- Rotate keys regularly.
2. OAuth 2.0: The VIP Bouncer
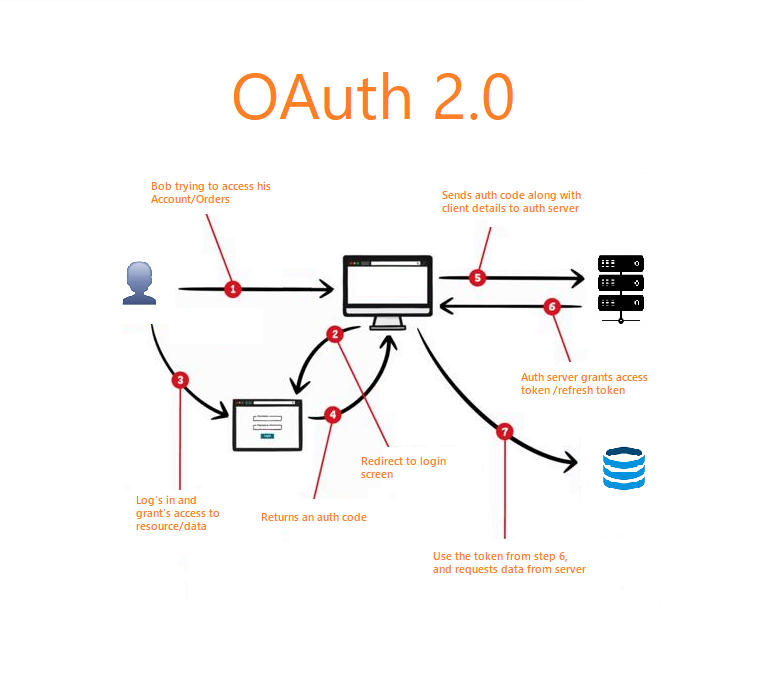
What it is: A protocol for delegated access. Think “Login with Google” but for machines.
Use case: When third-party apps need limited access without sharing passwords.
How It Works (Simplified):
(1). Get a Client ID/Secret: Register your app with the API provider.
(2). Redirect to Auth Server: Users log in and approve access.
(3). Grab the Token: The provider gives you a short-lived access token.
Try It with EchoAPI:
(1). In EchoAPI, select the OAuth 2.0 tab.
(2). Paste your auth URL, token URL, client ID, and secret.
(3). Click “Authorize”—EchoAPI handles the redirects and token storage. Now you can call protected endpoints seamlessly.
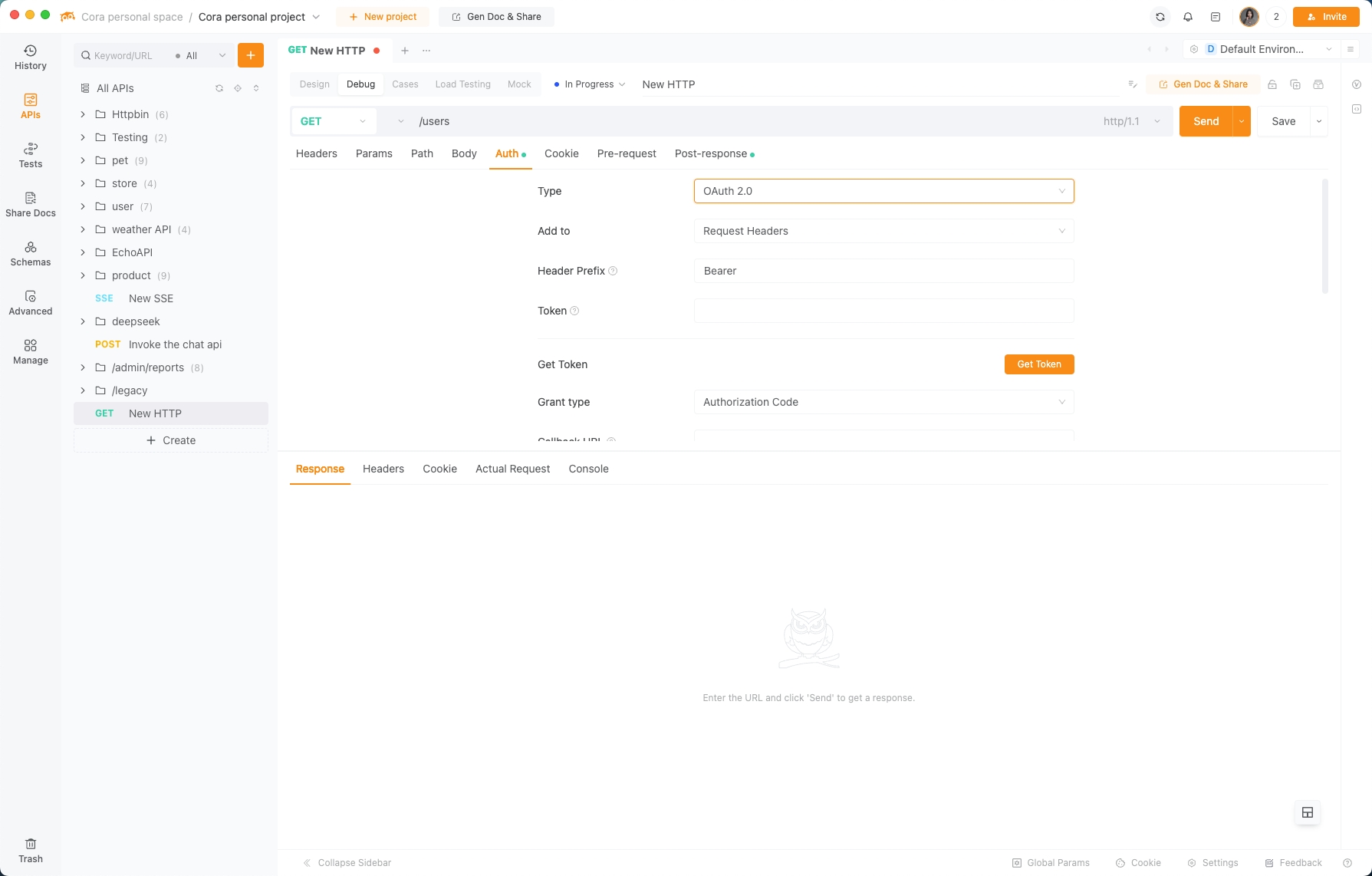
Pro Tip: Use PKCE for mobile/native apps to avoid secret leakage.
3. JWT (JSON Web Tokens): The Self-Sufficient Passport
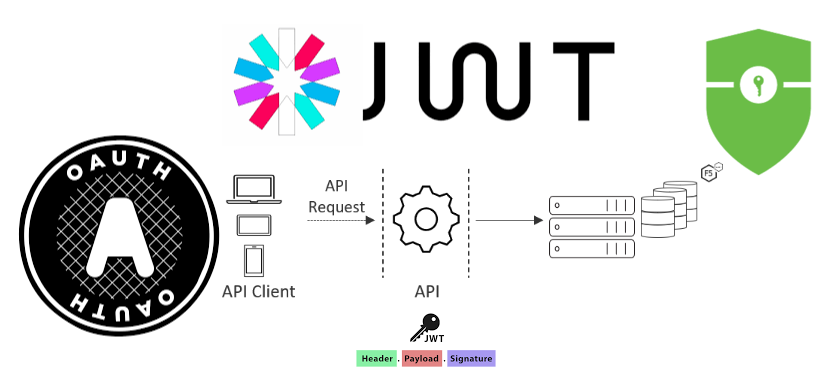
What it is: A compact, URL-safe token (e.g., xxxx.yyyy.zzzz
) that contains user data and a signature.
Use case: Stateless authentication (great for microservices!).
Build a JWT in 3 Steps:
(1). Header: Specifies algorithm (e.g., HS256).
(2). Payload: Holds claims like user_id
or exp
(expiration time).
(3). Signature: Hashes the header + payload with a secret key.
Example Token:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
Verify with EchoAPI:
(1). Send a login request to get a JWT.
(2). In EchoAPI, add the token to the Authorization: Bearer <token>
header.
(3). Test an endpoint—EchoAPI automatically validates the token’s expiry and signature.
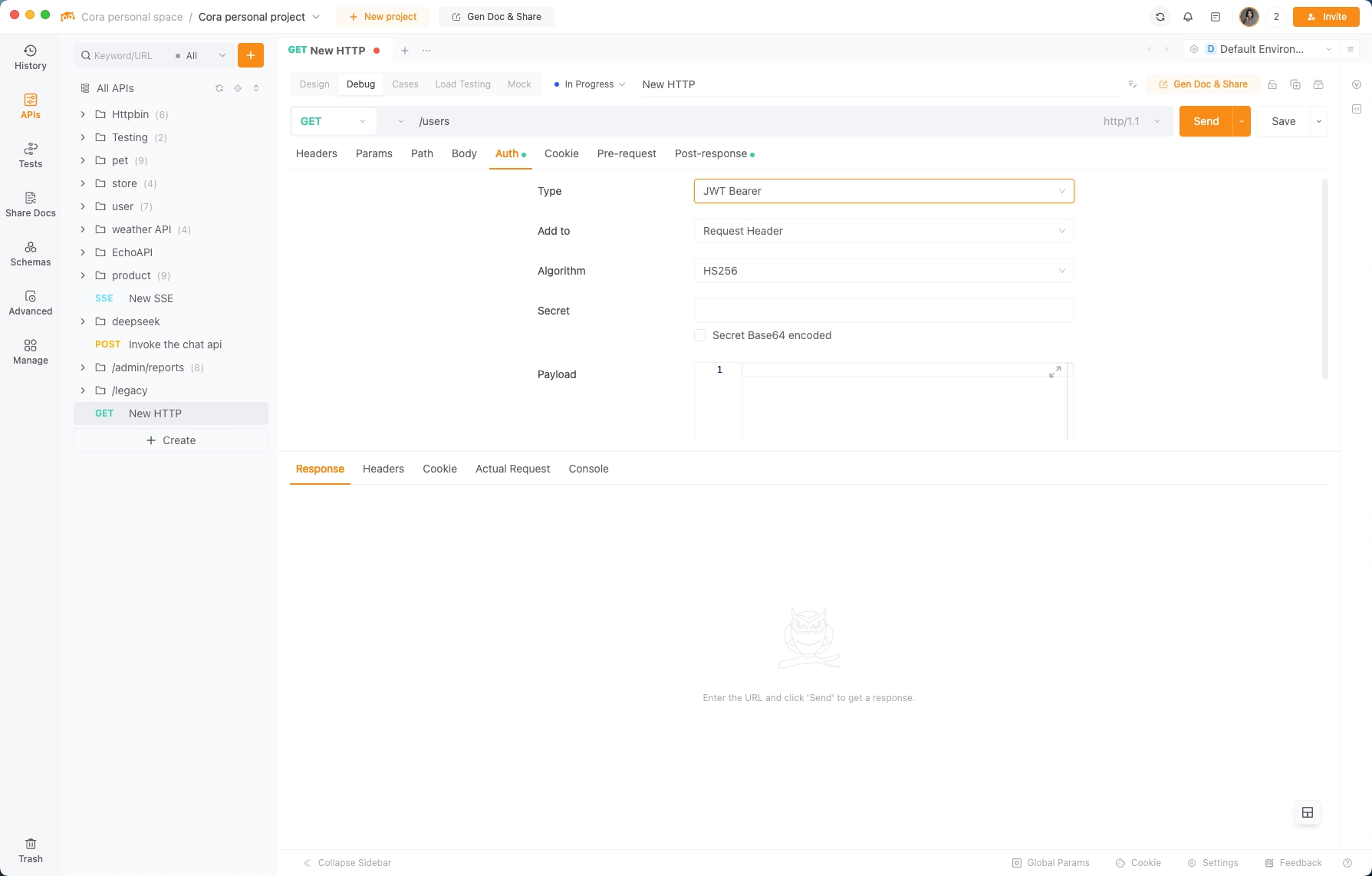
Why JWT Rocks: No database lookups! The token IS the proof.
Closing: Your Authentication Cheat Sheet
Let’s recap:
- API Keys: Easy but fragile. Use for internal/low-risk tasks.
- OAuth 2.0: The gold standard for third-party access. Let EchoAPI handle the heavy lifting.
- JWT: Perfect for scalable, stateless systems. Validate tokens in seconds with tools like EchoAPI.
The Bottom Line: Authentication isn’t optional—it’s your API’s immune system. And just like you wouldn’t skip a flu shot, don’t skip testing. Tools like EchoAPI turn complex auth workflows into a 3-click process, letting you focus on building features instead of fighting fires.
Now go lock that API door. (But leave a key under the mat for EchoAPI.)