API Design Secrets: How to Build Endpoints Developers Actually Want to Use (Hint: It’s Not Just About Code)
Great API design isn’t about writing perfect code. It’s about creating a developer experience so smooth that your endpoints become your best sales team.
Let’s start with a brutal truth: Most APIs suck.
You’ve probably felt it yourself – endpoints that behave like riddles, documentation that reads like hieroglyphics, and error messages that say "Something went wrong" (thanks, Captain Obvious). But here’s the kicker: bad API design isn’t just annoying – it’s a business killer.
Think I’m exaggerating?
- Netflix’s API failures once caused 3 days of streaming outages (cost: millions).
- Twitter’s infamous "rate limit" fiasco drove developers to competing platforms overnight.
- Even Google’s early Maps API missteps sparked mass migraines (and migration).
Now here’s the twist: Great API design isn’t about writing perfect code. It’s about creating a developer experience so smooth that your endpoints become your best sales team. Let’s flip the script.
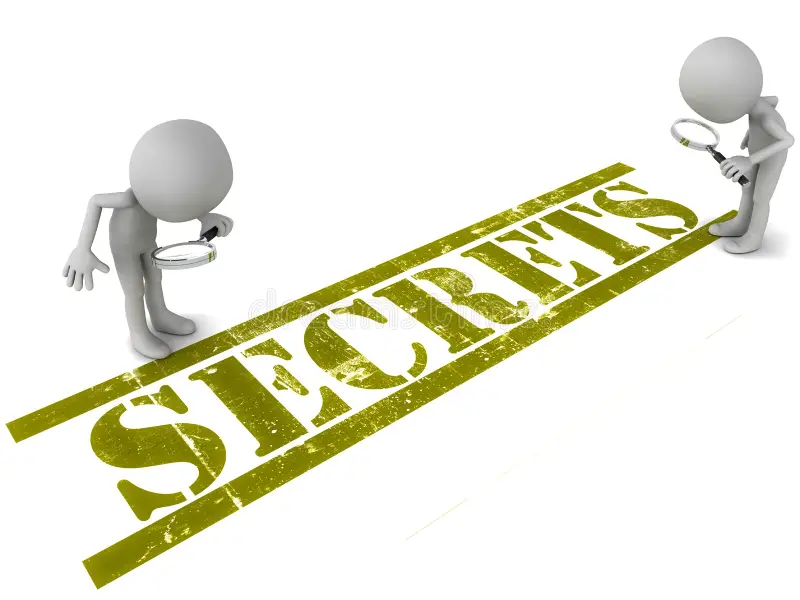
Step 1: Speak REST Fluently (But Don’t Be a Purist)
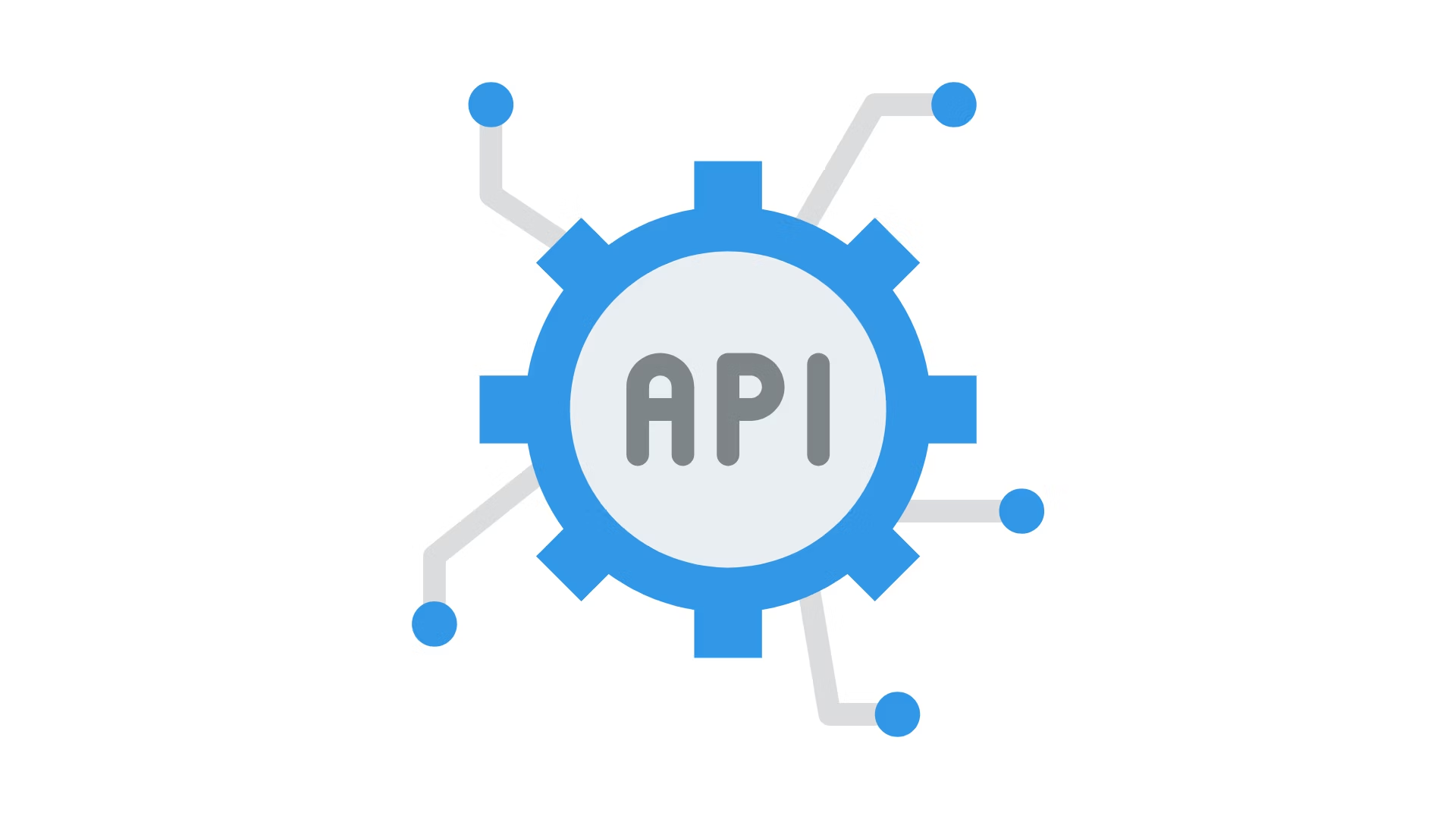
Problem: Developers expect REST conventions – break them at your peril.
Solution:
- Nouns > Verbs:
/users/123
NOT/getUserById
- HTTP Verbs Matter:
- GET = Read
- POST = Create
- PUT = Full update
- PATCH = Partial update
- DELETE = Obvious
- Status Codes Are Your Secret Weapon:
- 200 OK (Success)
- 201 Created (New resource)
- 400 Bad Request (You messed up)
- 401 Unauthorized (Login first)
- 429 Too Many Requests (Slow your roll)
Step 2: Version Like a Time Traveler
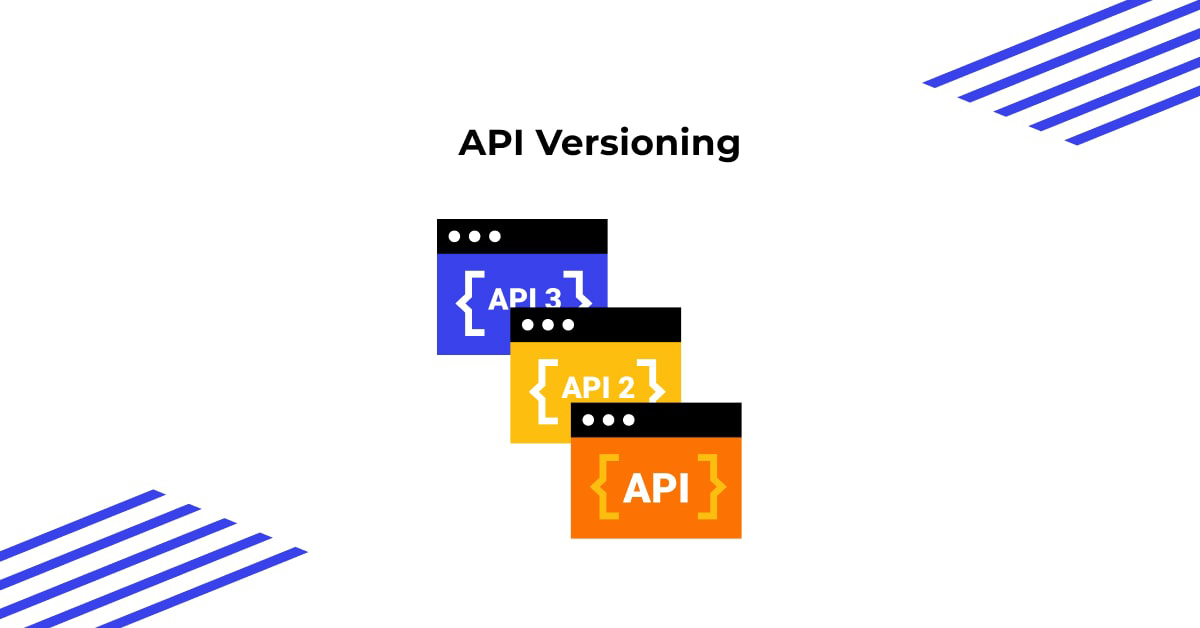
Problem: Updates break integrations → Developers hate you.
Solution:
- URL Versioning:
/api/v2/users
(Clear but clunky) - Header Versioning:
Accept: application/vnd.yourapi-v2+json
(Clean but obscure) - Golden Rule: Never sunset versions without a 12-month warning.
Step 3: Error Messages That Don’t Make You Want to Scream
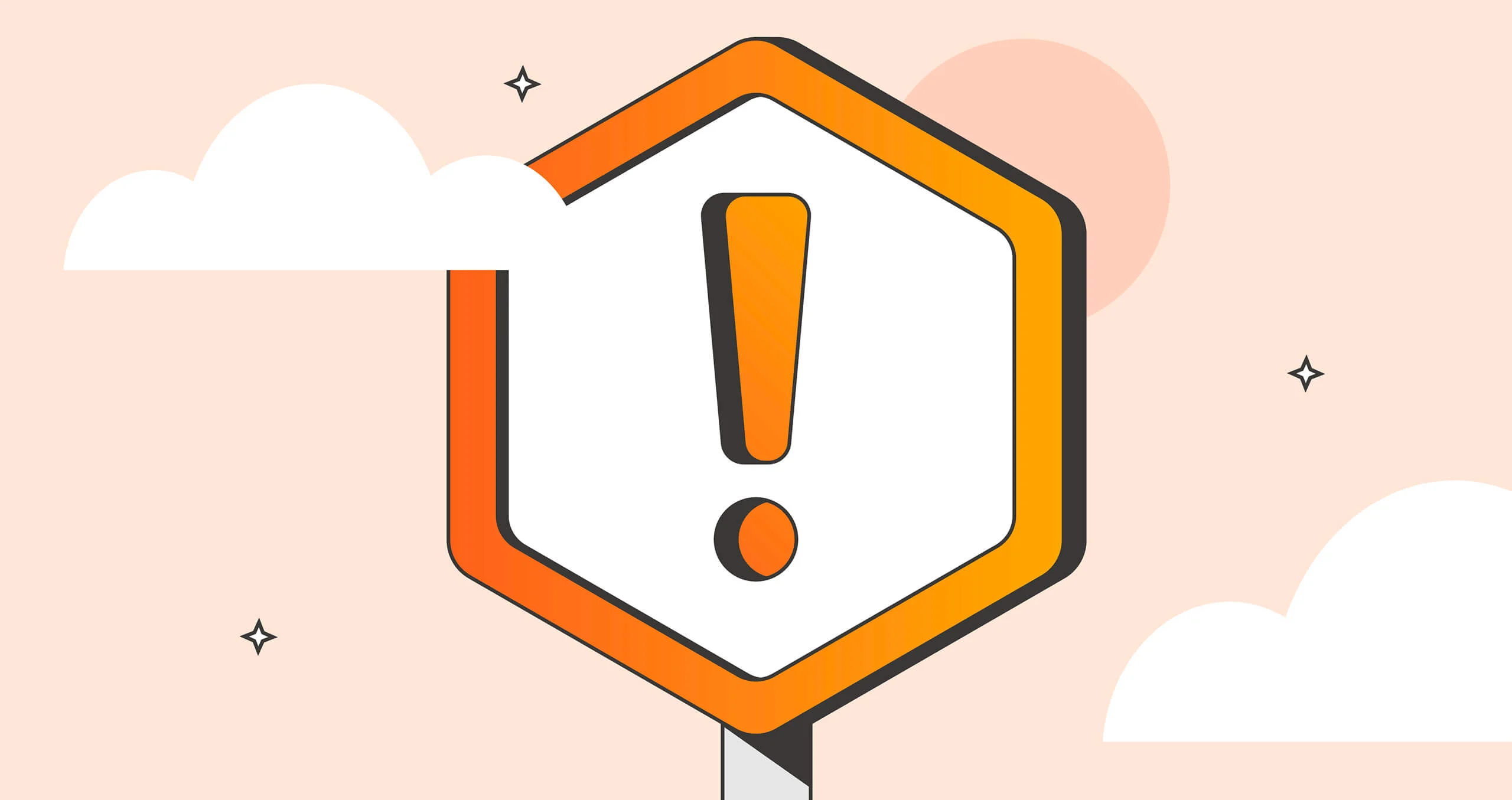
Bad Example:
{ "error": "Invalid request" }
(Translation: "Figure it out, genius.")
Good Example:
{
"error": {
"code": "VALIDATION_FAILED",
"message": "Email format invalid",
"field": "user.email",
"docs": "https://api.yourservice.com/errors#VALIDATION_FAILED"
}
}
Step 4: Documentation That Doesn’t Put People to Sleep
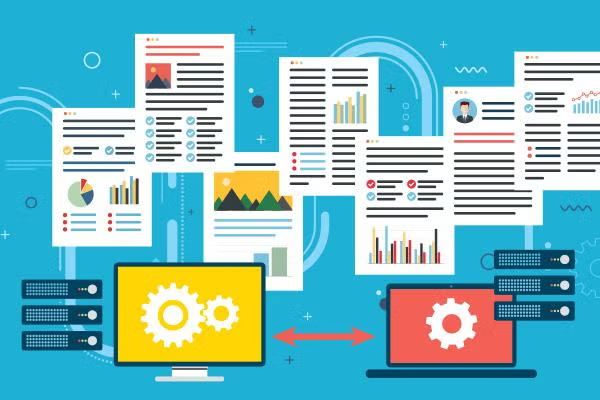
Forget 50-page PDFs. Your docs should be:
- Interactive: Let users test endpoints right in the browser (EchoAPI’s Live Docs does this automatically).
- Searchable: Ctrl+F friendly with clear section headers.
- Human Examples:
Bad: "Utilize the POST verb to initiate resource creation."
Good: "Want to create a user? Send this:"
curl -X POST https://api.yourservice.com/users \
-H "Content-Type: application/json" \
-d '{"name": "Alice", "email": "alice@example.com"}'
Step 5: Test Like the World Depends on It (Because It Does)
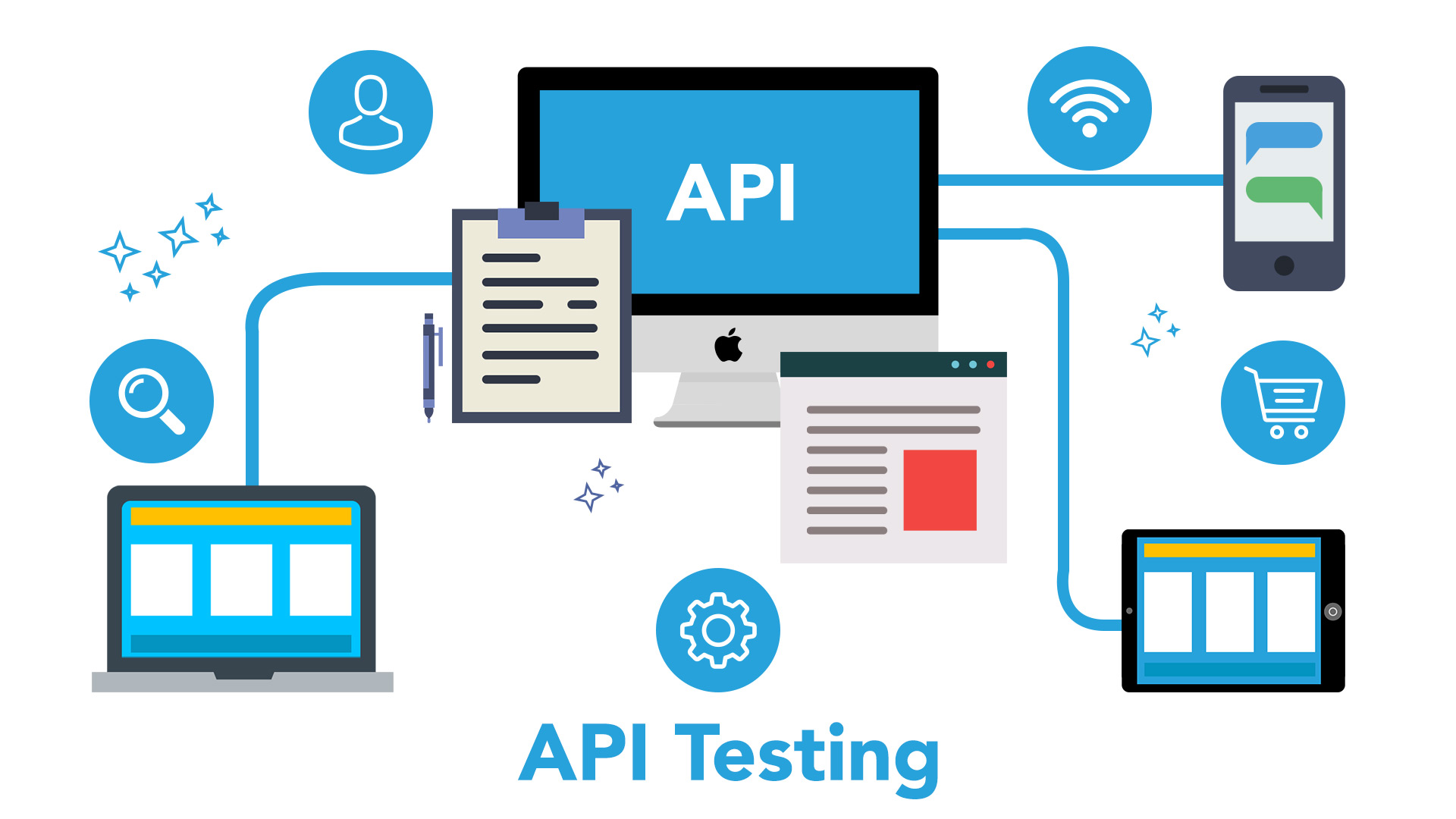
Critical Tests:
- Edge Cases: Empty inputs, max payload sizes, weird characters
- Rate Limits: Does your API throttle properly?
- Security: Inject bad data (SQL, XSS) – does your API sanitize inputs?
Conclusion
Let’s recap the big wins:
✅ RESTful = Predictable (but stay pragmatic)
✅ Versioning = No Surprises
✅ Errors = Actionable Intel
✅ Docs = Immediate Clarity
✅ Testing = Sleep at Night
The pattern? Empathy. Every design choice should answer: "Would this make a developer’s life easier?"
And here’s your unfair advantage: Tools like EchoAPI automate the grunt work – validation, testing, docs – so you can focus on the big picture.
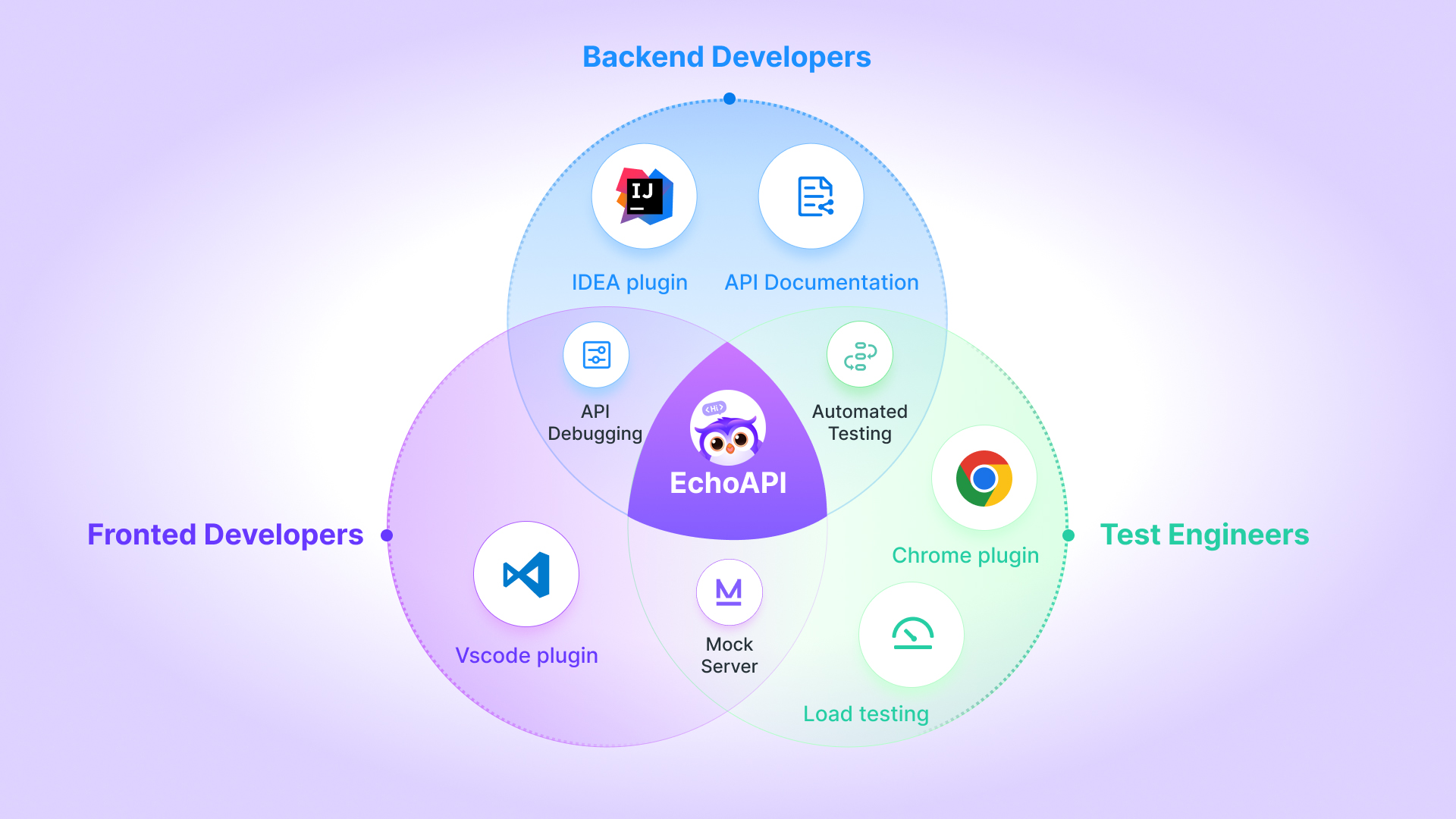
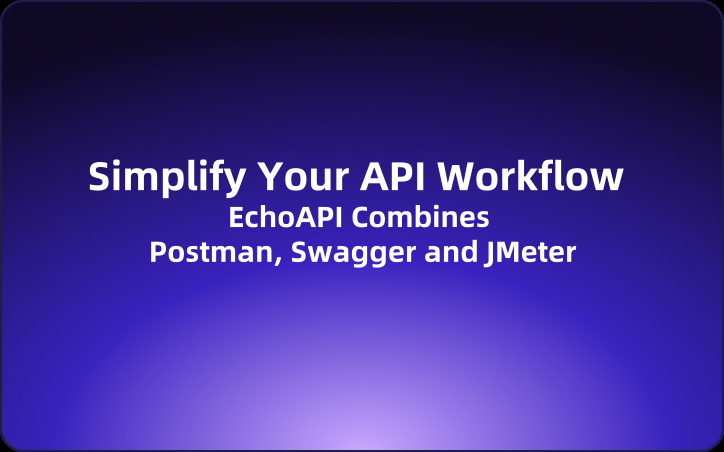
Final Thought: Your API isn’t just code. It’s a handshake with developers. Make it firm, clear, and worth remembering.
Now go design something that doesn’t suck.