API Security 101: Why "It Works on My Machine" Isn't Good Enough (And How to Fix It)
This article outlines the fundamentals of security and provides concrete steps to avoid common pitfalls.
API security is crucial in today’s technological landscape. This article outlines the fundamentals of security and provides concrete steps to avoid common pitfalls.
The Silent Disaster Every Developer Ignores
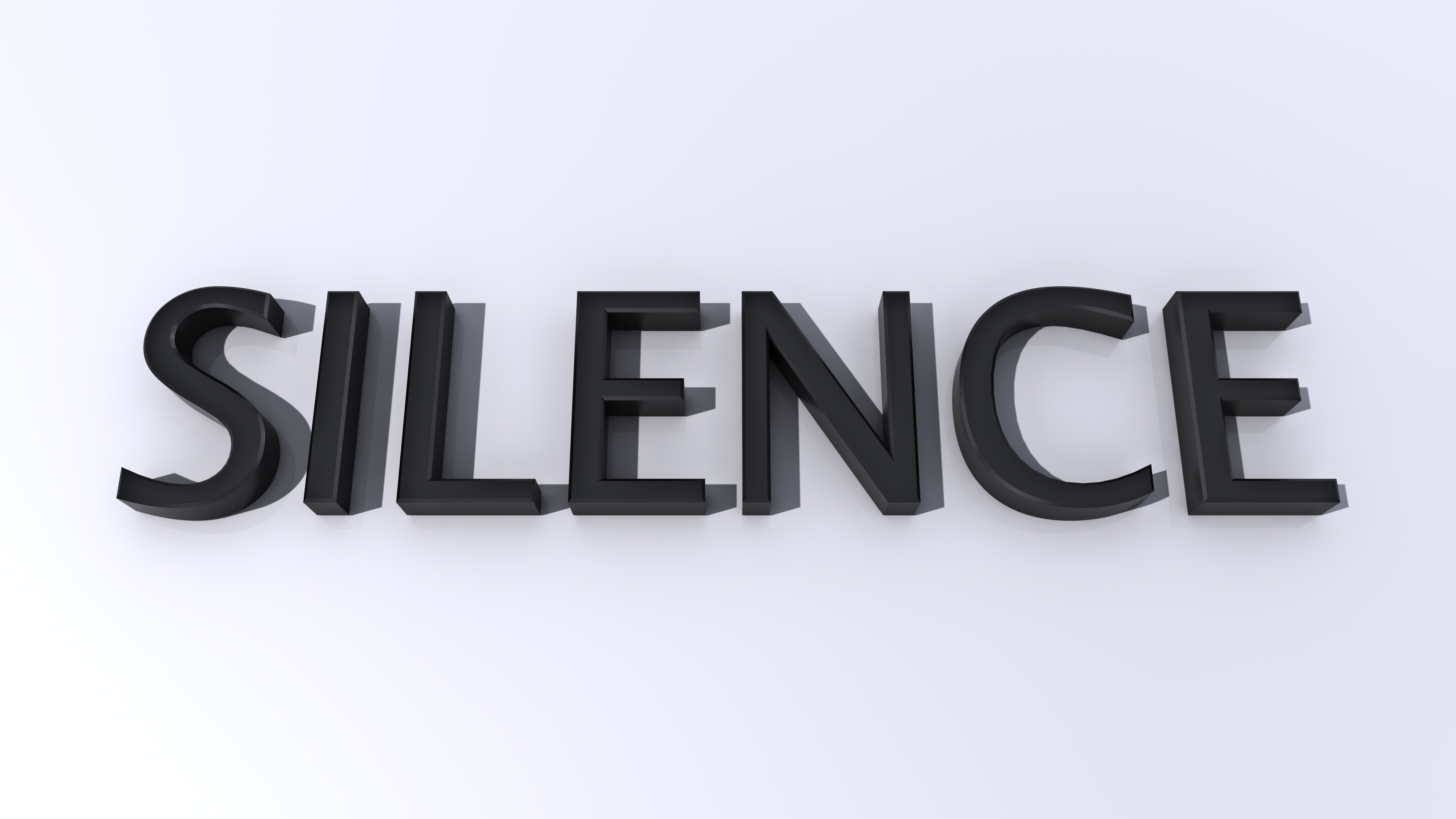
You just built a sleek new API. It passes all your unit tests. It works flawlessly on Postman. You deploy it, high-five your team, and move to the next sprint.
Here’s the problem:
Your API is already compromised.
While you were celebrating, hackers are running automated scripts that found:
- An unauthenticated
/admin
endpoint - User IDs exposed in error messages
- Rate limits set to "generous" (read: nonexistent)
This isn’t paranoia. 83% of web traffic is API calls, and 34% of companies suffered API breaches last year (Salt Security, 2023). The scariest part? You won’t know until it’s too late.
APIs aren’t just code – they’re front-line soldiers in a cyberwar. Treat them like pet projects, and you’re handing attackers a skeleton key to your entire system.
Locking Down APIs Like a Pro (Even If You’re Just Starting Out)
Step 1: Authentication ≠Authorization (And Why Both Matter)
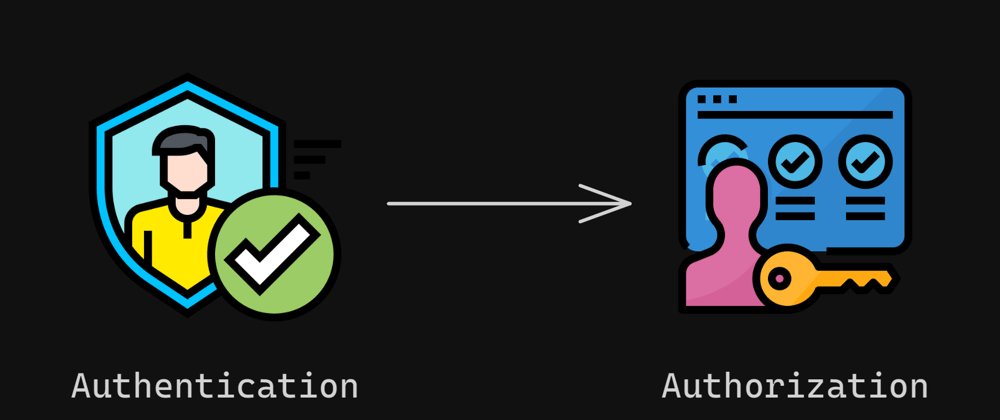
Authentication answers "Who are you?"
Authorization answers "What can you do?"
The Fix:
- Use JWT (JSON Web Tokens) with short expiration times
- Implement OAuth 2.0 for third-party access
- Never trust client-side validation
# Bad: Trusting client role claims
user_role = request.json.get('role')
# Good: Server-side check with EchoAPI
# (EchoAPI automatically validates JWT signatures and scopes)
user_role = EchoAPI.get_verified_claims(request)['role']
Step 2: Input Validation: Assume Everything’s Poisoned
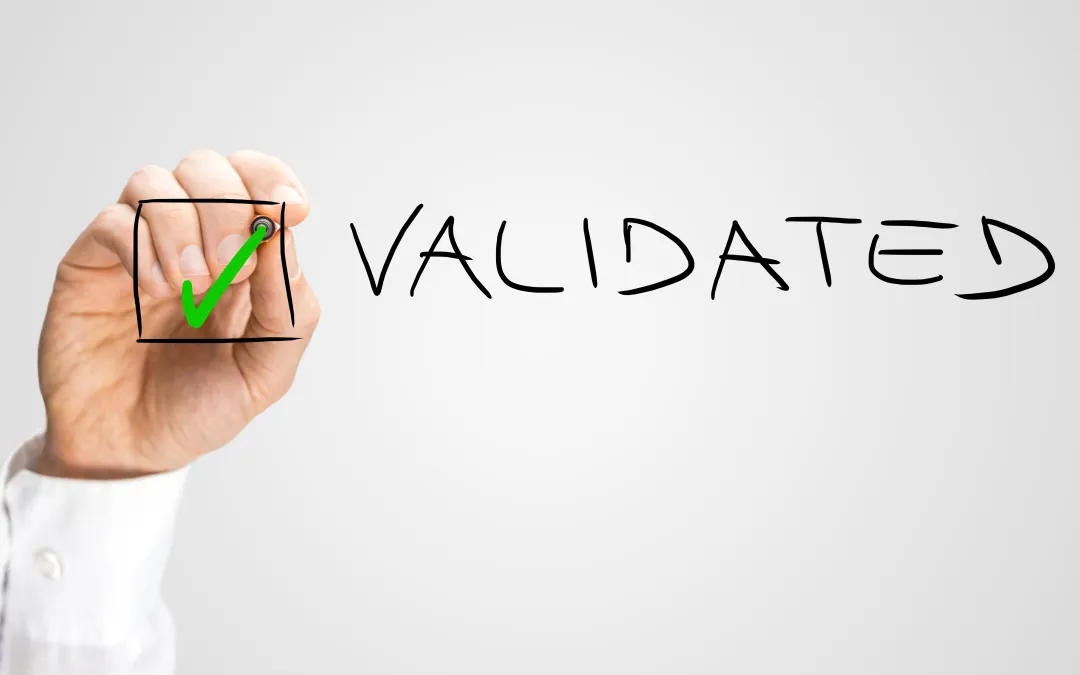
That innocent-looking POST request? It’s a Trojan horse.
Defense Tactics:
- Use strict schema validation (JSON Schema > 90% of attacks)
- Sanitize all inputs – yes, even "read-only" fields
- Block unexpected content types
# Test payloads fast with Attack Simulator
$ echoapi test --endpoint /checkout --attack SQLi,XSS
â–ş Found 2 critical vulnerabilities:
- SQL injection via 'user_id' parameter
- Cross-site scripting in 'notes' field
Step 3: Rate Limiting: Stop Brute-Force Tsunamis
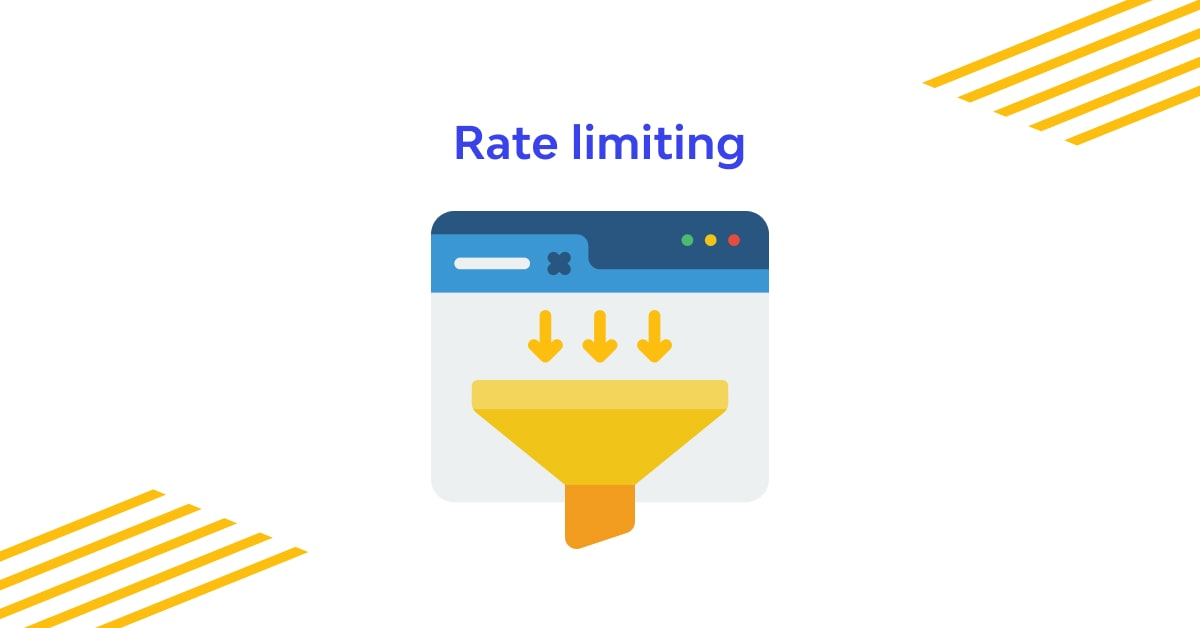
APIs without rate limits are like ATMs without withdrawal caps.
Implement:
- IP-based throttling
- Token bucket algorithm for smooth traffic
- Granular limits per endpoint (e.g.,
/login
vs/profile
)
Step 4: Encryption: More Than Just HTTPS
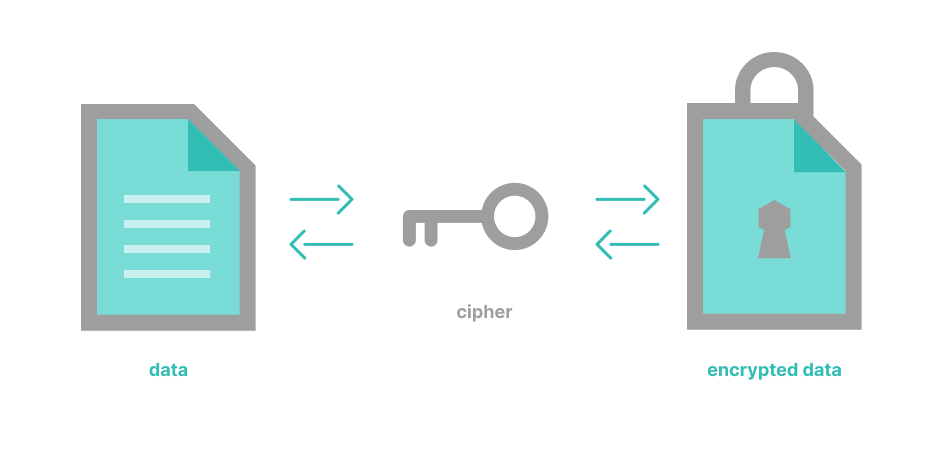
SSL is table stakes. Real security needs:
- HMAC signatures for critical requests
- Encrypted payloads for sensitive data
- Certificate pinning in mobile SDKs
Pro Tip:
Use EchoAPI’s Auto-Documentation feature to:
- Generate interactive API specs
- Flag undocumented endpoints (common hacker targets)
- Share secure test environments with clients
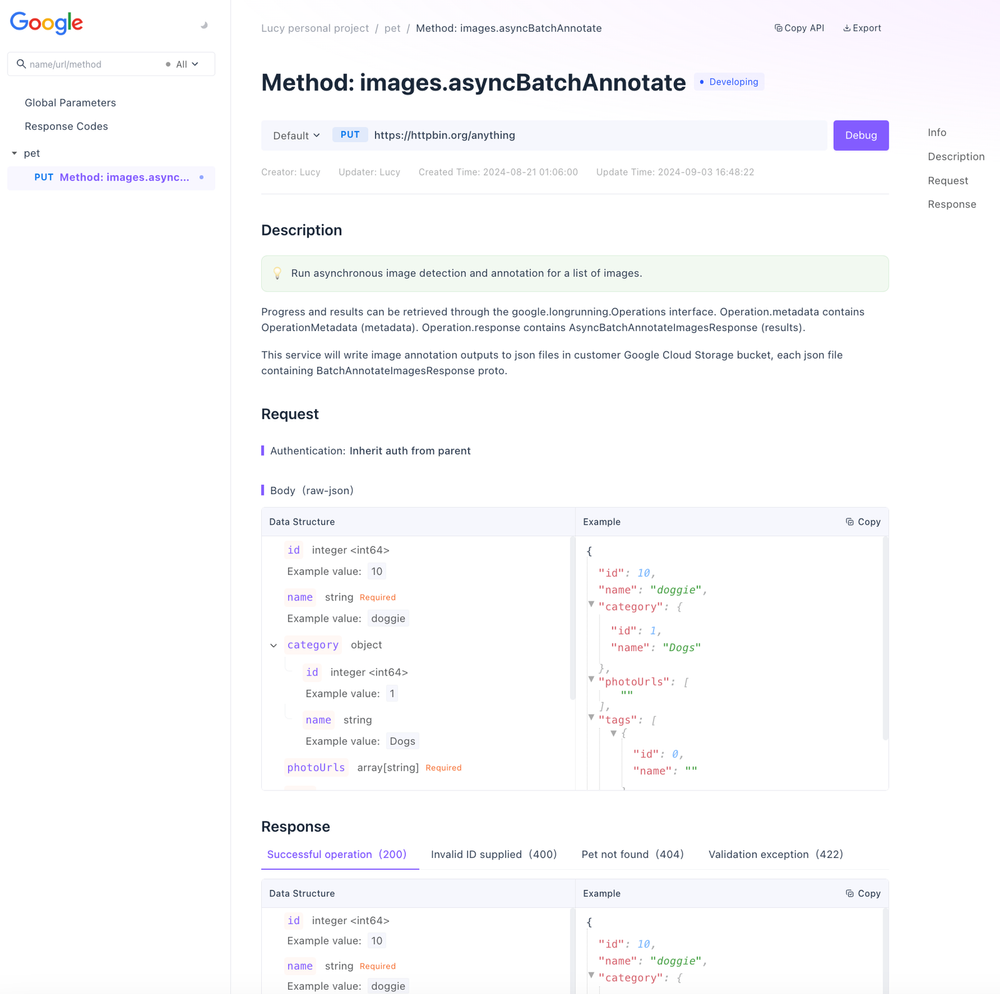
Security Isn’t a Feature – It’s the Foundation
APIs live in the wild west of the internet. Every unvalidated parameter is a potential ransom note. Every missing CORS header is an invitation to hijack sessions.
Key Takeaways:
- Verify twice, trust never: Assume all incoming data is hostile
- Automate defenses: Tools like EchoAPI turn security from a chore to a checklist
- Monitor relentlessly: 76% of breaches exploit existing vulnerabilities (IBM Cost of Data Breach 2023)
Final Reality Check:
Your API will get attacked. The only question is whether it’ll be a minor skirmish or a front-page data breach.
Next Steps:
- Audit your APIs with EchoAPI
- Implement the 4 defenses above (takes <2 hours with proper tooling)
- Sleep soundly knowing your endpoints aren’t the low-hanging fruit
Because in API security, "good enough" is the enemy of "still in business."