API Testing Made Easy: How to Stop Drowning in Code and Start Surfing on JSON
Most developers spend more time debugging API tests than writing actual code.
Let’s rip off the Band-Aid: 90% of developers hate API testing.
Wait, what? Isn’t API testing just sending a few HTTP requests and checking if you get a 200 OK? That’s what the tutorials say! But here’s the dirty secret nobody tells you: Most developers spend more time debugging API tests than writing actual code. You’re not testing APIs—you’re babysitting cURL commands, wrestling with Postman environments, and praying your Swagger docs don’t lie.
But what if I told you API testing could be as simple as ordering pizza? That instead of drowning in scripts, you could automate 80% of the work with tools so intuitive, even your non-tech co-founder could use them?
Spoiler: You’ve been overcomplicating this. Let’s fix that.
The Lazy Developer’s Guide to Painless API Testing
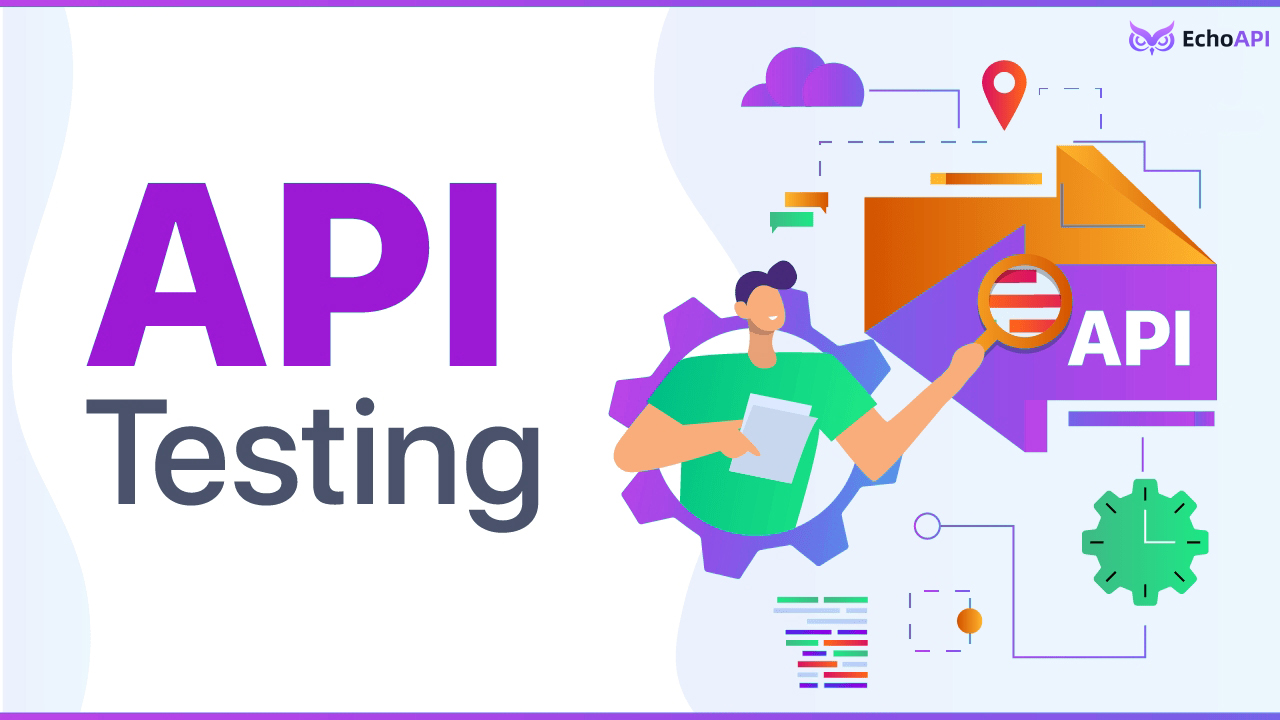
Step 1: Ditch cURL (Yes, Seriously)
cURL is the duct tape of API testing—it works, but nobody wants to admit how ugly it gets. Instead, use a tool designed for humans.
Meet EchoAPI: Think of it as the “Netflix of API testing.” No installations, no confusing setup. Just open your browser, paste your endpoint, and go. Here’s how to test a GET request in 10 seconds:
- Go to EchoAPI’s live demo.
- Paste your API URL (e.g.,
https://httpbin.org/anything
). - Click Send.
- Boom—you’ll see the response, status code, and even a timeline of the request.
No JSON formatting. No terminal commands. Just results.
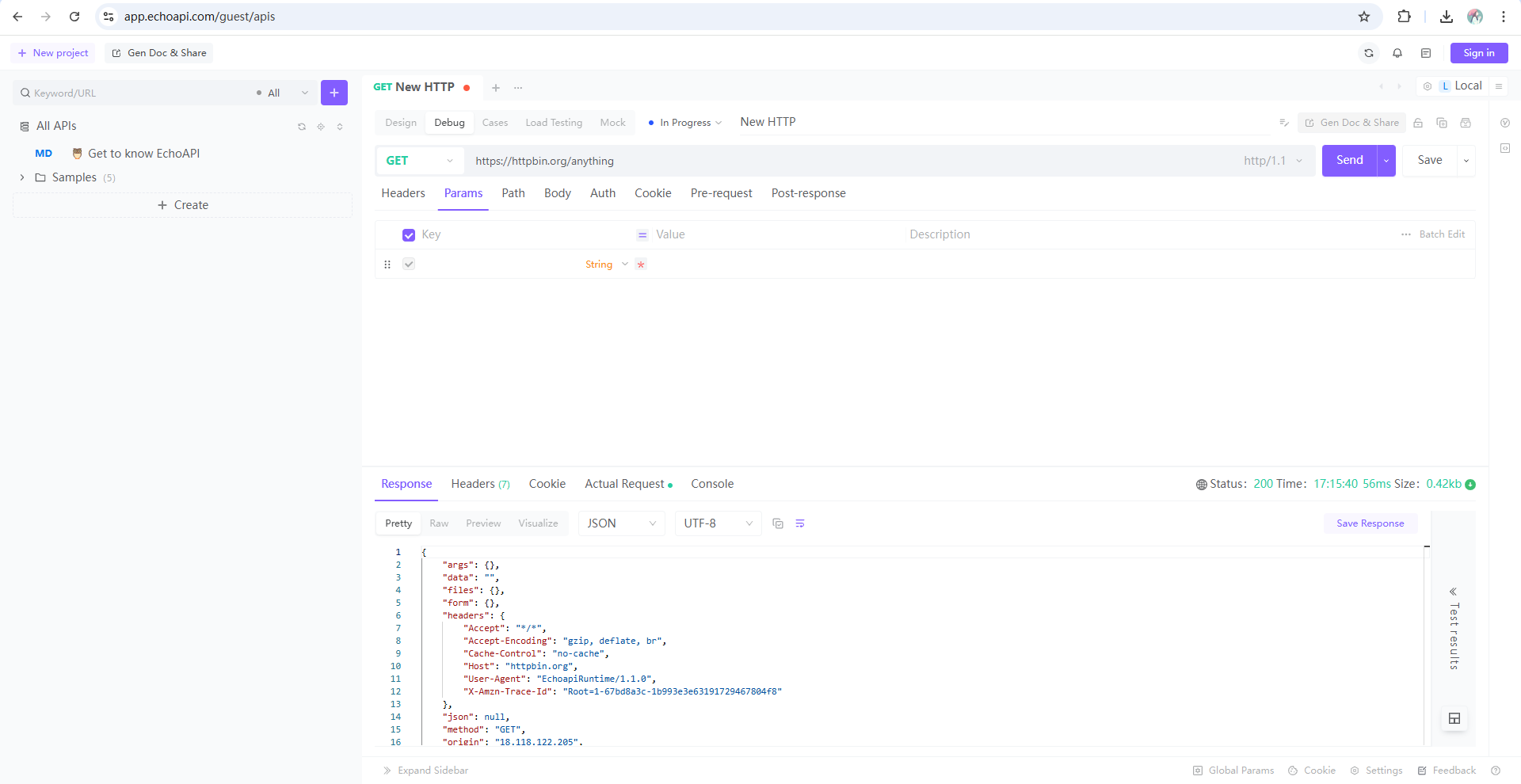
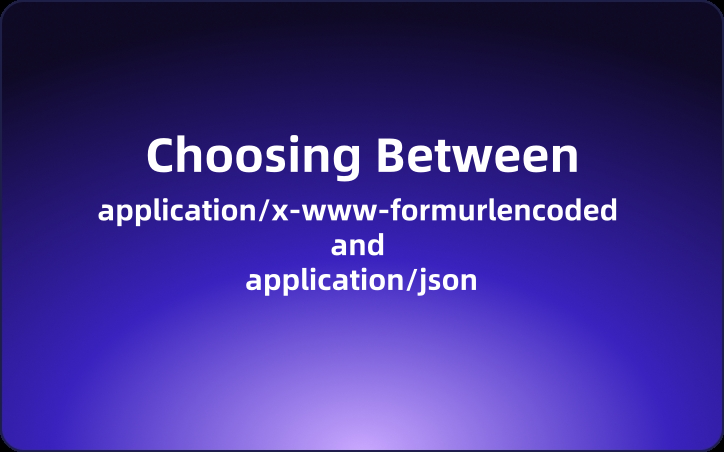
Step 2: Automate the Boring Stuff
Manual testing is for masochists. Let’s automate validation with schema checks.
Example: You’re testing a /users
endpoint that should return a list of users with id
, name
, and email
. Instead of eyeballing the response, write a 1-line assertion in EchoAPI:
expect(response.json.data).to.include.all.keys(['id', 'name', 'email']);
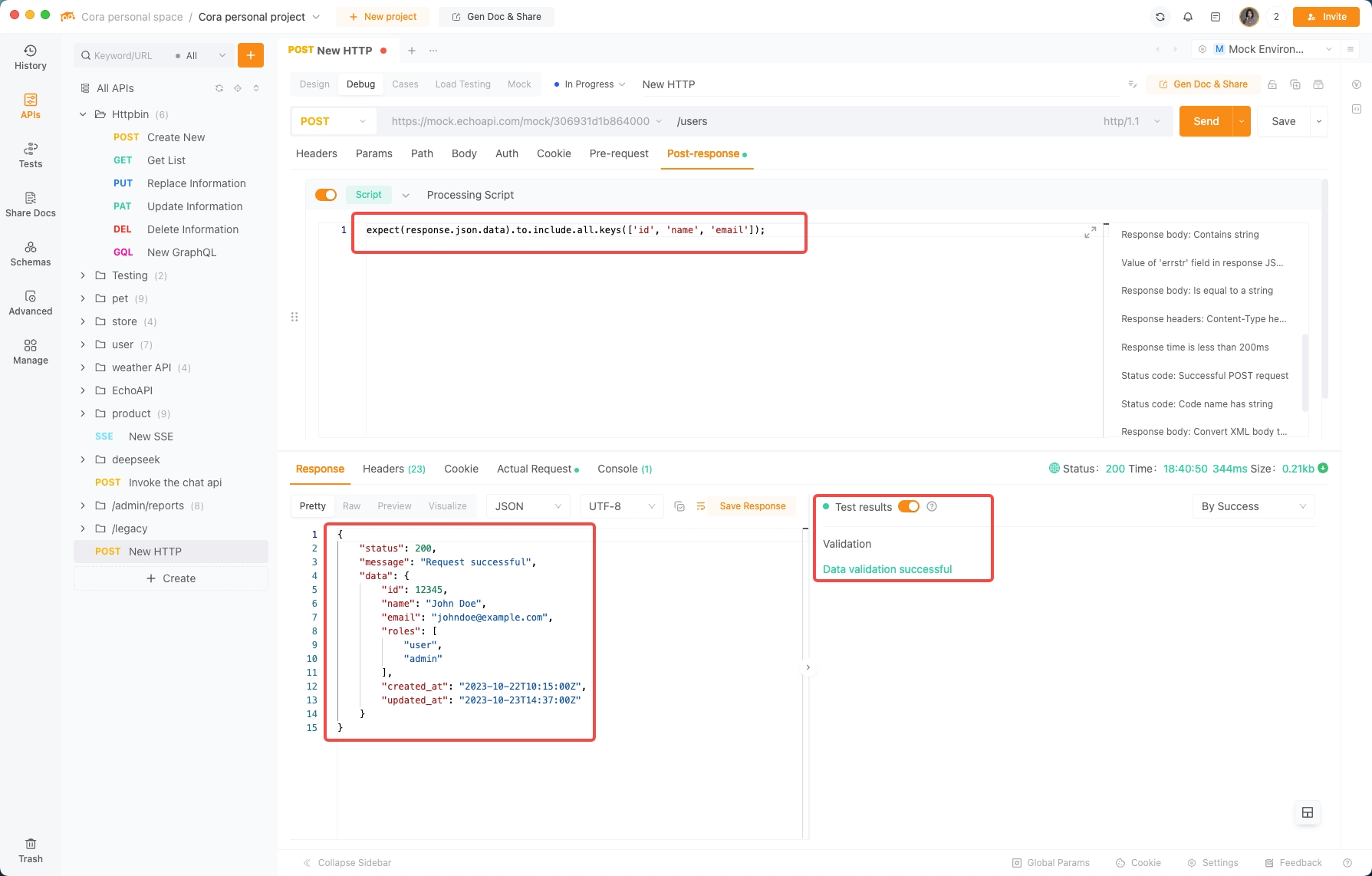
If the API returns a user without email
, EchoAPI slaps a big red “FAIL” on your screen. Done.
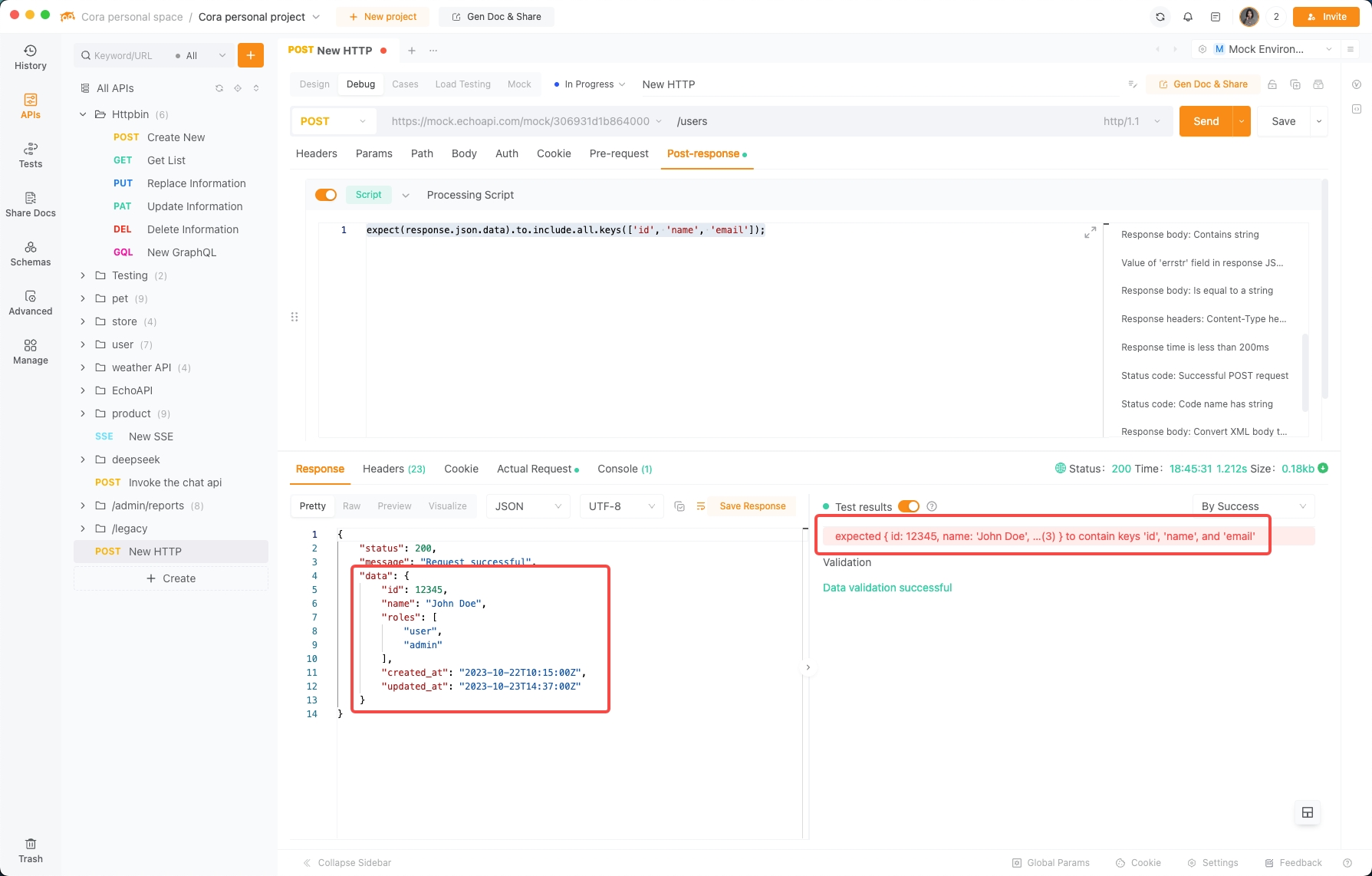
Step 3: Mock APIs Like a Pro
Waiting for the backend team to finish the API? Stop. Mock it.
In EchoAPI:
- Click Design in the sidebar.
- Define your endpoints and sample responses (e.g.,
GET /users
âž” returns 3 test users). - Share the mock URL with your team.
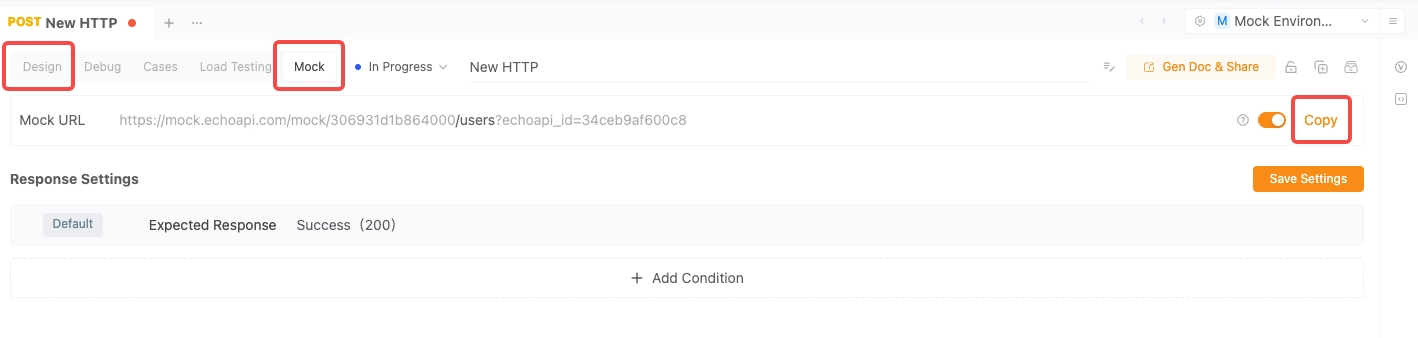
Now your frontend can work today, even if the real API is still in development purgatory.
Step 4: Debug Failures Without Losing Your Mind
Your test failed. Now what? Most tools give you a vague error like "Unexpected token '}'".
EchoAPI’s Time Travel Debugger shows you:
- The exact request headers sent
- The millisecond-by-millisecond breakdown
- A diff between expected and actual responses
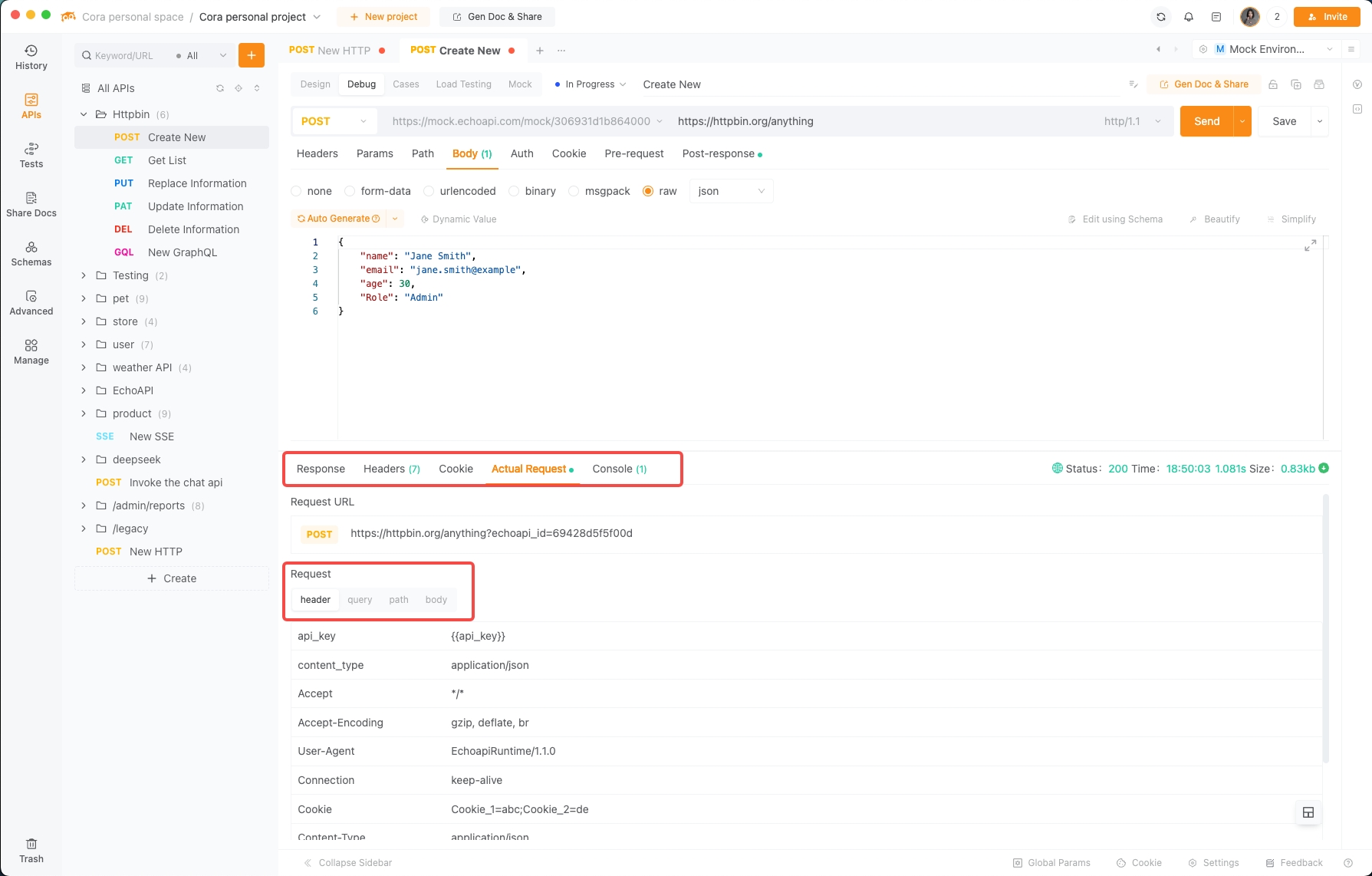
No more guessing why your PUT
request turned into a 404.
API Testing Isn’t Hard—You’re Just Using the Wrong Tools
Let’s recap:
- Stop using Stone Age tools (like cURL).
- Automate validation instead of playing “spot the typo” in JSON.
- Mock early to unblock your team.
- Debug visually—because nobody likes stack trace archaeology.
The bottom line? API testing isn’t supposed to hurt. Tools like EchoAPI exist to turn a 2-hour testing nightmare into a 5-minute task.
Your Homework:
- Download EchoAPI.
- Next time you test an API, ask: “Am I overcomplicating this?”
- Automate one test this week.
Remember: The best developers aren’t the ones who write the most code—they’re the ones who delete the most. Start deleting unnecessary complexity today.