Beginner’s Guide to Master Pre-Scripts in API Testing: Save Time, Tokens, and Hassle
Mastering pre-scripts in API testing is like having a blueprint for your digital construction. This guide helps you save time, reduce errors, and streamline your testing process, ensuring every API call is mission-ready.
You’re a LEGO master architect.
You’ve been chosen to build the most epic LEGO city ever — skyscrapers, flying cars, magical roads, even a self-driving train that loops around a mountaintop.
Sounds like a dream job, right?
But hold on...
Would you seriously just dump all the bricks on the floor and start stacking at random?
No way.
Before you even snap the first two pieces together, you'd need:
- A blueprint that shows what goes where
- A map for roads, bridges, and tunnels
- A color scheme (no one wants a purple police station)
- And a power plan so the train actually runs
Because without that, your LEGO dream city turns into a wobbly mess of half-built blocks and lost wheels.
API testing is exactly the same.
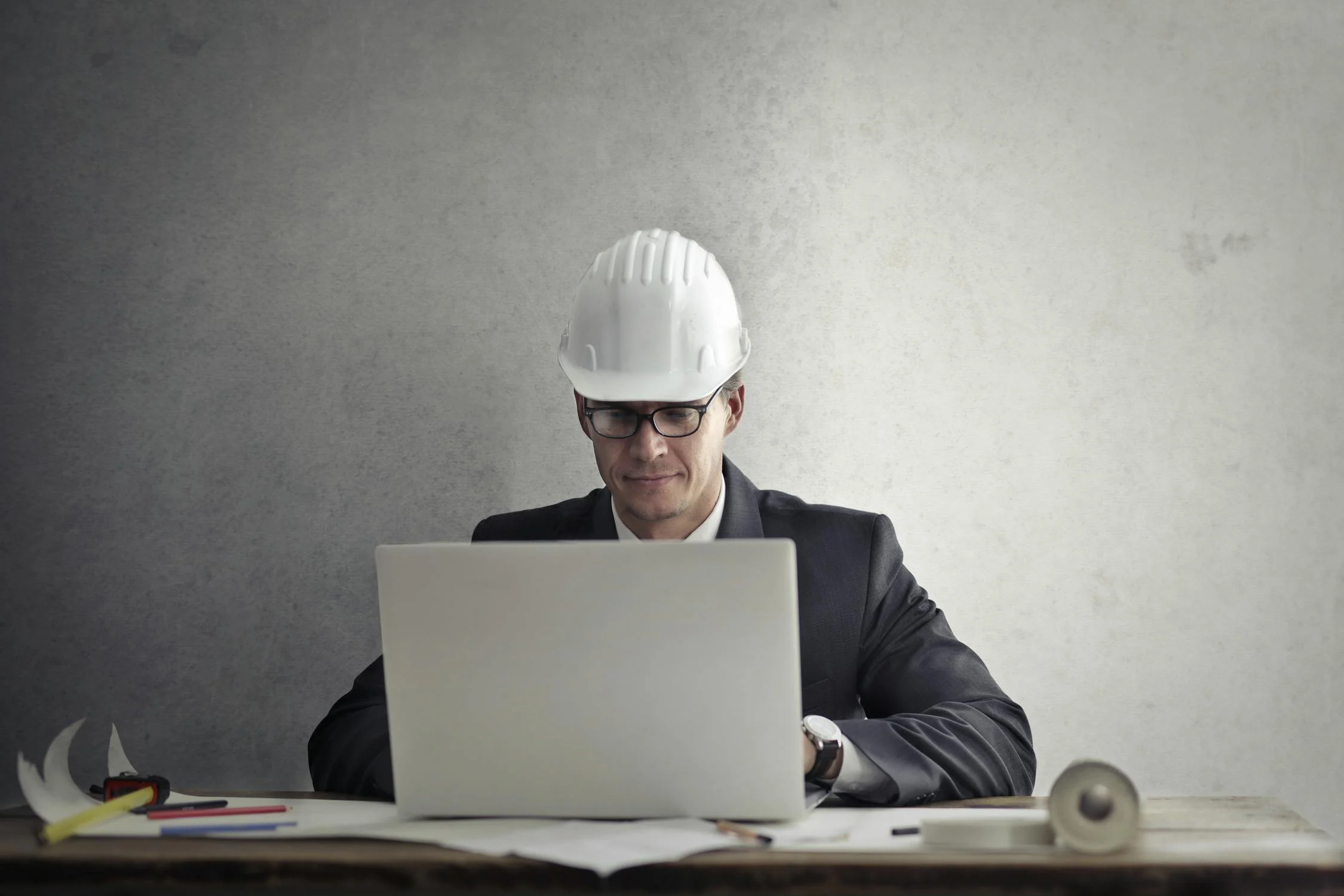
You’ve got endpoints (your LEGO pieces), environments (your building zone), tokens (like the electricity), and test logic (your map).
If you jump into testing without a plan, things get messy. Fast.
Variables go missing, tokens expire, headers are wrong, and you end up doing the same setup over and over again.
That’s where a pre-script comes in.
It’s the blueprint that sets up your testing city.
It makes sure everything is in the right place — before your tests begin.
So your requests aren’t just flying blind; they’re fully geared up, mission-ready, and built to scale.
The LEGO City (aka Your API Testing) Needs a Plan
You’re building this massive, intricate LEGO city. Every piece is important, every road needs to connect, and every building needs power to function. Without a plan, you’re just stacking bricks randomly, hoping it all fits together. The same thing happens in API testing if you skip the pre-script.
Testing APIs without pre-scripts is like:
- Forgetting to set up authentication tokens
- Repeating setup steps in every single test case
- Manually setting headers or parameters in every request
It's frustrating and time-consuming, and trust me, it's not the most effective way to get things done.
How to Set the Foundation (the Pre-Script)
Now, what’s your task here? Easy! Before you even touch the first API endpoint, you need to set up a clean, repeatable foundation.
Your mission:
- Define variables, such as authentication tokens, user IDs, or environment-specific settings.
- Set global configurations, like headers or paths, that apply across all your tests.
- Automate repetitive tasks, so you're not manually entering the same setup info for each API call.
Think of it like a pre-show checklist: Make sure all the actors are in place, the stage is set, and the lights are on, before the show begins.
With pre-scripts, you are organizing all that behind-the-scenes magic. The train doesn’t move unless you’ve powered it up first, right?
Implement Your Pre-Script to Prepare the Testing Stage
Now here’s the fun part.
Writing your pre-script means getting everything ready for action — kind of like warming up before a race.
Instead of repeating the same setup in every test, you write it once, and it runs before your actual API calls. Neat, right?
Let’s look at this real-world example, where we log in, grab a token, and save it so we don’t have to do it again in every test.
🧩 The Full Pre-Script
// Pre-script for EchoAPI: Automate login and token setup
if (!pm.environment.get("token")) {
pm.sendRequest({
url: "https://api.superapp.com/auth",
method: "POST",
header: {
"Content-Type": "application/json"
},
body: {
mode: "raw",
raw: JSON.stringify({
username: "apiuser",
password: "supersecret"
})
}
}, function (err, res) {
const json = res.json();
pm.environment.set("token", json.token); // Save token globally
pm.environment.set("userId", json.user.id); // Save user ID
});
}
🔍 Step-by-Step Breakdown
1️⃣ Check if a Token Already Exists
if (!pm.environment.get("token")) {
Only run the login request if there’s no token saved in the environment.
This avoids making unnecessary login calls over and over again — saving time and reducing server load.
2️⃣ Send a Login Request to the Auth Endpoint
pm.sendRequest({
url: "https://api.superapp.com/auth",
method: "POST",
header: {
"Content-Type": "application/json"
},
This sends a POST request to your API’s/auth
endpoint, asking for a token using login credentials.
Think of it like saying: “Hey server, here’s my username and password — please give me a key so I can come in.”
3️⃣ Add the Login Credentials in JSON Format
body: {
mode: "raw",
raw: JSON.stringify({
username: "apiuser",
password: "supersecret"
})
}
Most APIs expect credentials as JSON in the body of a POST request.JSON.stringify()
converts the login info into a proper JSON string format before sending it.
4️⃣ Handle the Response from the Server
}, function (err, res) {
const json = res.json();
This function waits for the response and converts it to a JSON object usingres.json()
.
Now we can easily extract useful info from the response liketoken
anduserId
.
5️⃣ Save the Token for Later Use
pm.environment.set("token", json.token);
This saves the token into the environment.
You can then reference it in any request using{{token}}
, like this:
Authorization: Bearer {{token}}
This means all future requests are automatically authenticated, without having to log in again.
6️⃣ Save the User ID (Optional but Handy!)
pm.environment.set("userId", json.user.id);
In many APIs, you’ll need the user ID to get profile details, update settings, or fetch orders.
Saving it now saves you time later — no need to hardcode it.
Here’s what this pre-script does:
✅ Checks if you already have a token
✅ If not, logs in using your credentials
✅ Waits for the response
✅ Saves the token and user ID in the environment
✅ Makes all future tests run automatically, with no manual setup
Why This Matters? Testing Becomes Efficient, Reliable, and Scalable
With pre-scripts, you’ll notice some fantastic results:
- No more repetitive setup in each test.
- Faster test execution, because setup is automated.
- Less room for error, since the process is automated and consistent.
- Scalability, meaning you can scale up tests across thousands of requests without breaking a sweat.
Now, whether you have 5, 50, or 500 tests to run — your foundation is rock solid.
Why EchoAPI Makes Pre-Scripts Even Better
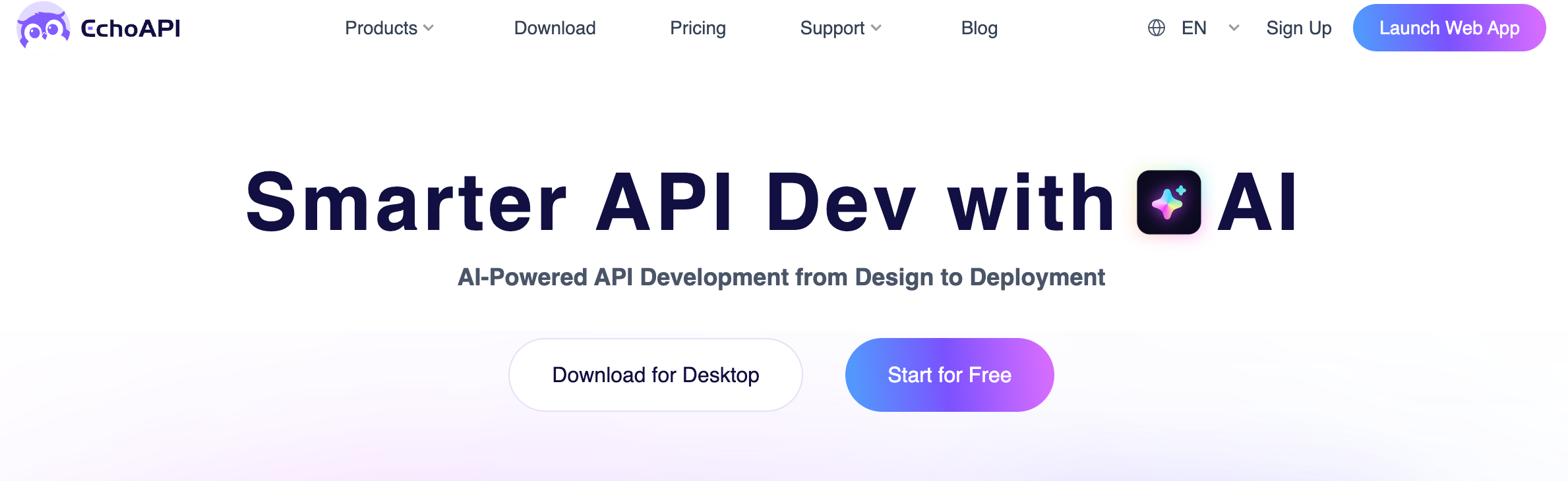
At EchoAPI, we’ve made pre-scripts simple, intuitive, and easy to integrate. Not only can you write pre-scripts to automate authentication, set global variables, and configure your environment, but you also get:
- Real-time debugging so you can catch errors right away.
- Global variable syncing, ensuring all your tests share the same data and environment.
- Flexible pre-script settings, whether you need one for the entire collection or just specific requests.
🎉 Ready to Try EchoAPI? It's Free, Fast, and Easy!
Don’t let your API testing be a chaotic LEGO pile. Get organized, automate the setup, and watch your tests run smoother than ever.
👉 Try EchoAPI now and see how pre-scripts can save you time, reduce errors, and make your tests more reliable. It only takes a few minutes to set up, and the results will blow your mind!
Takeaway: Pre-Scripts Are Your Secret Weapon
Just like you wouldn’t build a LEGO city without a plan, you shouldn’t test APIs without a pre-script.
It’s the blueprint for your testing environment — and it ensures every test is set up properly, consistently, and automatically.