Essential Tool Experiences in API Development: Lessons and Solutions for Developers
This article delves into the author's decade-long journey with API design, debugging, testing, documentation, and Mock tools, sharing valuable lessons, practical solutions, and lingering challenges.
As a developer with a decade of experience, my daily work revolves around API design, debugging, testing, documentation, and Mock. Throughout this process, tools are indispensable assistants, yet they often cause headaches. Whether it's Swagger, Postman, JMeter, or Fiddler, these tools create new problems while solving others.
Today, I want to share some issues I've encountered in actual projects, how I've resolved them, and the unresolved pain points. I hope these experiences can help you avoid some pitfalls and let you know you're not alone in facing these problems.
API Design Phase
Tool: Swagger
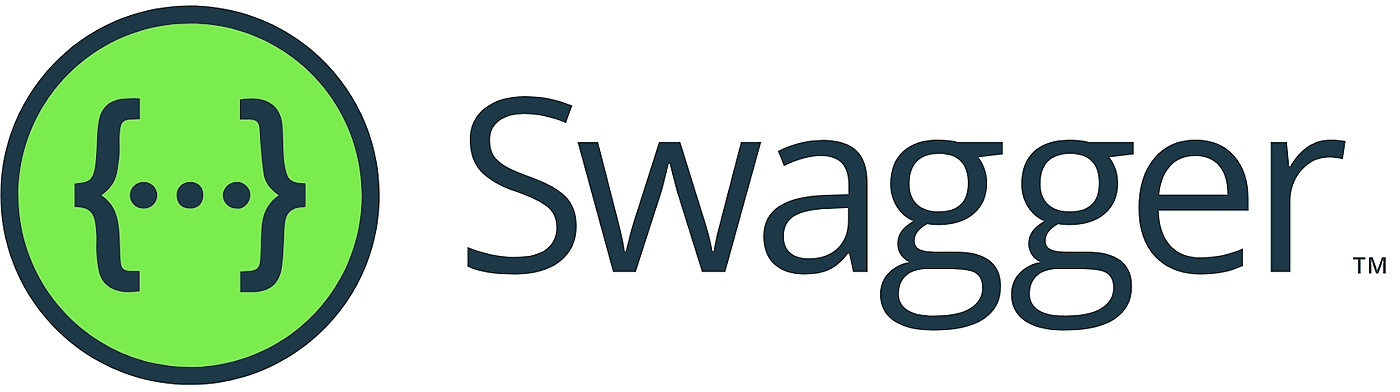
Issues:
- Inconsistent Fields: When designing APIs with Swagger, I often find inconsistencies in how front-end and back-end define fields. For instance, the back-end defines a field as type int, while the front-end expects a string.
- Untimely Documentation Updates: Swagger documentation requires manual updates, which are often forgotten during development, leading to discrepancies between the documentation and actual code.
- Difficulty in Describing Complex Data Structures: For nested JSON data structures, Swagger definitions become cumbersome and error-prone.
Solutions:
- Agree on Field Types: At the project's start, front-end and back-end teams should agree on field types and naming conventions to reduce inconsistencies.
- Automated Documentation Generation: Use code annotations to generate Swagger documentation, ensuring it updates in sync with the code.
- Use of Auxiliary Tools: Leverage JSON Schema tools to describe complex data structures and minimize manual errors.
Unresolved Pain Points:
- Documentation Readability: Even with automated tools, the readability of documentation remains poor, especially for complex APIs.
- Version Management: Swagger documentation version management is cumbersome, making it hard to trace historical versions.
Actual Scenario Example:
When developing an e-commerce project, the back-end designed a user information API that returned a user_id of type int. The front-end, however, treated user_id as a string, causing parsing errors.
Solutions:
- Agree on Field Types: At the project's start, front-end and back-end teams agreed to统一 all ID types as strings.
- Automated Documentation Generation: Use code annotations to generate Swagger documentation, ensuring it updates in sync with the code.
Unresolved Pain Points:
- Documentation Readability: Even with automated tools, the readability of documentation remains poor, especially for complex APIs.
Tool: Postman
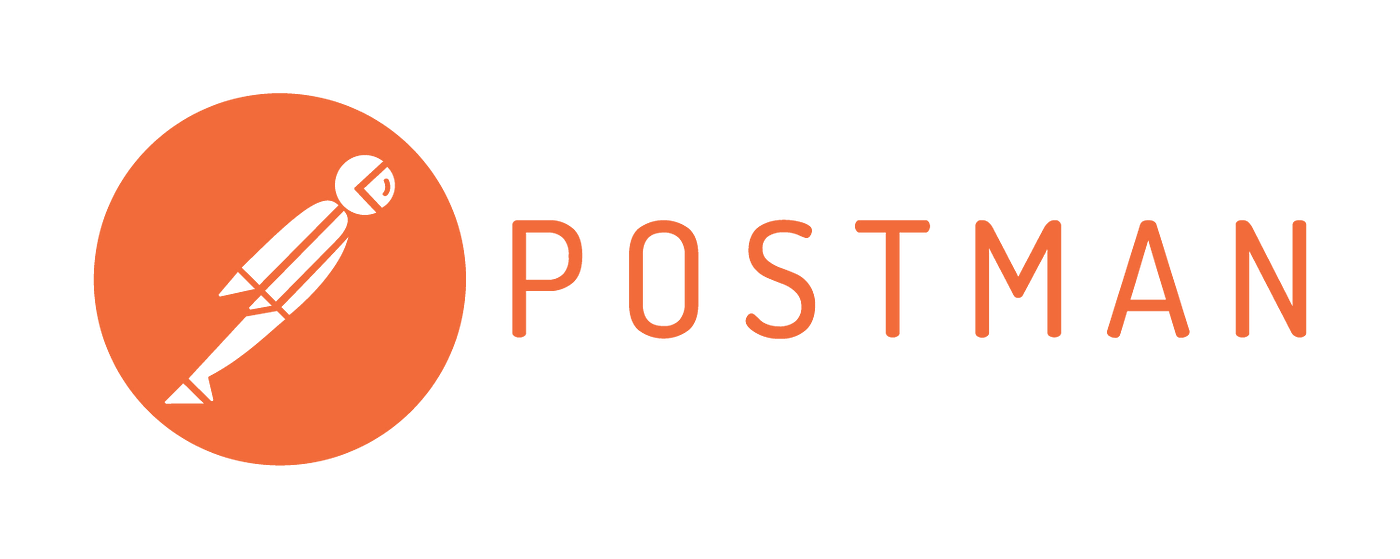
Issues:
- Complex Environment Variable Management: Managing multiple environment variables in Postman is prone to confusion, especially in team collaborations.
- Difficulty in Collection Synchronization: When sharing Postman collections among team members, inconsistencies often arise.
Solutions:
- Use Postman Workspaces: Manage collections and environment variables through team workspaces to ensure consistency.
- Regular Export and Import: Regularly export and import collections and environment variables to ensure all members use the same configuration.
Unresolved Pain Points:
- Performance Issues: Postman's performance significantly degrades when collections contain a large number of APIs.
- Low Collaboration Efficiency: Even with workspaces, team collaboration efficiency is still inferior to code repositories.
Actual Scenario Example:
In team development of an e-commerce project, different members need to switch between development, testing, and production environments. Managing environment variables in Postman becomes very complex, with frequent inconsistencies in collections among members.
Solutions:
- Use Postman Workspaces: Manage collections and environment variables through team workspaces to ensure consistency.
- Regular Export and Import: Regularly export and import collections and environment variables to ensure all members use the same configuration.
Unresolved Pain Points:
- Performance Issues: Postman's performance significantly degrades when collections contain a large number of APIs.
API Debugging Phase
Tool: Fiddler
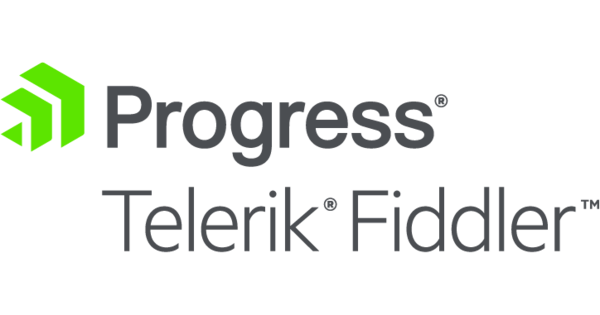
Issues:
- Cross-Domain Problems: When debugging local APIs, Fiddler often fails to capture requests due to cross-domain issues.
- Complex Configuration: Each debugging session requires reconfiguring Fiddler, which is tedious.
Solutions:
- Set Up Proxy: Configure a proxy in the local development environment to ensure requests go through Fiddler.
- Automate Configuration with Scripts: Write Fiddler scripts to automate the configuration process.
Unresolved Pain Points:
- Compatibility Issues: Fiddler has poor support for certain modern encryption protocols, making it unable to capture requests.
- Steep Learning Curve: Fiddler's powerful features come with a high learning cost.
Actual Scenario Example:
When debugging an API for retrieving user orders, local development environment requests couldn't be captured by Fiddler due to cross-domain issues causing browser interception.
Solutions:
- Set Up Proxy: Configure a proxy in the local development environment to ensure requests go through Fiddler.
- Automate Configuration with Scripts: Write Fiddler scripts to automate the configuration process.
Unresolved Pain Points:
- Compatibility Issues: Fiddler has poor support for certain modern encryption protocols, making it unable to capture requests.
API Testing Phase
Tool: JMeter
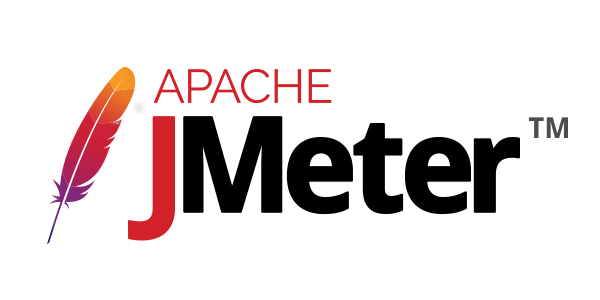
Issues:
- Complex Performance Testing: JMeter's performance testing configuration is cumbersome, especially when simulating high-concurrency scenarios.
- Difficult Result Analysis: Test result analysis requires manual processing, lacking intuitive visualization tools.
Solutions:
- Use Plugins: Install JMeter plugins (like JMeter Plugins) to simplify configuration and result analysis.
- Combine with Grafana: Import JMeter test results into Grafana for visual analysis.
Unresolved Pain Points:
- High Learning Cost: JMeter has a steep learning curve, making it difficult for new members to get started.
- Difficulty Integrating with CI/CD: JMeter is hard to directly integrate into CI/CD pipelines, resulting in low testing automation.
Actual Scenario Example:
When testing an API for an e-commerce promotion that required simulating 1000 concurrent users, JMeter's configuration was very cumbersome, with thread groups and HTTP request settings needing multiple adjustments.
Solutions:
- Use Plugins: Install JMeter Plugins to simplify configuration and result analysis.
- Combine with Grafana: Import JMeter test results into Grafana for visual analysis.
Unresolved Pain Points:
- High Learning Cost: JMeter has a steep learning curve, making it difficult for new members to get started.
Tool: Postman
Issues:
- Difficult Test Script Maintenance: Postman test scripts (Tests) need frequent updates after API changes, resulting in high maintenance costs.
- Team Collaboration Issues: Sharing and synchronization of test collections are still inefficient.
Solutions:
- Modular Test Scripts: Break test scripts into modules to reduce duplicate code and facilitate maintenance.
- Use Postman Monitor: Regularly run test collections with Postman Monitor to ensure API stability.
Unresolved Pain Points:
- Limited Performance Testing Capabilities: Postman's performance testing features are weak and can't meet high-concurrency demands.
- Insufficient Test Coverage: Manually written test scripts are hard to cover all scenarios, leading to potential omissions.
Actual Scenario Example:
When testing a user login API, Postman test scripts (Tests) required frequent updates to adapt to API changes, resulting in high maintenance costs.
Solutions:
- Modular Test Scripts: Break test scripts into modules to reduce duplicate code and facilitate maintenance.
- Use Postman Monitor: Regularly run test collections with Postman Monitor to ensure API stability.
Unresolved Pain Points:
- Limited Performance Testing Capabilities: Postman's performance testing features are weak and can't meet high-concurrency demands.
API Documentation Phase
Tool: Swagger
Issues:
- Untimely Documentation Updates: During development, API documentation often lags behind code updates.
- Poor Documentation Readability: Auto-generated documentation lacks examples and detailed explanations, resulting in poor readability.
Solutions:
- Automated Generation: Use code annotations to auto-generate documentation, ensuring it updates in sync with the code.
- Add Examples: Manually supplement examples and explanations in the documentation to improve readability.
Unresolved Pain Points:
- Version Management: Swagger documentation version management is still inadequate, making it hard to trace historical versions.
- Limited Multilingual Support: For international projects, Swagger documentation has weak multilingual support.
Actual Scenario Example:
When developing a user registration API, the back-end added a phone field, but the Swagger documentation wasn't updated in time, causing front-end developers to miss the correct field information.
Solutions:
- Automated Generation: Use code annotations to auto-generate documentation, ensuring it updates in sync with the code.
- Add Examples: Manually supplement examples and explanations in the documentation to improve readability.
Unresolved Pain Points:
- Version Management: Swagger documentation version management is still inadequate, making it hard to trace historical versions.
Tool: Postman
Issues:
- Complex Documentation Export: Postman's documentation export functionality is limited and can't generate high-quality API documentation.
- Low Collaboration Efficiency: Team members are prone to duplicate work when maintaining documentation.
Solutions:
- Combine with Markdown: Export Postman API information to Markdown and manually supplement explanations.
- Use Postman API: Automate documentation generation and export with the Postman API.
Unresolved Pain Points:
- Poor Documentation Aesthetics: Postman-generated documentation has a poor format and lacks visual appeal.
- Lack of Synchronization with Code: Documentation and code synchronization still requires manual operations, increasing the risk of errors.
Actual Scenario Example:
When maintaining an API for retrieving user information, Postman's documentation export functionality was limited, requiring team members to manually supplement explanations.
Solutions:
- Combine with Markdown: Export Postman API information to Markdown and manually supplement explanations.
- Use Postman API: Automate documentation generation and export with the Postman API.
Unresolved Pain Points:
- Poor Documentation Aesthetics: Postman-generated documentation has a poor format and lacks visual appeal.
API Mock Phase
Tool: Faker.js
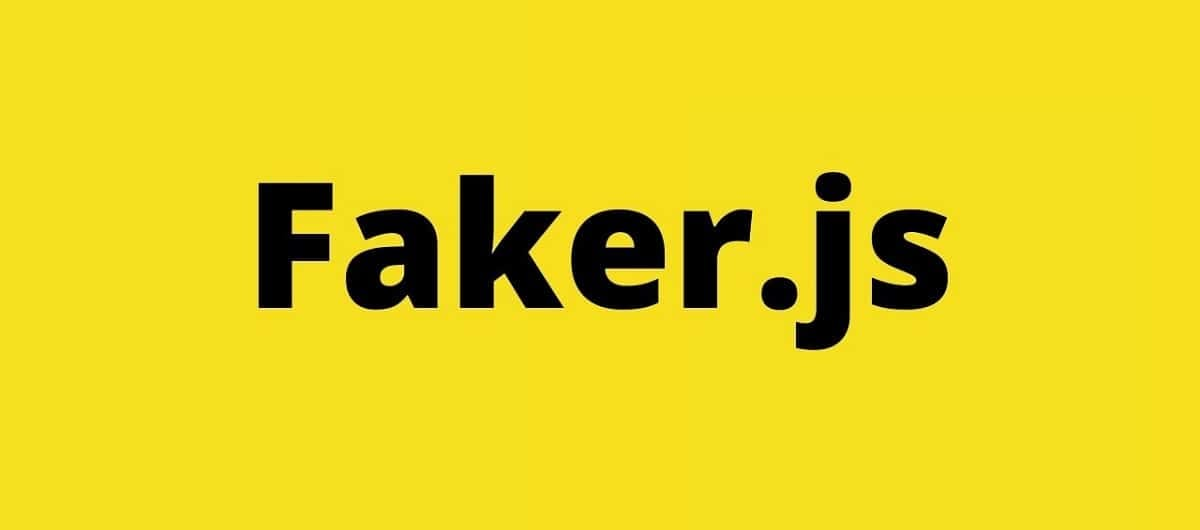
Issues:
- Complex Mock Data: Writing rules for complex data structures with Mock.js is very cumbersome.
- Performance Issues: Mock.js performs poorly under high concurrency, prone to lag.
Solutions:
- Use Templates: Prepare Mock templates for common data structures to reduce repetitive writing.
- Combine with Backend Services: Use backend services to generate Mock data in complex scenarios.
Unresolved Pain Points:
- Weak Dynamic Data Support: Mock.js has poor support for dynamic data, making it hard to simulate real scenarios.
- High Learning Cost: Mock.js's rule syntax is complex, making it difficult for new members to get started.
Actual Scenario Example:
When developing an API for retrieving product lists that required simulating complex nested JSON data structures, writing rules with Mock.js was very cumbersome, reducing development efficiency.
Solutions:
- Use Templates: Prepare Mock templates for common data structures to reduce repetitive writing.
- Combine with Backend Services: Use backend services to generate Mock data in complex scenarios.
Unresolved Pain Points:
- Weak Dynamic Data Support: Mock.js has poor support for dynamic data, making it hard to simulate real scenarios.
Tool: Postman
Issues:
- Difficult Mock Data Maintenance: Postman's Mock Server requires manual data updates, resulting in high maintenance costs.
- Performance Issues: Under high concurrency, Postman Mock Server's performance is poor.
Solutions:
- Combine with Automation Scripts: Use automation scripts to regularly update Mock data.
- Use External Mock Services: In complex scenarios, use external Mock services (like Mocky.io).
Unresolved Pain Points:
- Weak Dynamic Data Support: Postman Mock Server has poor support for dynamic data, failing to meet complex demands.
- Inconsistency with Actual API: Mock data and actual API consistency is hard to guarantee, potentially causing testing deviations.
Actual Scenario Example:
When testing an API for retrieving user orders, Postman's Mock Server required manual data updates, had high maintenance costs, and poor performance under high concurrency.
Solutions:
- Combine with Automation Scripts: Use automation scripts to regularly update Mock data.
- Use External Mock Services: In complex scenarios, use external Mock services (like Mocky.io).
Unresolved Pain Points:
- Weak Dynamic Data Support: Postman Mock Server has poor support for dynamic data, failing to meet complex demands.
EchoAPI: An All-in-One Tool for API Design, Debugging, Testing, Documentation, and Mock
Today, I want to share a tool that has impressed me—EchoAPI. It not only addresses many issues I've encountered in API design, debugging, testing, documentation, and Mock phases but also shows the future direction of development tools.
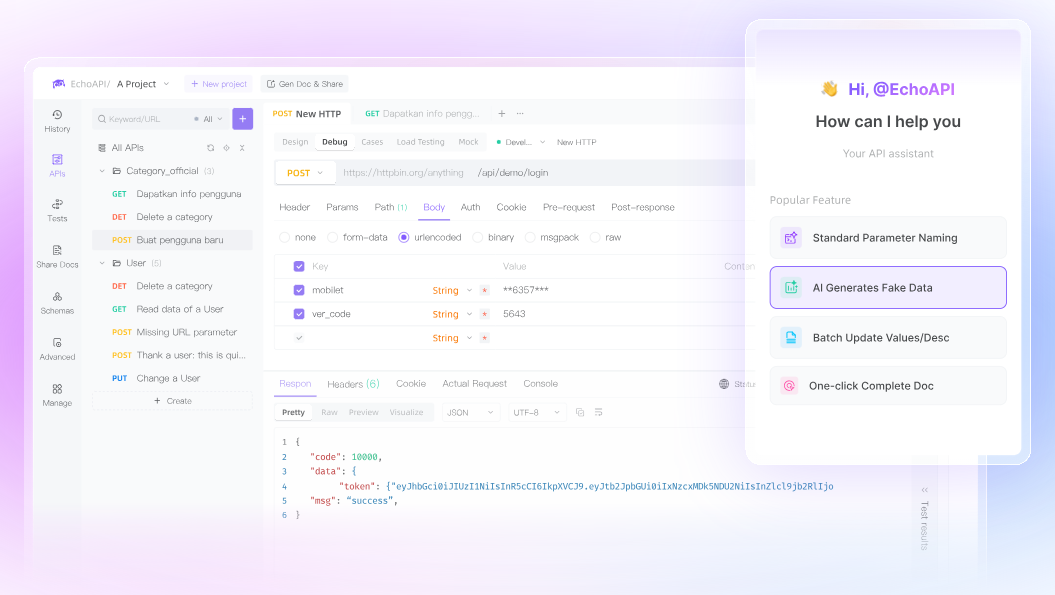
Design Phase
Issues:
Complex Environment Variable Management: EchoAPI allows setting up private environments that don't affect other collaborators, preventing confusion.
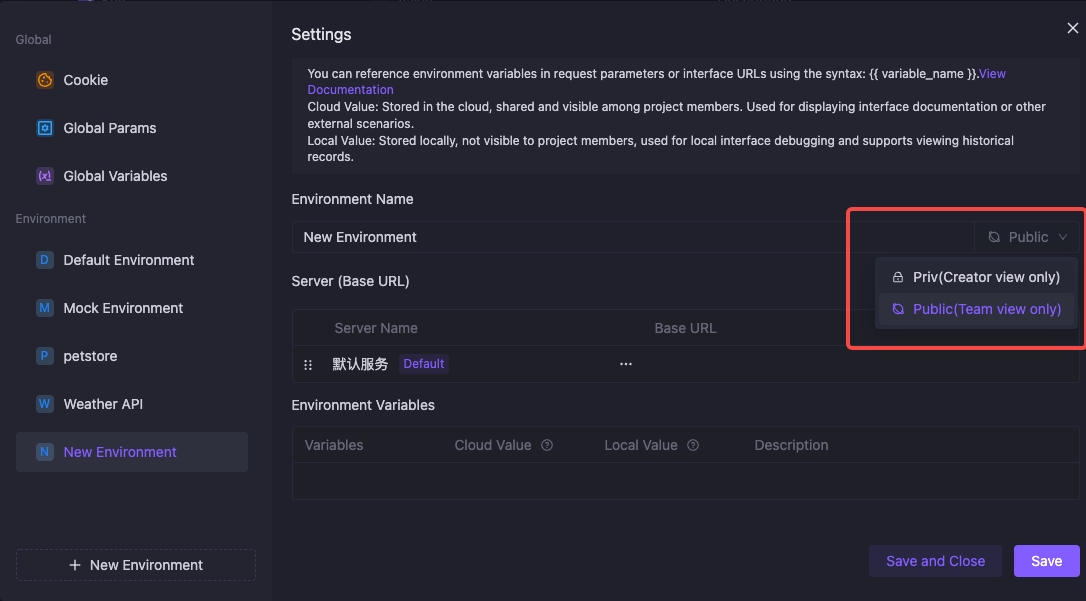
Complex Data Structures: EchoAPI comes with a built-in Schemas library that can be directly imported and used in fields.
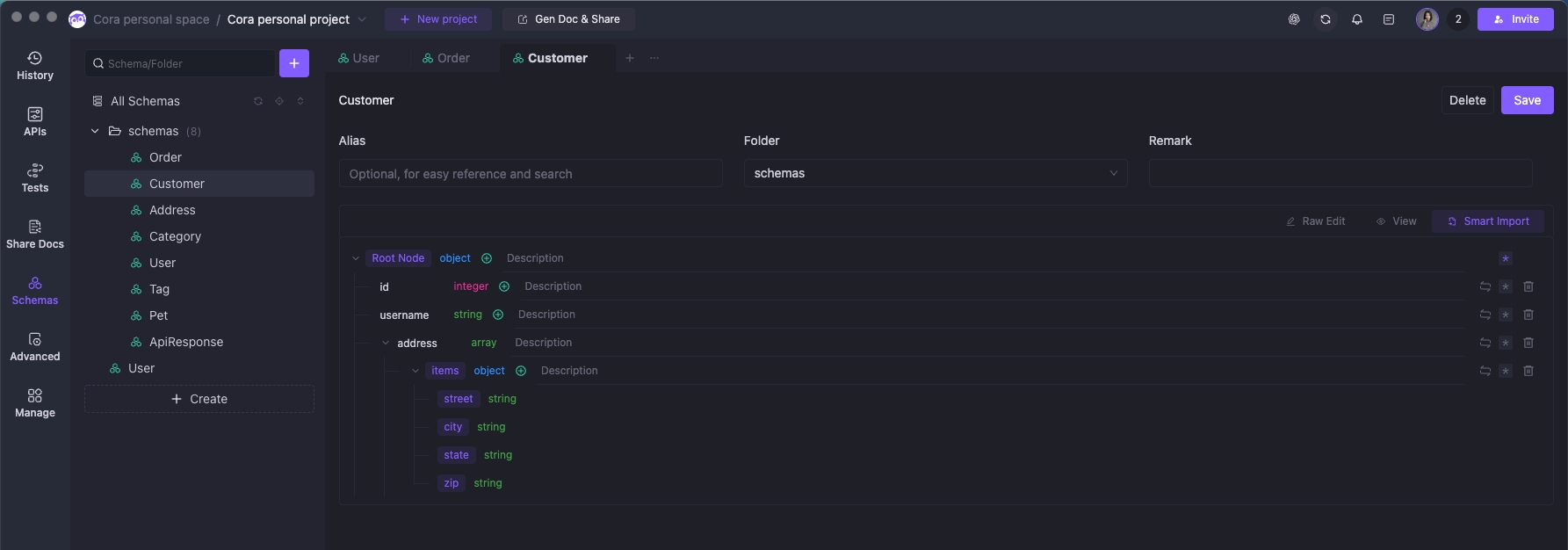
Inconsistent Fields: With EchoAPI, field types can be directly defined during design to prevent inconsistencies between front-end and back-end field definitions.
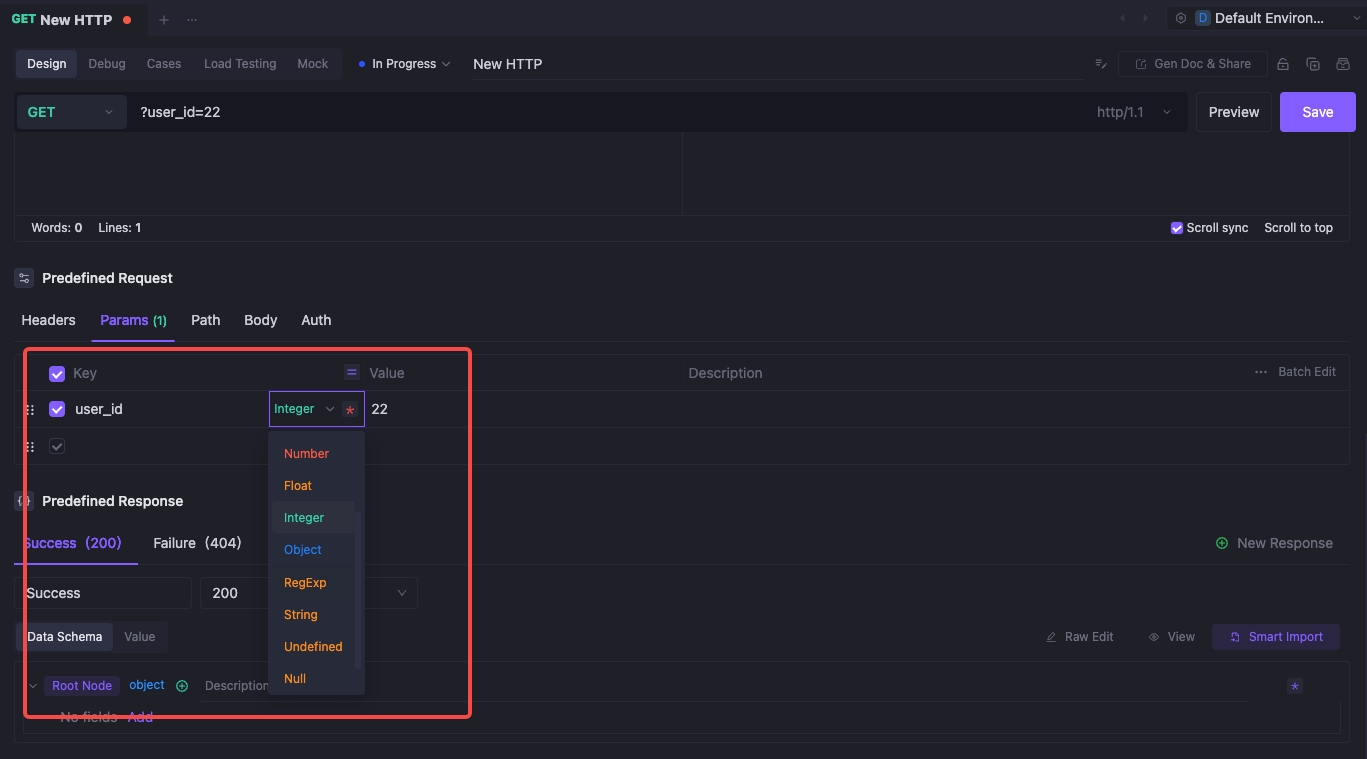
API Debugging Phase
Issues:
Learning Curve: The operation is simple and easy to get started with.
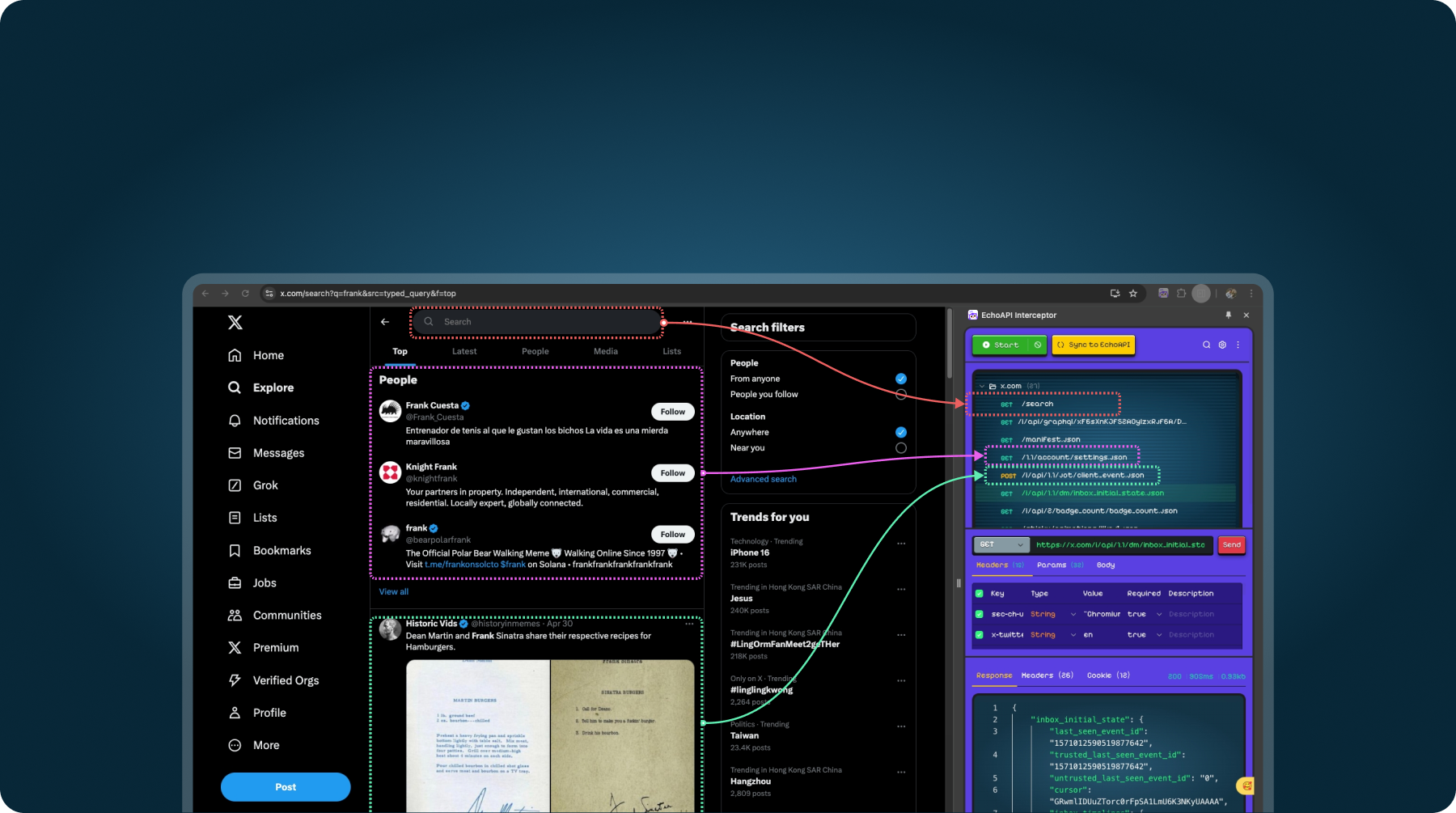
Cross-Domain Problems: EchoAPI's browser extension, EchoAPI Interceptor, eliminates cross-domain issues and easily captures interfaces.
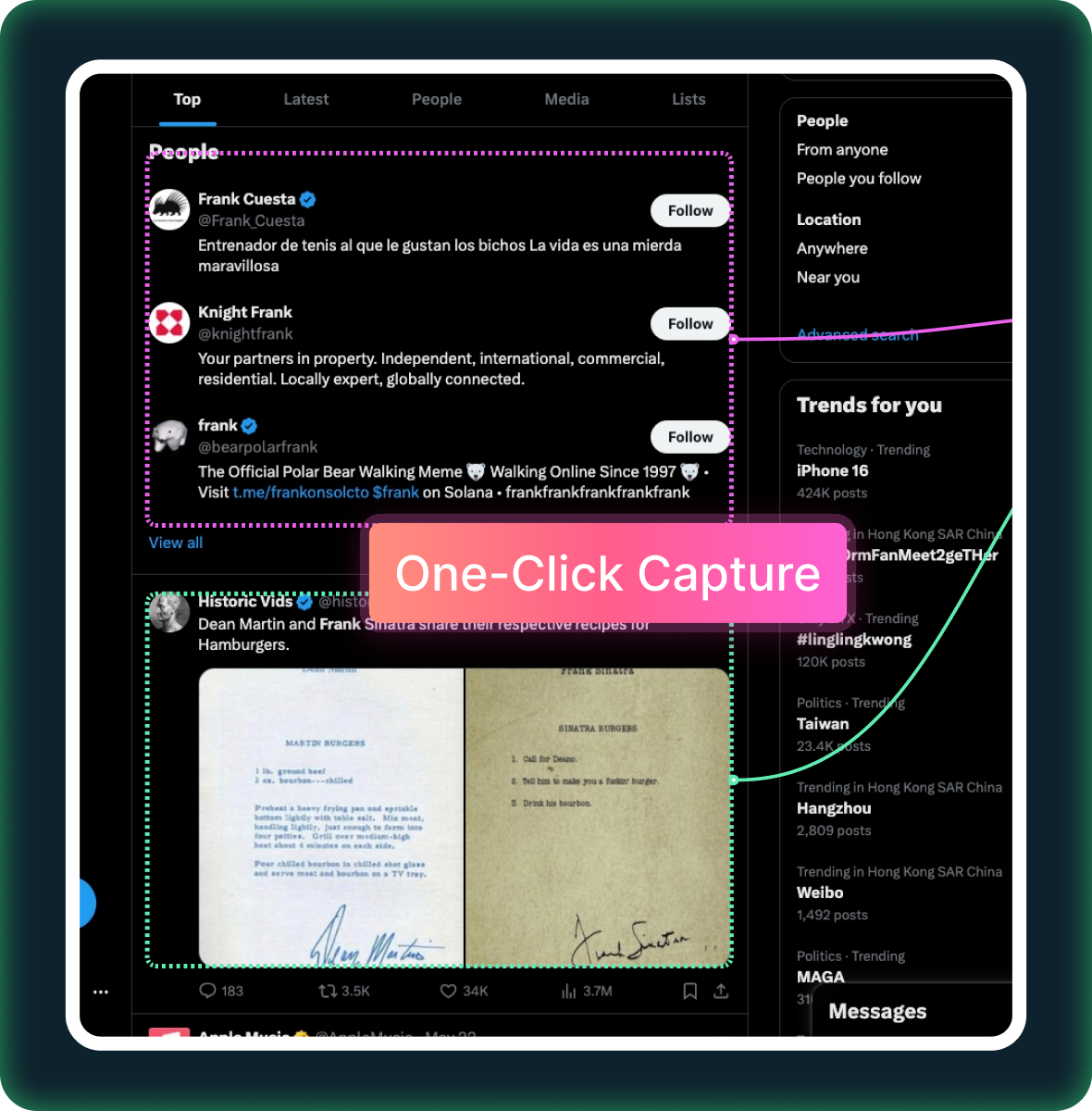
API Testing Phase
Issues:
- Difficult Result Analysis: EchoAPI's performance testing reports combine charts for intuitive data presentation.
Complex Performance Testing: EchoAPI's performance testing visualization page simplifies configuration with a one-click completion.
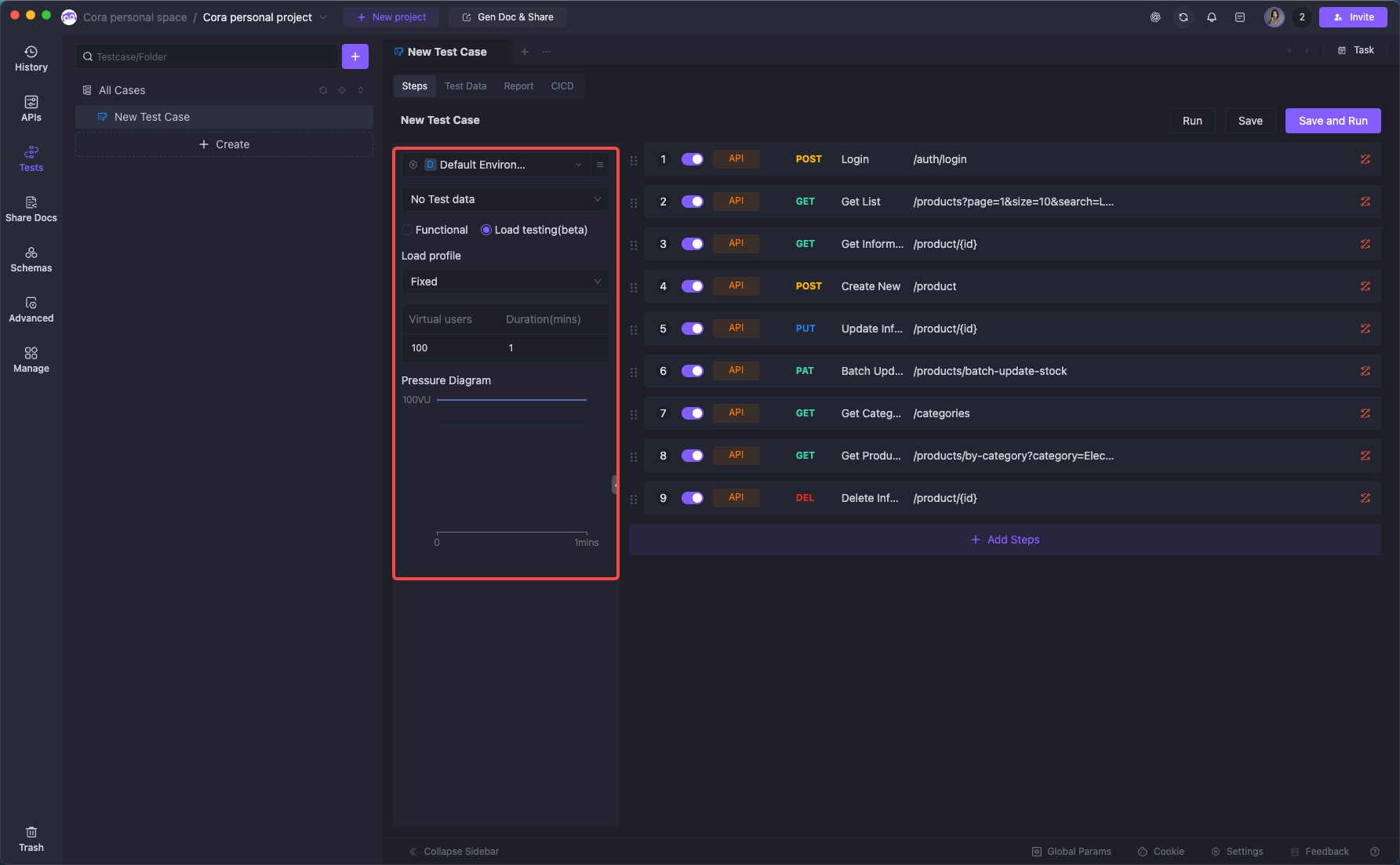
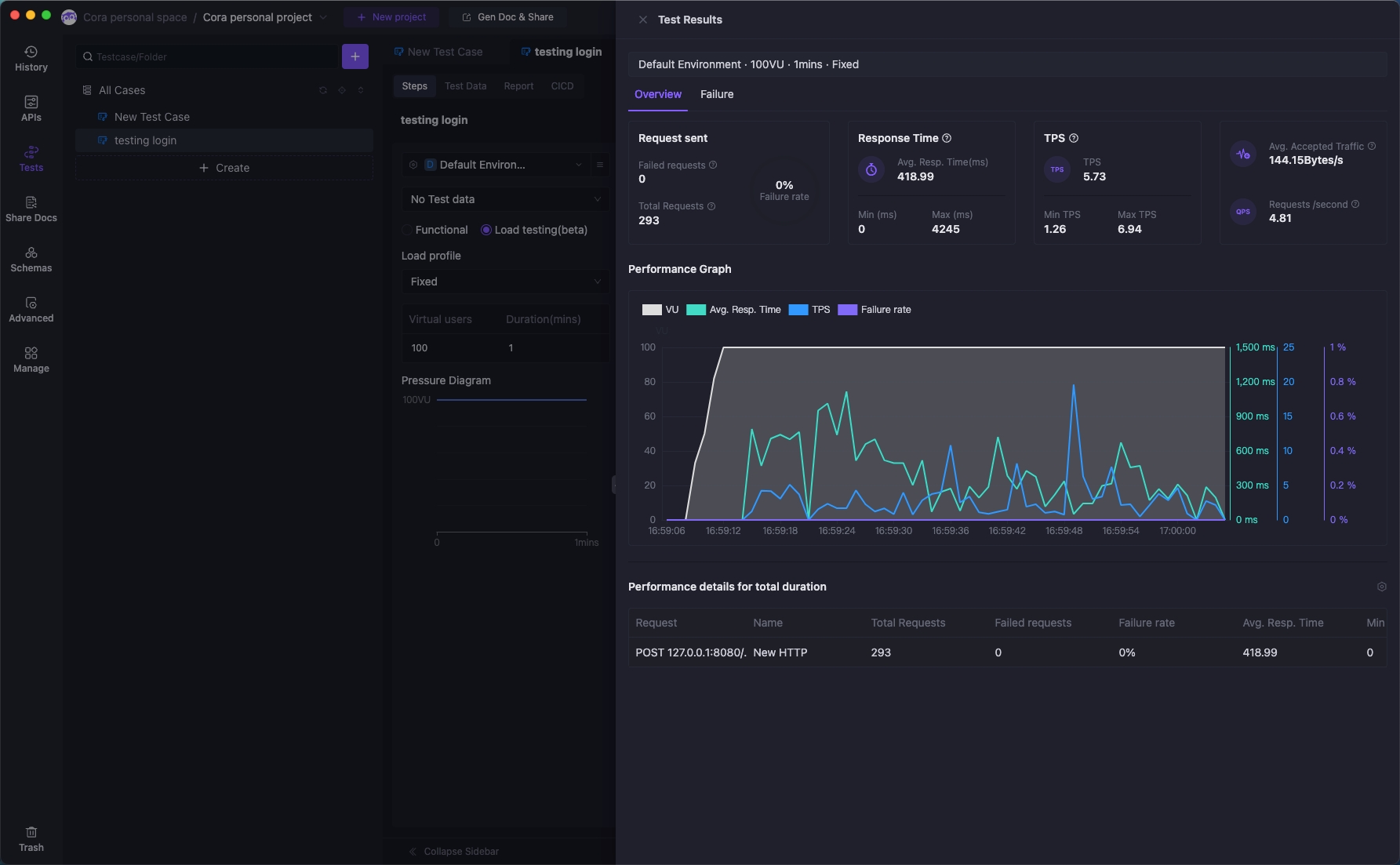
Difficulty Integrating with CI/CD: EchoAPI can generate CI/CD integrations with one click based on testing scenarios.
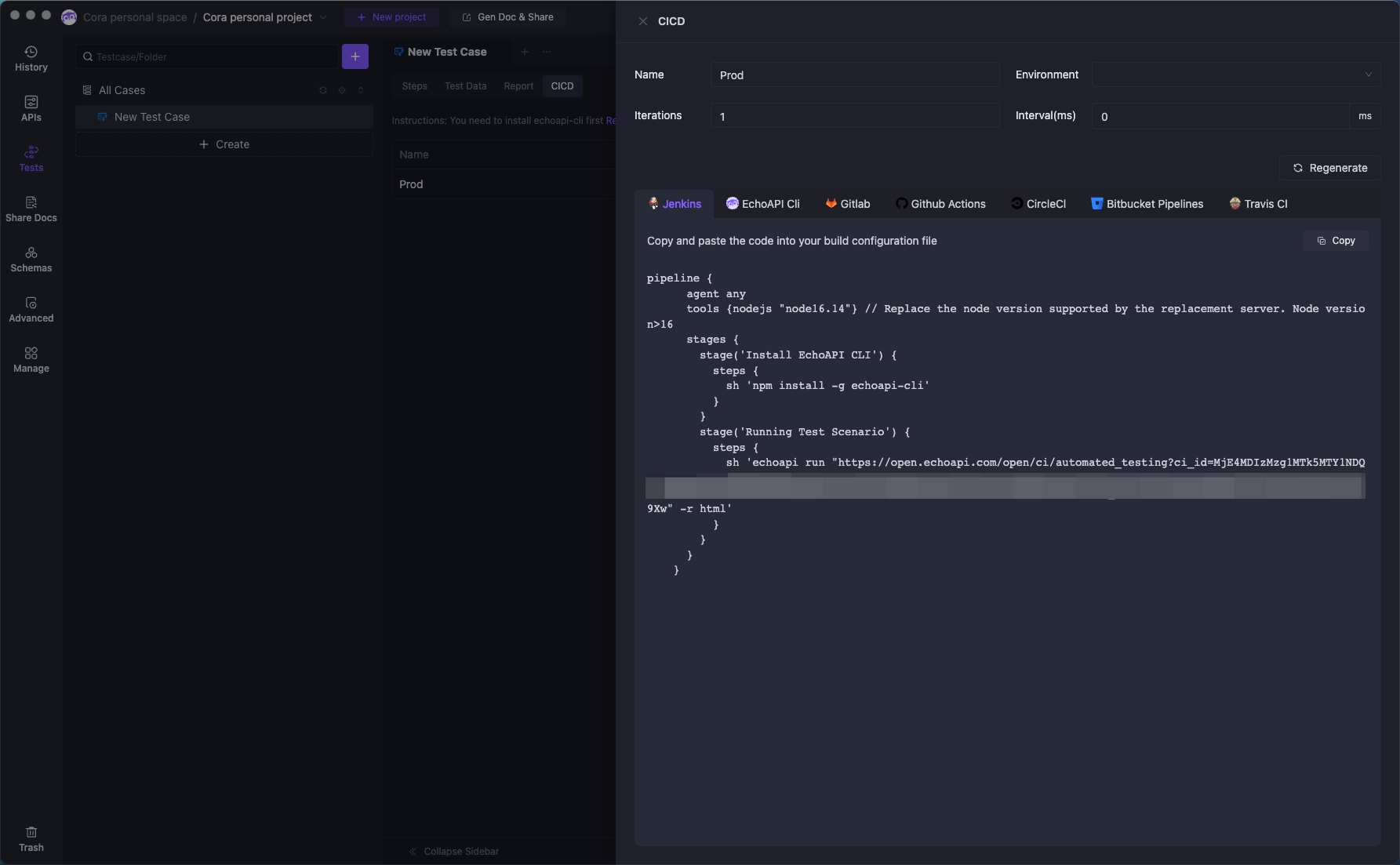
High Learning Cost: Conducting performance testing with EchoAPI requires no additional configurations, making it simple and easy to learn.
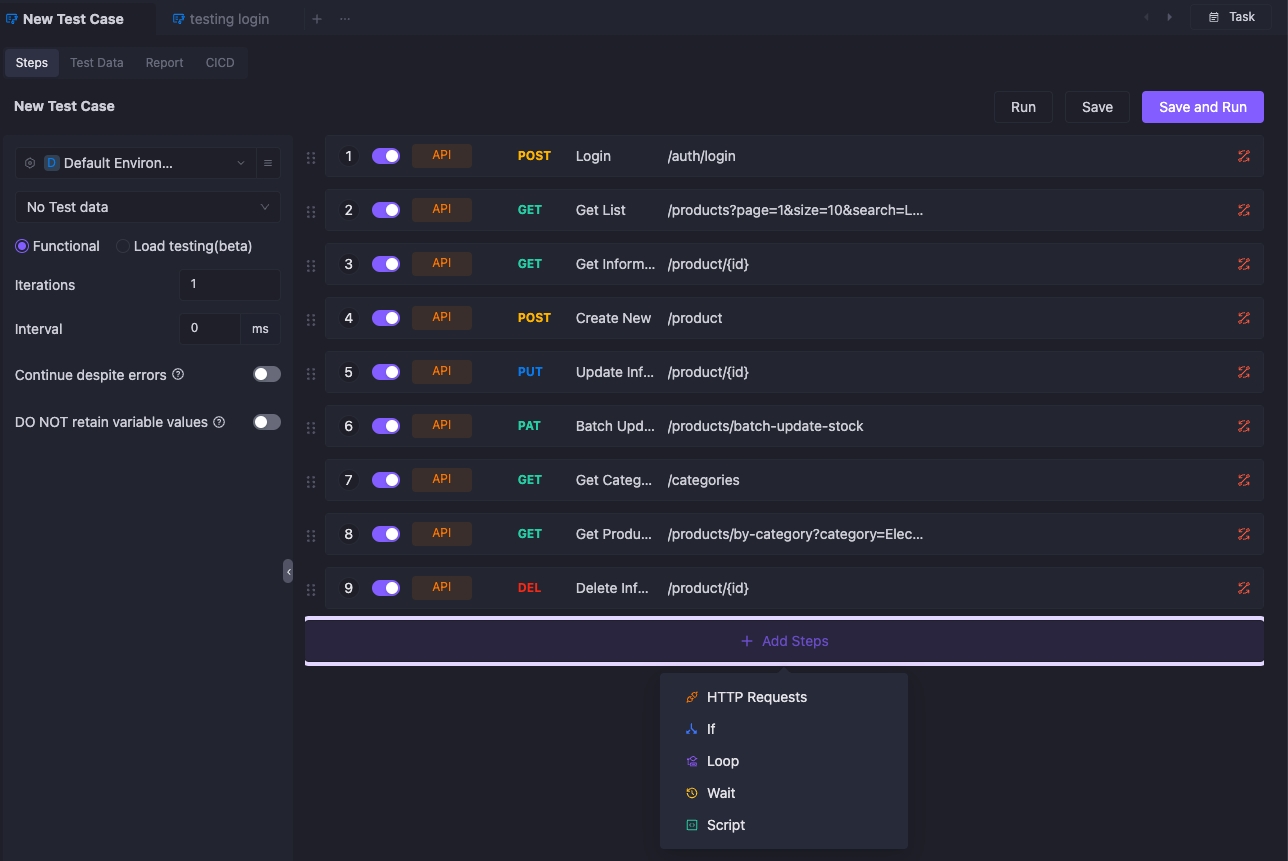
API Documentation Phase
Issues:
Multilingual Support: EchoAPI offers English, Japanese, Indonesian, and Traditional Chinese.
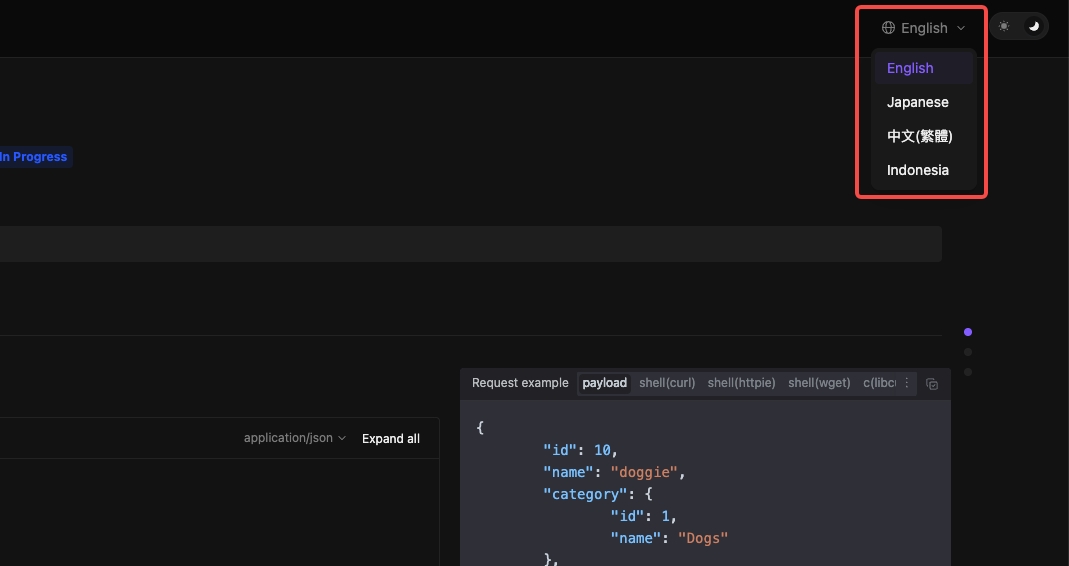
Documentation Readability: EchoAPI excels in documentation readability and aesthetics, generating well-structured and readable API documentation.
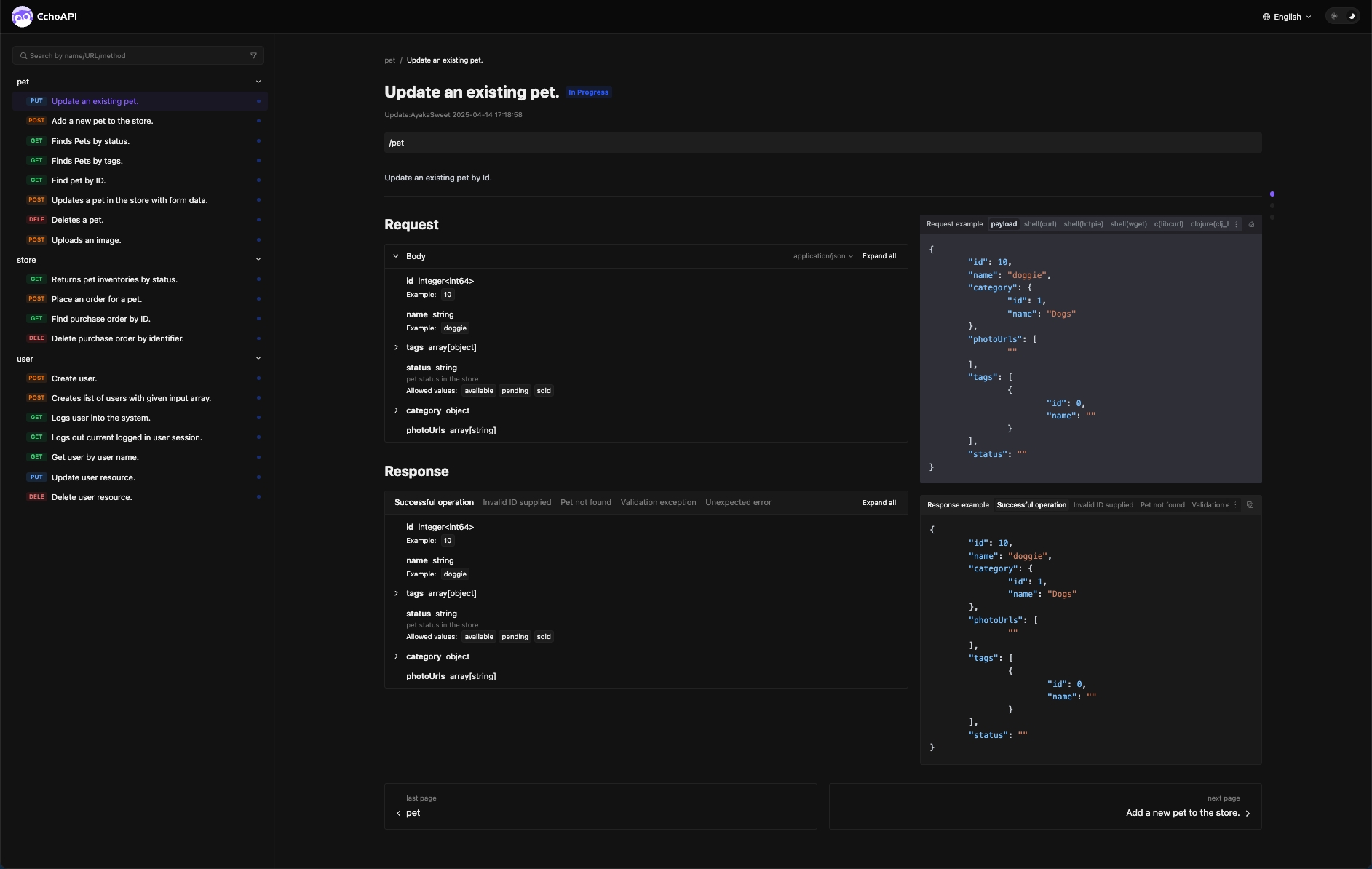
Untimely Documentation Updates: When APIs change in the EchoAPI workspace, the documentation automatically updates to the latest API information.
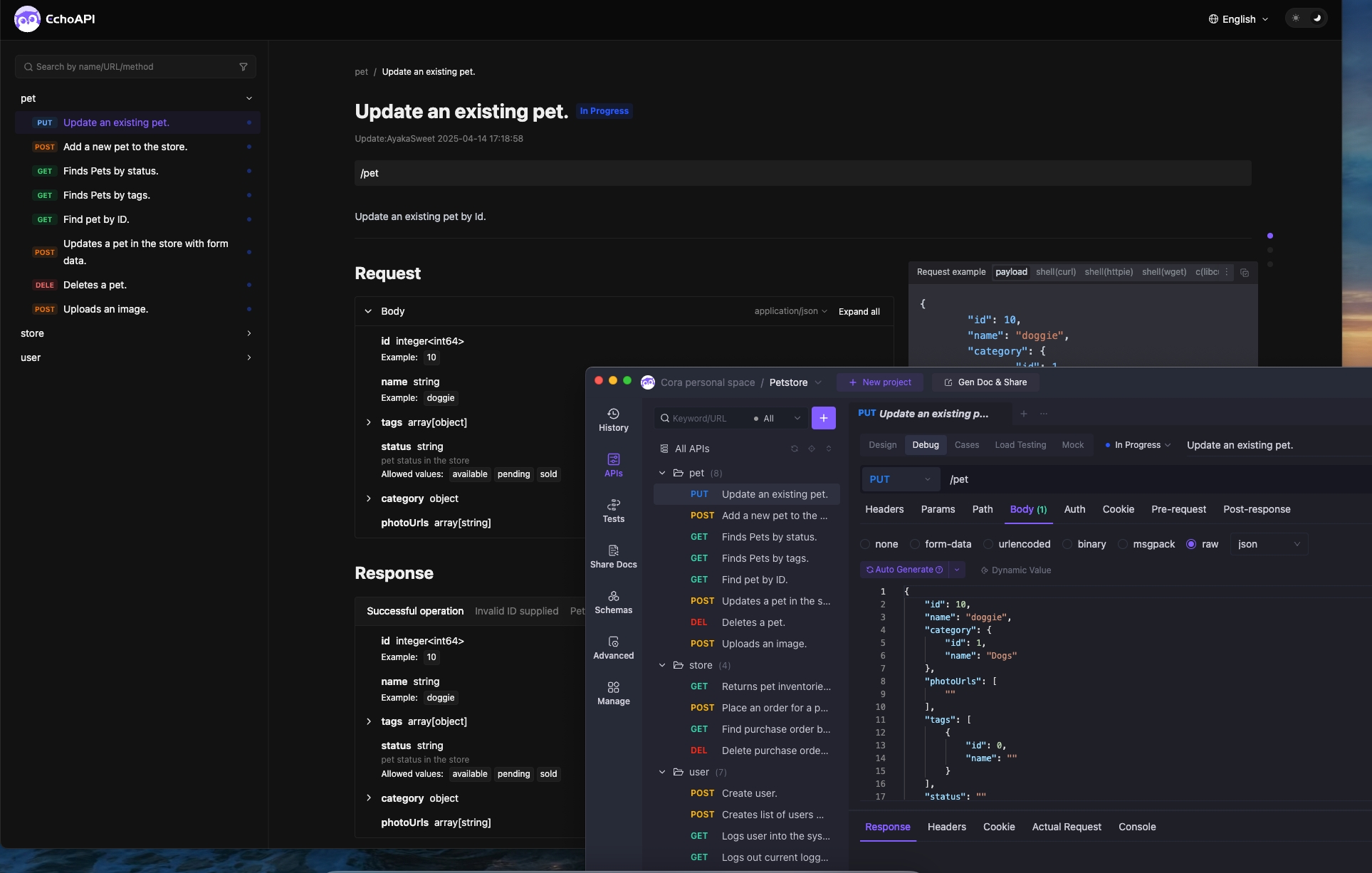
API Mock Phase
Issues:
Weak Dynamic Data Support: EchoAPI's dynamic value functionality allows for Mocking various types of data.
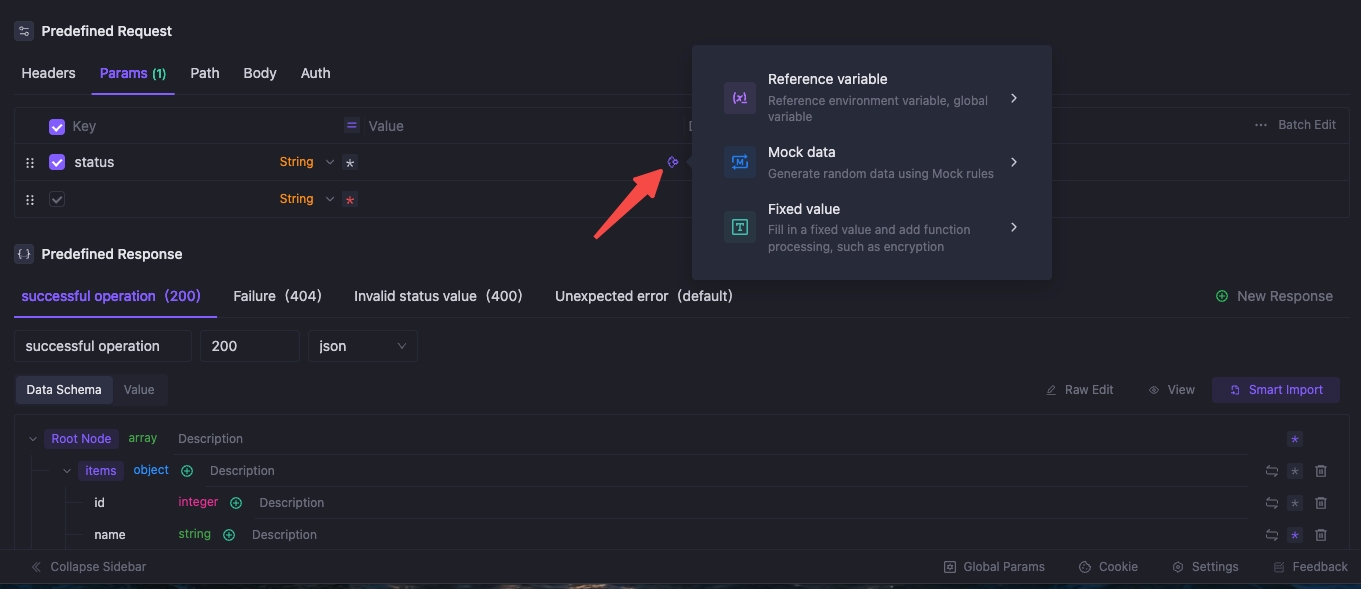
Complex Mock Data: Through API design, the required API can be directly Mock-generated.
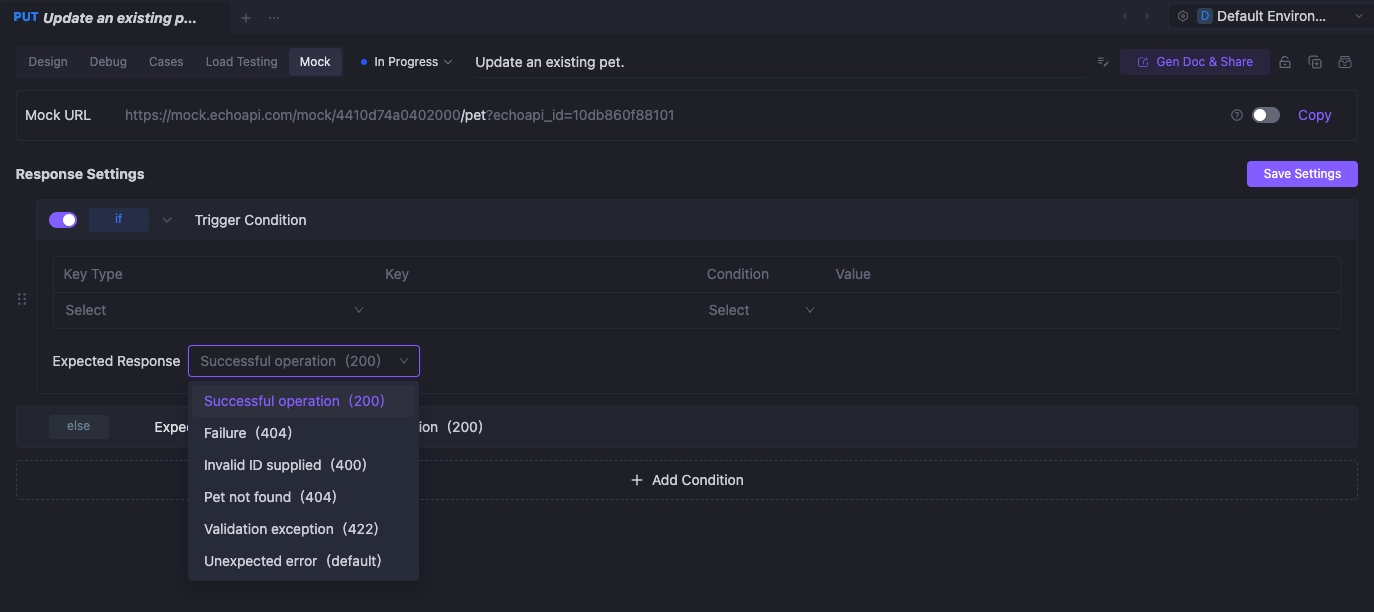
Summary
Looking back on my development journey, I have to admit that tool usage is a double-edged sword. They enable us to work more efficiently but can also slow progress due to complex configurations, poor performance, or collaboration difficulties. However, EchoAPI brings hope. It not only resolves many long-standing pain points but also provides developers with a smarter and more efficient development environment.
I believe that with continuous technological advancements, tools like EchoAPI will become more prevalent, helping us tackle various development challenges more easily. If you're looking for a tool to boost development efficiency, give EchoAPI a try—it might surprise you. Let's look forward to the future of development tools and smoother progress on the development path.