From Development to Testing: The Complete Path for Java Developers
This article walks you through the complete journey of Java development to testing, showcasing tools, tips, and a practical scenario to help you understand the workflow.
Java has been a dominant force in the software development world for decades. With its "write once, run anywhere" (WORA) nature, Java has become the backbone of enterprise-level applications, web services, mobile apps (via Android), and even modern cloud-based solutions. Its extensive ecosystem, strong community support, and continual evolution make it an indispensable language for developers worldwide.
But building quality software with Java doesn’t just rely on knowing the language. A solid set of development and testing tools is essential to navigate the full lifecycle of a project. This article walks you through the complete journey of Java development to testing, showcasing tools, tips, and a practical scenario to help you understand the workflow.
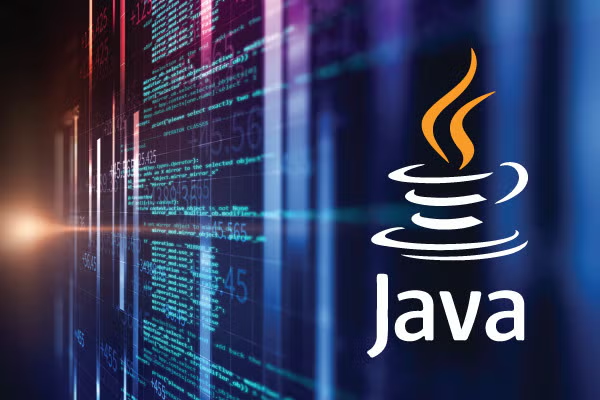
Why Java?
Before we dive into the workflow, let’s take a quick look at why Java remains such a reliable choice for developers:
- Platform Independence: Java applications can run on any machine capable of running the Java Virtual Machine (JVM), making it a highly portable language.
- Scalability: Its strong object-oriented principles, multithreading capabilities, and extensive frameworks make Java perfect for scalability.
- Security Features: Java's built-in security features and garbage collection ensure data safety and efficiency.
- Ecosystem Support: Rich libraries and frameworks like Spring, Hibernate, and Jakarta EE support a wide range of use cases, from web to cloud services.
With these advantages in mind, let’s dive into the tools that every Java developer needs in their toolkit.
Essential Java Development Tools
To be a successful Java developer, having the right tools is as important as knowing the language itself. Here are some must-have tools:
1. Integrated Development Environment (IDE)
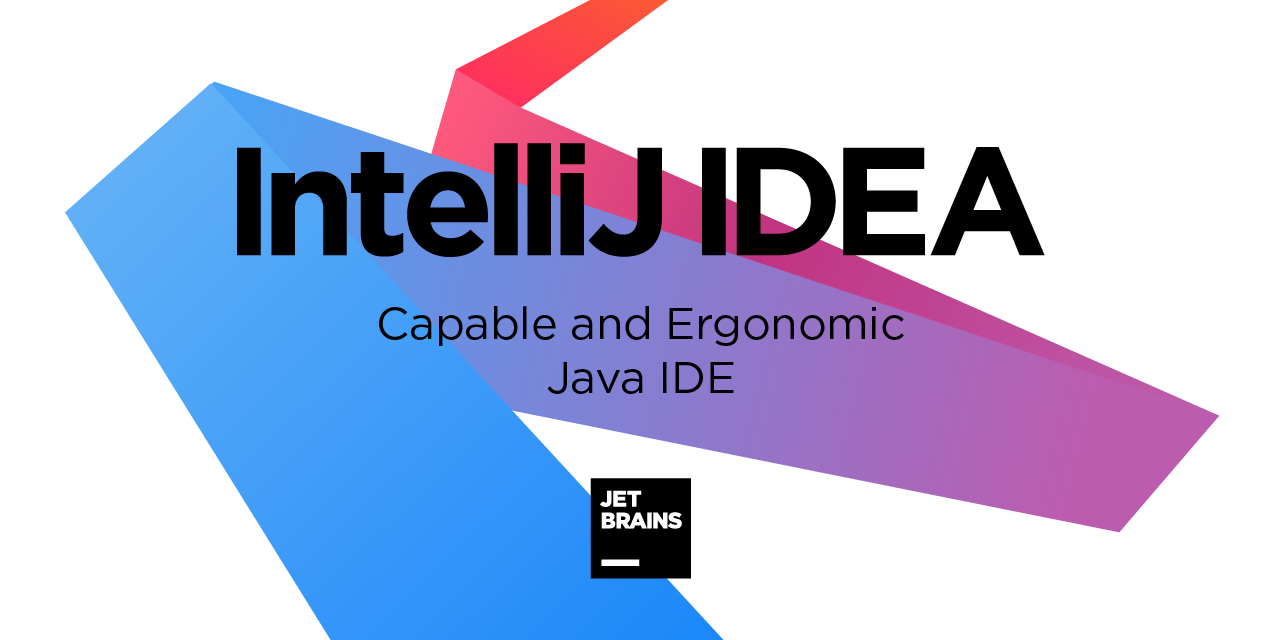
A powerful IDE makes Java development much easier by offering debugging, code assistance, and testing support. Popular IDEs:
- IntelliJ IDEA: Ideal for enterprise development, offering excellent refactoring and debugging.
- Eclipse: Time-tested, with a comprehensive plugin ecosystem.
- NetBeans: Lightweight and beginner-friendly.
2. Build Management Tools
Java projects often require efficient dependency management tools like:
- Maven: Great for managing project dependencies and building processes.
- Gradle: Extremely flexible and faster for larger projects.
3. Version Control
Collaborative projects require effective version control to track changes:
- Git: Essential for version control, paired with platforms like GitHub or Bitbucket.
4. Testing and Debugging
Testing tools ensure your application performs as expected:
- JUnit: The standard framework for unit testing in Java.
- TestNG: Offers more flexible test configuration compared to JUnit.
5. API Debugging and Documentation
- Postman: Popular for testing RESTful APIs.
- Swagger: Excellent for API documentation and testing.
By complementing Java’s versatility with these tools, developers are prepared for every stage of the software lifecycle.
From Development to Testing: A Practical Journey
Now let’s walk through a practical end-to-end scenario of building and testing a Java-based application. Imagine you’re developing a backend system for an e-commerce platform. Here’s what the process might look like:
Step 1: Write the Code (Developing)
1. Set up your Development Environment
- Use IntelliJ IDEA to create a new Maven project for this backend system. Set up dependencies for essential frameworks like Spring Boot, Hibernate, and MySQL Driver.
2. Write the Code with Frameworks
- Build a RESTful API using Spring Boot for managing user accounts, products, and orders.
Example: Create an endpoint to retrieve product details.
@RestController
public class ProductController {
@GetMapping("/product/{id}")
public ResponseEntity<Product> getProduct(@PathVariable Long id) {
Product product = productService.getProductById(id);
return ResponseEntity.ok(product);
}
}
3. Save Code to Version Control
- Use Git for version control. Push your commits to GitHub for collaboration with the team.
Step 2: Test the API
Developing the API isn’t the end of the journey. Testing the API ensures it performs as expected.
1. Write Unit Tests
Use JUnit to write unit tests for your service methods. For instance, test the ProductService
to ensure it fetches data correctly.
@Test
public void testGetProductById() {
Product product = productService.getProductById(1L);
assertEquals("Laptop", product.getName());
}
2. Debug APIs Using EchoAPI
- Open EchoAPI to test the
/product/{id}
API. Create a GET request and specify test cases for different scenarios (e.g., valid product ID, invalid ID, missing authorization). Verify:- Status codes (200, 404).
- The structure and correctness of response data.
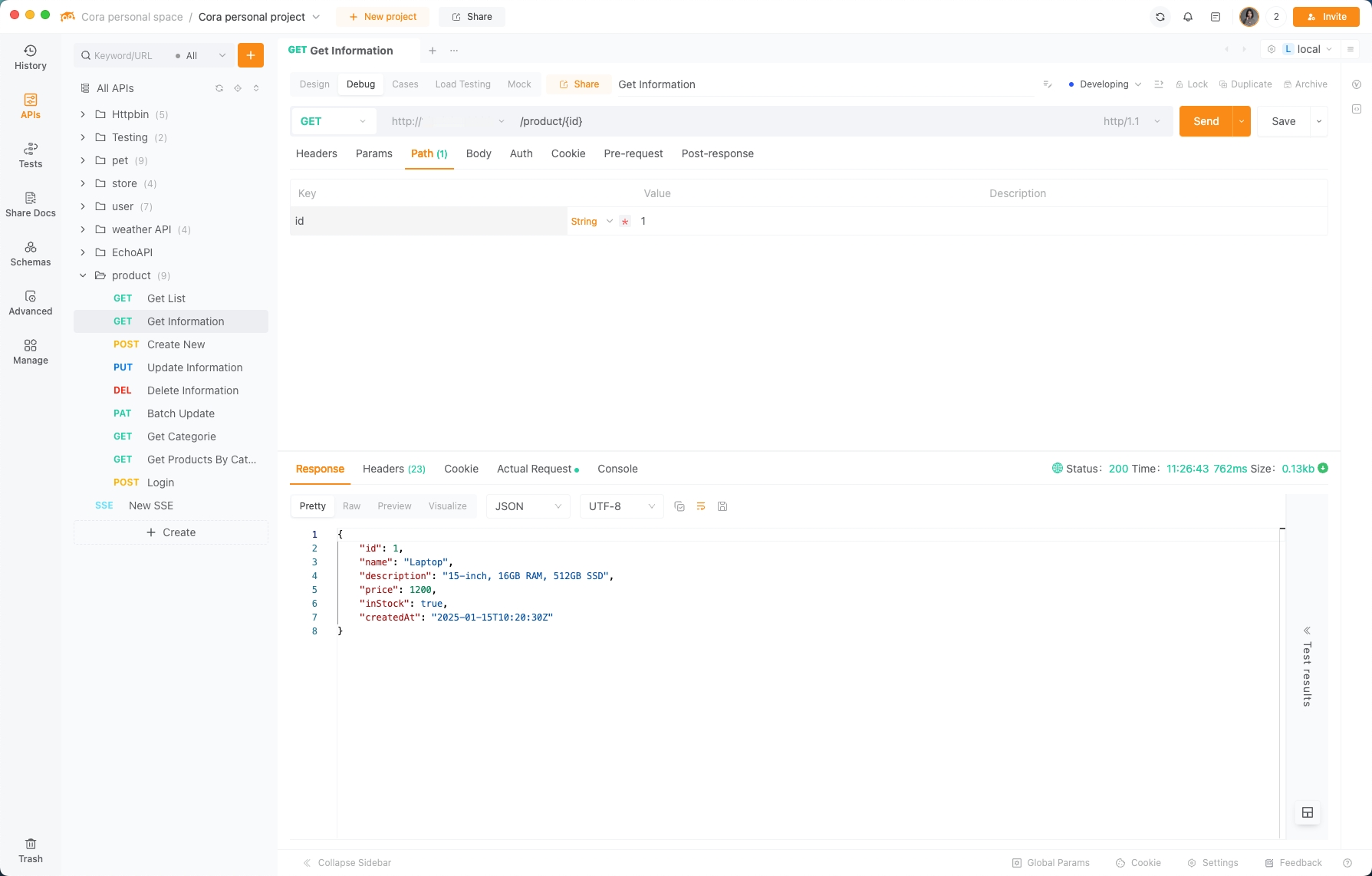
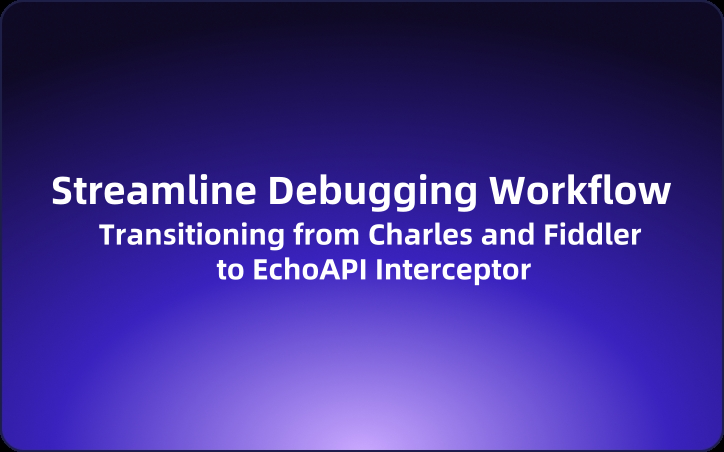
Step 3: Write API Documentation
After your API works as expected, documenting it is crucial so other developers or testers can understand how to use it.
Use EchoAPI for Automated API Documentation
- Integrate EchoAPI into your Spring Boot application. This generates an interactive documentation page where users can try out each API.
Example output:
{
"/product/{id}": {
"get": {
"summary": "Get product by ID",
"parameters": [{
"name": "id",
"in": "path",
"required": true,
"type": "integer"
}]
}
}
}
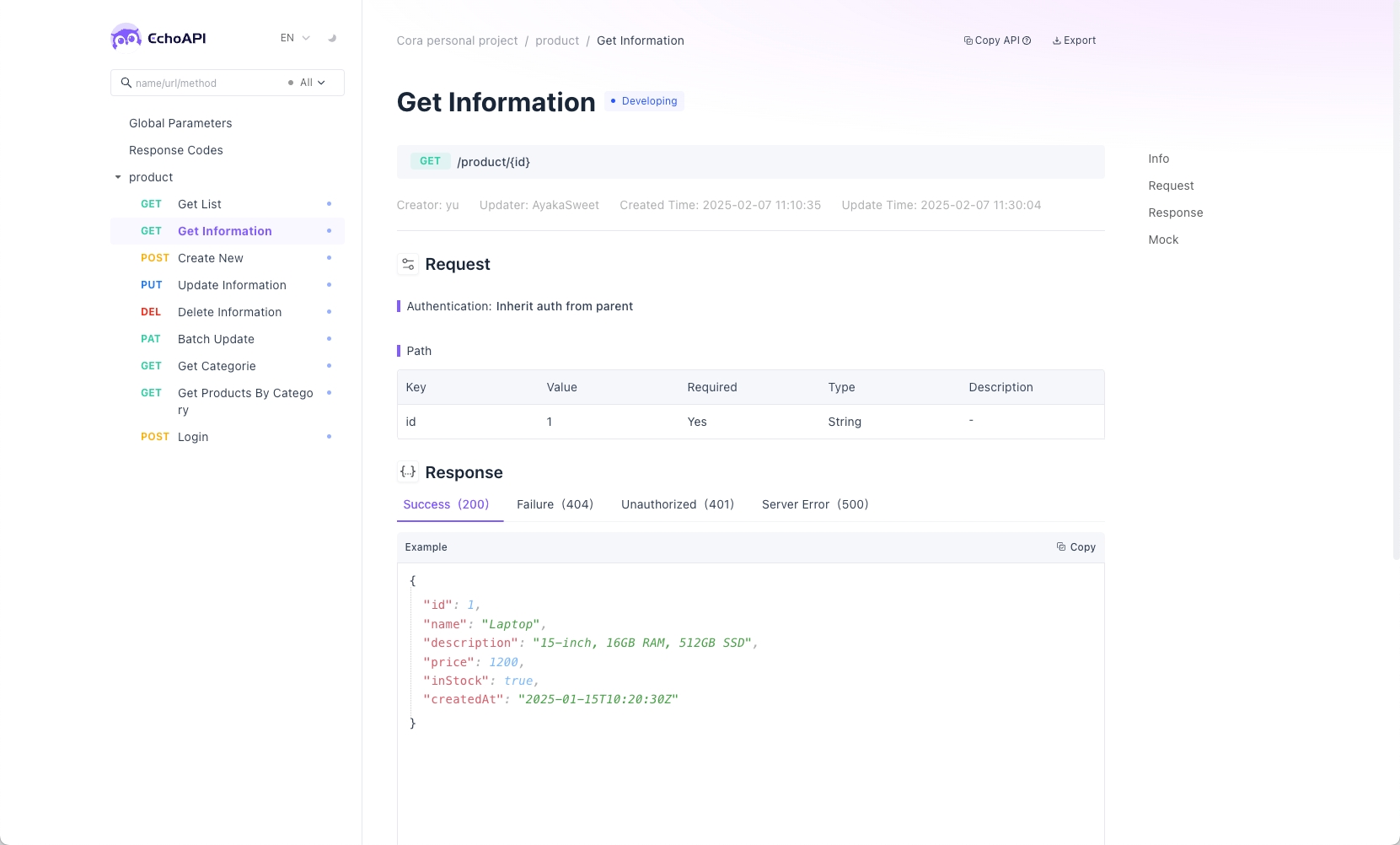
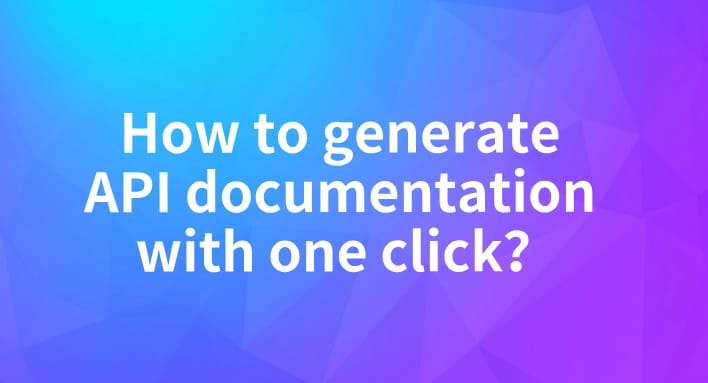
Step 4: Conduct API Testing
Once integration is complete, consider broader end-to-end testing:
- Use EchoAPI’s Automated Testing to test multiple API calls in bulk.
- Leverage automation testing frameworks like RestAssured for advanced test scenarios, including authentication, headers, and performance benchmarks.
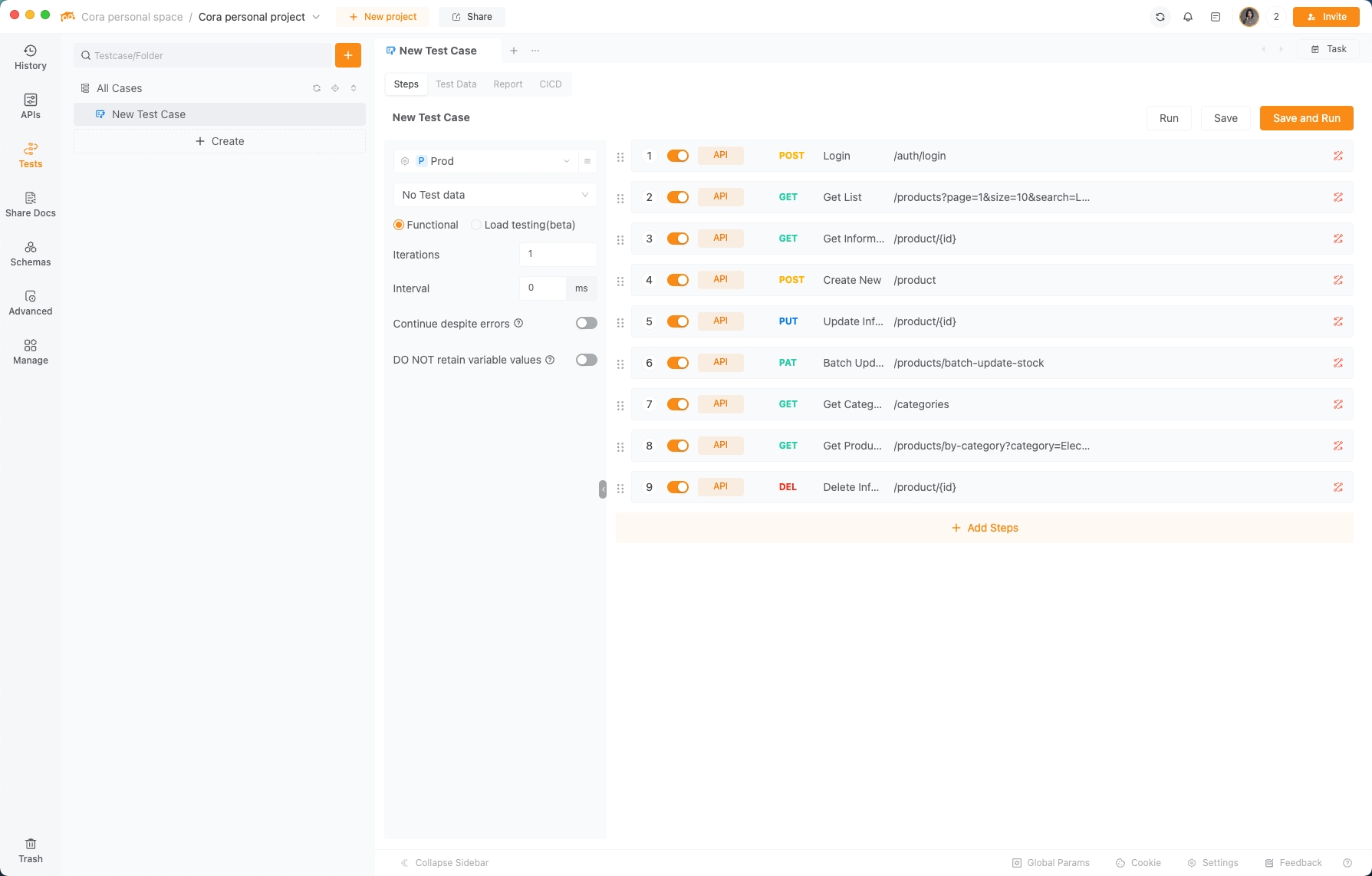
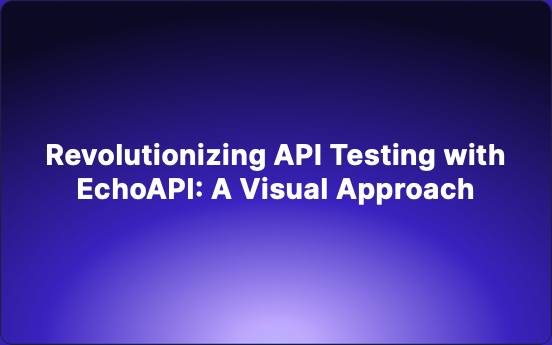
Step 5: Final Testing and Deployment
After thorough unit, API, and end-to-end testing, deploy the application. Use tools like EchoAPI for CI/CD pipelines. Testing doesn’t end here—monitor performance using logging tools such as ELK Stack or Grafana to ensure everything runs seamlessly.
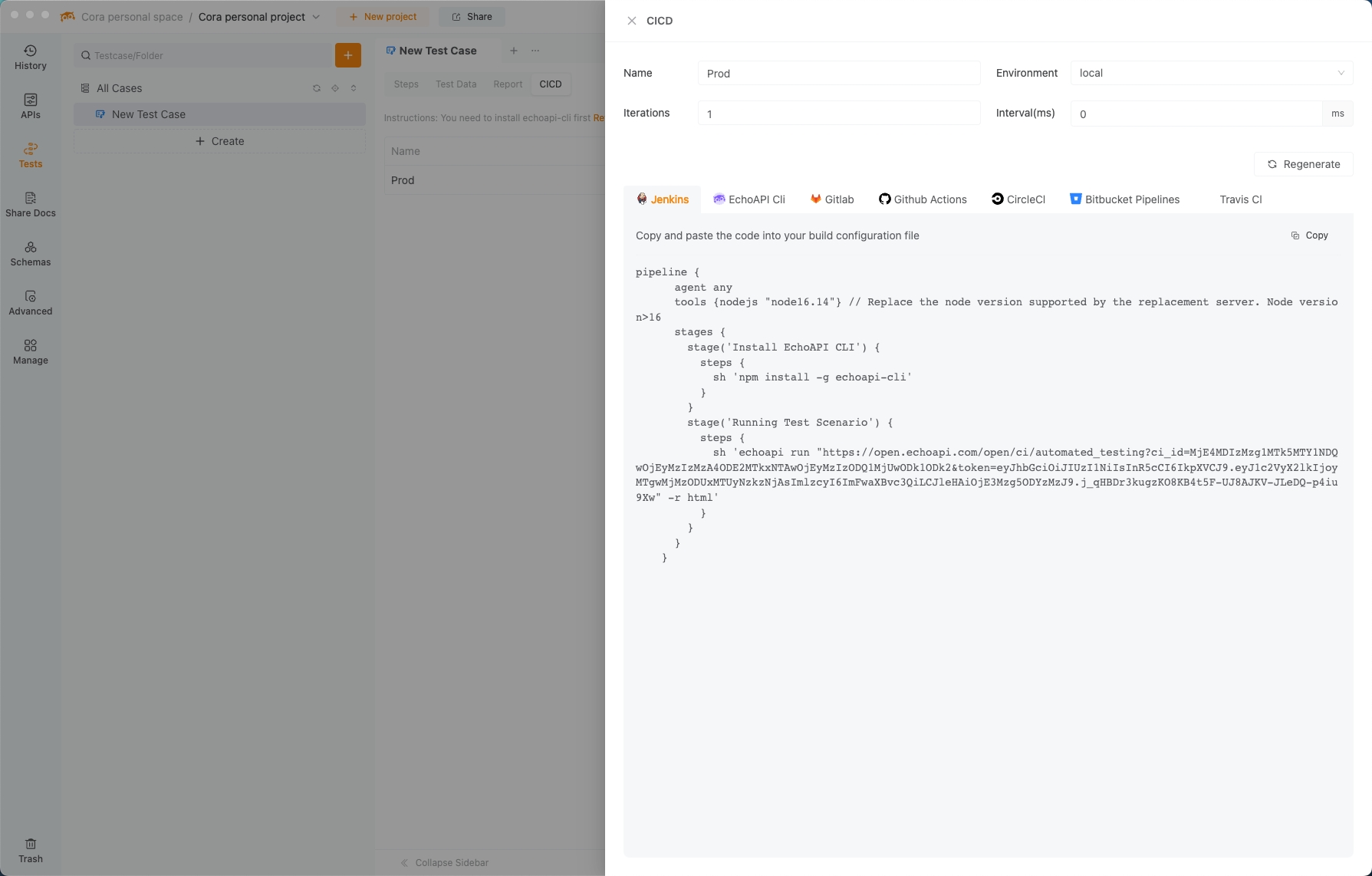
Conclusion
From developing robust backend systems to testing APIs and documenting endpoints, Java developers rely on a full suite of tools to ensure success at every stage of the development lifecycle.
We started by writing Java code in IntelliJ IDEA, tested our APIs using JUnit and EchoAPI, documented endpoints with EchoAPI, and verified complete workflows using a combination of automation tools. Together, these tools bring efficiency, reliability, and scalability to the table.
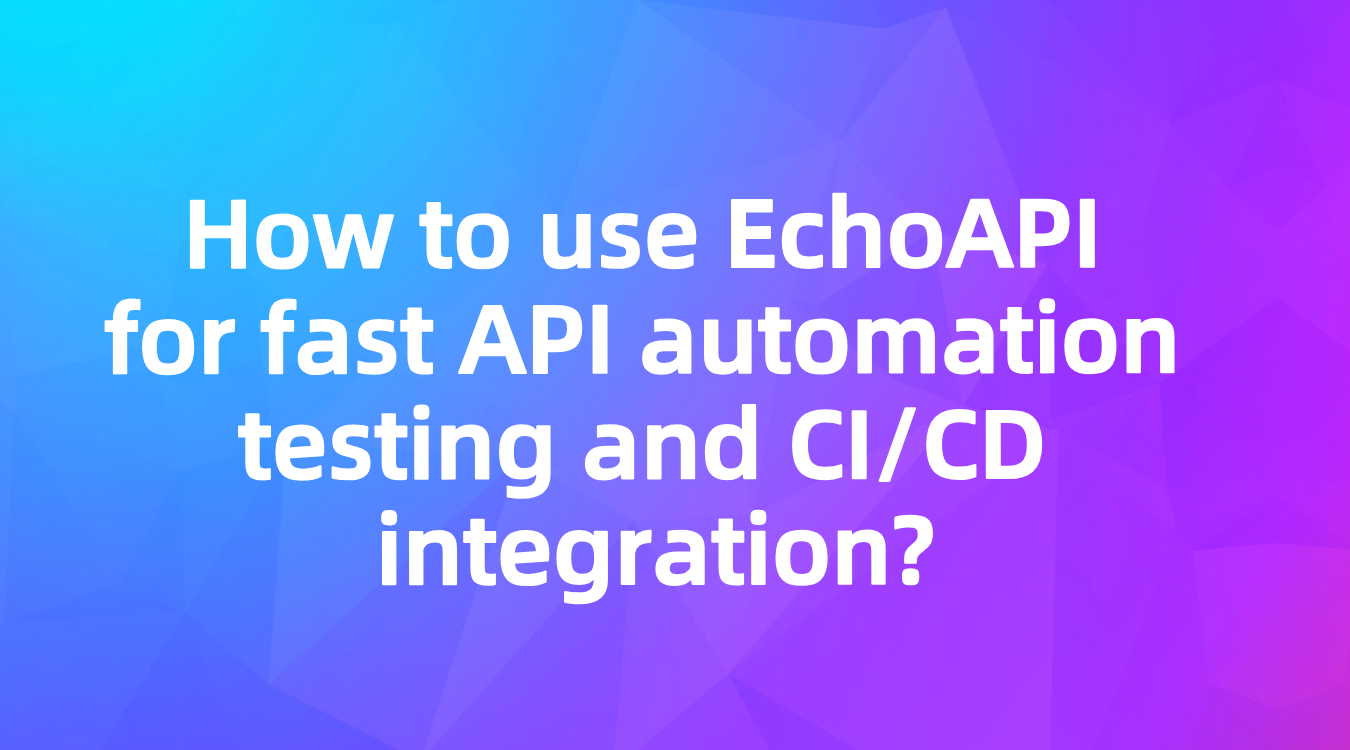
In today’s fast-paced development world, mastering the full cycle of Java development and testing allows you to deliver high-quality applications with confidence—every single time. So equip yourself with the right tools, refine your skills, and start building!