From Zero to API Hero: A Beginner’s Guide to API Design and Development
APIs aren’t rocket science; they’re just a way for apps to talk to each other. If you’ve ever used a weather app that pulls data from another service, you’ve already interacted with an API.
APIs Aren’t Magic—They’re Just LEGO Blocks for Grown-Ups
Let’s start with a hard truth: APIs sound intimidating because we’ve made them sound that way. Developers toss around terms like “RESTful endpoints” and “OAuth flows” like they’re ordering coffee. Meanwhile, beginners stare at documentation like it’s hieroglyphics.
But here’s the twist: APIs are simpler than you think. Imagine building with LEGO—every block (or API endpoint) has a purpose, and you snap them together to create something functional. APIs aren’t rocket science; they’re just a way for apps to talk to each other. If you’ve ever used a weather app that pulls data from another service, you’ve already interacted with an API.
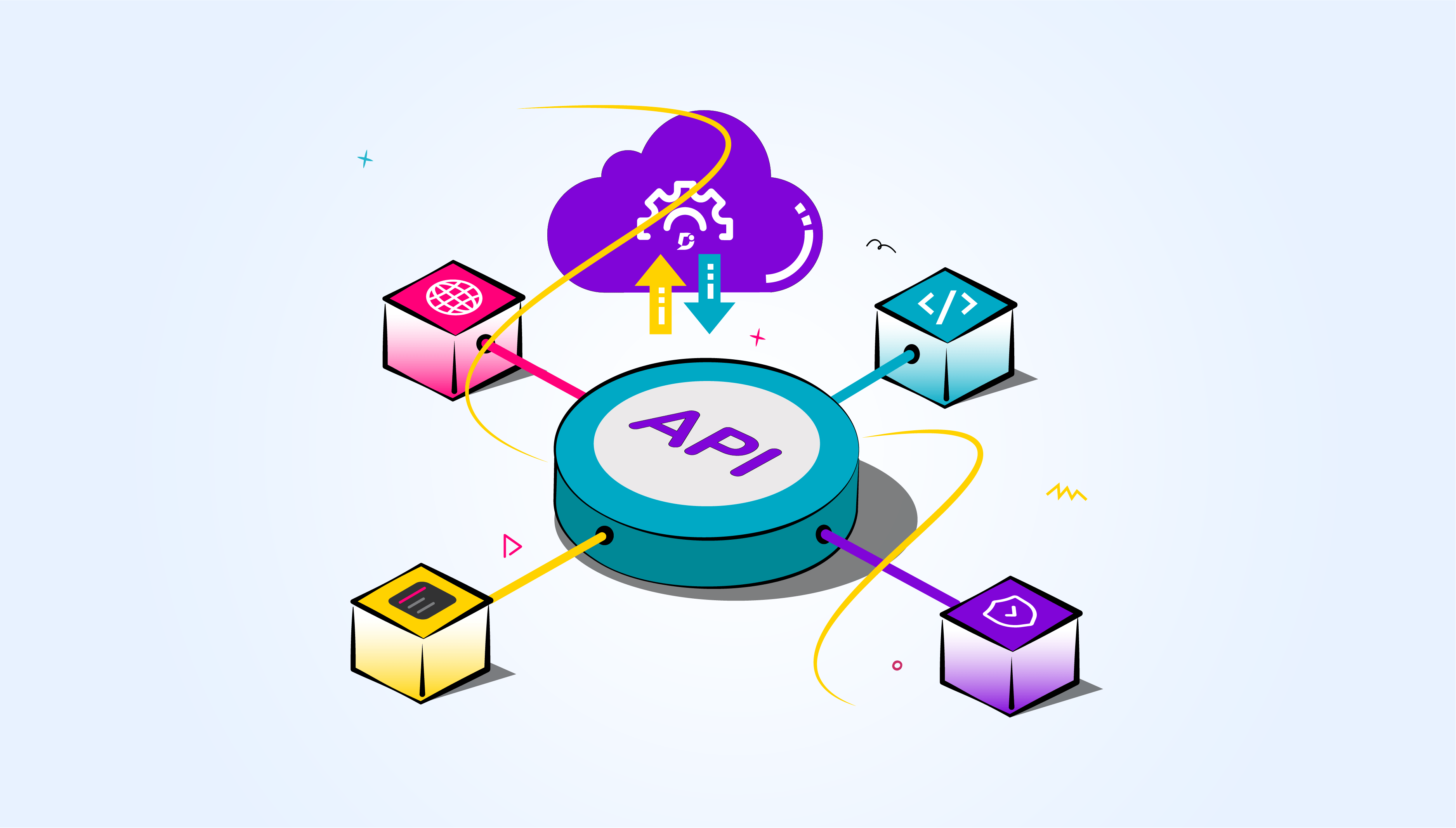
Still skeptical? Let’s demystify APIs step by step—no jargon, no fluff. By the end, you’ll design, test, and even debug your first API.
What Even Is an API? (The 60-Second Crash Course)
An API (Application Programming Interface) is a rulebook for how software components interact. Think of it like a waiter in a restaurant: you (the user) give an order (request), the waiter (API) takes it to the kitchen (server), and brings back your food (response).
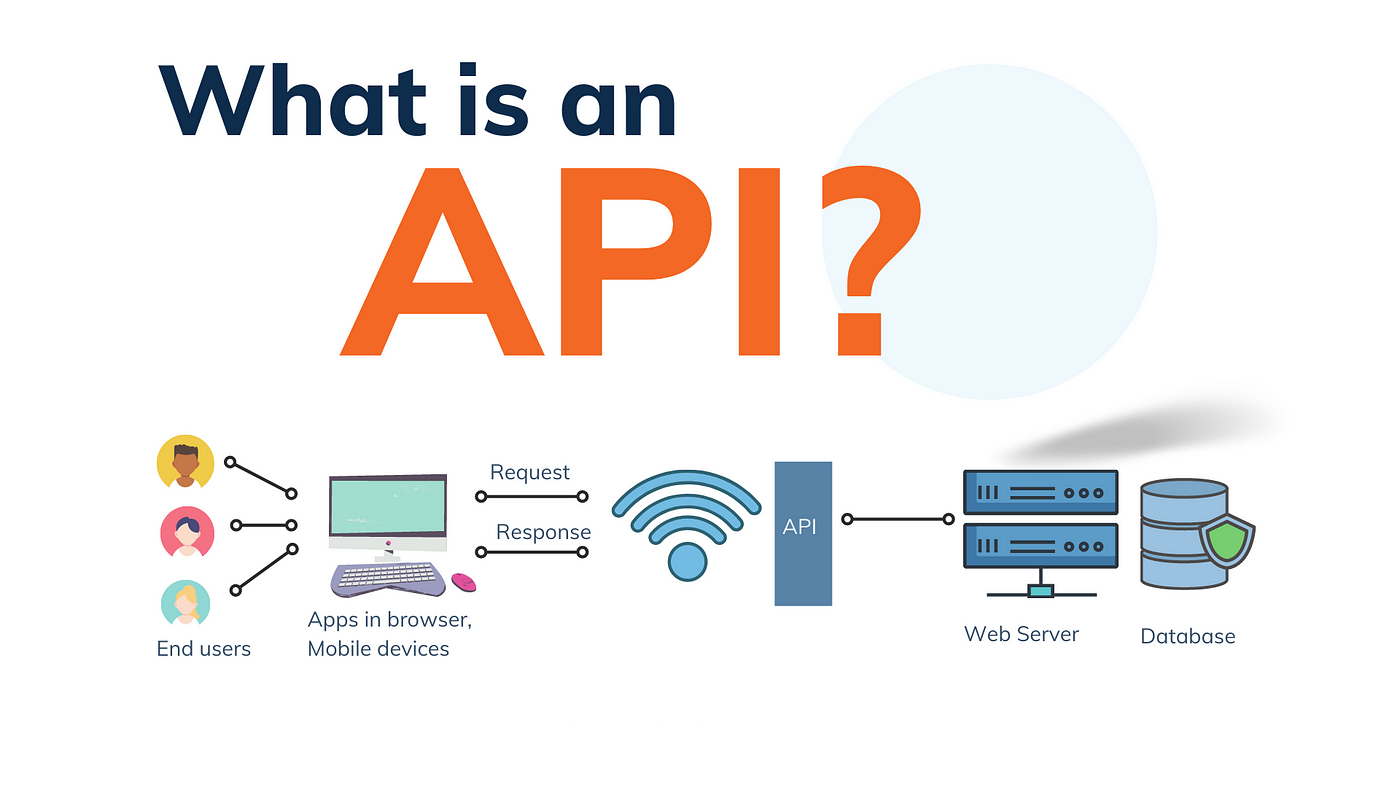
Key Concepts in Plain English:
- Endpoint: A URL where your API “lives” (e.g.,
https://api.example.com/users
). - HTTP Methods: Actions like
GET
(fetch data),POST
(create data),PUT
(update data),DELETE
(remove data). - Request/Response: Send a request (e.g., “Get me user #123”), receive a response (e.g.,
{ "name": "Alice", "id": 123 }
).
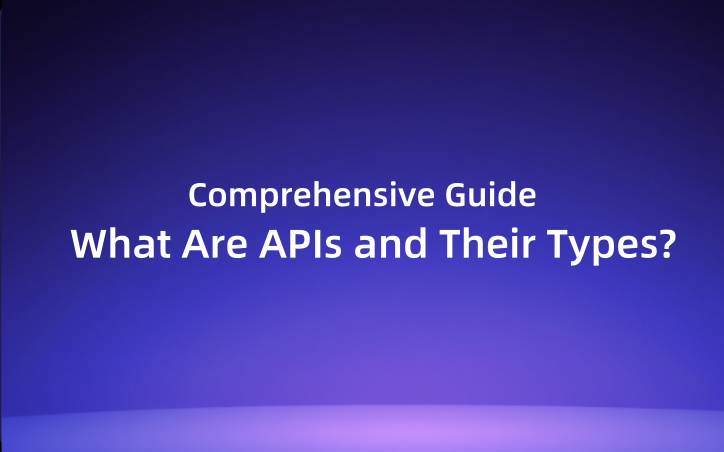
Designing Your First API (Like a Pro)
Let’s build a simple “To-Do List” API. We’ll use RESTful principles because they’re beginner-friendly and widely adopted.
Step 1: Plan Your Endpoints
Define what your API should do:
- GET /tasks: Fetch all tasks.
- GET /tasks/{id}: Fetch a single task.
- POST /tasks: Create a new task.
- PUT /tasks/{id}: Update a task.
- DELETE /tasks/{id}: Remove a task.
Step 2: Structure Your Data
Decide what a “task” looks like. Example JSON format:
{
"id": 1,
"title": "Buy milk",
"completed": false
}
Step 3: Build a Mock API
No coding yet! Use EchoAPI to simulate your API instantly:
- Go to EchoAPI.
- Create a new HTTP.
- Define your endpoints and sample responses.
- Share the mock URL with teammates or test it yourself.
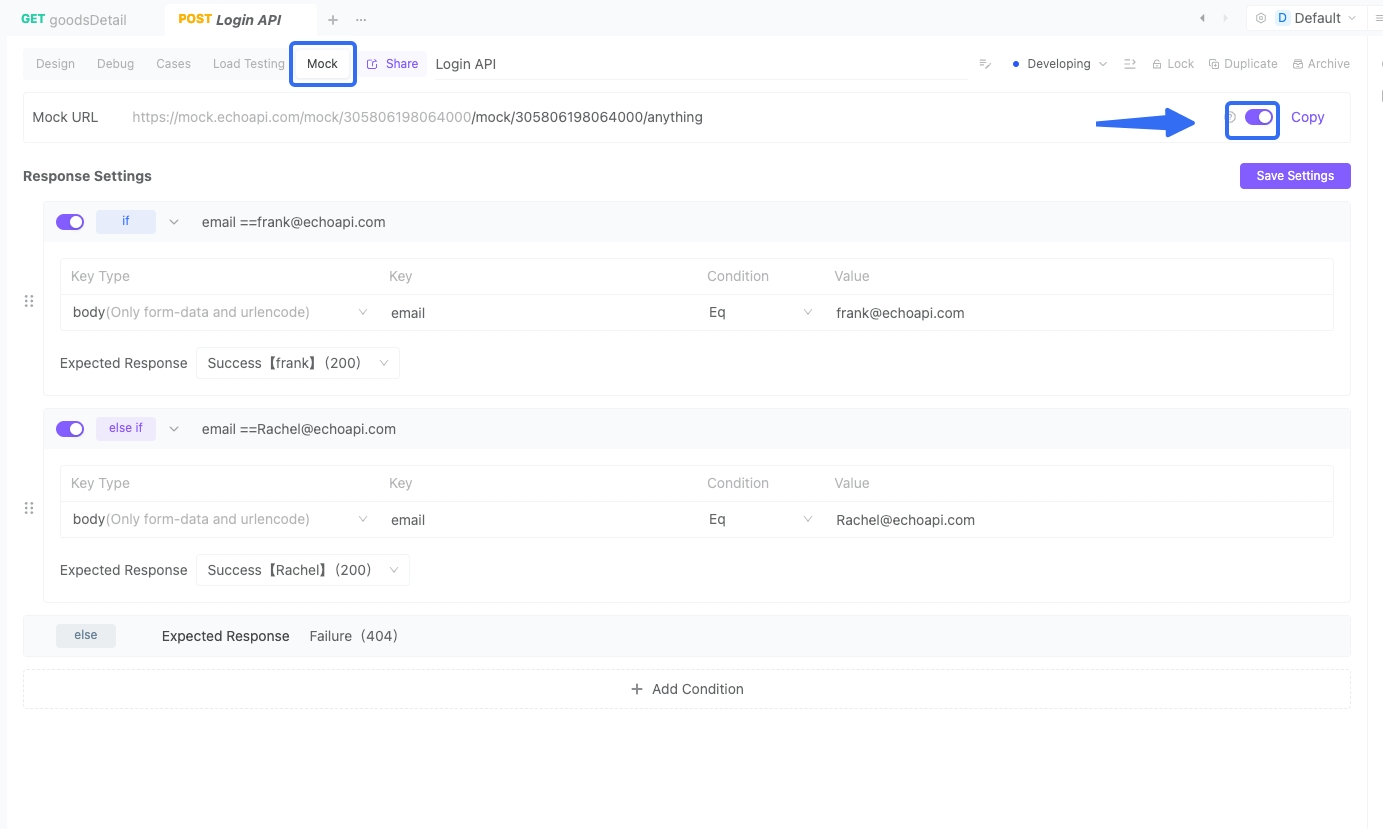
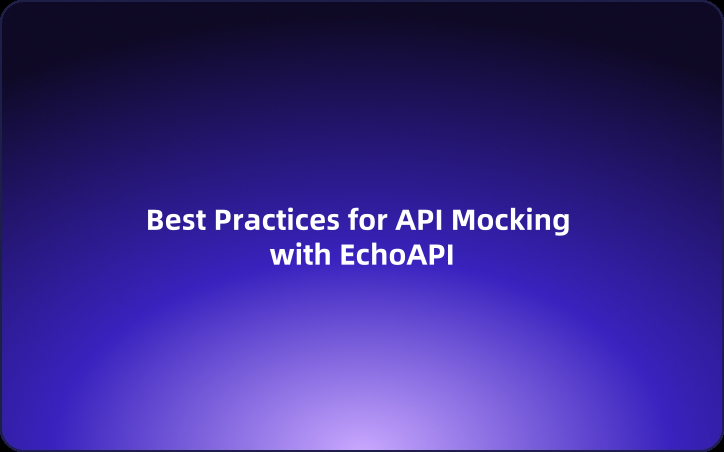
Why EchoAPI? It’s free, requires no setup, and lets you validate your design before writing code.
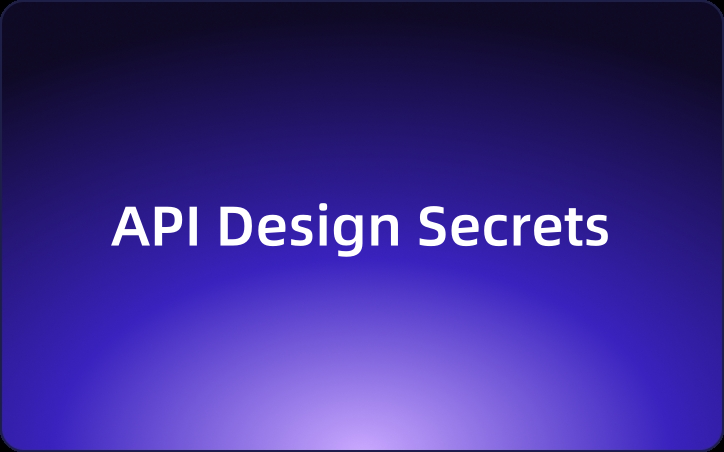
Testing & Debugging (Without Losing Your Mind)
Testing APIs is where most beginners get stuck. Let’s fix that.
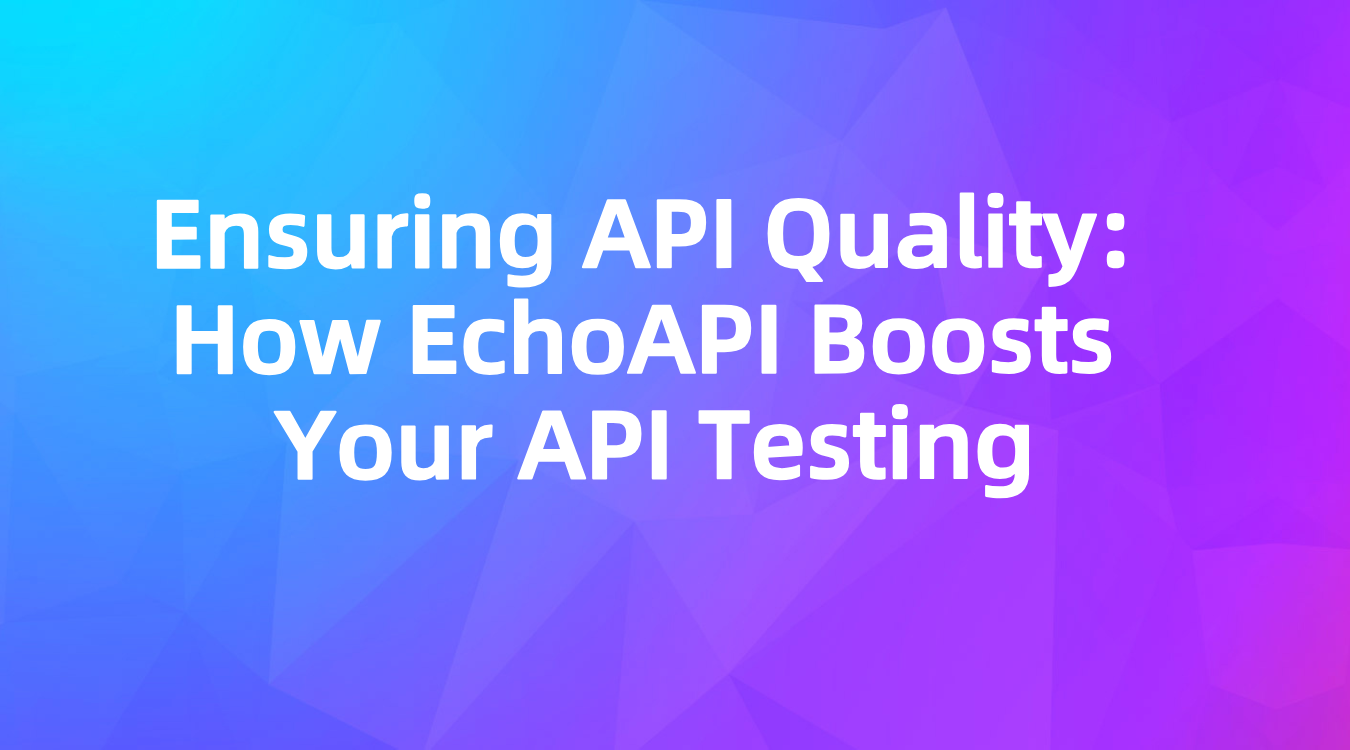
Testing with EchoAPI
- Use EchoAPI’s Live Testing feature to send requests to your mock API.
- Check if responses match your expectations.
- Debug errors instantly with detailed logs (no guesswork!).
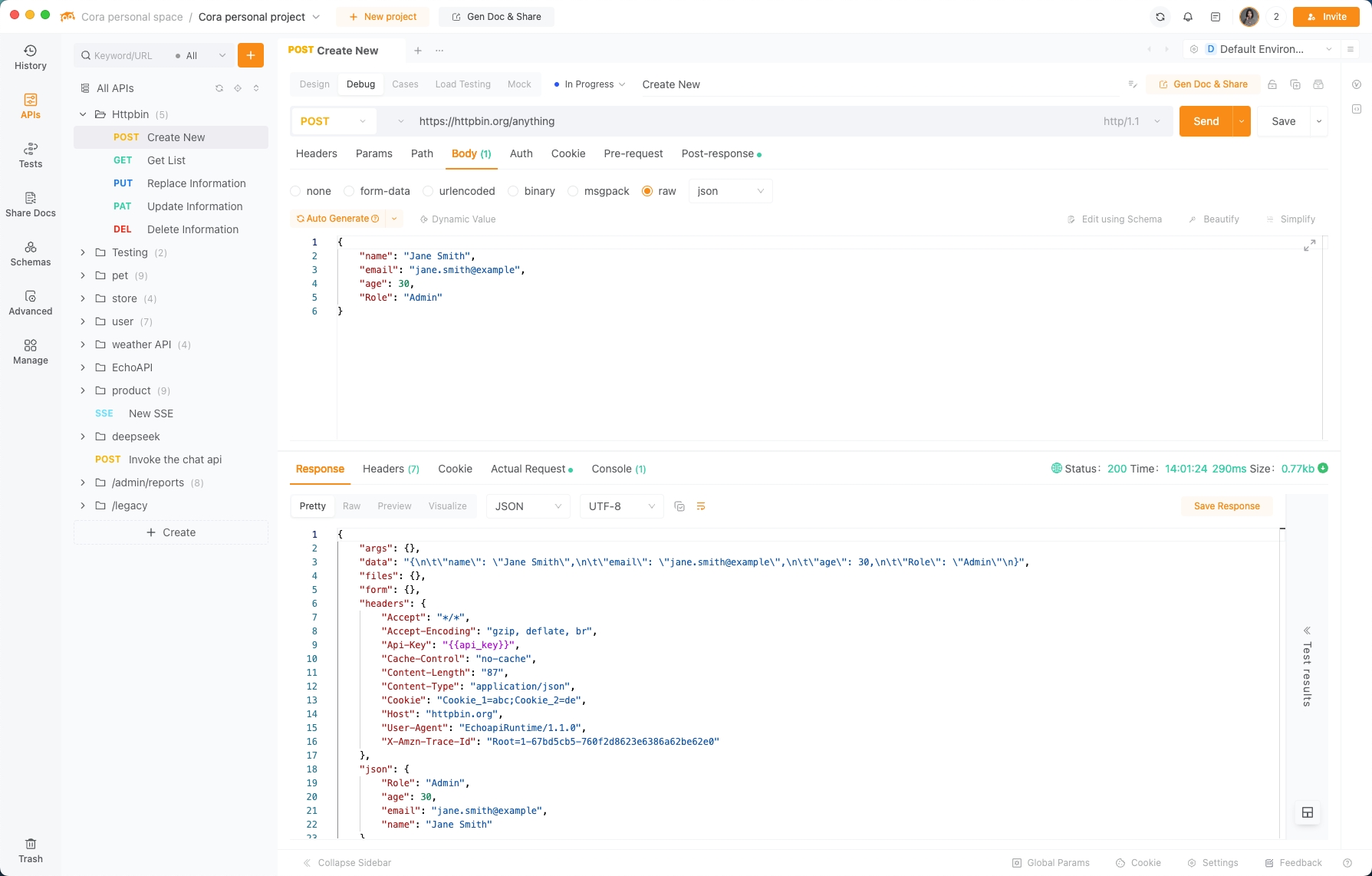
Common Pitfalls (and Fixes):
- 404 Not Found: Did you spell the endpoint wrong? Double-check the URL.
- 500 Server Error: Likely a bug in your backend code. Use EchoAPI’s logs to trace the issue.
- Slow Responses: Optimize your database queries or caching.
You’re Now an API Architect
Let’s recap:
- APIs are just rulebooks for software communication—no magic involved.
- Design endpoints first, mock them with EchoAPI, then build.
- Test relentlessly; tools like EchoAPI turn debugging from nightmare to checklist.
The best part? You don’t need to be a senior developer to work with APIs. Start small, iterate, and remember: even Netflix and Spotify started with a single endpoint.
Ready to build something awesome? Grab EchoAPI and turn your ideas into working APIs today.