Harnessing the Power of HTTP/2 for Modern Web Development
This article delves into the essence of HTTP/2, its distinctive characteristics, and its advantages over the previous versions, with practical insights and an example scenario demonstrating its practical implementations.
In the rapidly evolving world of web development, staying updated with the latest technologies is crucial. While the necessity of understanding GraphQL remains high among developers for efficient API interactions, grasping the significance of HTTP/2, the successor to HTTP/1.1, is equally important.
What is HTTP/2?
HTTP/2 is a major revision of the HTTP network protocol, primarily developed by the IETF’s HTTP Working Group. Officially standardized in May 2015, it was based on SPDY, an earlier experimental protocol by Google. HTTP/2 was designed to address some of the notable shortcomings of HTTP/1.1, particularly around performance—specifically, the way browsers and servers communicate.
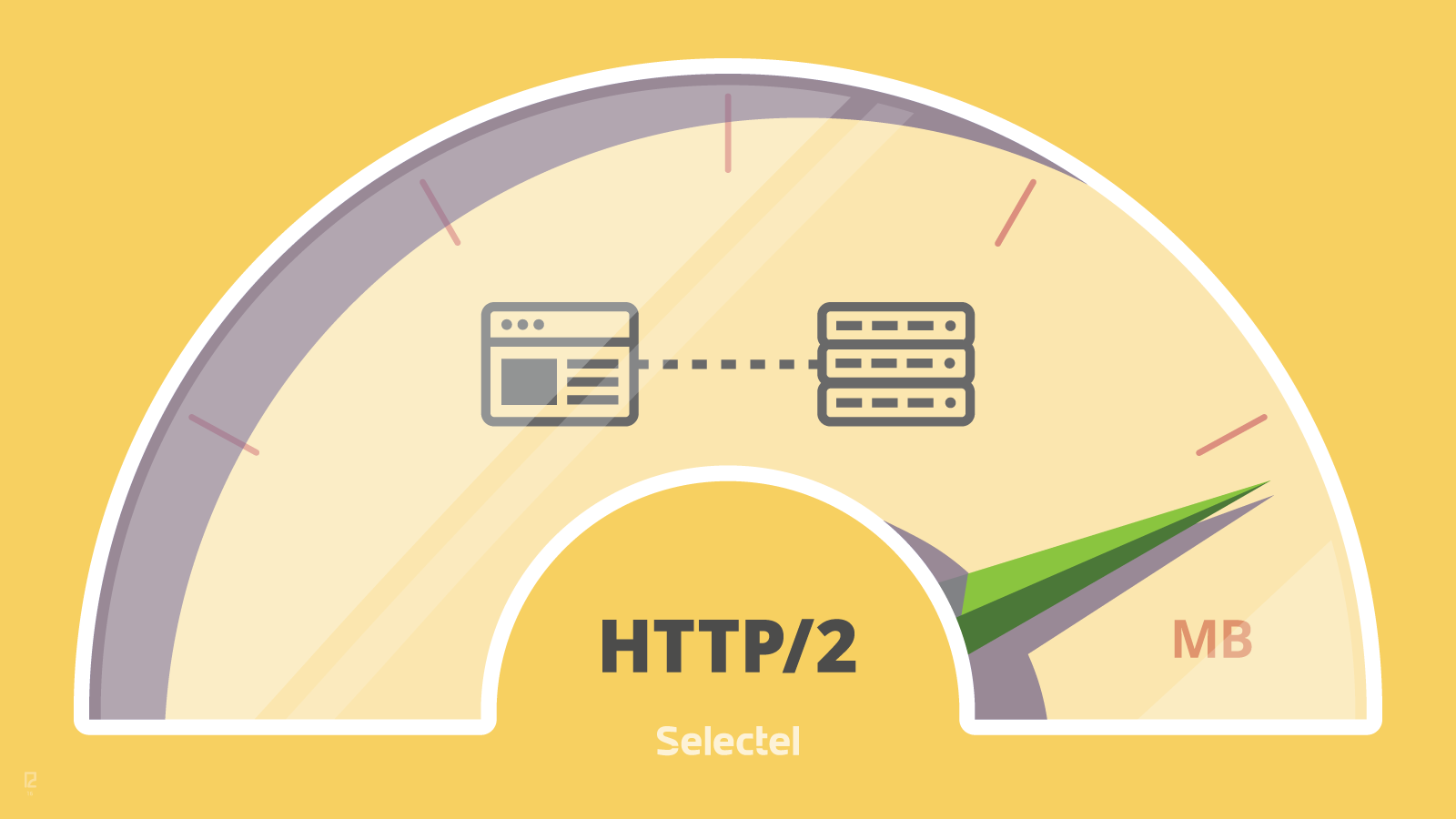
Key Features of HTTP/2
- Binary Framing Layer: HTTP/2 introduces a binary protocol that is more efficient to parse, more compact to compress, and reduces error-prone text-based parsing.
- Multiplexing: Multiple requests and responses can be sent in parallel over a single TCP connection, avoiding the disadvantages of HTTP/1.x connection management.
- Server Push: Servers can push responses proactively into client caches instead of waiting for a new request for each resource, enhancing load times.
- Header Compression: HTTP/2 uses HPACK compression, which reduces overhead.
- Stream Prioritization: Clients can prioritize requests, letting servers know which resources are more important.
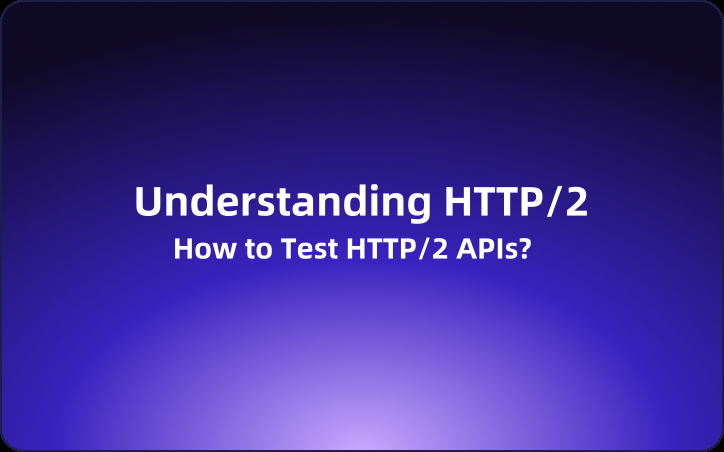
HTTP/2 vs. HTTP/1.x
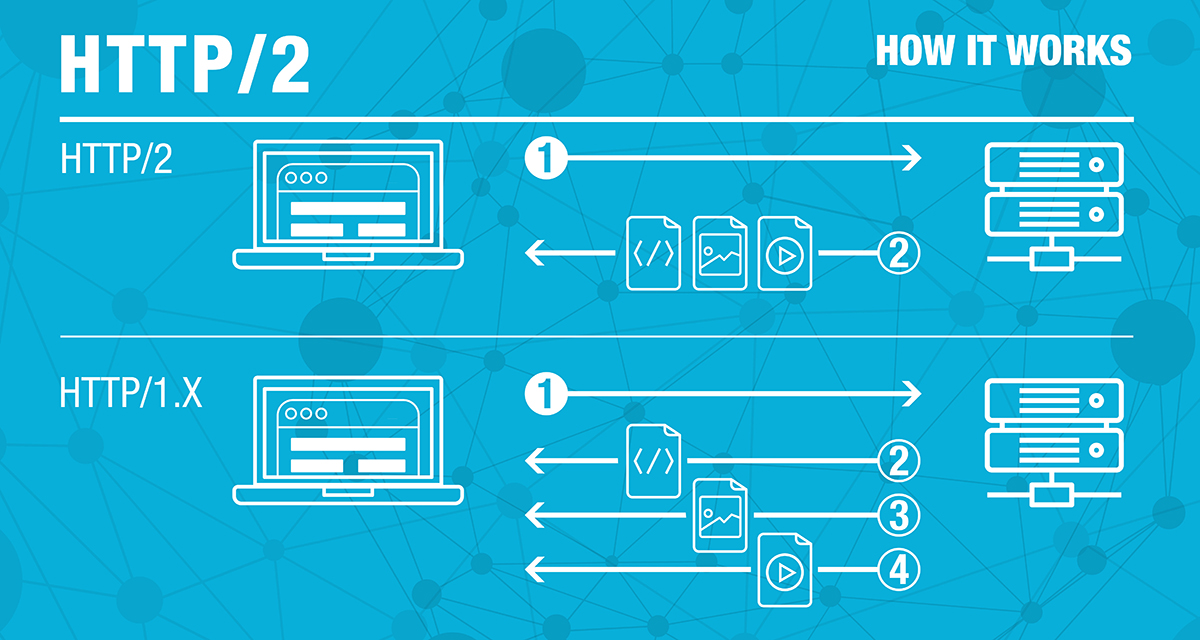
Feature | HTTP/1.x | HTTP/2 |
---|---|---|
Connection Handling | One request per connection | Multiple requests per connection |
Encoding | Textual | Binary |
Compression | Optional, deflate/gzip | Mandatory, HPACK for headers |
Server Push | Not supported | Supported |
Prioritization | Not supported | Supported |
Common Use Cases for HTTP/2
HTTP/2 excels in environments that benefit from reduced latency and improved handling of concurrent connections:
- High-traffic websites
- Web applications with real-time interaction
- APIs serving mobile applications
- Secure applications over TLS (Transport Layer Security)
Practical Business Example: E-commerce Platform
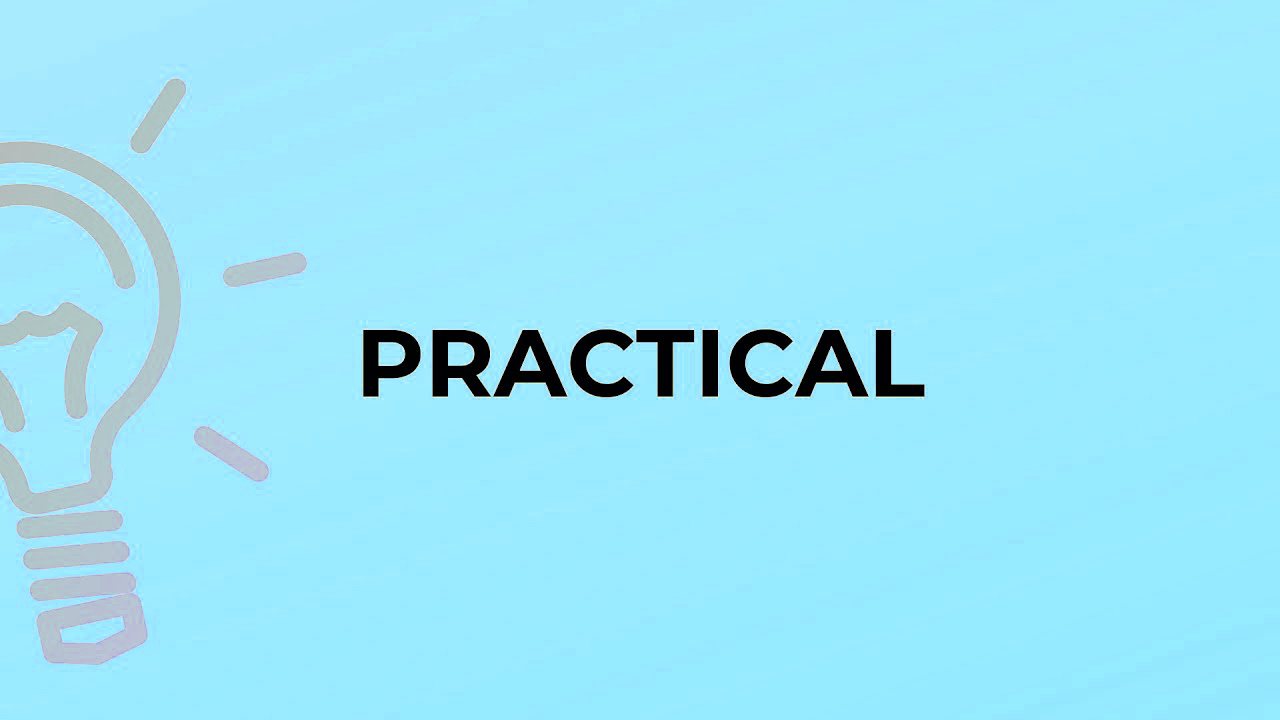
Scenario
An e-commerce platform experiencing slow load times during high-traffic events like sales or product launches.
Benefits of Using HTTP/2
- Reduced Page Load Times: Faster loading times due to multiplexing and server push.
- Improved User Experience: Enhanced interactivity and quicker responses to user actions.
- Cost Efficiency: Reduced resource utilization on server side due to improved management of connections.
Technical Implementation
Sample Implementation in Node.js
const http2 = require('http2');
const server = http2.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello World with HTTP/2');
});
server.listen(3000);
Debugging with EchoAPI
EchoAPI is a comprehensive API debugging tool that now supports HTTP2, enabling developers to test and debug their HTTP/2 communications effectively.
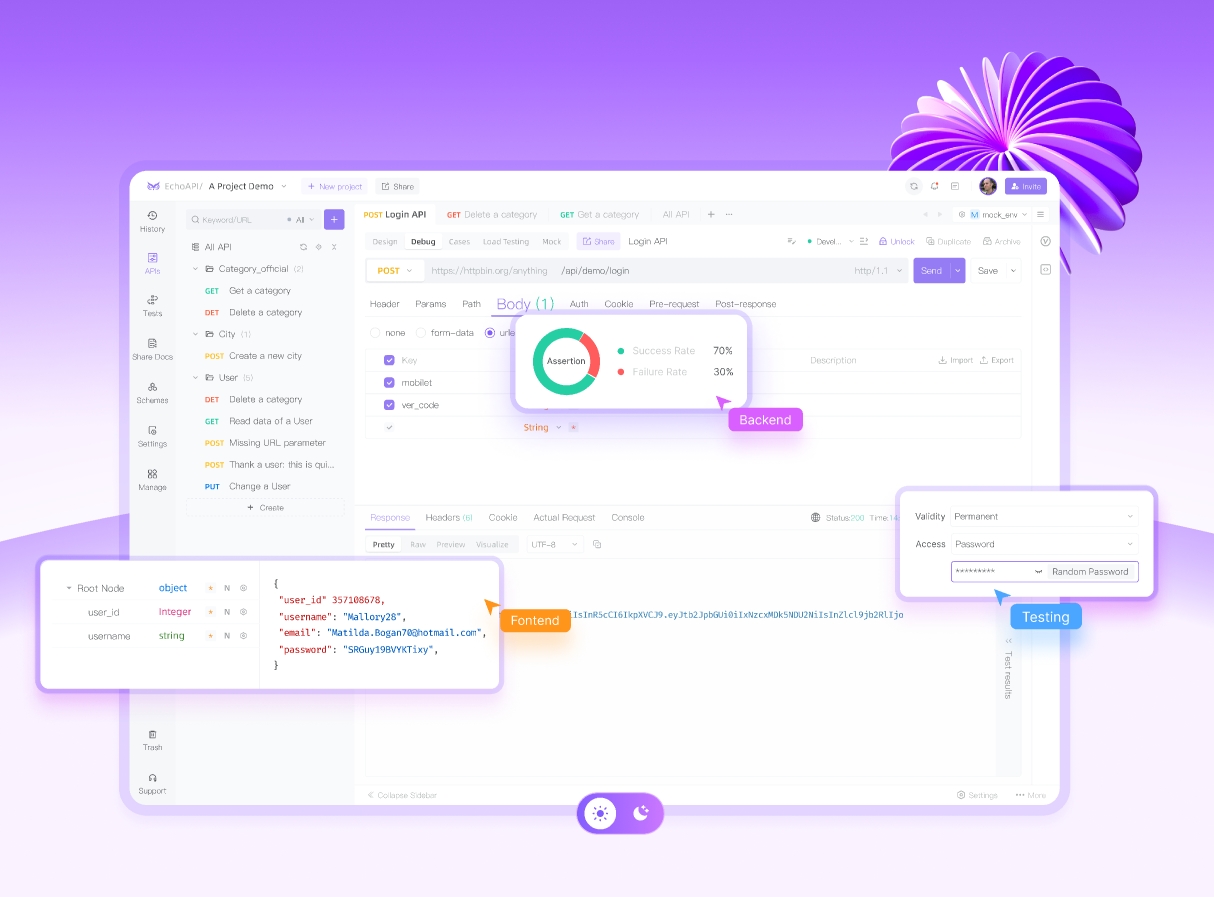
How to Debug HTTP/2 with EchoAPI
Request Address
Method: Supports a dropdown selection of various Method request methods.
URL: You can directly enter the interface's request address in the address bar.
Protocol: Currently supports sending of http1.1 and http2 protocols.
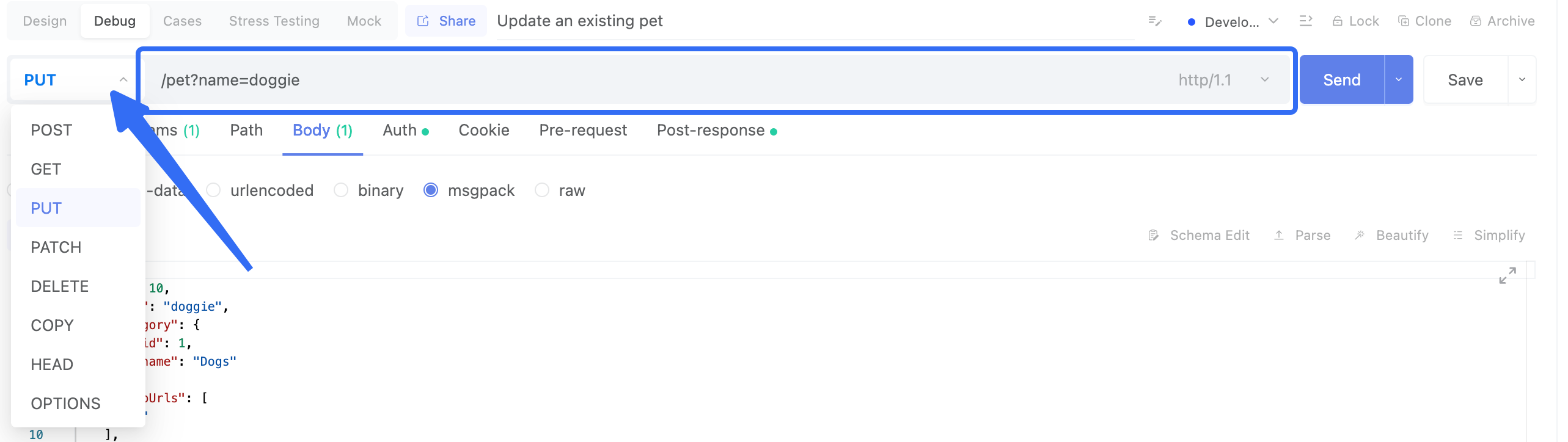
Request Area
You can visually define the interface's request header, query, and other input parameters. Use the global parameter feature to set common parameters across the entire project quickly.
Header
Header input parameters: Support for quickly searching common header parameter names and entering request parameter values.
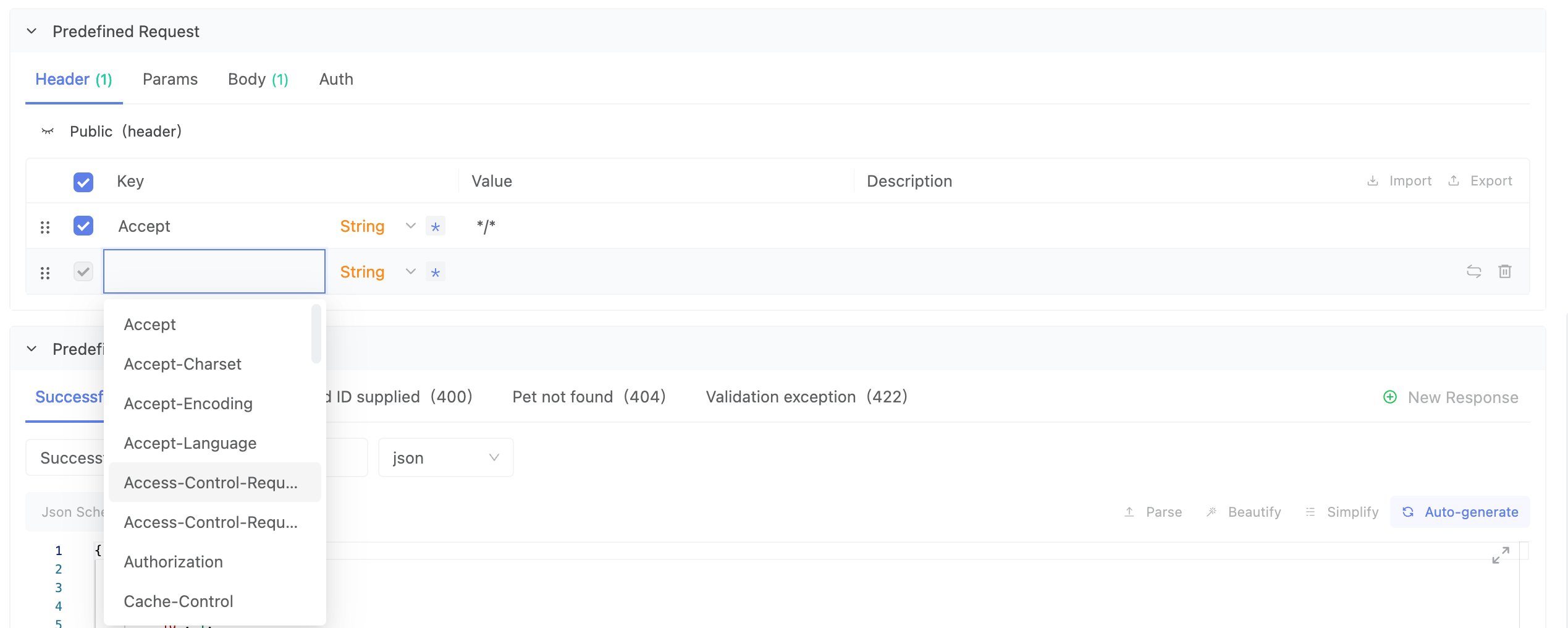
Query
Query input parameters: The set query parameter values will automatically be appended to the URL address; and you can define whether to append an "=" sign when the parameter value is empty through the setting of "=".
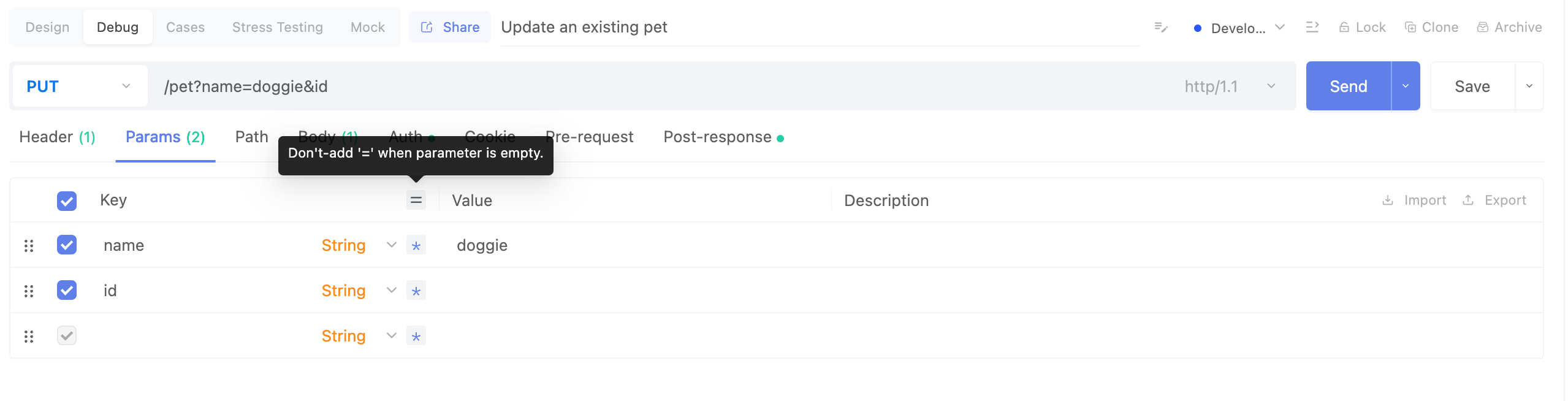
Body
Supports multiple data formats: none
, form-data
, x-www-form-urlencoded
, binary
, msgpack
, raw (json/xml/javascript/plain/html)
.
- none: there is no request body.
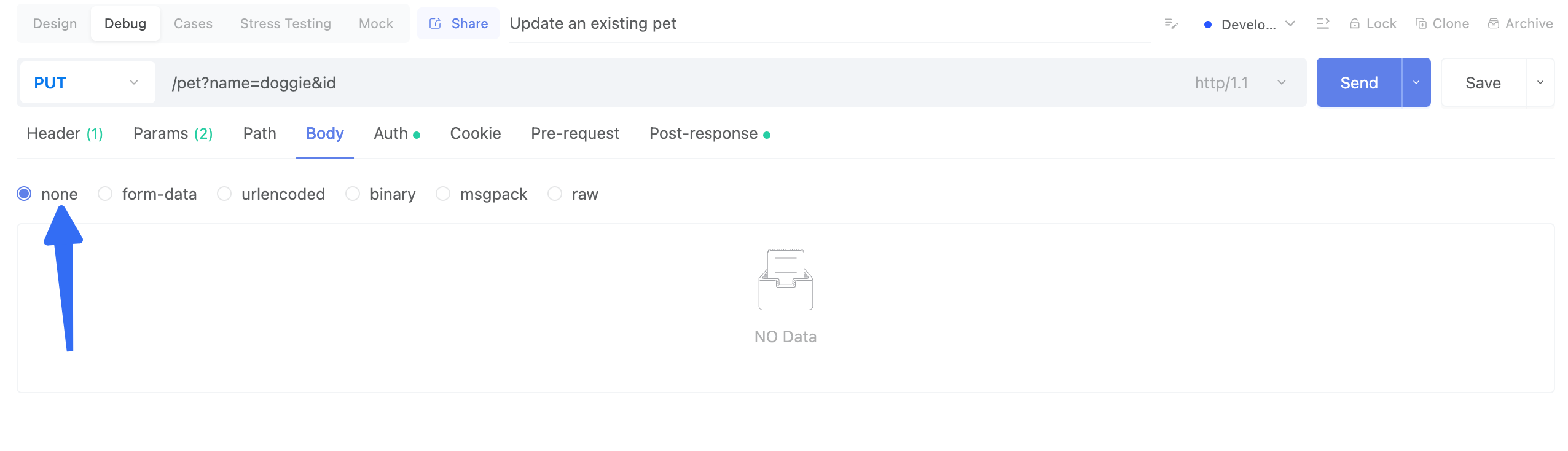
- form-data: supports file uploads and content type selection. Switch to form-data when you need to submit a form with files.
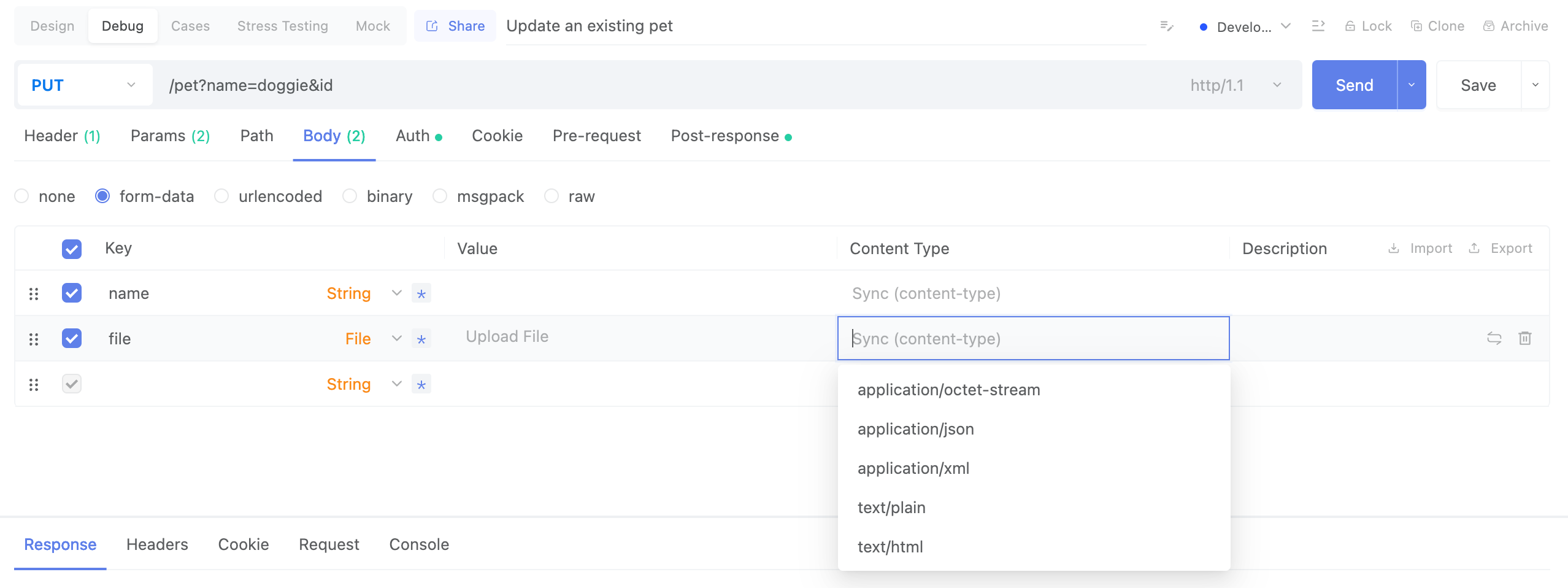
- urlencode (x-www-form-urlencoded): Switch to urlencoded when you need to submit a form.
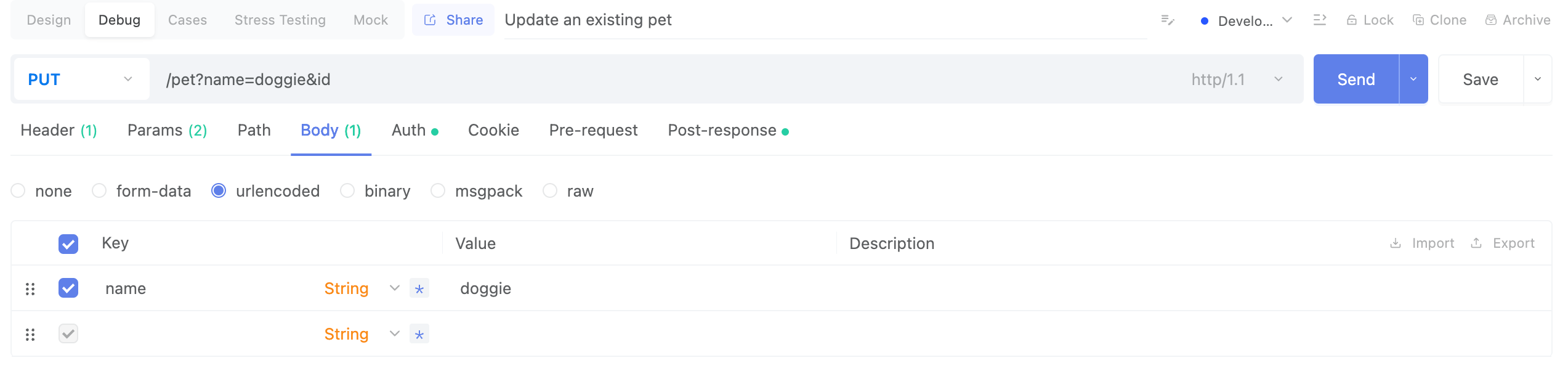
- binary: When you need to upload a binary file
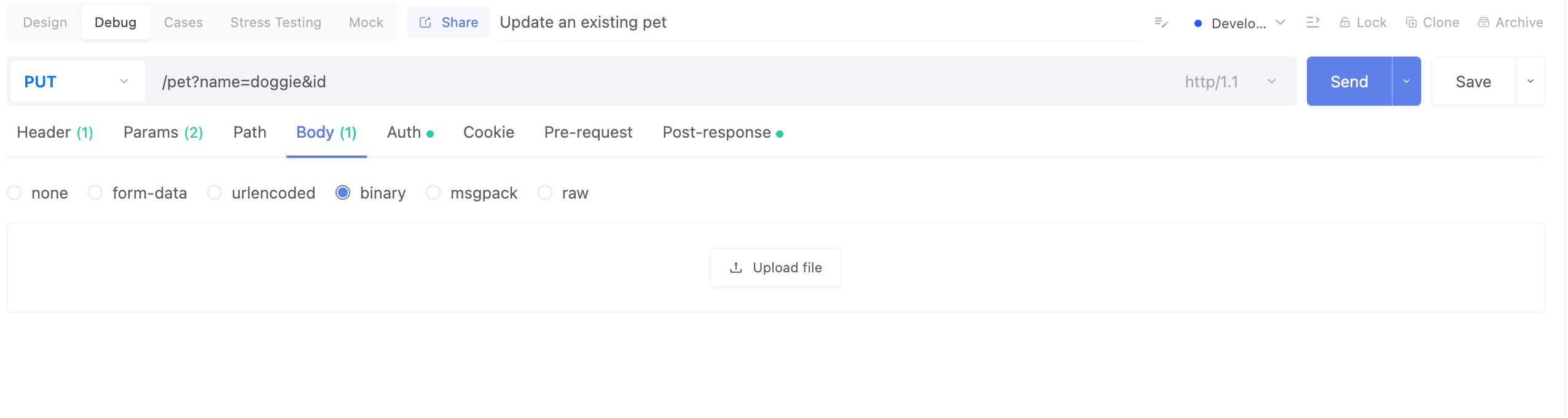
- msgpack
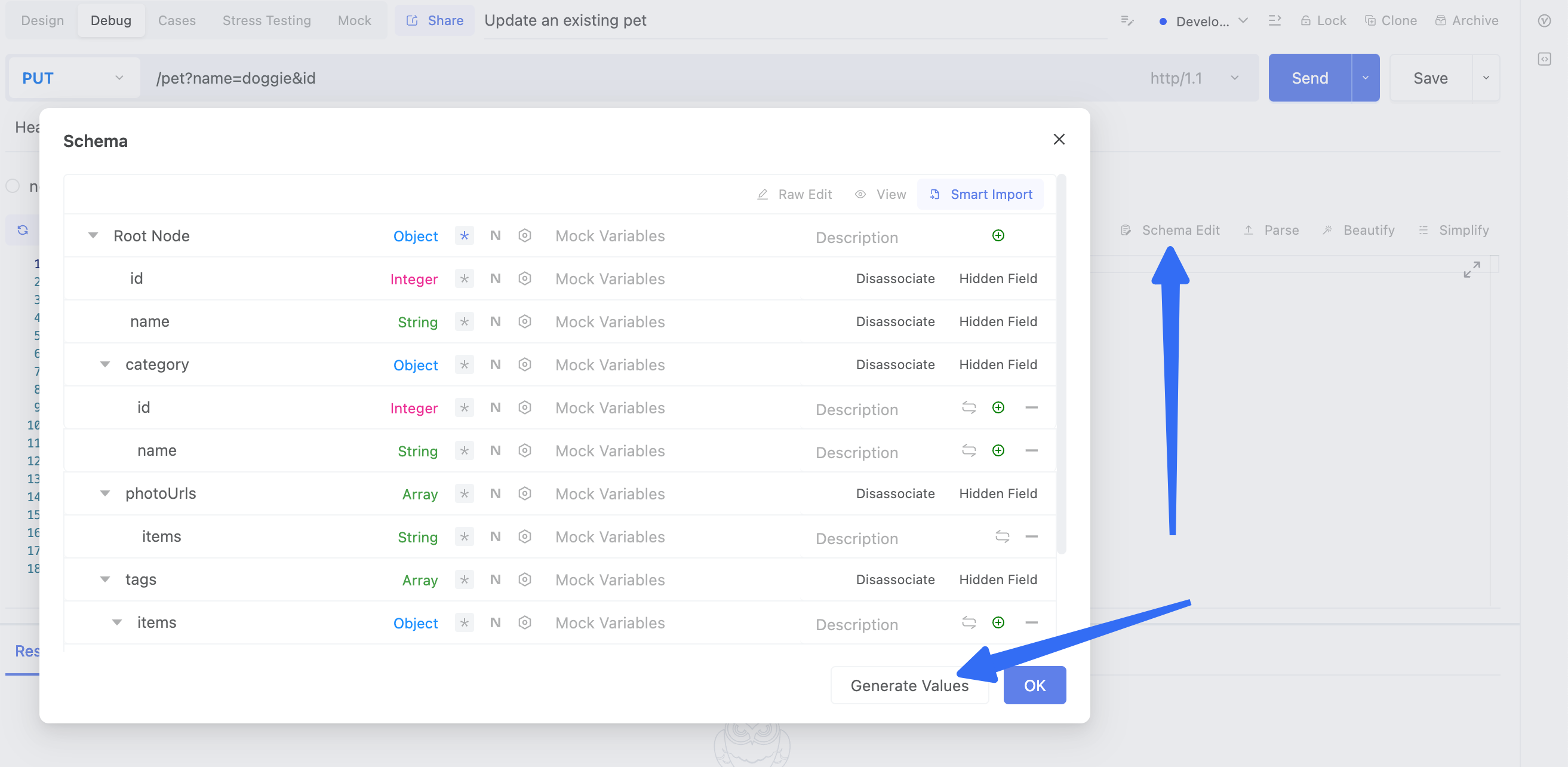
Generate values based on the defined schema.
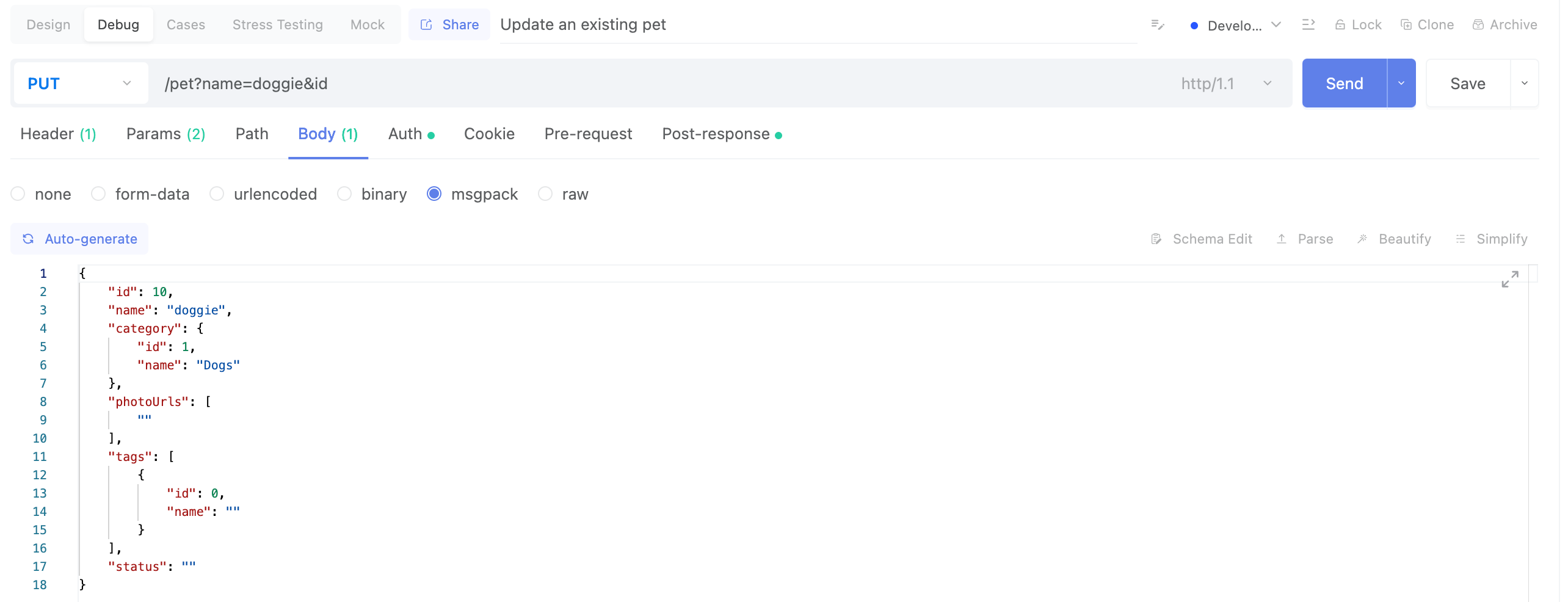
- raw: When you need to send a JSON object or other objects, switch to the corresponding raw type.
- raw (json/xml) , supports schema edit for hierarchical interface parameters in json or xml.
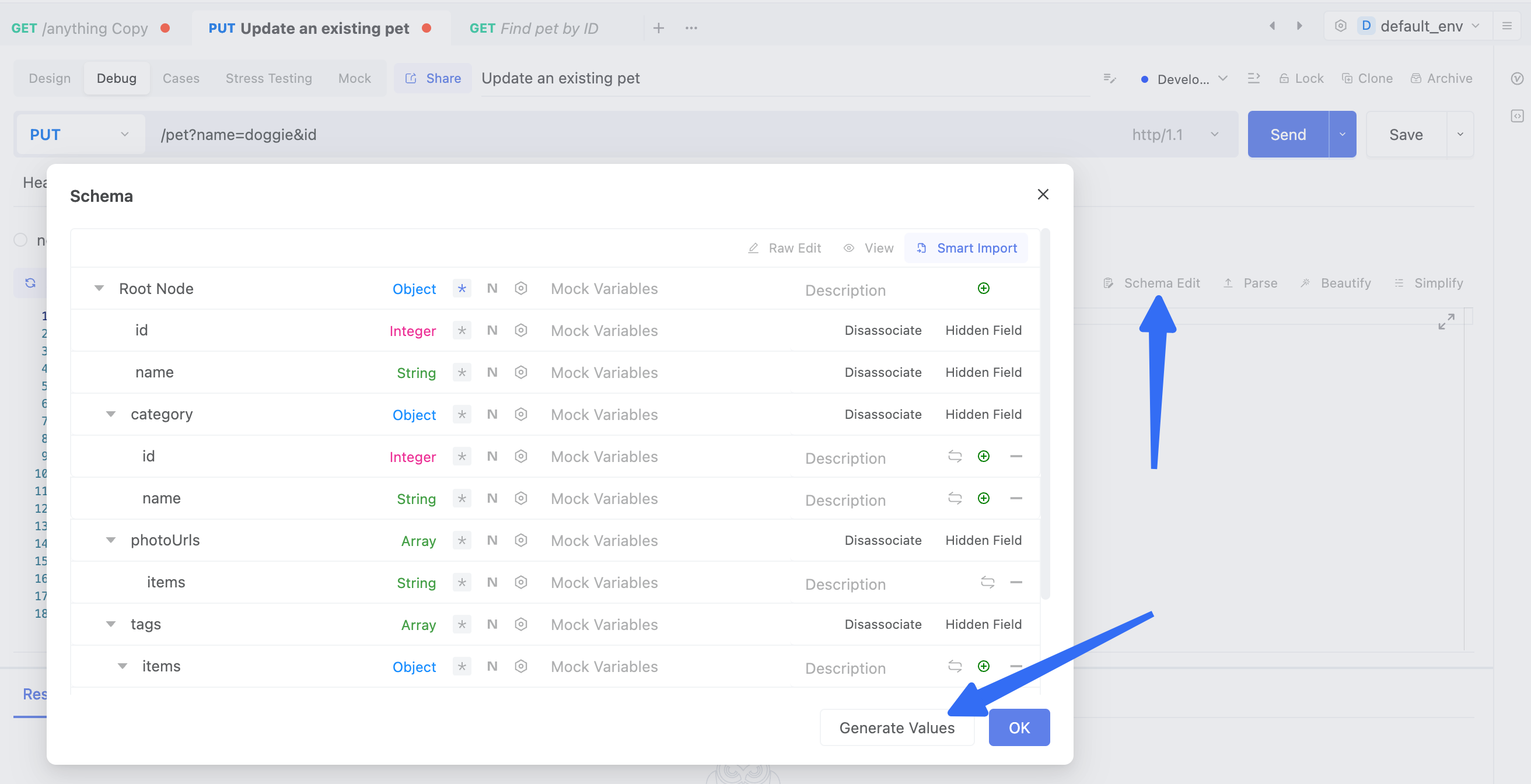
Generate values based on the defined schema.
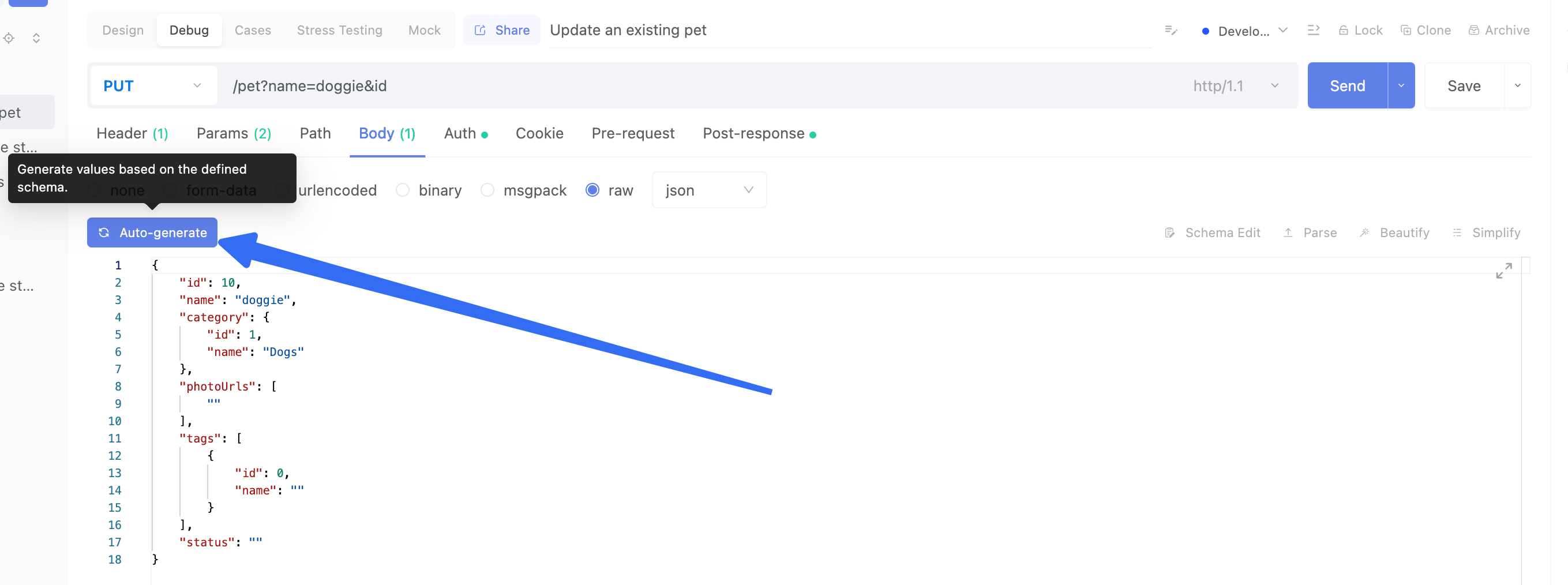
- raw (javascript/plain/html) supports source code editing for designing parameter values.
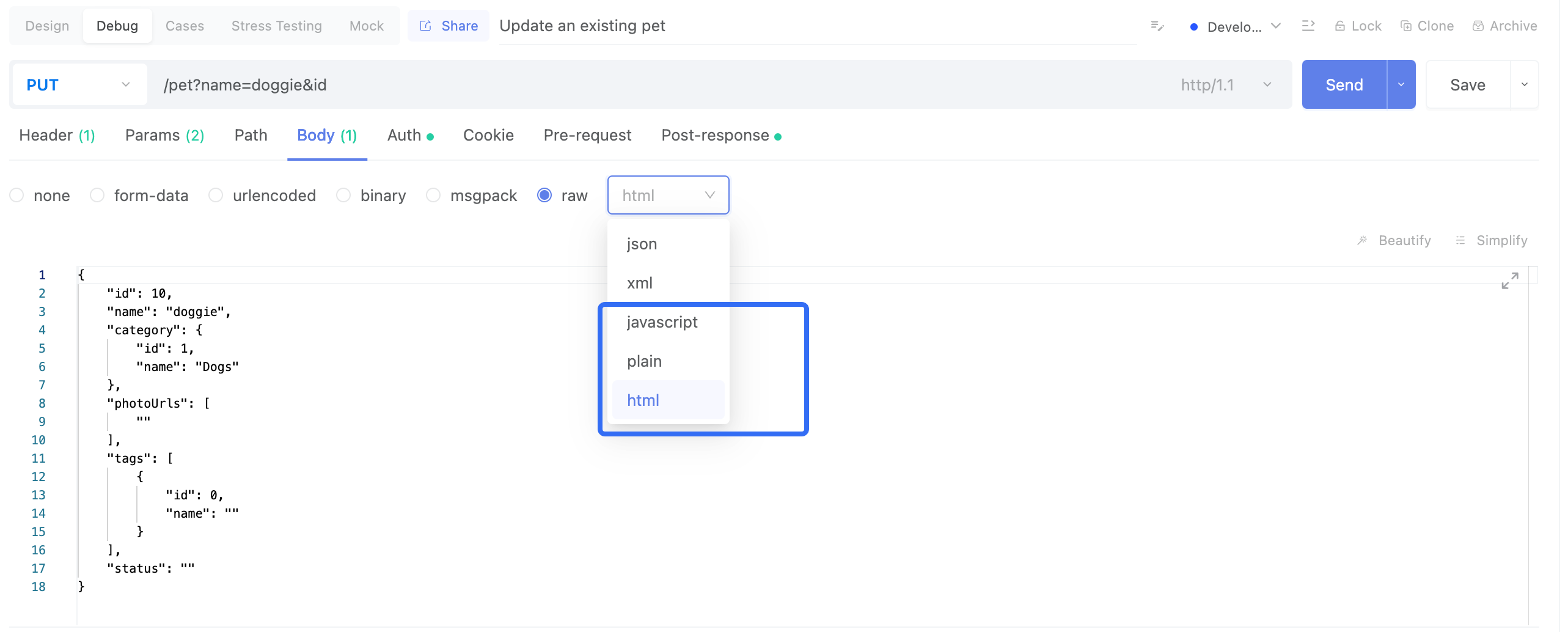
Path
Supports RESTful API design by adding variables in the path using {}
or :
. These variables will be displayed in the input parameters.
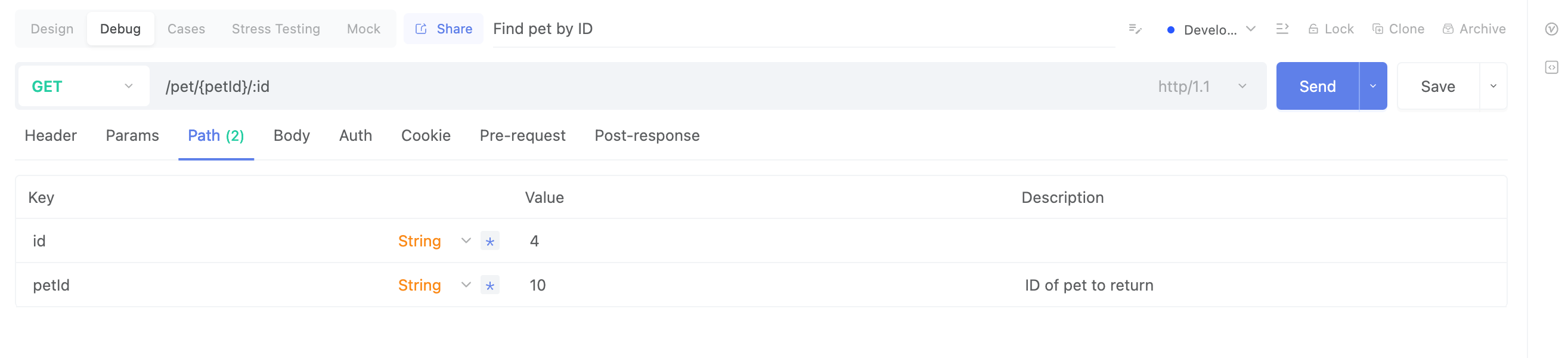
####### Authentication
Supports Bearer token, Basic auth, Digest auth, OAuth 1.0, Hawk authentication, AWS Signature, NTLM Authentication (Beta), Akamai EdgeGrid and other authentication methods.
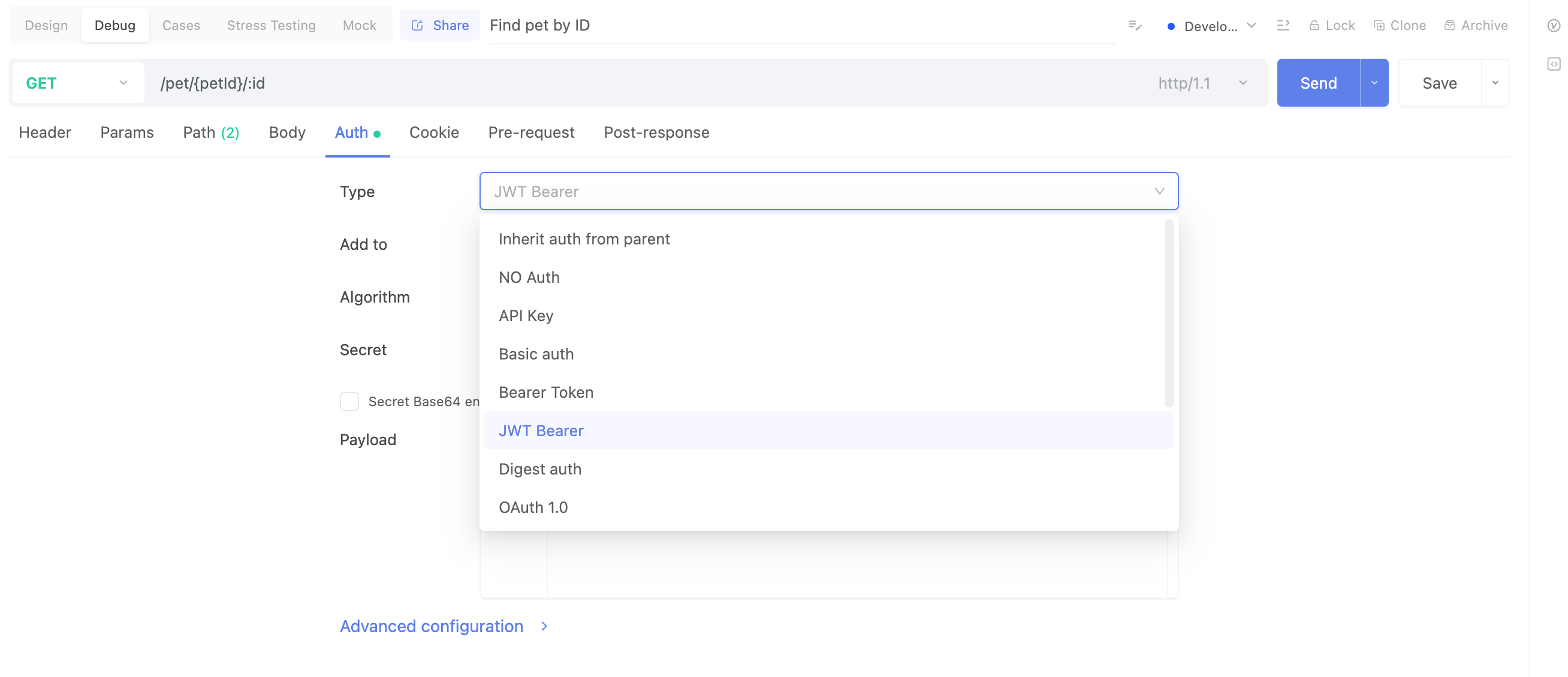
Cookie
Supports directly entering the request content of cookies.
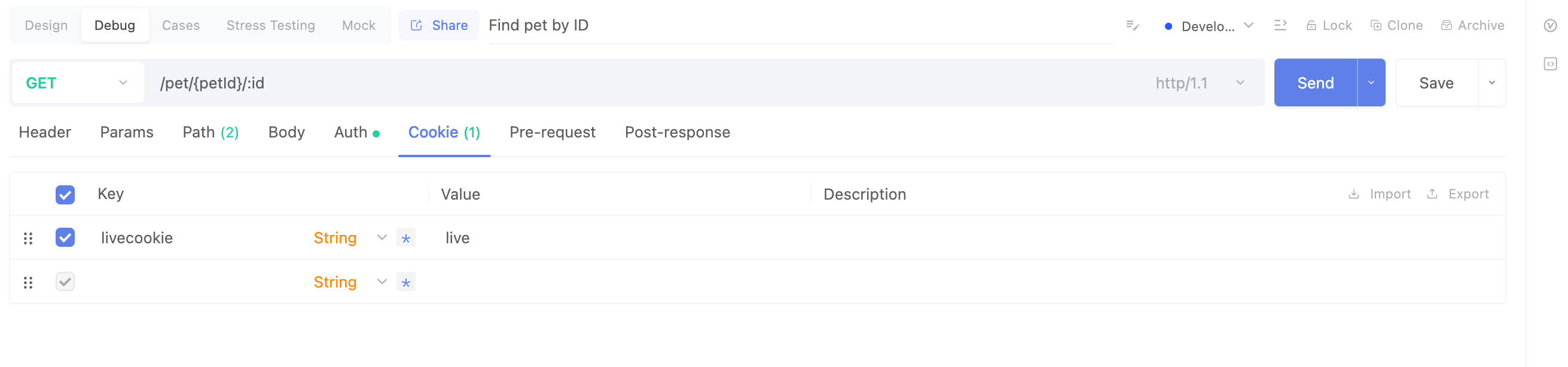
Pre-request/Post-response
Pre-request operations occur before the request is sent. These operations support custom scripts and database connections, often used for setting input parameters or printing data.
Post-response operations occur after the request is sent and are typically used for setting variables or making assertions. They support custom scripts, database connections, and variable extraction.
- Support drag sorting, name modification, and deletion
- The switch indicates whether to perform this task
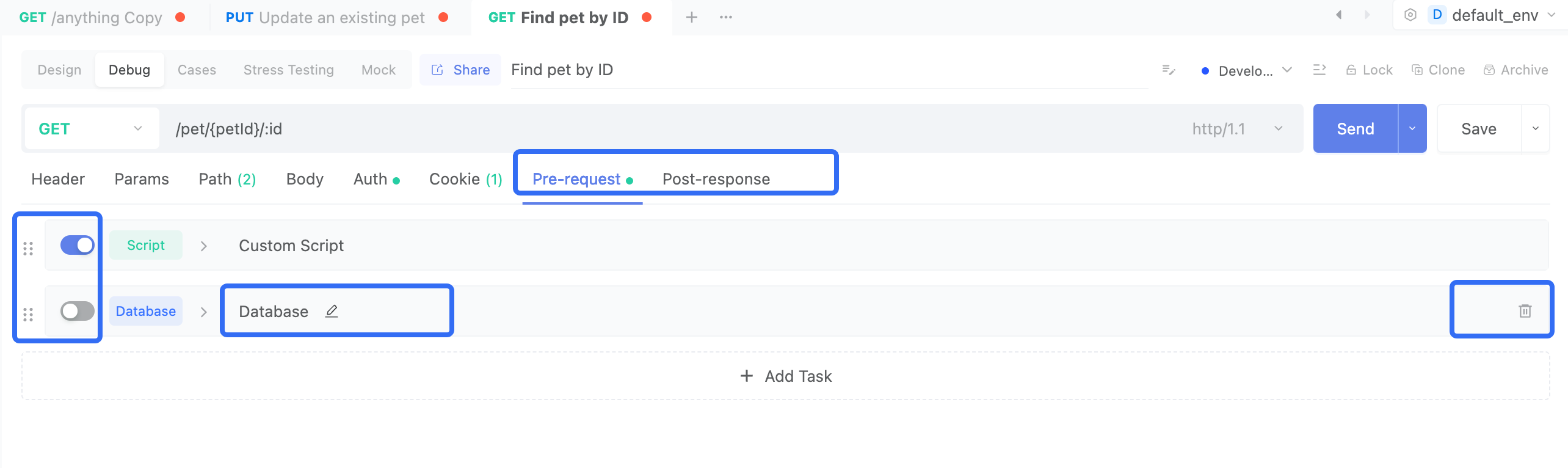
Response Area
Real-time Response
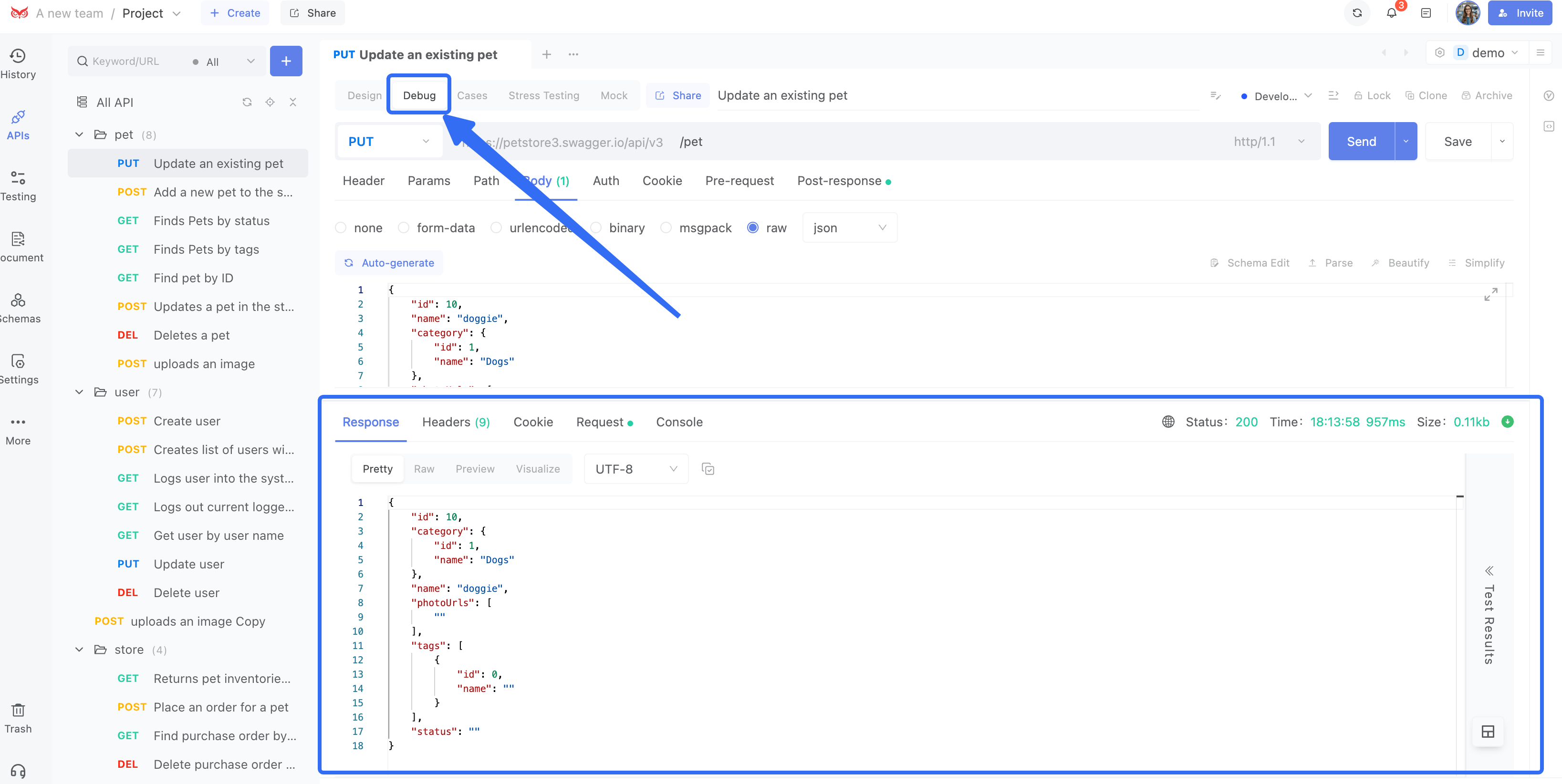
Response body & Test results
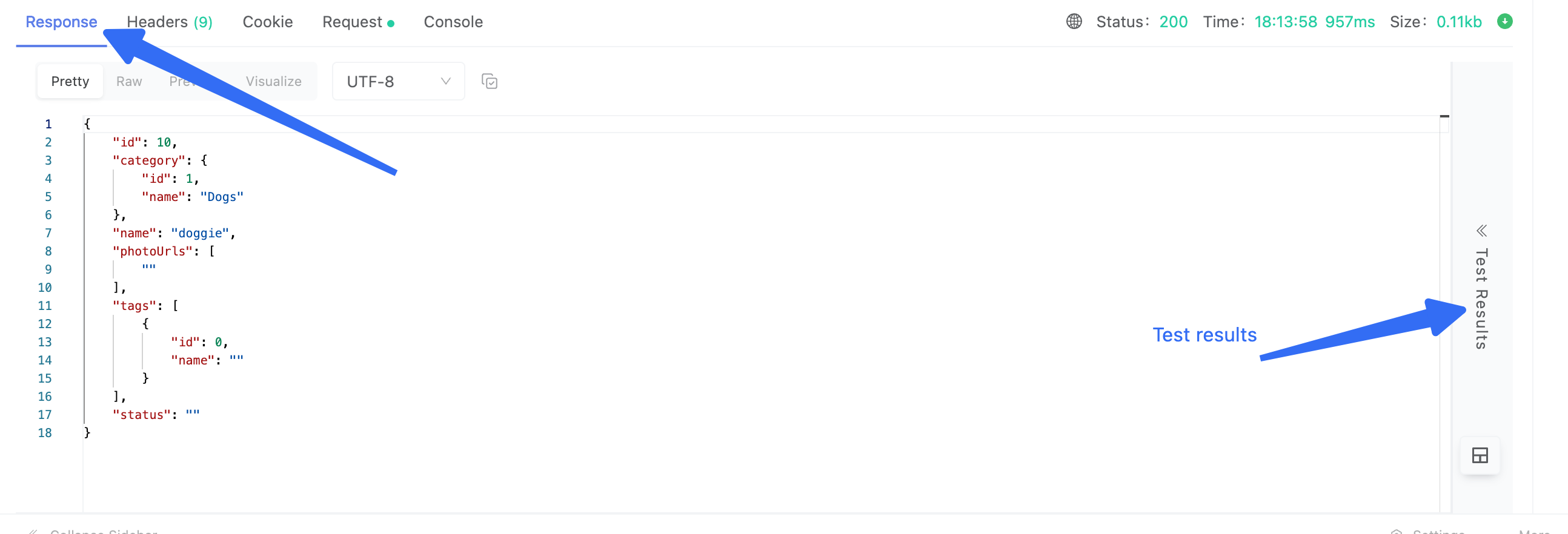
Response headers
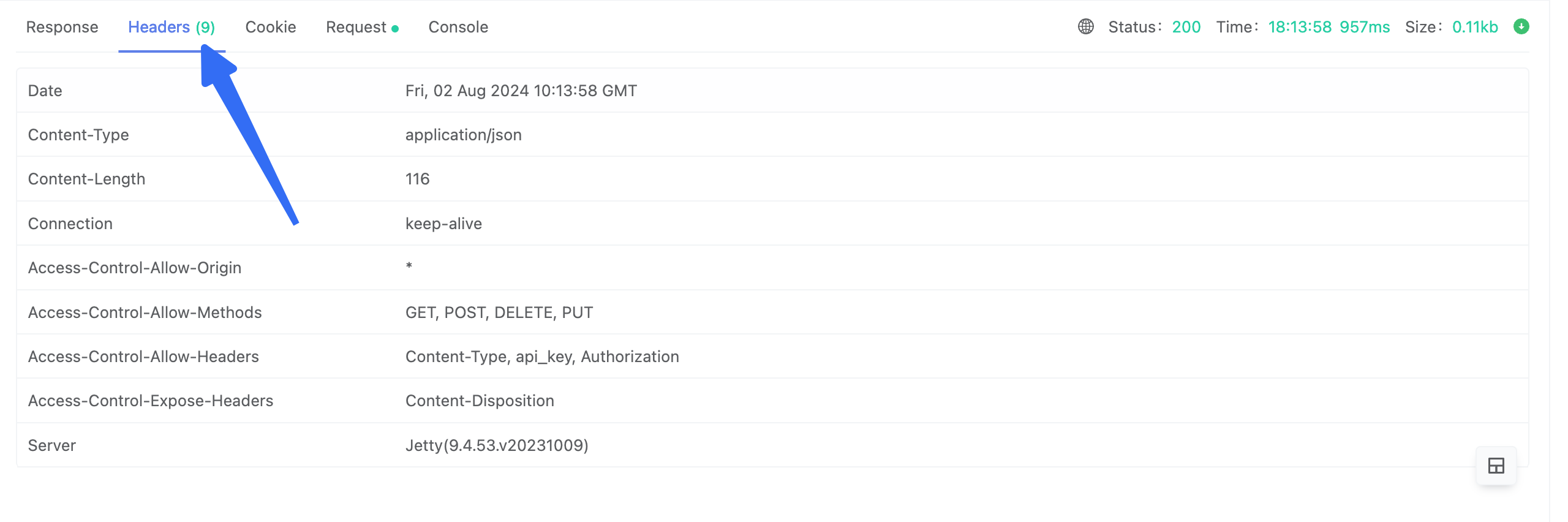
Response cookie
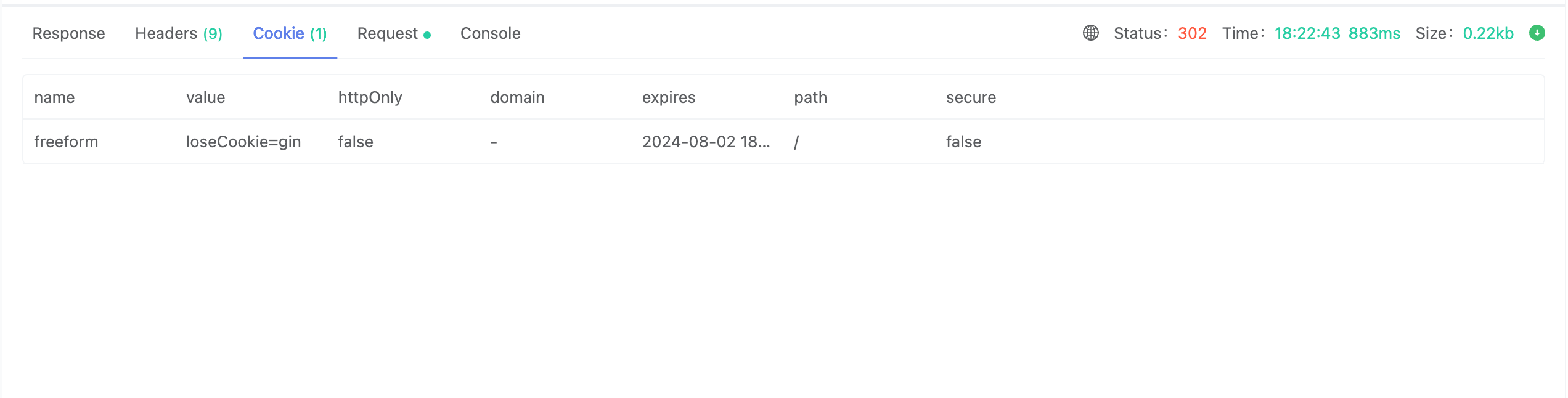
Actual Request
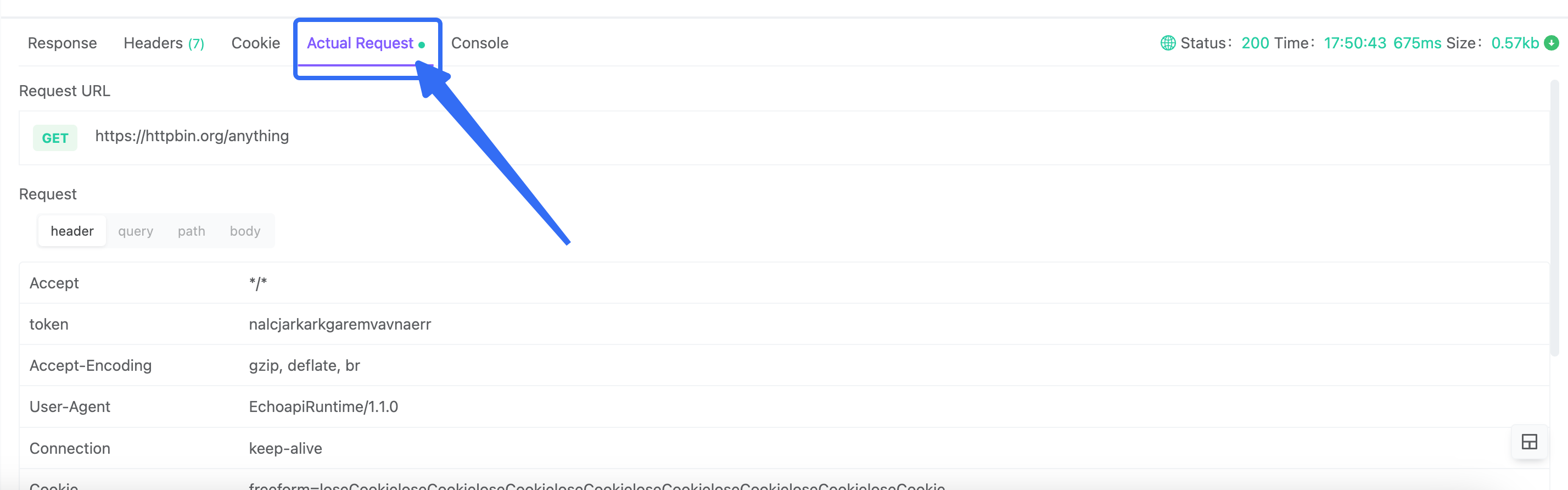
Console
The console can view the content printed by pre-request and post respons scripts.
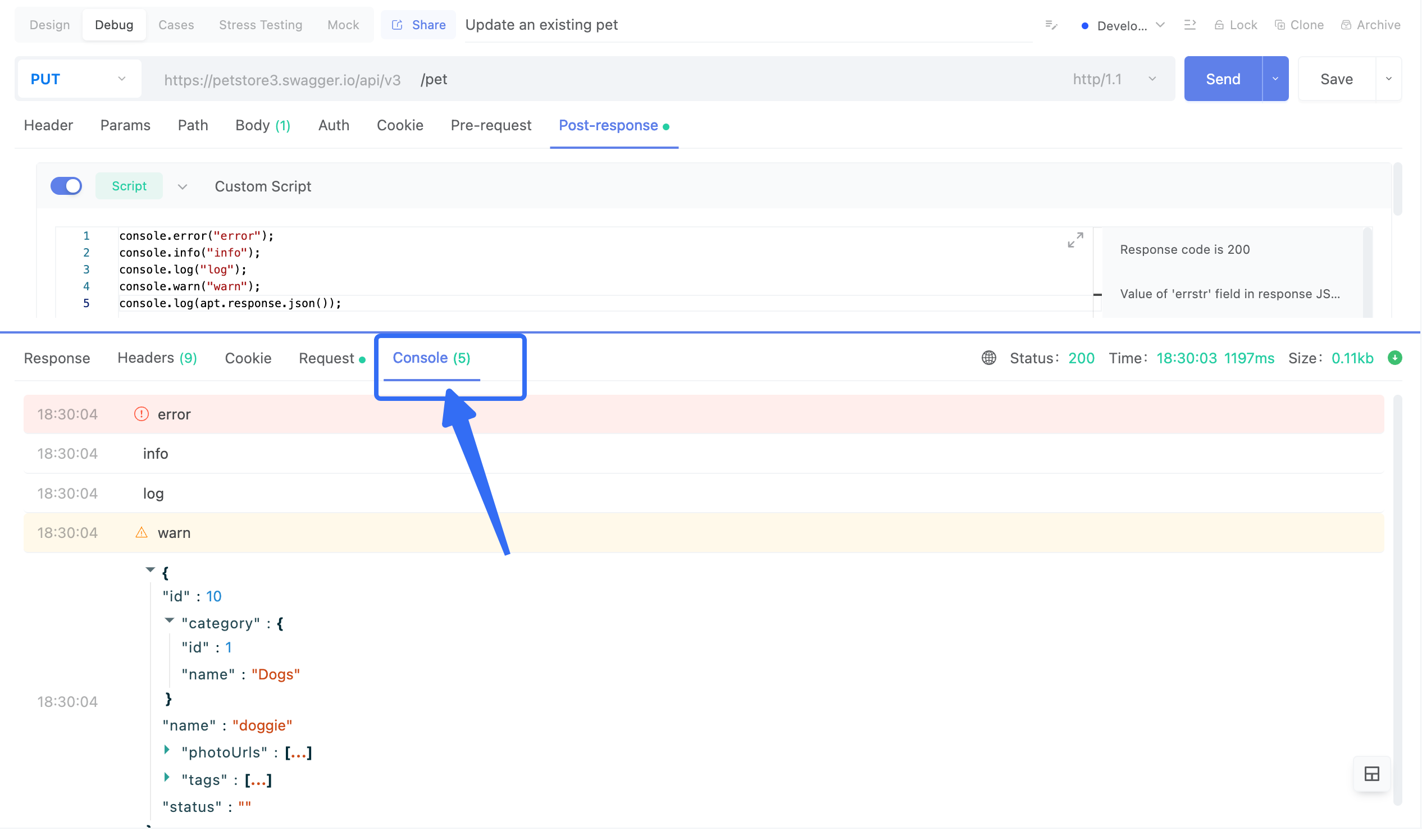
Proxy and Response Time
If you've configured a proxy in the system settings, you can verify its effectiveness in the response area. Additionally, you can refer to the documentation for detailed instructions on using a proxy.
Click on the response time to view the duration of each event:
tip
Request: Time from sending the request to initiating the socket operation.
Socket: Duration of the socket operation.
Lookup: Time taken for DNS query.
Connect: Time required to establish a connection to the server.
SecureConnect: Time to establish an SSL secure connection.
Response: Time to receive a response from the server.
End: Time taken to conclude the request.
Done: Total time for the entire request processing.
These time metrics help analyze network request performance, identifying which stages are taking the longest. If a particular stage is found to be slow, further optimization may be needed.
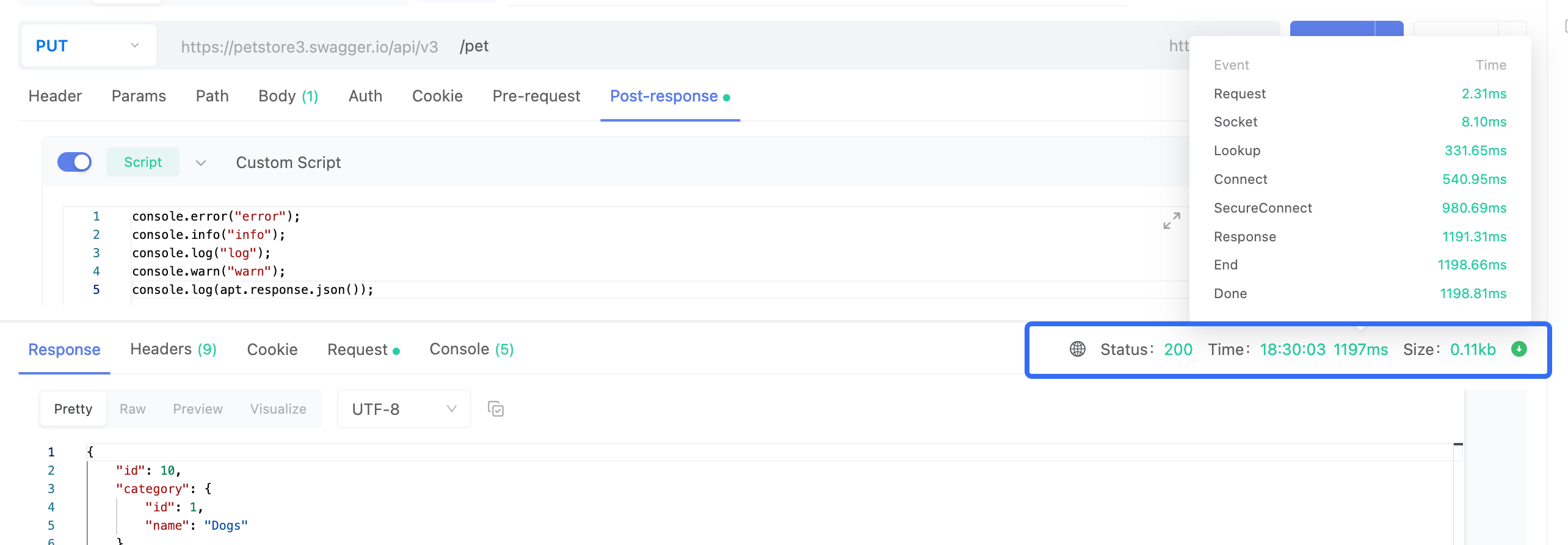
Test results
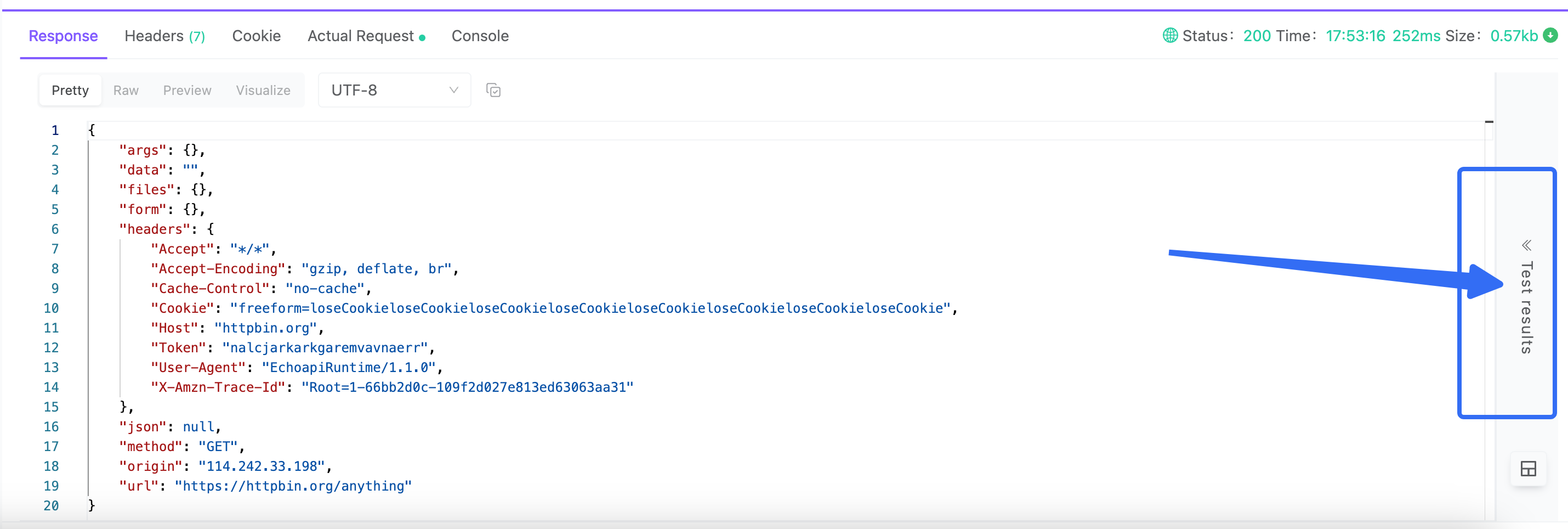
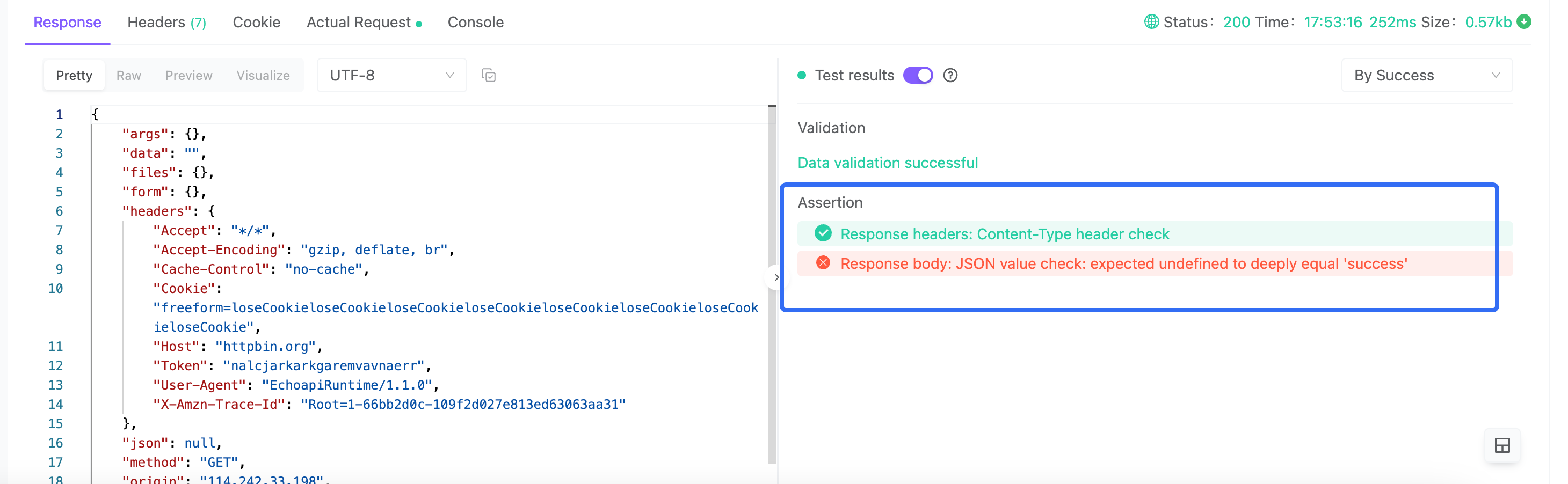
The Necessity of HTTP/2
The adoption of HTTP/2 provides tangible benefits in performance, security, and user experience. As websites and applications grow in complexity and user expectation, HTTP/2 addresses critical efficiency issues inherent in HTTP/1.x, making it not just desirable but necessary for modern web development.
Conclusion
HTTP/2 brings a new dimension to web communications which effectively tackles the challenges posed by modern web applications and user demands. Developers must harness the capabilities of HTTP/2 to ensure optimized performance and user satisfaction. By embracing HTTP/2, developers are equipped to meet the future of web development head-on, making their applications faster, more secure, and more efficient.