How to Integrate DeepSeek APIs into Your Apps within ten minutes
Integrating advanced capabilities like natural language processing, image recognition, or predictive analytics into apps is no longer optional—it’s essential.
In today’s AI-driven world, integrating advanced capabilities like natural language processing, image recognition, or predictive analytics into apps is no longer optional—it’s essential. DeepSeek APIs stand out by offering cutting-edge AI models that are fast, scalable, and easy to implement. Whether you’re building a smart chatbot, automating workflows, or analyzing large datasets, DeepSeek provides the tools to supercharge your app’s intelligence—without requiring a PhD in machine learning.
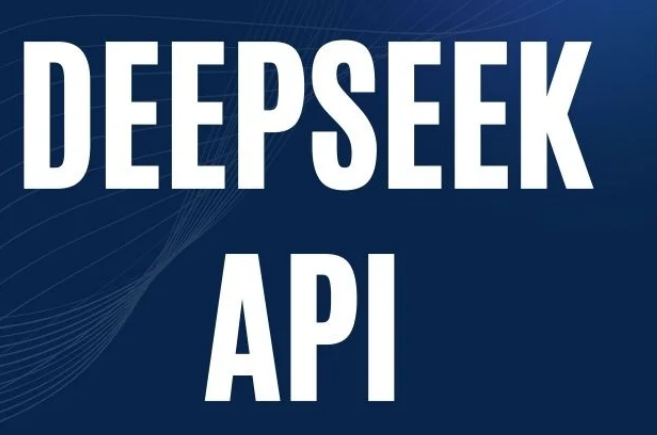
Before diving into code, let’s set up your toolkit:
- DeepSeek API Documentation: Your go-to guide for endpoints, parameters, and authentication.
- EchoAPI: A lightweight API testing tool (similar to Postman or Insomnia) to debug requests, simulate responses, and generate ready-to-use code snippets.
- Your Favorite IDE: Whether it’s VS Code, PyCharm, or JetBrains, keep it open!
Why EchoAPI?
While tools like Postman or Swagger are great, EchoAPI simplifies the process with:
- One-click code generation (Python, JavaScript, cURL, etc.).
- Real-time collaboration for team projects.
- Auto-saved history to track API call changes.
It’s perfect for developers who want to test, debug, and generate code in one place.
Step 1: Authenticate with DeepSeek
Most DeepSeek APIs require an API key for access. Here’s how to test authentication with EchoAPI:
- Sign up for a DeepSeek account and grab your API key from the dashboard.
- Test the connection with a simple
GET
request to DeepSeek’s/ping
endpoint (if available). A200 OK
response means you’re in!
In EchoAPI, create a new request and set the Authorization header to:
Authorization: Bearer YOUR_API_KEY
Step 2: Test Your First API Call
Let’s say you’re integrating DeepSeek’s text summarization API:
- In EchoAPI, set the endpoint to
POST https://api.deepseek.com/v1/summarize
. - Add your API key to the headers.
- Hit Send! If successful, you’ll get a summarized version in the response.
In the request body, include your input text and parameters:
{
"text": "The rise of renewable energy... [long text]",
"max_length": 150
}
Pro Tip: Use EchoAPI’s “Mock Server” feature to simulate edge cases (e.g., empty input, rate limits) before touching production code.
Step 3: Generate Code Snippets
Once your API call works in EchoAPI, let’s turn it into code:
- Click the </> Code button in EchoAPI.
- Copy-paste this into your app and customize error handling or retry logic.
Choose your language (Python, JavaScript, etc.). For example, a Python snippet using requests
:
import requests
url = "https://api.deepseek.com/v1/summarize"
headers = {
"Authorization": "Bearer YOUR_API_KEY",
"Content-Type": "application/json"
}
data = {
"text": "Your input text...",
"max_length": 150
}
response = requests.post(url, headers=headers, json=data)
print(response.json())
Step 4: Handle Errors Like a Pro
DeepSeek APIs might throw errors for invalid requests, rate limits, or server issues. Use EchoAPI to test these scenarios:
- 429 Too Many Requests: Add retry logic with exponential backoff.
- 400 Bad Request: Validate inputs before sending (e.g., text length).
- 502 Bad Gateway: Implement fallback mechanisms for critical workflows.
Final Touches: Optimize and Scale
- Cache frequent requests: Reduce costs and latency by storing common responses.
- Monitor usage: Track API calls with tools like Prometheus or Datadog.
- Stay updated: Follow DeepSeek’s changelog for new features or deprecated endpoints.
Conclusion
Integrating DeepSeek APIs doesn’t have to be a headache. With tools like EchoAPI, you can prototype faster, debug smarter, and ship features confidently. Whether you’re a solo developer or part of a team, this workflow ensures your app leverages AI without sacrificing speed or reliability.
Ready to start? Grab your DeepSeek API key, fire up EchoAPI, and turn your app into an AI powerhouse today!
Resources: