How to Send HTTP/2 Request?
Explaining how to send HTTP/2 requests using code (Java, Node, etc.) and tools like cURL and EchoAPI.
What is HTTP/2? π€
HTTP/2 π‘ is the latest version of the Hypertext Transfer Protocol, designed to improve performance and speed of web browsing. Unlike HTTP/1.1, HTTP/2 uses multiplexing, header compression, and prioritization to enhance efficiency and reduce latency. This leads to a better and faster user experience on websites.
How to Send HTTP/2 Request with Code π»
Python π
Ensure you have httpx
installed. You can install it using pip install httpx
.
import httpx
response = httpx.get("https://example.com")
print(response.text)
Java β
The following code requires JDK 11 or higher and uses the official HTTP Client library.
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.net.URI;
public class Http2Example {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newBuilder().version(HttpClient.Version.HTTP_2).build();
HttpRequest request = HttpRequest.newBuilder().uri(URI.create("https://example.com")).build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
}
PHP π
Ensure the cURL
extension is enabled and supports HTTP/2:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_2_0);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Node.js π
Ensure you are using Node.js version 10.10.0 or higher and have the http2
module:
const http2 = require('http2');
const client = http2.connect('https://example.com');
const req = client.request({ ':path': '/' });
req.setEncoding('utf8');
req.on('data', (chunk) => { console.log(chunk); });
req.on('end', () => { client.close(); });
req.end();
Golang πΉ
Ensure you are using Go version 1.6 or higher and have the golang.org/x/net/http2
package:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"golang.org/x/net/http2"
)
func main() {
client := &http.Client{}
http2.ConfigureTransport(client.Transport.(*http.Transport))
resp, err := client.Get("https://example.com")
if err != nil {
fmt.Println("Error:", err)
return
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading body:", err)
return
}
fmt.Println(string(body))
}
How to Send HTTP/2 Request with Tools π οΈ
cURL π
Ensure you are using a version of cURL that supports HTTP/2:
curl -I --http2 https://example.com
EchoAPI π¦
EchoAPI now supports HTTP/2, enhancing your API lifecycle management with faster and more efficient communication. This upgrade reduces latency and boosts overall performance for designing, testing, and sharing APIs.
If you're interested in taking advantage of HTTP/2 in EchoAPI, here's how to get started:
Step β Open EchoAPI and create a new request.
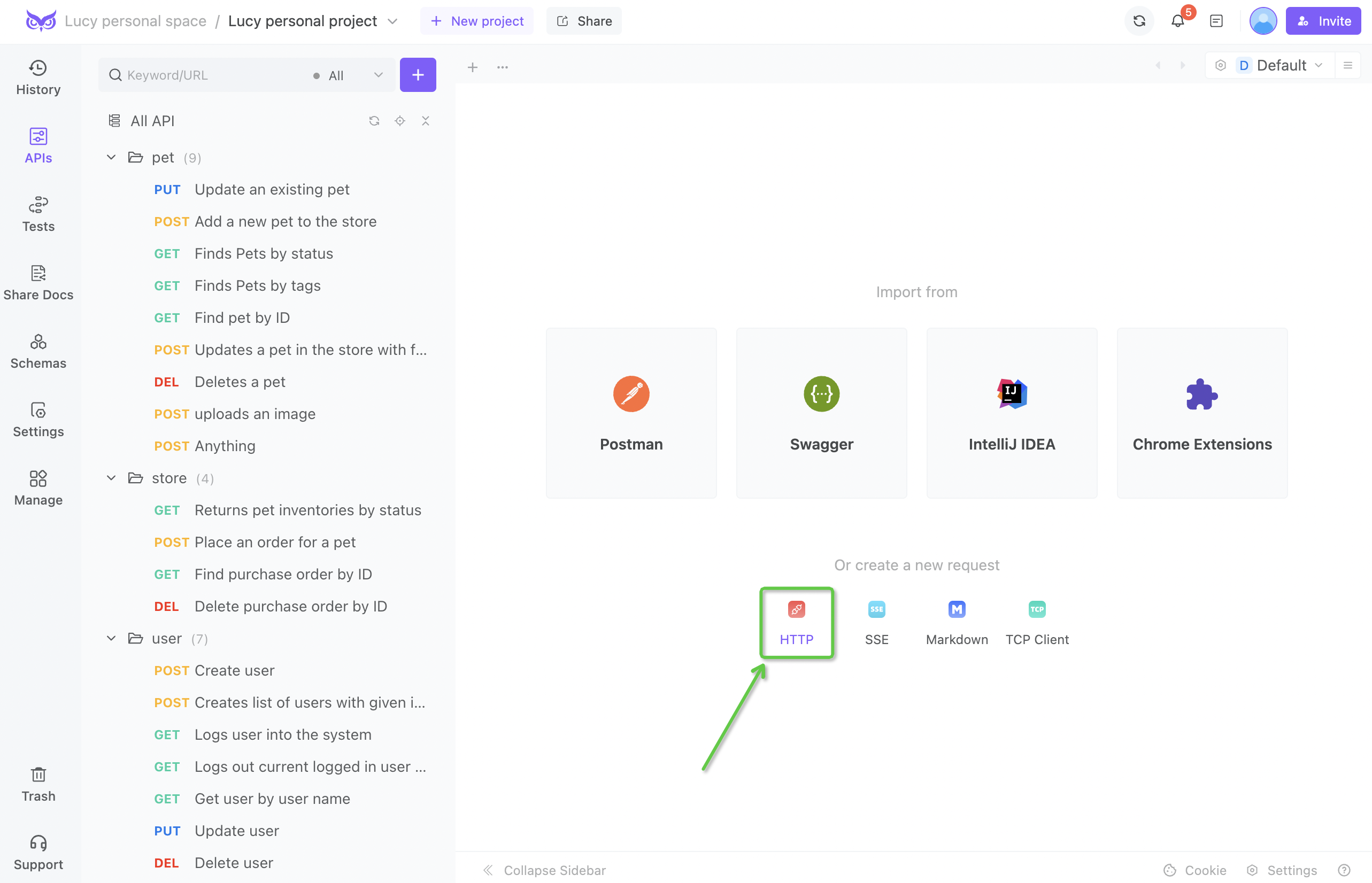
Step 2. Select the HTTP/2 protocol and click "Send" button.
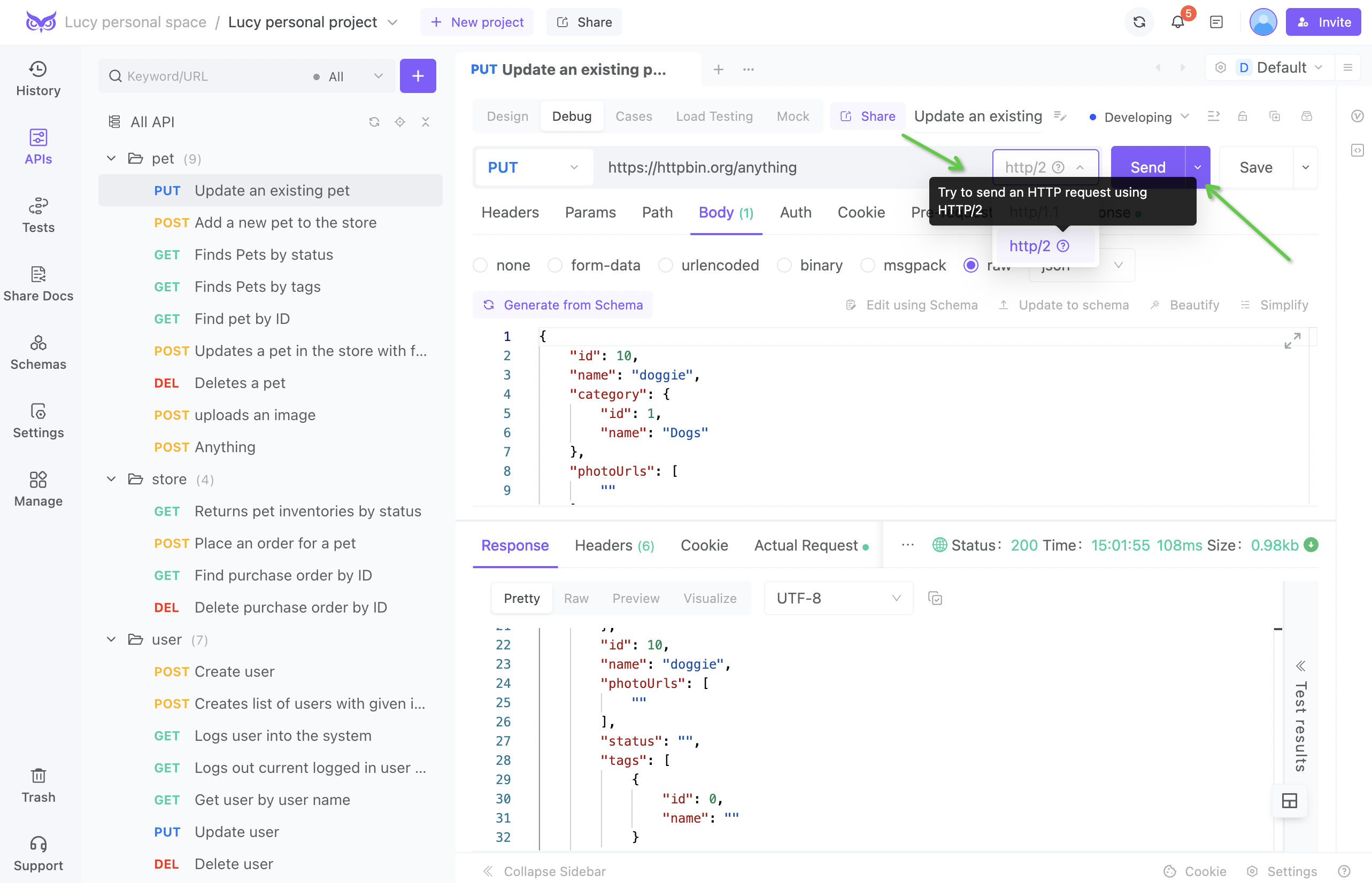
Conclusion π
Sending HTTP/2 requests across different languages has become straightforward with library support and native implementations. Each language has its pros and cons, but with these examples, you can get started quickly. Letβs embrace the faster, more efficient web with HTTP/2! π