Mastering Postman: Data-Driven Testing with CSV and JSON Files
While running bulk test cases is an excellent feature, what happens when you need to cover both positive and negative scenarios in your testing? How do you efficiently handle such cases without manually changing the input values for every test?
In the previous article, we walked through how to run test cases in bulk using Postman. If you missed it, you can check it out here.
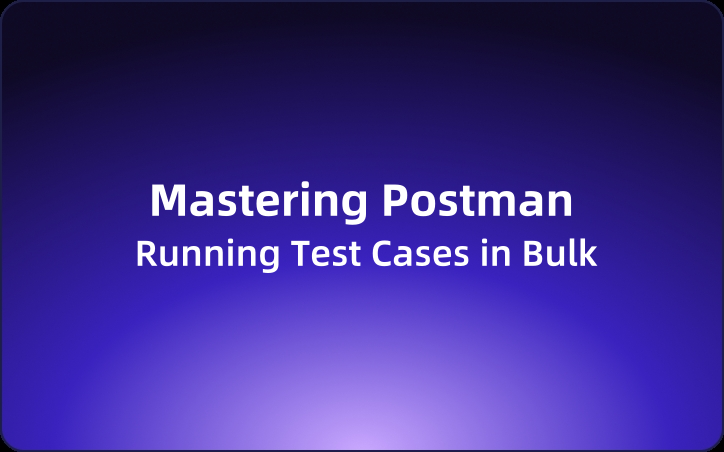
While running bulk test cases is an excellent feature, what happens when you need to cover both positive and negative scenarios in your testing? How do you efficiently handle such cases without manually changing the input values for every test?
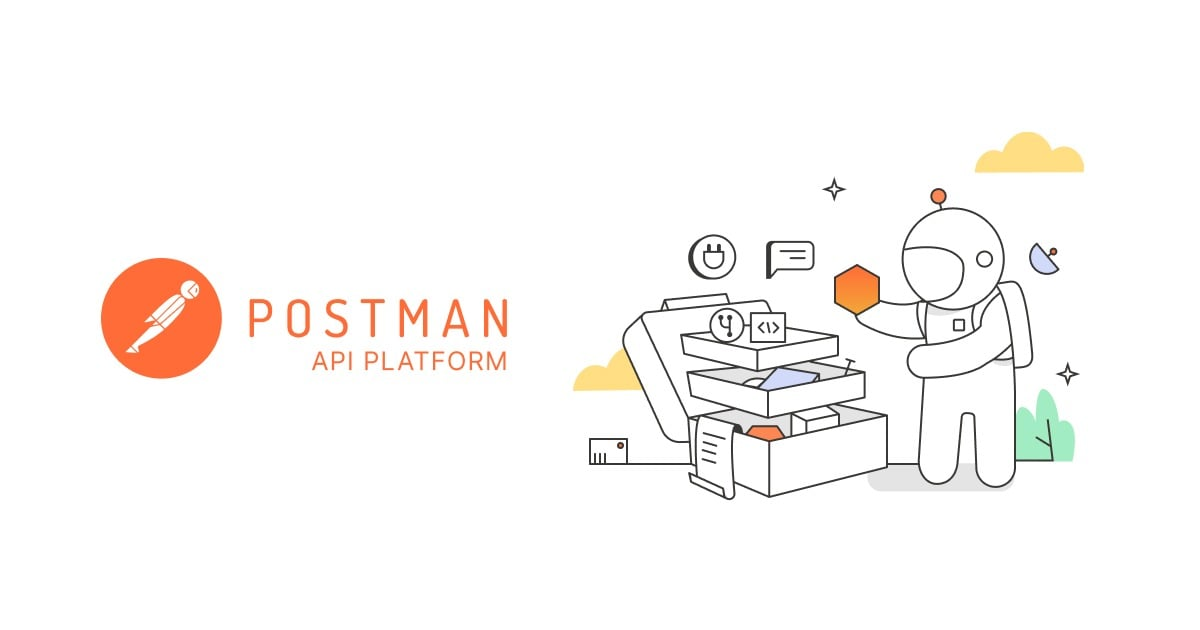
The answer lies in data-driven testing using CSV or JSON files, a powerful approach Postman offers to automate test cases with dynamic input sets. This article will guide you through implementing data-driven testing to cover all scenarios without breaking a sweat.
Practical Example Scenario
For this tutorial, let’s consider a Create a New User API endpoint. Here’s how the API responds based on the input:
- Case 1: When
username=echoapi
, the API successfully creates a user and returns:
{
"error": 0,
"msg": "ok",
"user": {
"id": 1787,
"username": "echoapi",
"firstName": "Echo",
"lastName": "Api",
"email": "support@echoapi.com",
"password": "12345",
"phone": "",
"userStatus": 0
}
}
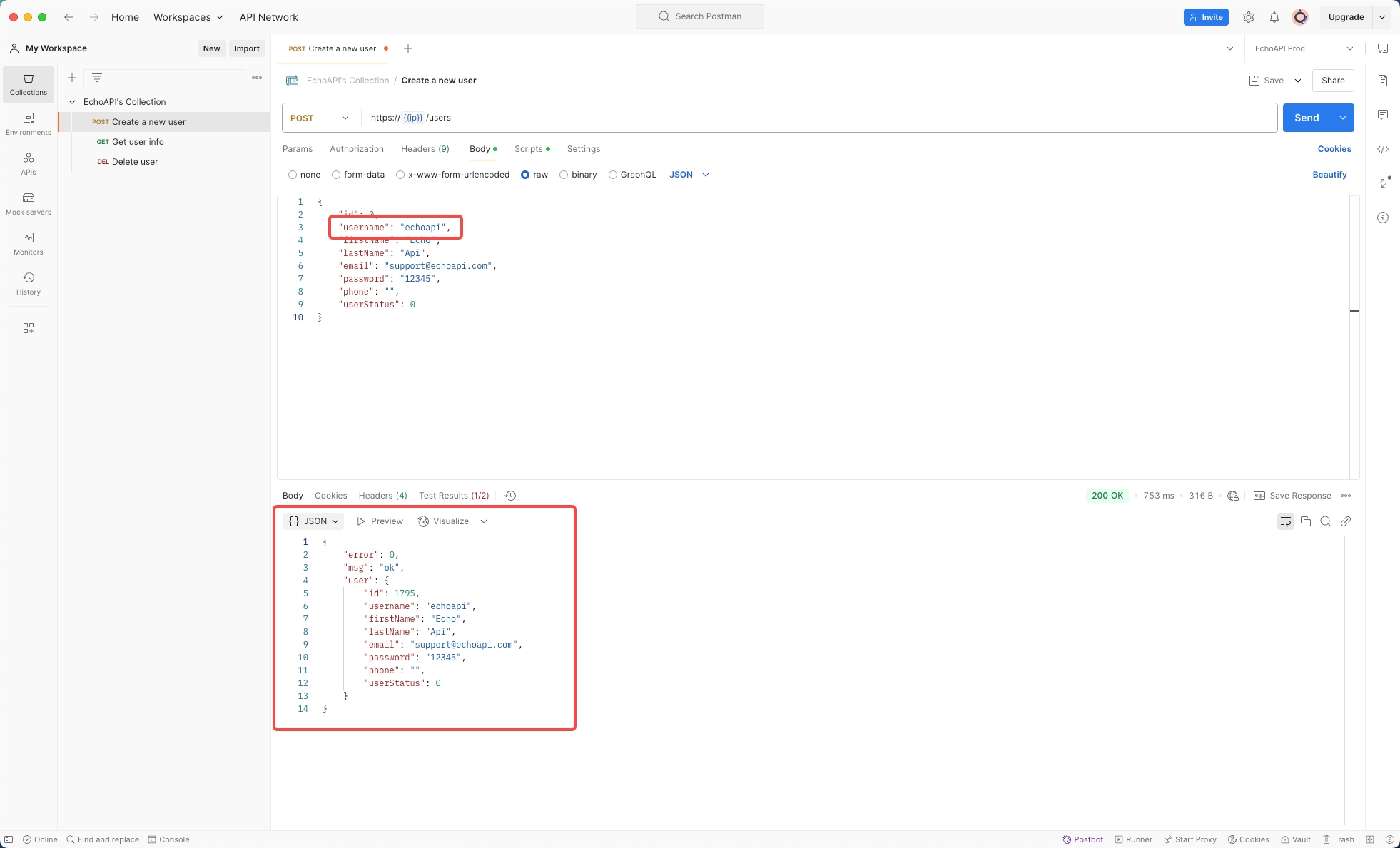
- Case 2: When
username
is empty, the API fails and returns:
{
"error": 1,
"msg": "Username is required"
}
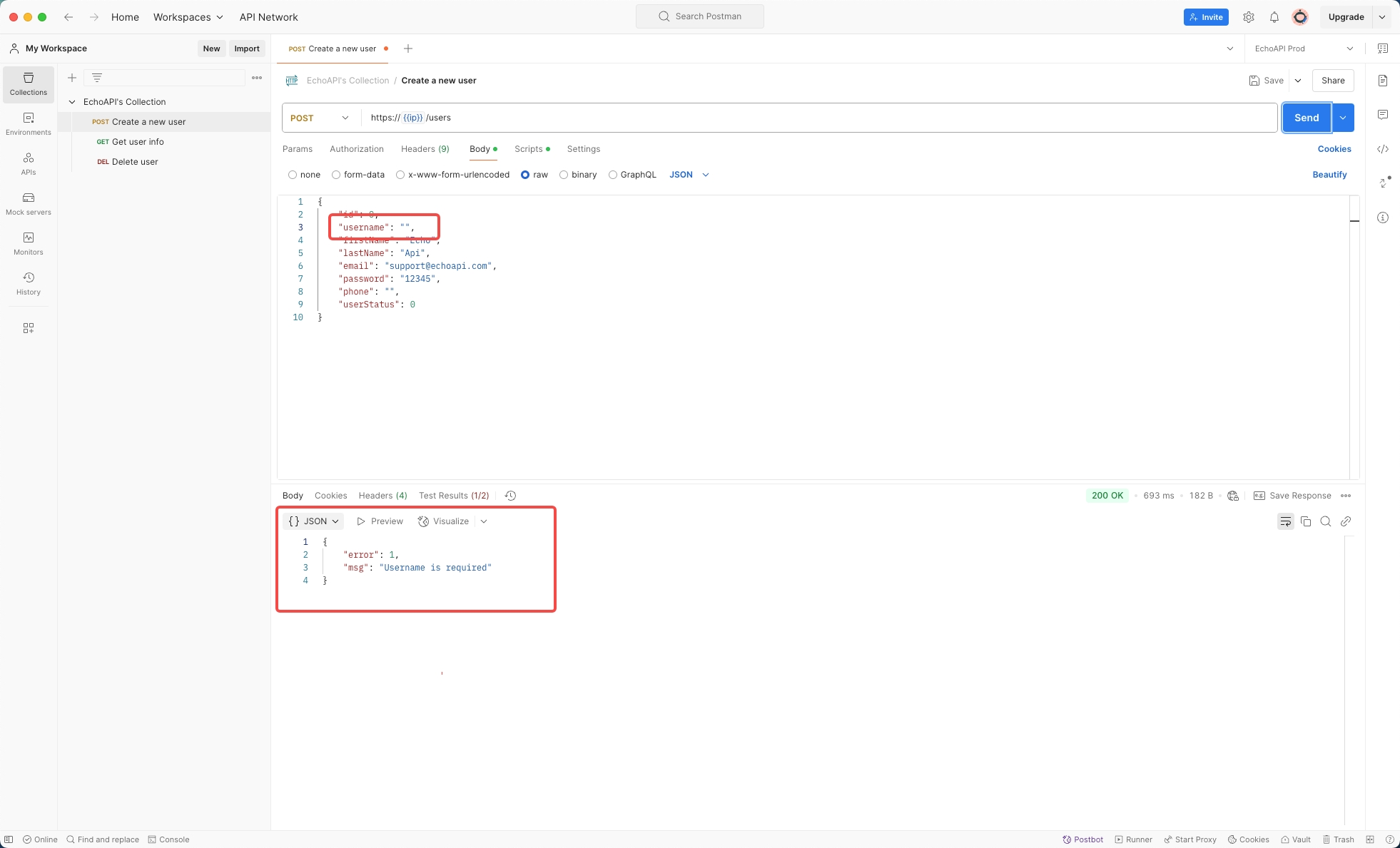
We’ll now demonstrate how to cover these scenarios with a CSV or JSON file to automate testing for these responses.
Step-By-Step Guide to Data-Driven Testing
Step 1: Create the Data File (CSV)
- On your local machine, create a new text document and name it
data.csv
. - Open the file in a text editor or any CSV editor.
Write your test data starting from the second row:
echoapi,0
,1
The first scenario tests for a valid username
, and the second scenario tests for an empty username
. Save the file.
Write the first row as the parameter names:
username,assert_value
Here, username
represents the input field, and assert_value
will store the expected value of the error
field for assertion.
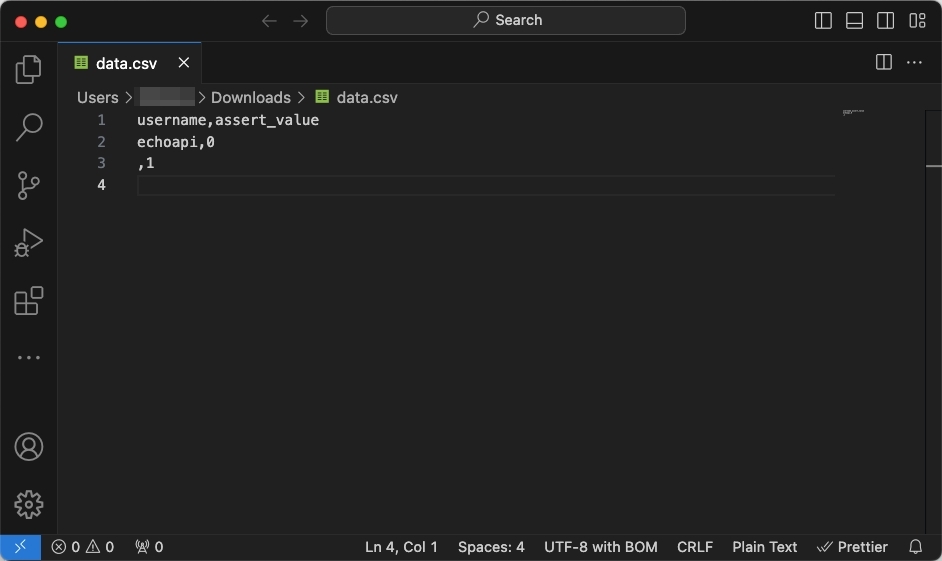
Step 2: Update the Request Body
In Postman’s request body, replace the username
static value with a dynamic variable using the double curly braces {{...}}
syntax:
{
"id": 0,
"username": "{{username}}",
"firstName": "Echo",
"lastName": "Api",
"email": "support@echoapi.com",
"password": "12345",
"phone": "",
"userStatus": 0
}
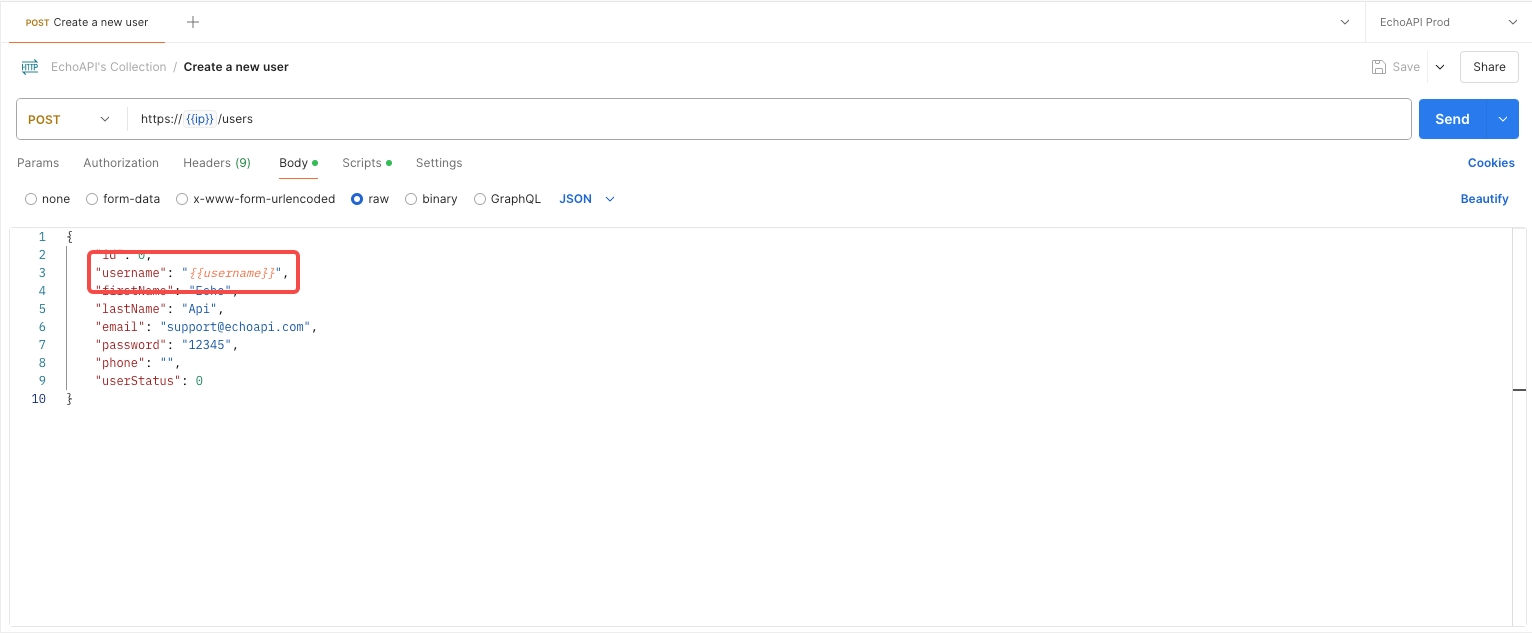
Postman will replace {{username}}
dynamically with the values from the username
column in your CSV file during runtime.
Step 3: Write the Assertions in the Test Script
The next step is scripting assertions in the Tests tab of the request. For this example, we need to:
- Validate the status code.
- Assert the
error
response value against the expected value provided in theassert_value
field of the CSV file.
Here’s the full script:
// Check if "username" exists in the response
if (responseBody.search("username") != -1) {
var result = responseBody.match(new RegExp('"username":"(.*?)"'));
console.log("Extracted username: " + result[1]);
// Set the username as a global variable
pm.globals.set("username", result[1]);
}
// Assertion: Status code is 200
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Assertion: Body contains the expected "error" value
pm.test("Body matches error", function () {
pm.expect(pm.response.text()).to.include(data.assert_value);
});
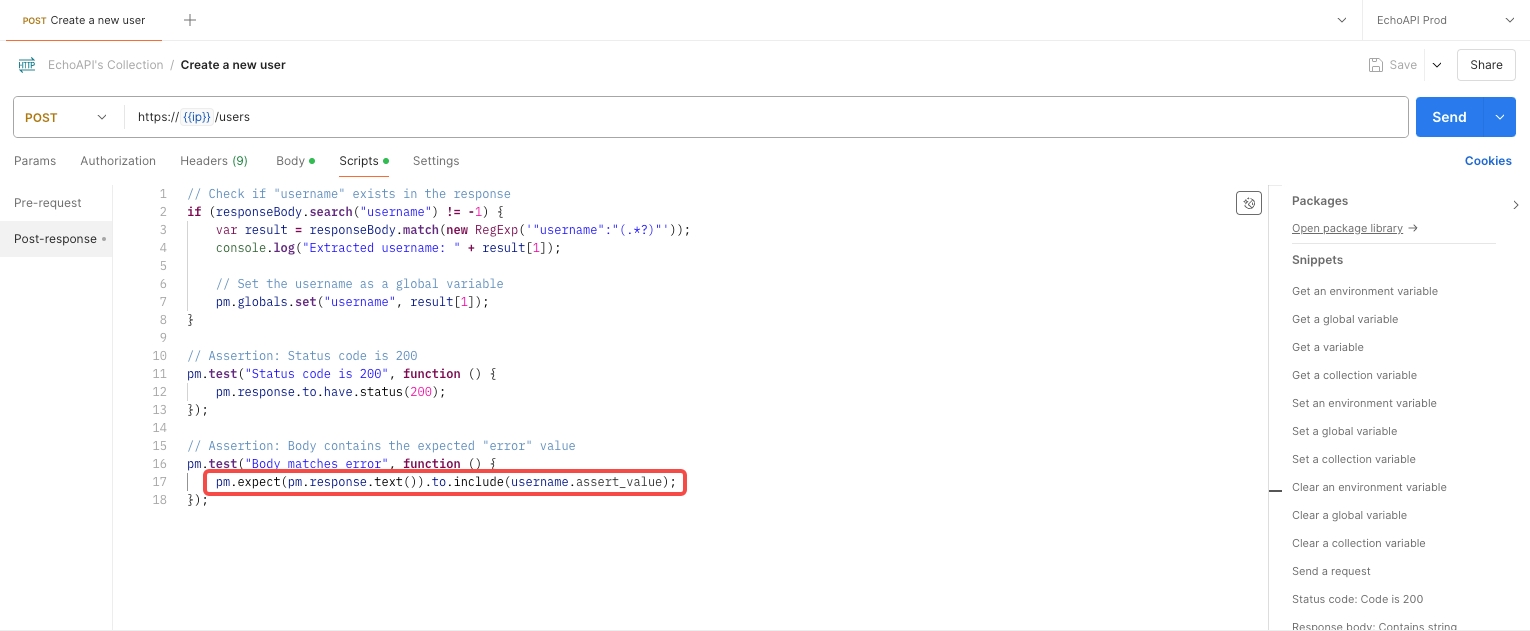
This ensures that the script works dynamically with data inputs while handling edge cases where username
may not be present in the payload.
Step 4: Add the Data File to the Collection Run
1. Click the Run collection button in Postman.
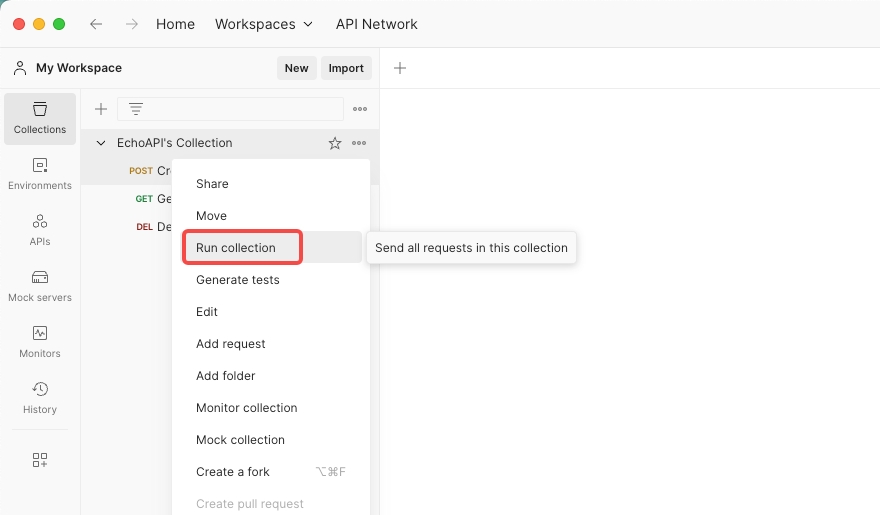
2. In the Data File section, upload your username.csv
file.
- You’ll notice that the Iterations field is automatically updated with the number of rows (test cases) in your CSV.
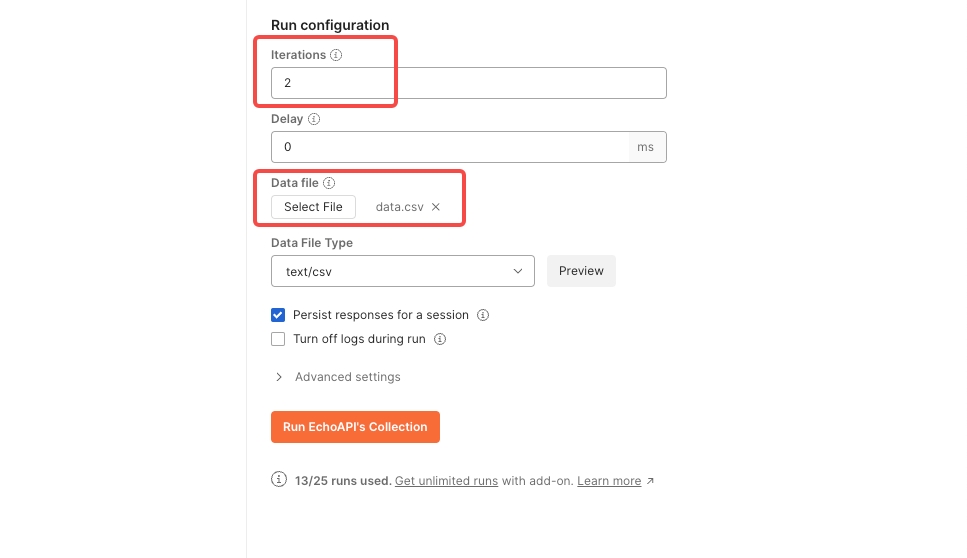
3. Click Preview to verify the test data loaded from the file.
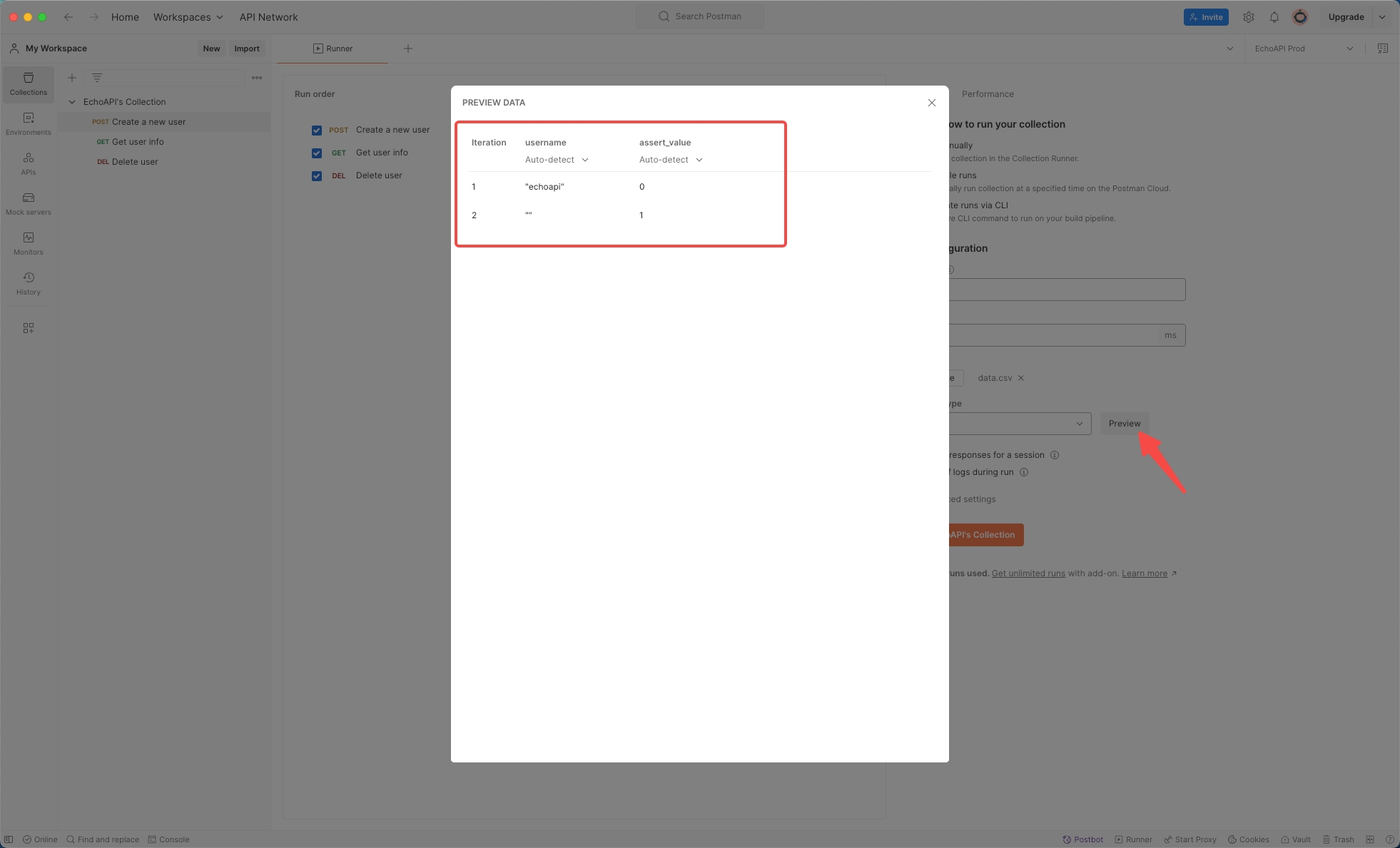
4. Choose the API to be executed and click Run EchoAPI's Collection.
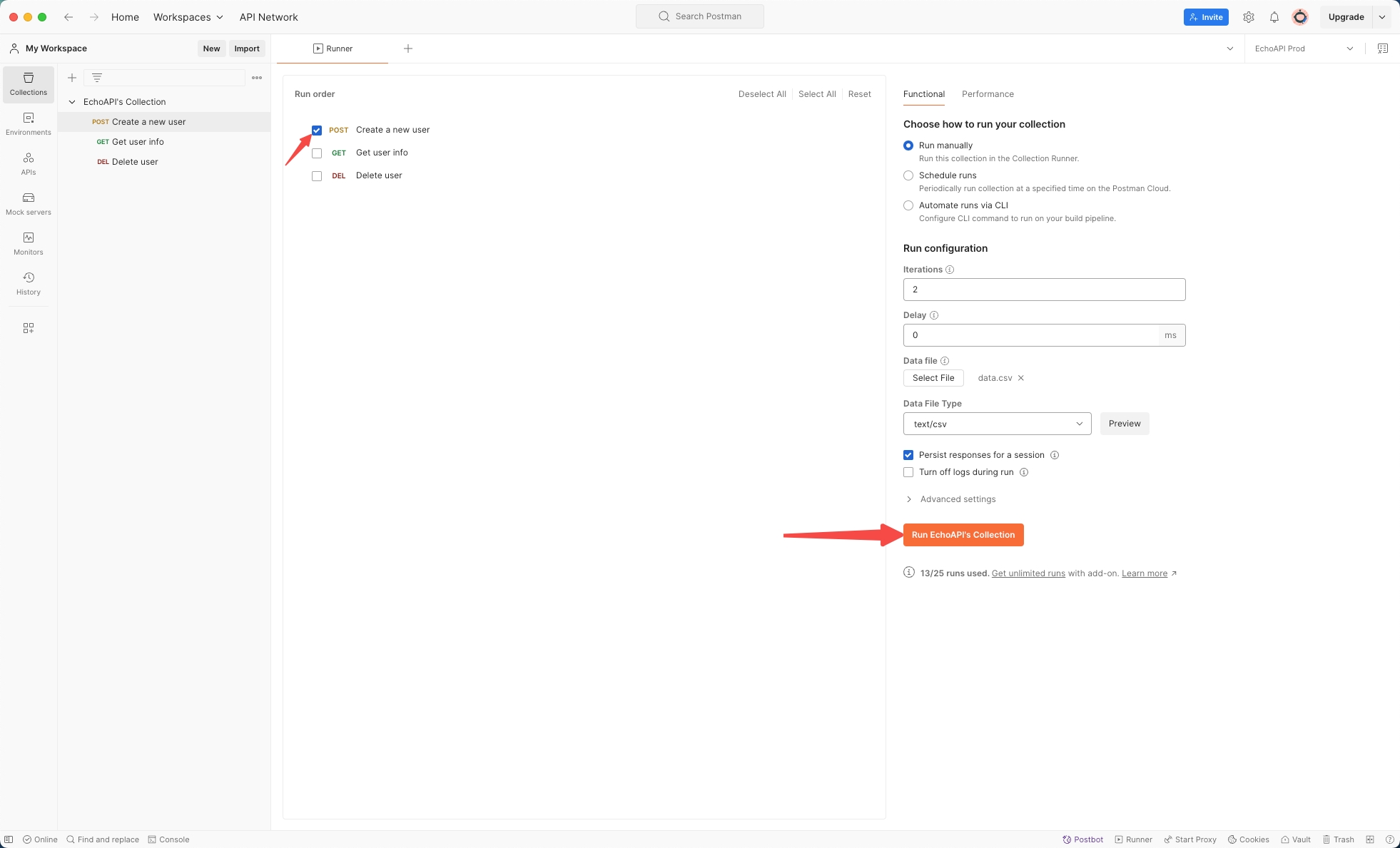
Step 5: Analyze the Results
After the collection run is complete, you can view the detailed report. It will display:
- The total number of iterations (corresponding to the rows in your data file).
- Passed, failed, and skipped test cases.
- Execution time, request, and response logs.
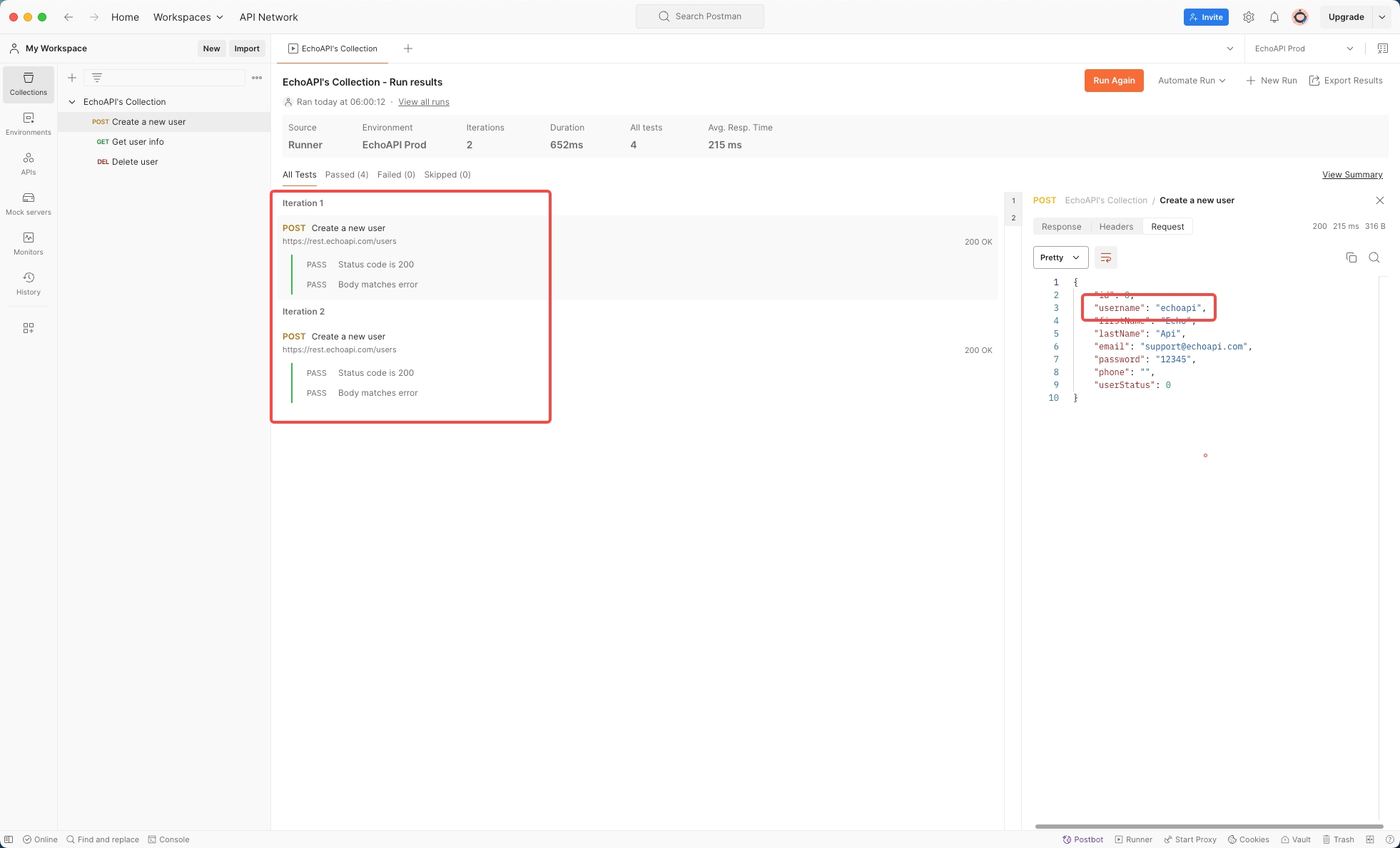
Postman will dynamically substitute the {{username}}
and {{assert_value}}
variables with their respective values from the CSV file and perform assertions for each iteration.
JSON File (Alternative Data Format)
The process for using a JSON file is identical to the CSV file method. The only difference lies in the data format. Here's how you can prepare the same test data as a JSON file:
[
{
"username": "echoapi",
"assert_value": 0
},
{
"username": "",
"assert_value": 1
}
]
When running the collection, select the JSON file instead of the CSV file, and Postman will handle the rest in the same way.
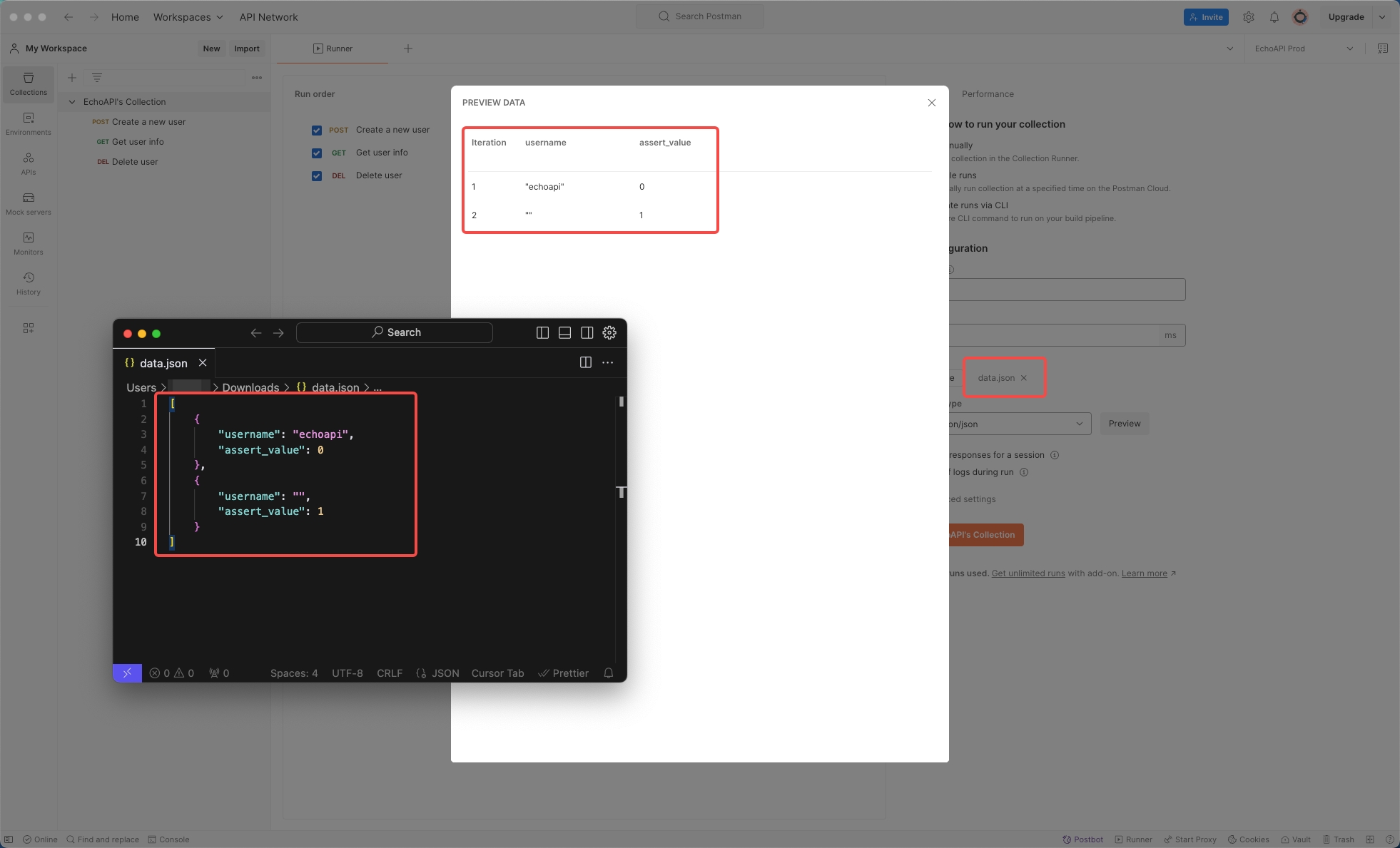
Conclusion
Data-driven testing with Postman is an incredibly efficient way to cover multiple scenarios and datasets in your API testing. By leveraging CSV or JSON files, you can dynamically feed data into your tests, enabling you to automate both positive and negative test cases with ease.
This approach not only saves time but also improves accuracy by avoiding hard-coded values in test scripts. Whether you’re testing edge cases or scaling up tests with hundreds of user inputs, data-driven testing ensures your APIs are robust and reliable for all scenarios.
Master this technique, and you’ll be equipped to handle any API testing challenge efficiently!
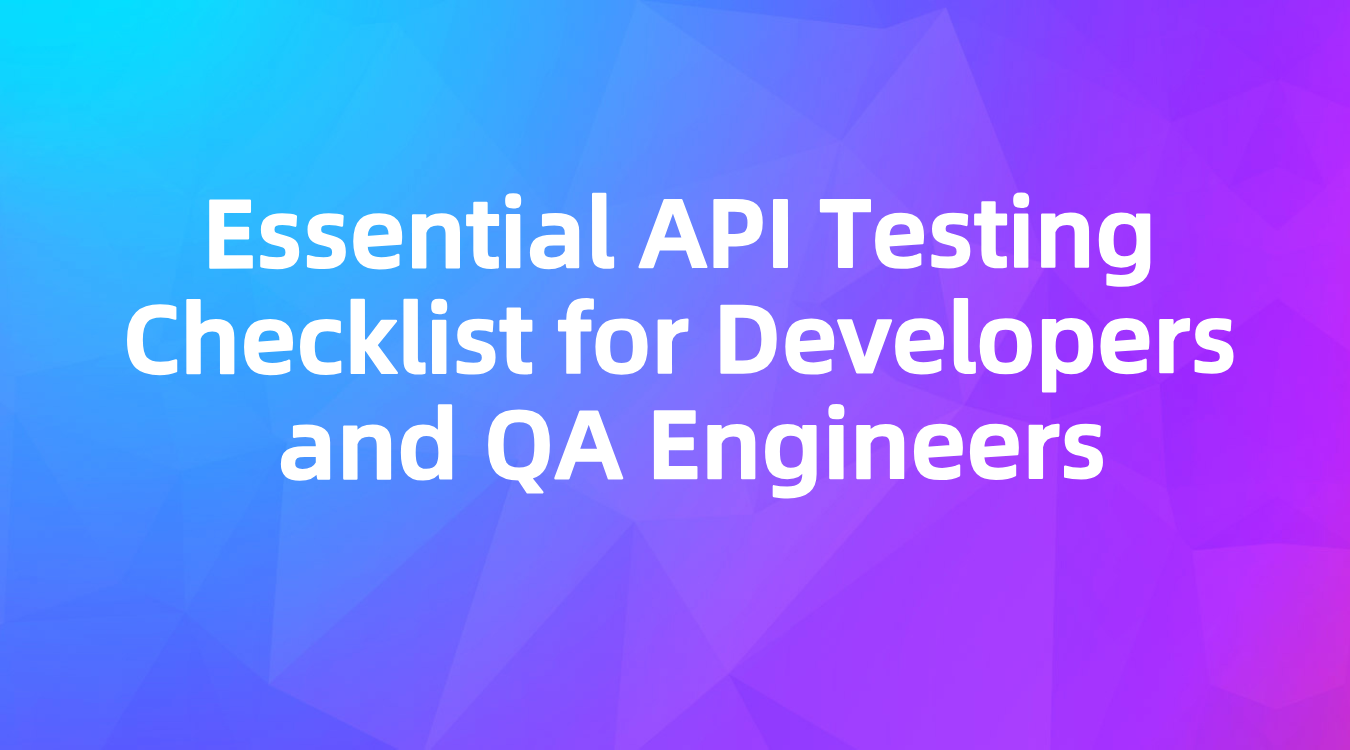