Mastering Postman: Implementing API Chaining with the JSON Extractor
In this article, we will take a hands-on approach to explore how to implement API chaining using the JSON extractor, a crucial technique in Postman that streamlines testing processes.
Postman is a staple tool for API debugging and testing, making it an essential skill for any developer to master. In this article, we will take a hands-on approach to explore how to implement API chaining using the JSON extractor, a crucial technique in Postman that streamlines testing processes.
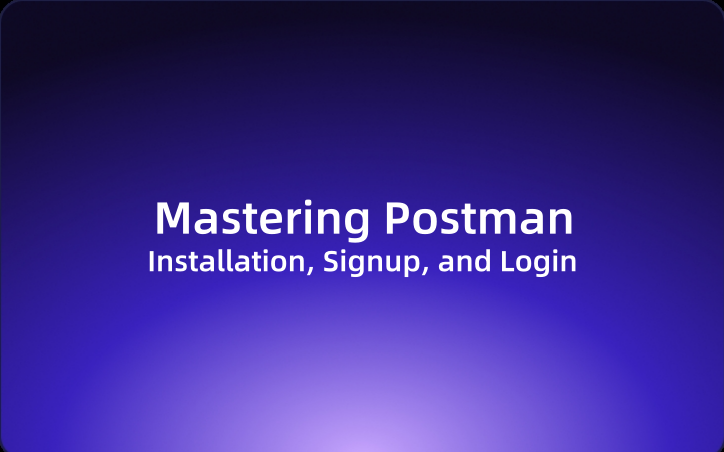
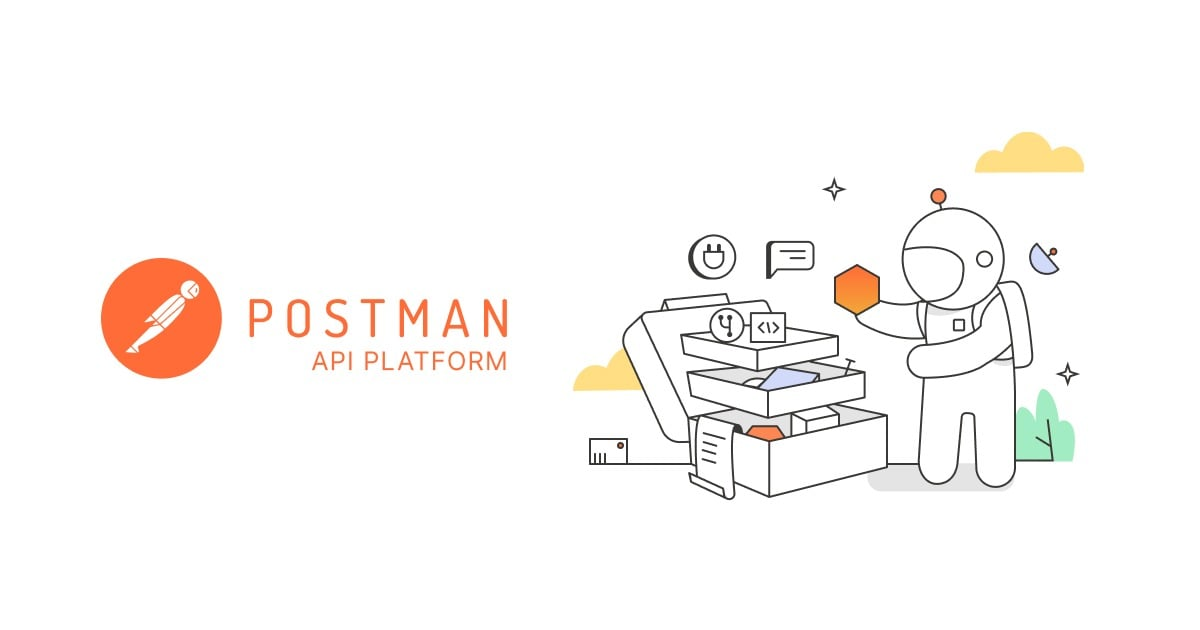
When testing APIs, developers often encounter the challenge of linking or "chaining" requests. Manually handling these links between requests can be cumbersome and error-prone. For instance, consider a scenario where you need to extract the username
from the response of a "create a new user" API and automatically insert it into subsequent "get user info" requests. How can this be achieved efficiently?
There are two primary methods to accomplish this task:
- Using the JSON extractor for API chaining.
- Using a regular expression extractor for API chaining.
In this article, we'll focus on how to implement API chaining using a JSON extractor.
Setting Up JSON Extractor for API Chaining
Step 1: Access the "Create a New User" API's Tests Section
Begin by navigating to the Scripts Post-response tab of the "create a new user" API. This area allows you to script the actions that occur after a request is made.
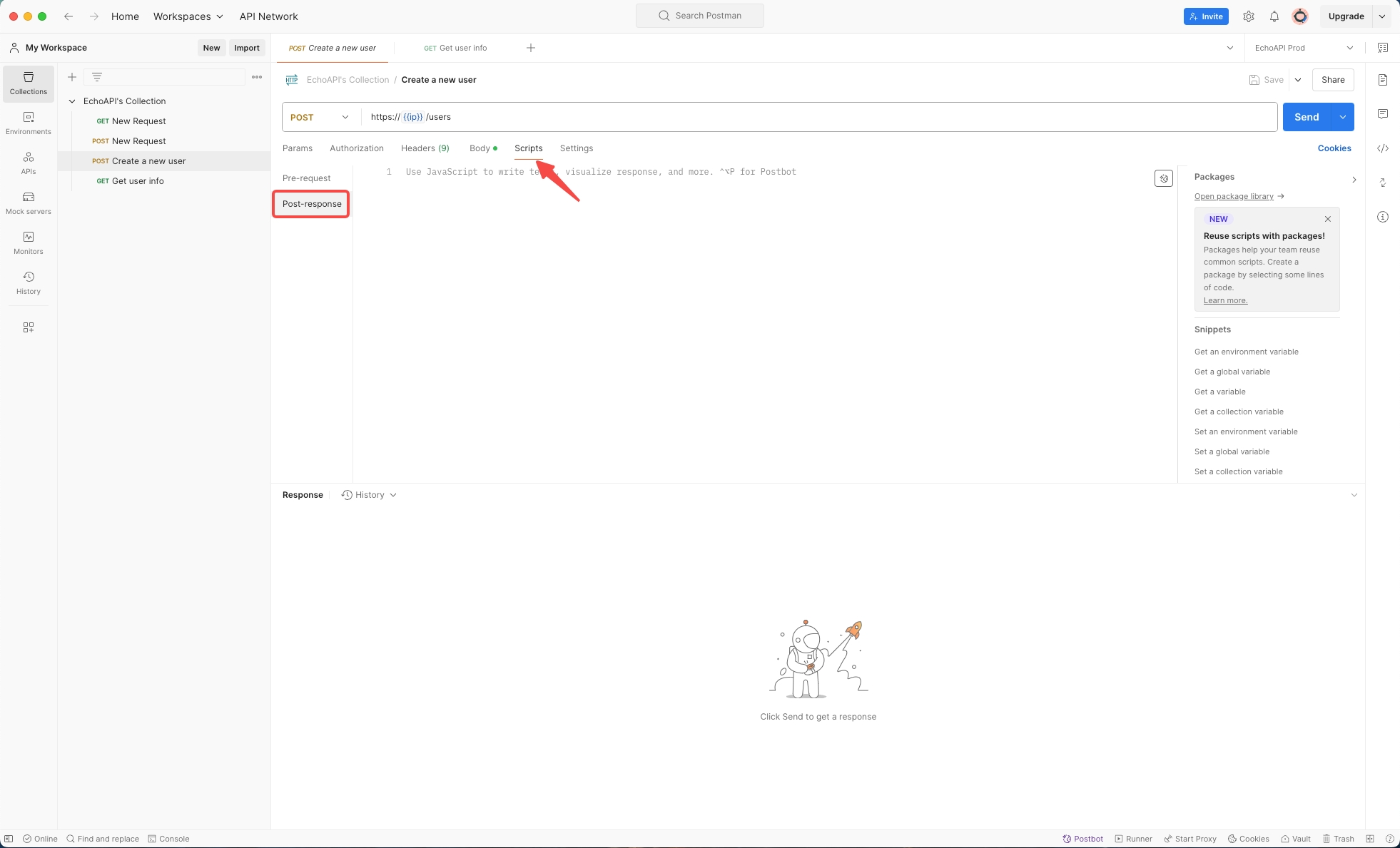
Start by logging the response body to the console:
console.log(responseBody);
Here, responseBody
represents the data returned from the request. You can view the printed information in the Console panel located at the bottom-right of the interface.
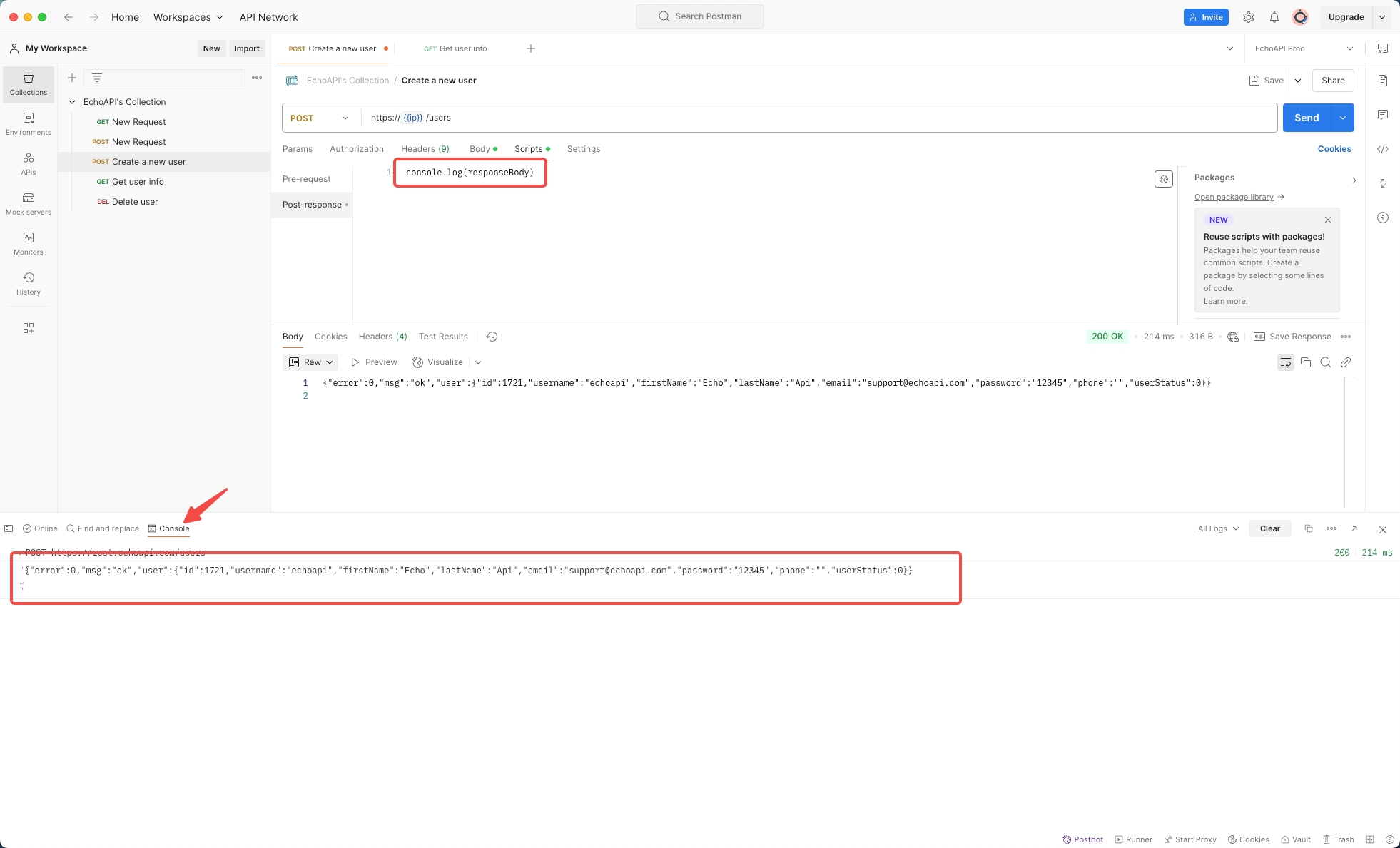
Step 2: Extract the Username
Using the JSON extractor, you can retrieve the username
value from the response.
First, convert the returned data string into an object with:
var result = JSON.parse(responseBody);
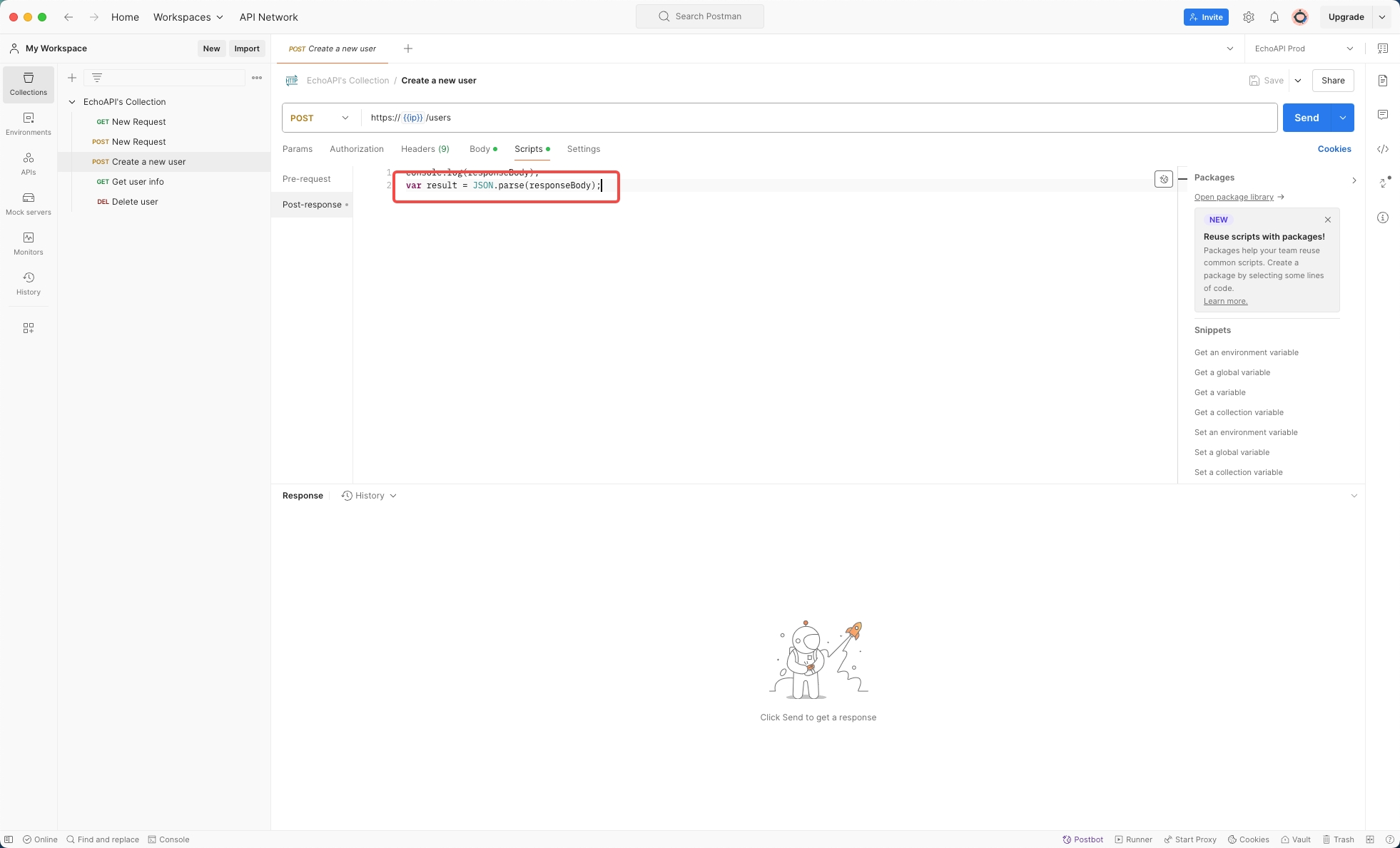
Next, ensure you've successfully extracted the username by printing it:
console.log(result.user.username);
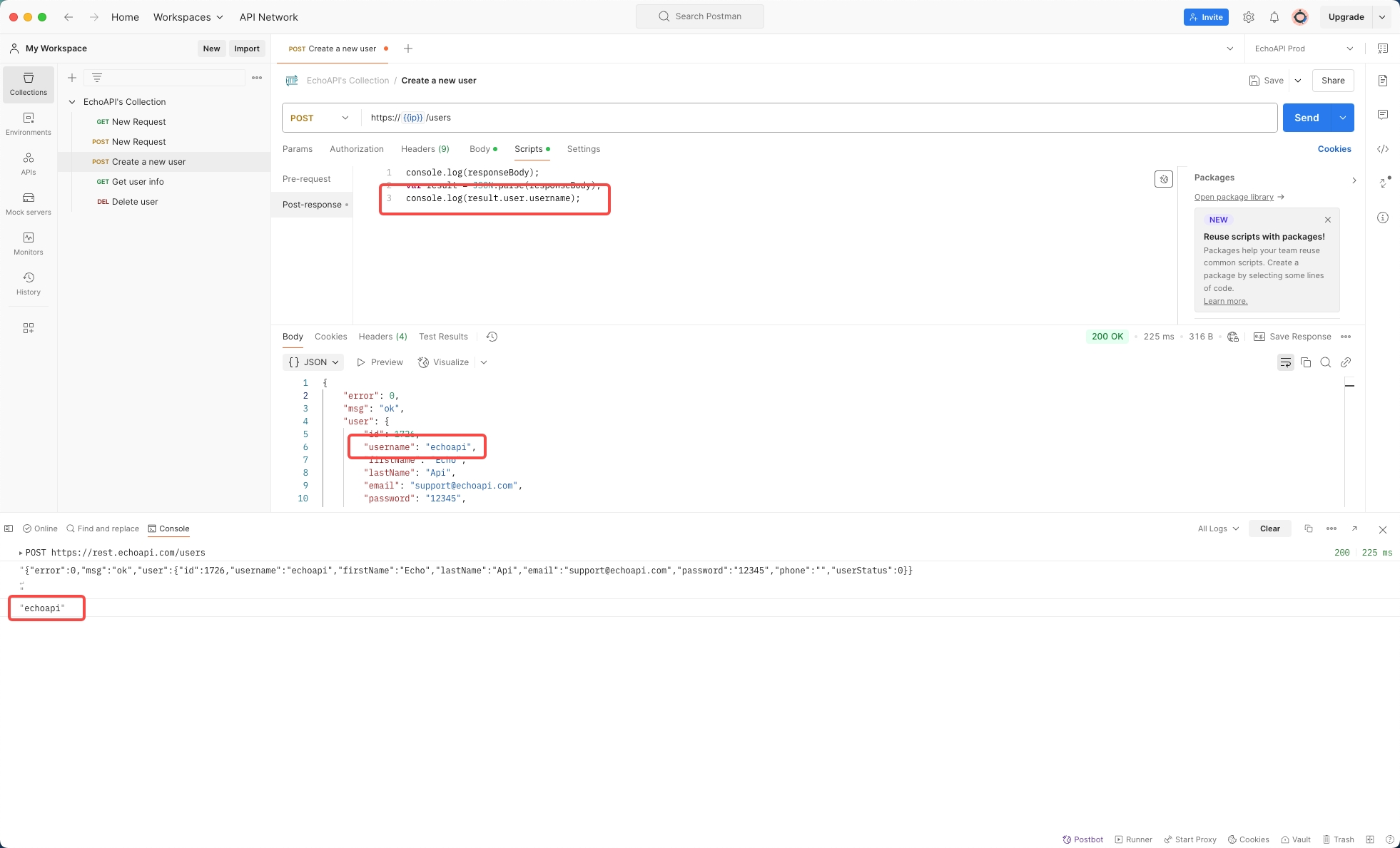
Step 3: Set the Username as a Global Variable
Once you've confirmed the correct extraction of the username, store it as a global variable:
pm.globals.set("username", result.user.username);
This command assigns the username to a global variable, making it accessible across different requests.
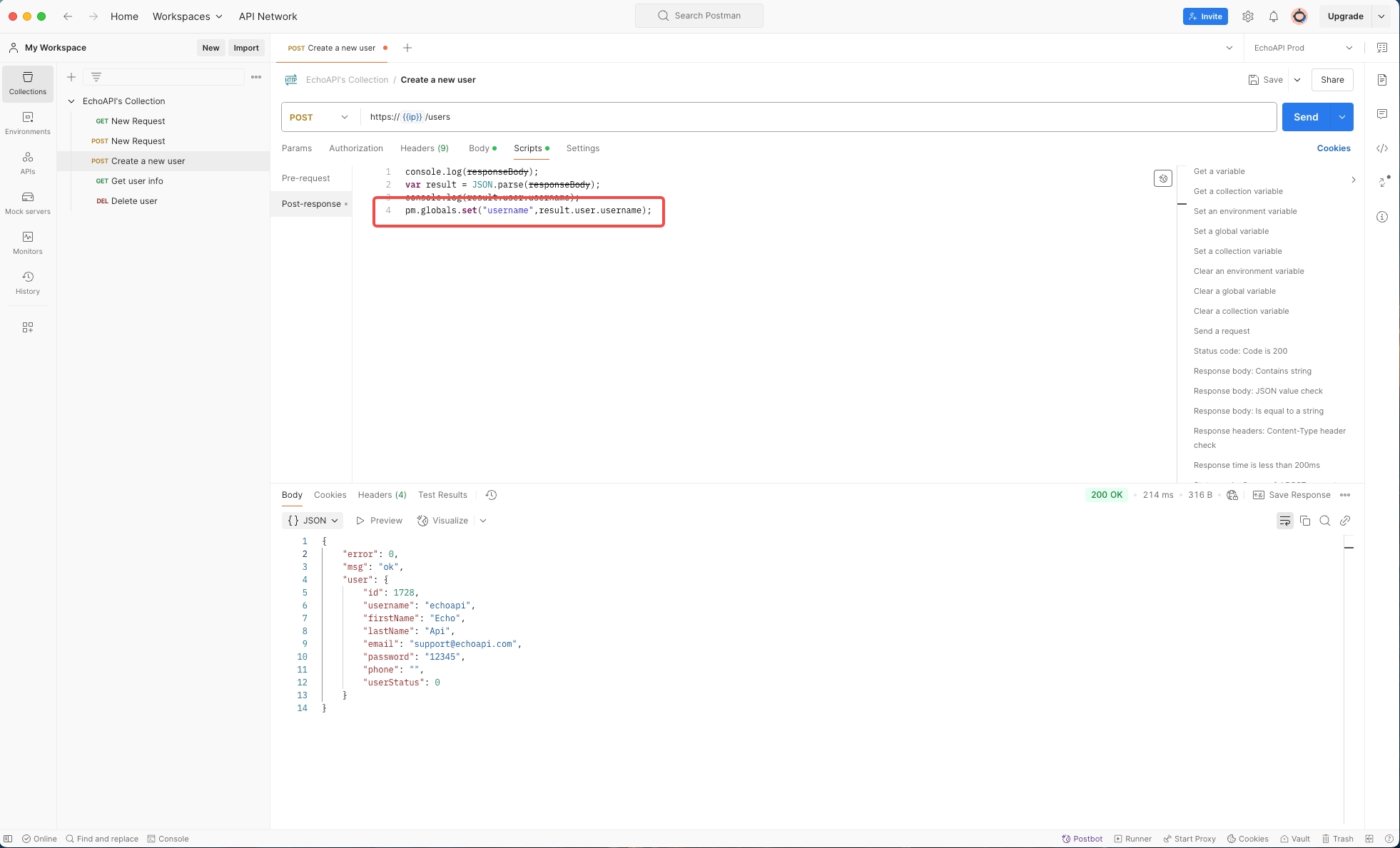
To confirm if the global variable is set correctly, you can click on the top-right button in Postman that displays global variables.
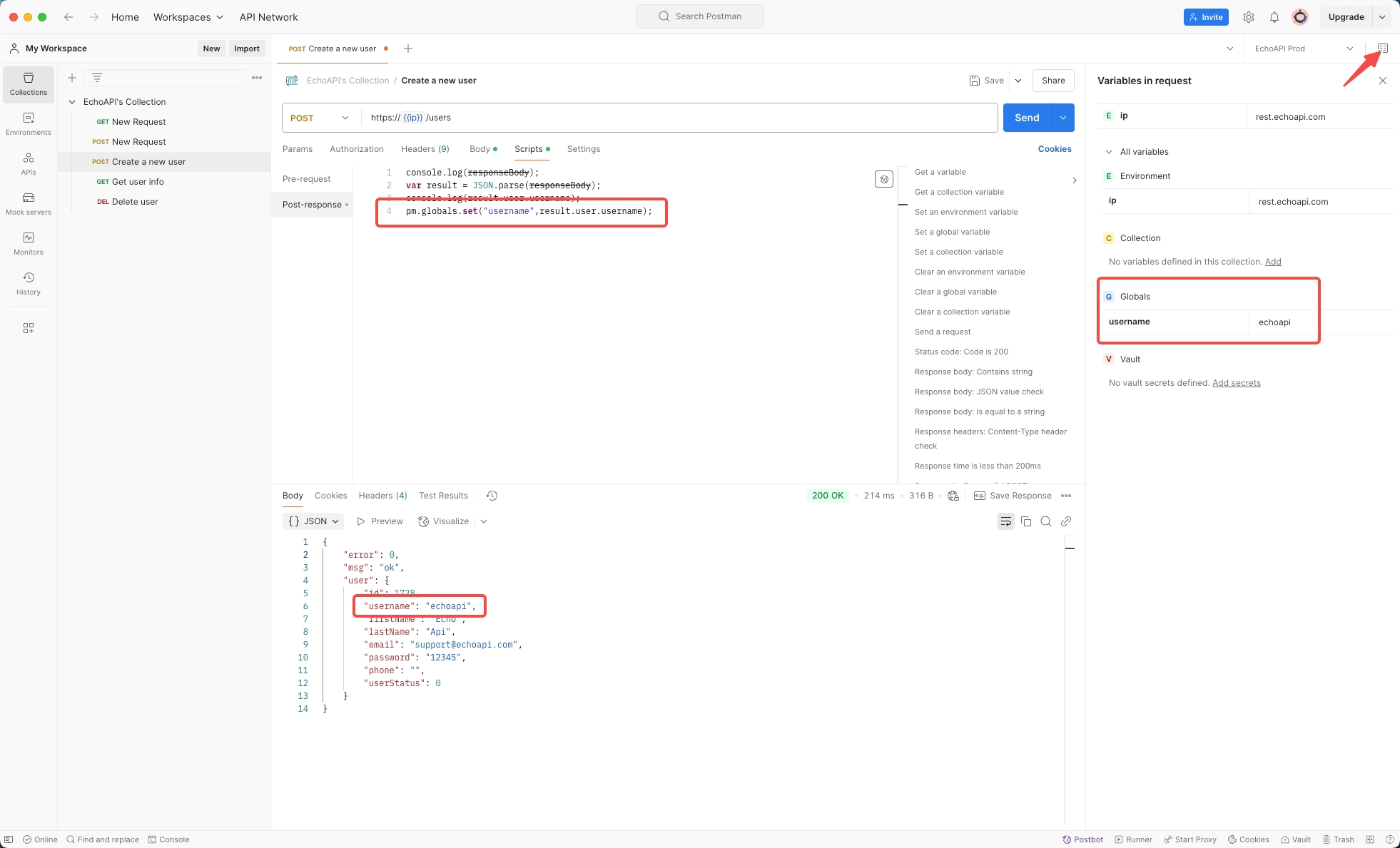
Step 4: Reference the Global Variable in "Get User Info" API
In the "get user info" API, you can reference the extracted username by using the global variable syntax: {{username}}
. This enables seamless API chaining, where the result of one API automatically informs the request of another.
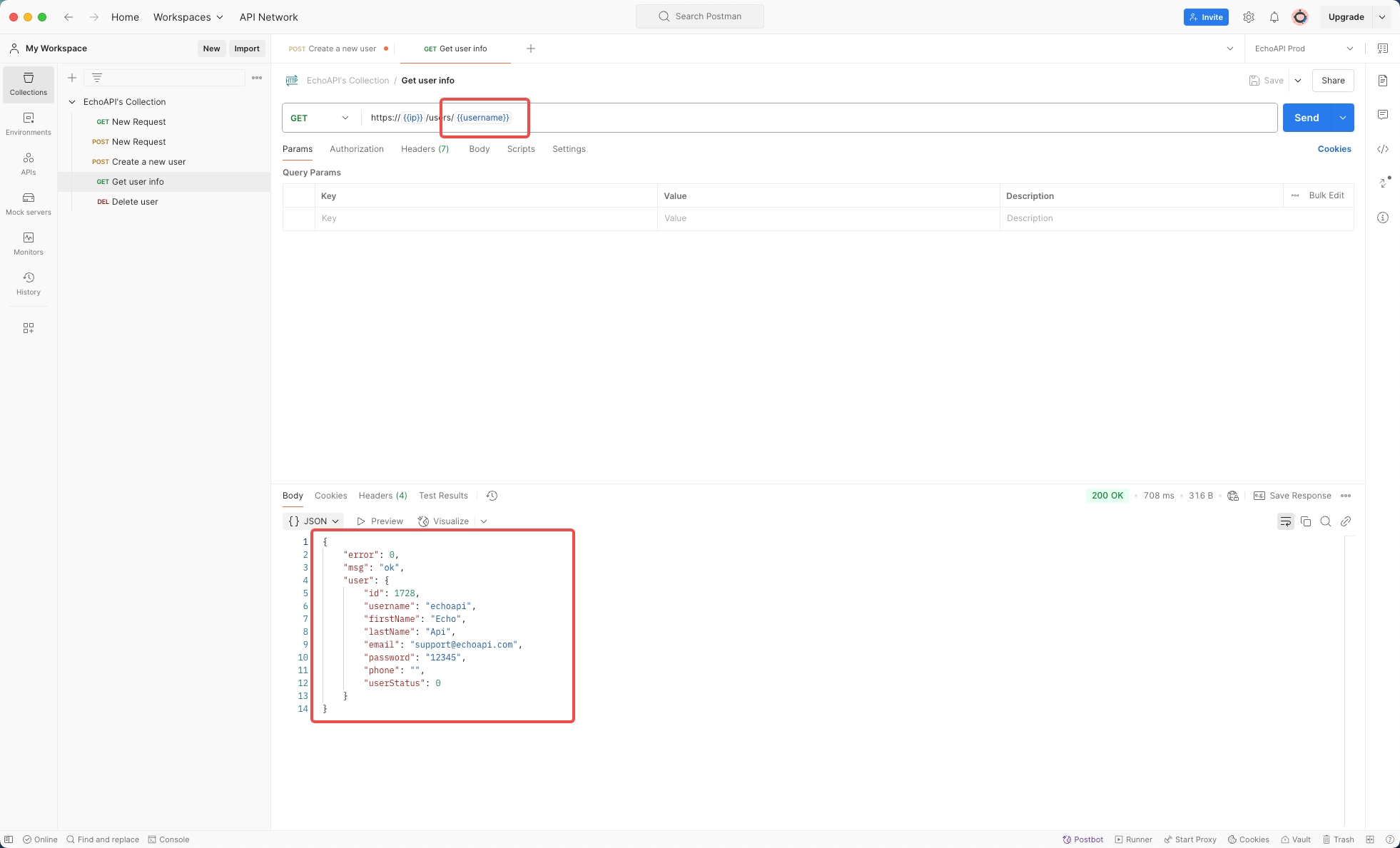
Conclusion
By utilizing the JSON extractor in Postman, you can simplify the process of API chaining, making your testing workflow more efficient and less prone to errors. As a developer, mastering this feature not only saves time but also enhances the robustness of your tests. Being able to automatically pass data between requests is a powerful capability that helps ensure the integrity and continuity of your API testing processes.