Mastering Postman: Implementing API Chaining with the Regex Extractor
In this article, we'll explore how to use the Regex extractor in Postman for API chaining, enhancing automation and efficiency in API testing.
In our previous article, we explored how to implement API chaining using the JSON extractor. If you're interested in diving deeper into that topic, feel free to follow this link to the article.
Today, we will focus on another essential technique for API testing in Postman—utilizing the Regex extractor for API chaining.
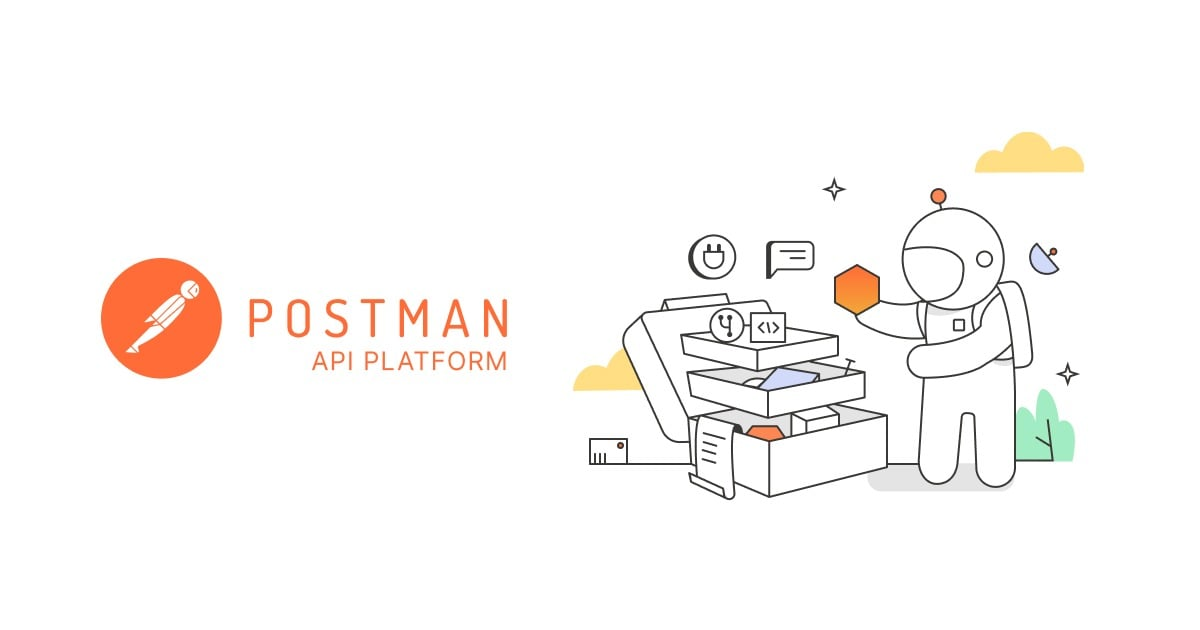
Implementing API Chaining with a Regex Extractor
So, how can we achieve API chaining using a Regex extractor? Here’s a step-by-step guide:
Step 1: Access the Post-Response Script Section
First, navigate to the Post-response section of your API request. This is where you’ll script the extraction process.
Begin with:
responseBody.match()
The match
function is used to match a regular expression against the body of the response data.
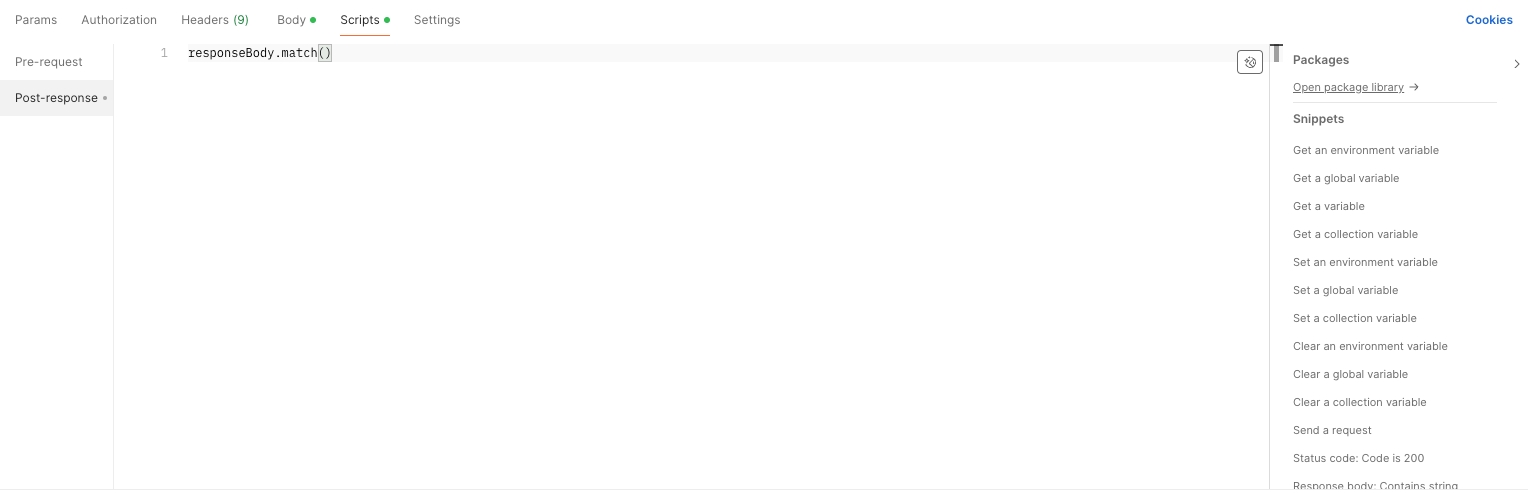
Step 2: Create a Regex Pattern
Your next step is to define a regular expression within the match
function:
responseBody.match(new RegExp())
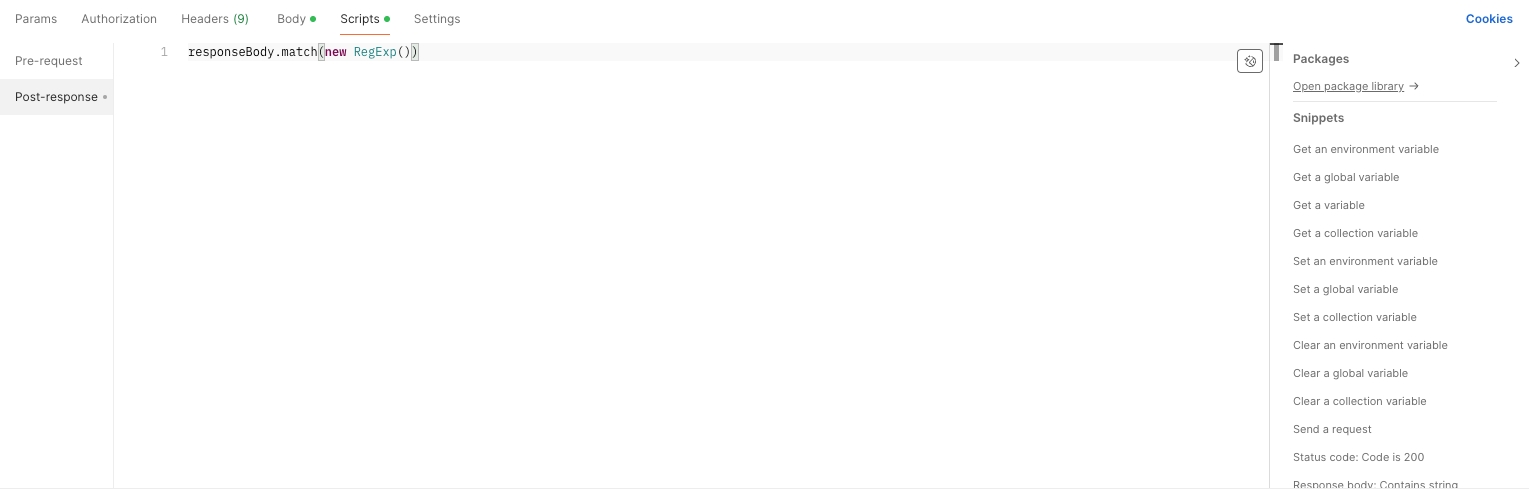
To write an effective regex pattern, first examine the raw format of your returned data in Postman.
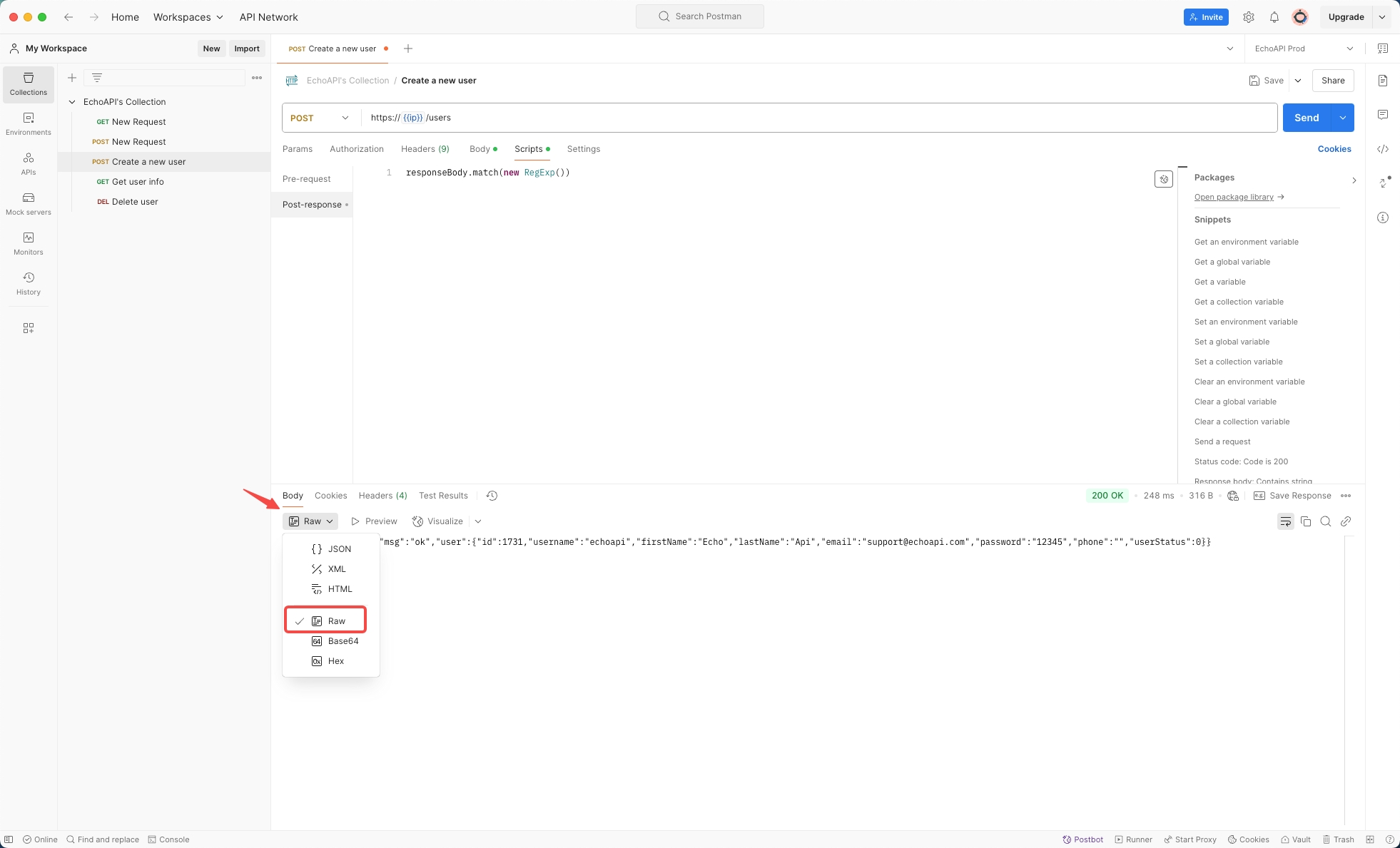
Identify the specific part you’d like to extract—let's say we're targeting the username
. Encapsulate this target in single quotes and insert it into your regex:
responseBody.match(new RegExp('"username":"echoapi"'))
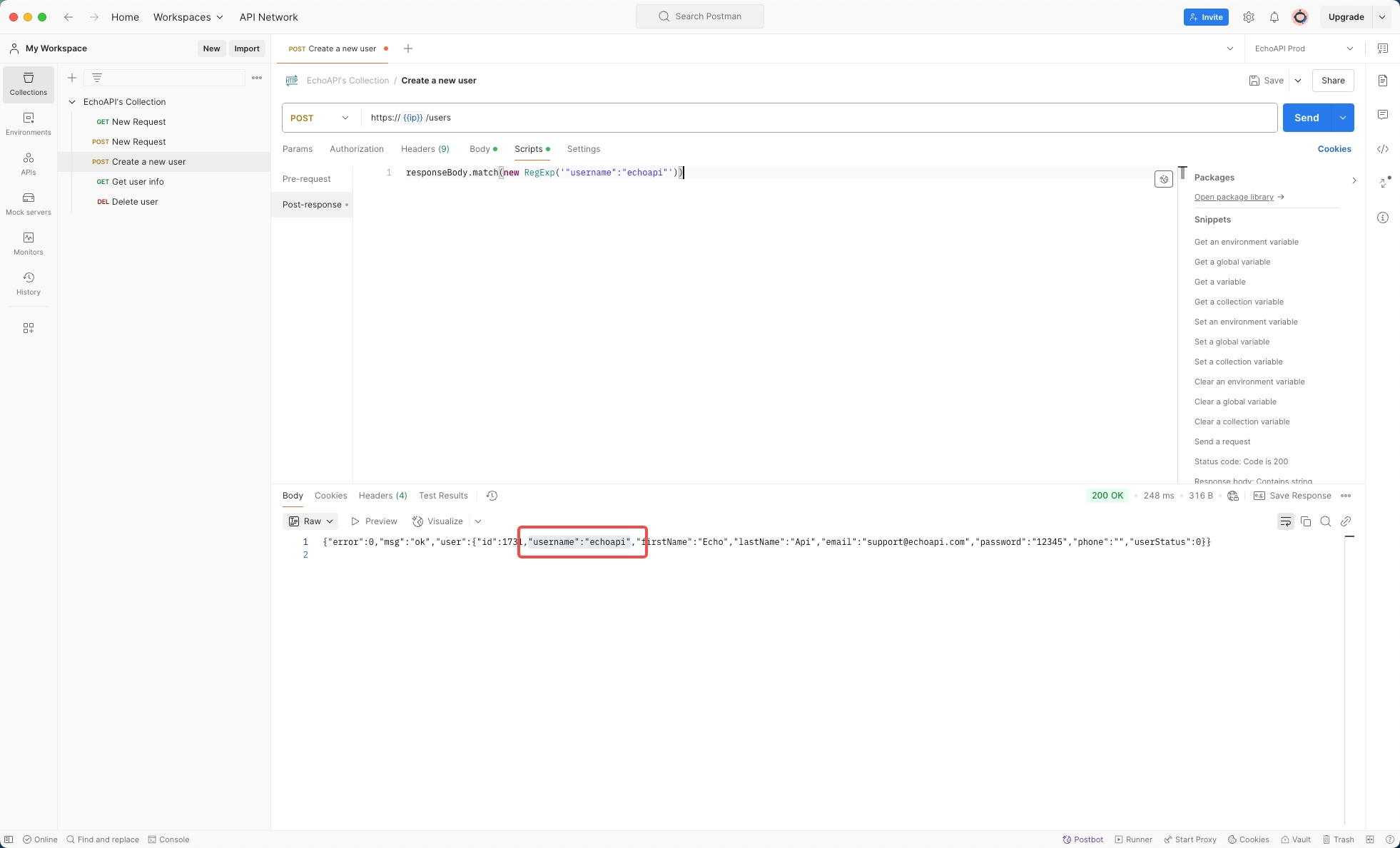
Now, replace the static value with a capturing group, using (.*?)
to allow dynamic extraction:
responseBody.match(new RegExp('"username":"(.*?)"')))
Step 3: Capture and Store the Extracted Value
Assign the matched result to a variable named result
:
var result = responseBody.match(new RegExp('"username":"(.*?)"'));
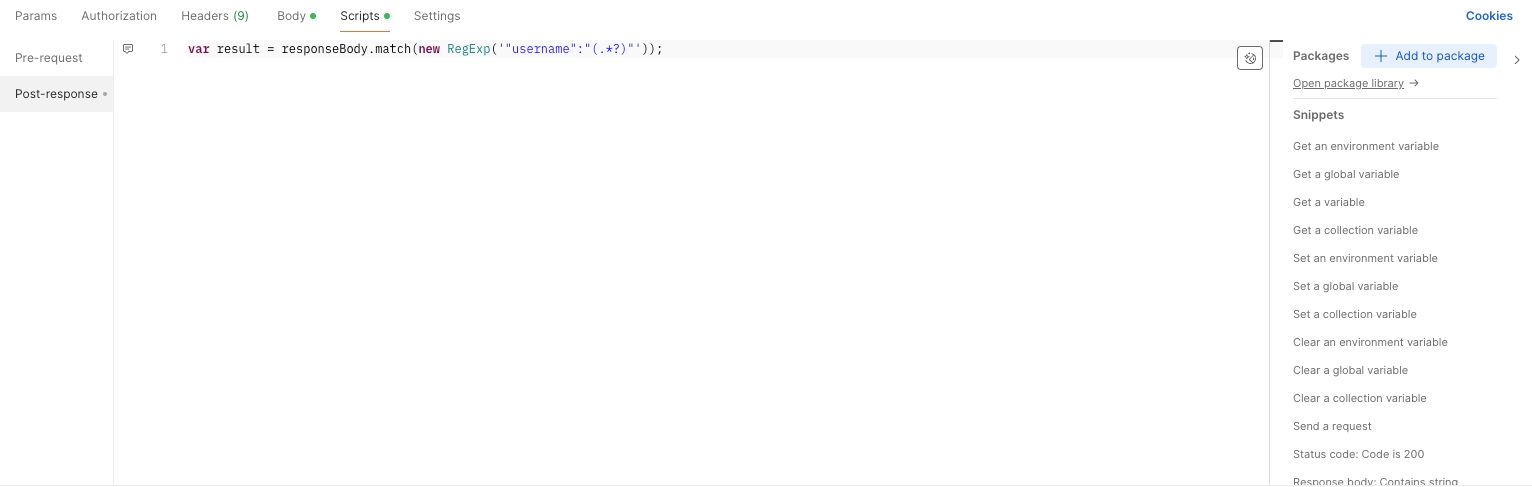
Print the result
to inspect the structure of the extracted data:
console.log(result);
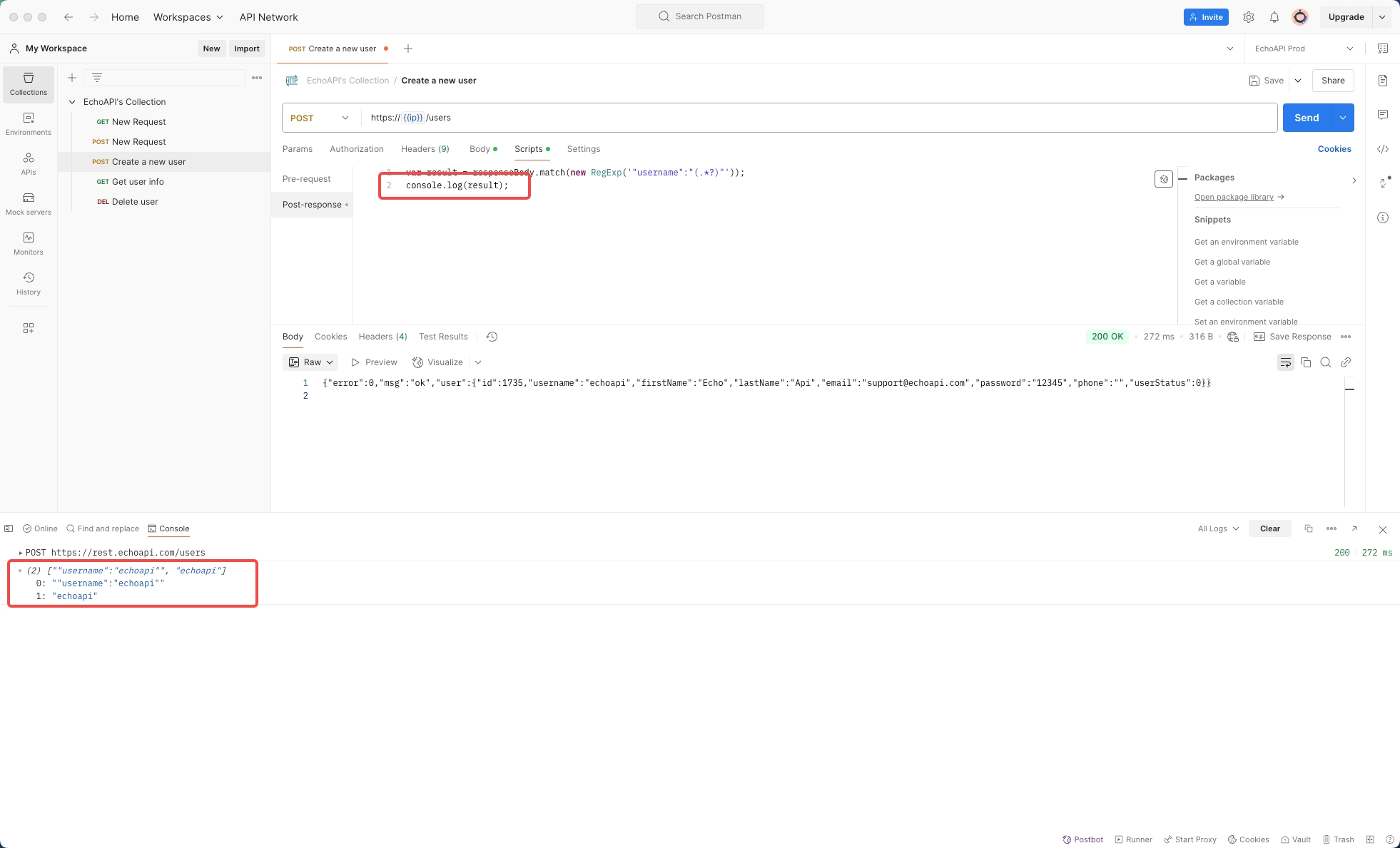
You'll notice result
contains an array where result[0]
holds the entire match, and result[1]
carries the extracted value we need. Print result[1]
to confirm you've obtained the correct value:
console.log(result[1]);
![result[1].jpg result[1].jpg](https://assets.echoapi.com/upload/user/218821375908265984/log/4da9528e-f972-4c07-8071-8b97748b312c.jpg)
Step 4: Set the Value as a Global Variable
Once verified, set the username
as a global variable:
pm.globals.set("username", result[1]);
This operation saves the username globally, making it accessible in other parts of your Postman tests.
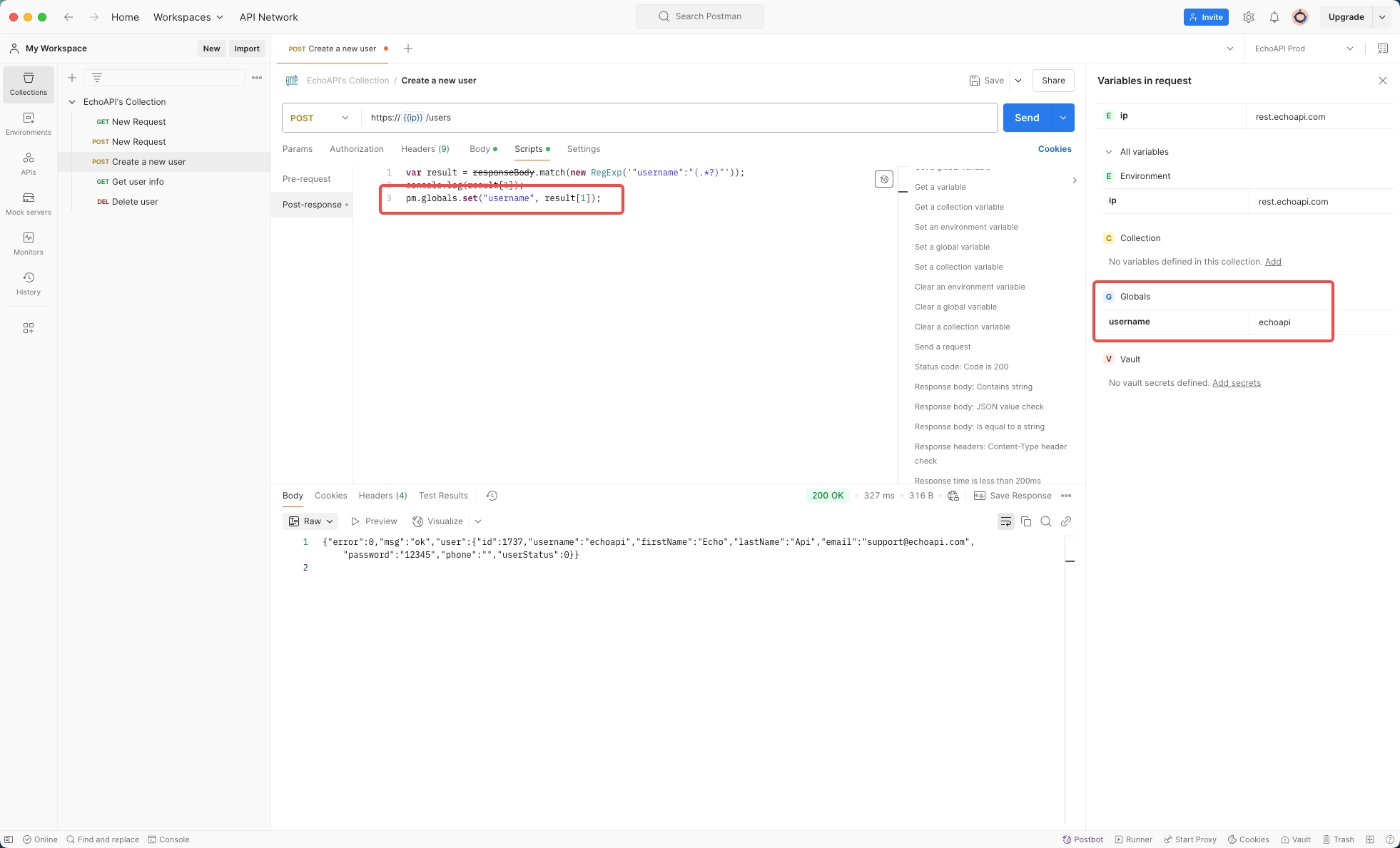
Step 5: Use the Global Variable in Another API Request
Now, move to the "get user info" API request. Here, you can reference the username
using the global variable syntax: {{username}}
. When you send this request, Postman uses the value extracted from the previous step, effectively chaining the two API calls.
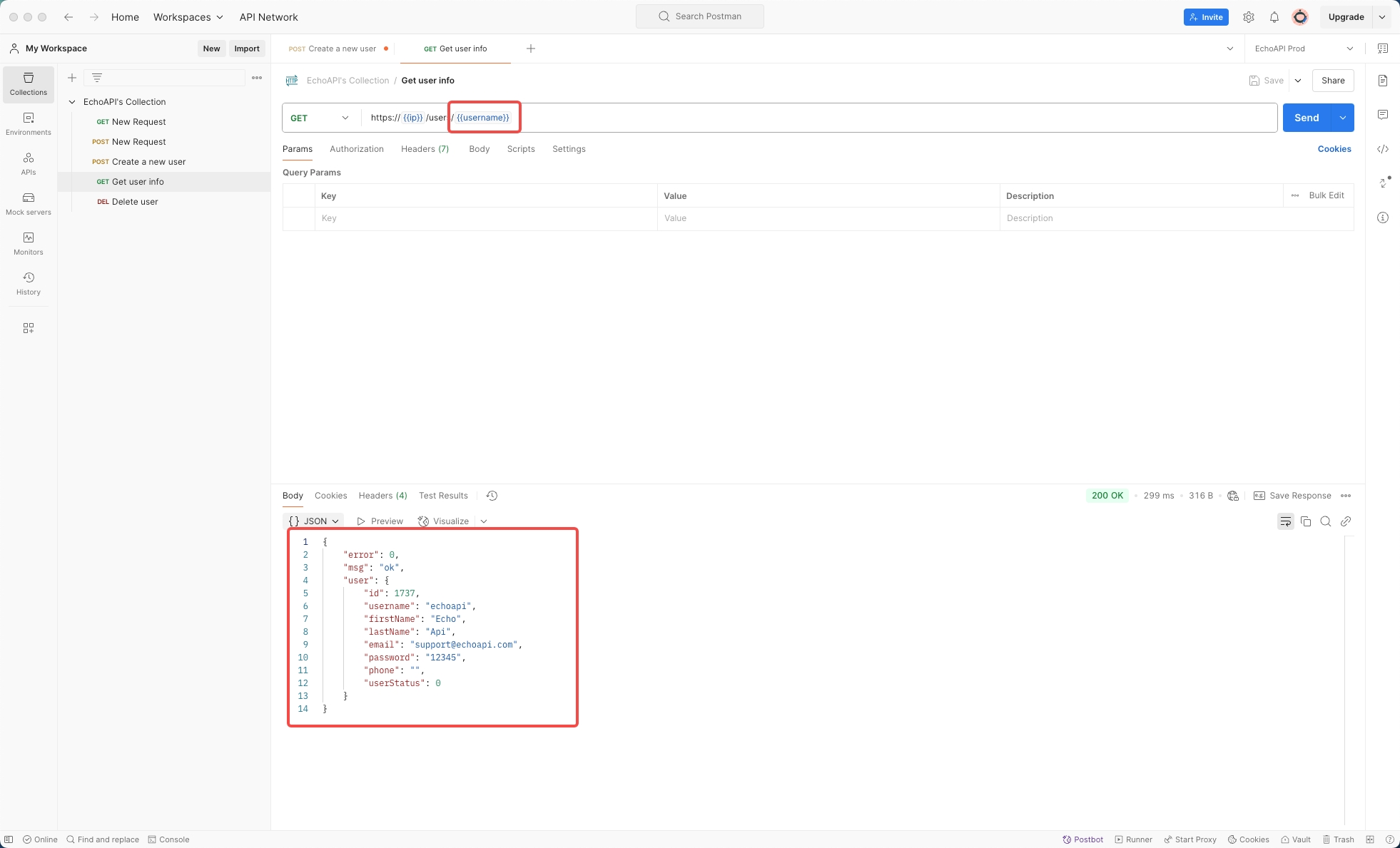
Conclusion
Using a regex extractor in Postman not only enhances your ability to automate API testing but also saves time by eliminating the need for manual data handling between requests. Mastering this technique enables you as a developer to perform more comprehensive and accurate testing, further ensuring robust and efficient API integrations. Embrace the power of regex in Postman to streamline your workflow and enhance your development toolkit.