Secrets to Creating API Documentation and the Latest Free Tools
API documentation is the backbone of modern software development, providing developers with the detailed information necessary to effectively utilize and integrate APIs. This article explores the concept, importance, best practices, and advanced tools for creating excellent API documentation.
API documentation is the backbone of modern software development, providing developers with the detailed information necessary to effectively utilize and integrate APIs. This article explores the concept, importance, best practices, and advanced tools for creating excellent API documentation.
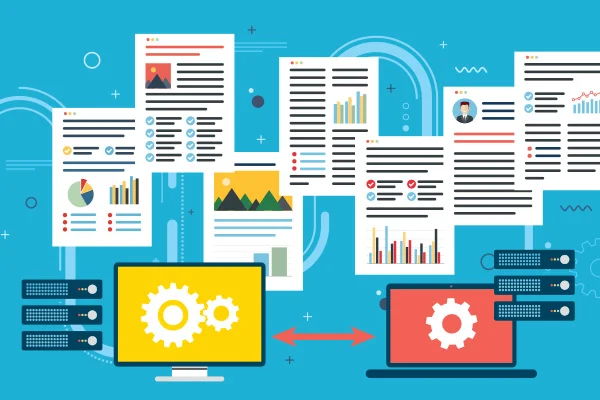
What is API Documentation?
API documentation is a technical guide explaining how to effectively use and integrate an API. It provides detailed information on API endpoints, methods, request parameters, response formats, authentication methods, error codes, and usage examples. Good API documentation offers developers all the information they need to understand and interact with an API.
Key Elements of API Documentation
- Endpoint Definitions: Detailed info for each API endpoint, including URL, HTTP methods, and required parameters.
- Authentication: Explanation of request authentication methods, token generation, and scope definitions.
- Request/Response Examples: Samples showing the API’s actual functionality.
- Error Handling: Explanation of potential error codes and messages to help developers resolve issues.
- Code Samples: Examples of how to implement API calls in various programming languages.
Importance of API Documentation
Enhance Developer Experience
Clear and comprehensive documentation reduces the learning curve, helping developers integrate APIs quickly and efficiently. It acts as a self-guide, minimizing support needs and speeding up development.
Aid New Developer Onboarding
Comprehensive API documentation helps new developers understand and use the API swiftly, reducing the learning curve and accelerating the development process.
Promote Collaboration
API documentation serves as a common reference for development teams, fostering collaboration. It ensures all team members have a consistent understanding of the API's behavior, facilitating coordinated development efforts.
Boost API Adoption
Well-documented APIs are more likely to be adopted by developers. Easy-to-navigate and understand documentation encourages more developers to use and recommend the API, expanding its reach and impact.
Reduce Support Costs
Good documentation reduces the need for extensive support by allowing developers to find answers to their questions directly within the documentation, easing the burden on support teams and minimizing downtime.
API Documentation Template
A basic API documentation template might include:
Introduction
- Overview of the API
- Use cases
Authentication
- Authentication methods
- API keys
Endpoints
- Endpoint URLs
- HTTP methods (GET, POST, PUT, DELETE)
- Request parameters
- Response formats
Error Codes
- List of error codes
- Descriptions and solutions
Rate Limiting
- Rate limit information
- Handling rate limit errors
Examples
- Request and response examples
- Code snippets in various programming languages
Standards for API Documentation
OpenAPI Specification (OAS)
The OpenAPI Specification is a standard for defining RESTful APIs, describing APIs in a format readable to both humans and machines.
RAML (RESTful API Modeling Language)
RAML is a standard for documenting RESTful APIs, focusing on providing readable and writable API documentation.
API Blueprint
API Blueprint is a standard for API design and documentation, emphasizing simplicity and readability.
Writing API Documentation
Understand Your Audience
Before writing, understand who will use the API and what they need. This ensures that the documentation meets users’ requirements.
Use Clear and Concise Language
Write simply and directly. Avoid jargon and complex sentences. The goal is to make documentation easy to read and understand.
Provide Detailed Information
Include all necessary details about endpoints, methods, request and response formats, authentication methods, error codes, and rate limits.
Include Examples
Provide code examples in various programming languages to show developers how to implement the API. Examples are especially helpful.
Use Visuals
Where applicable, incorporate diagrams, flowcharts, and screenshots. Visuals make complex concepts easier to understand.
Keep It Updated
Regularly update the documentation to reflect API changes and new features. Outdated documentation can cause confusion and errors.
Case Study: API Documentation Dilemma
In fast-paced software development, ensuring API documentation is accurate and user-friendly is crucial. Recently, a tech team faced severe issues due to poor API documentation.
Incident
A backend developer provided auto-generated Swagger UI API documentation, but it was problematic:
- Complex, hard-to-understand layered models
- Too many APIs made finding specifics difficult
- JSON formatting issues, with no formatting feature, making parameter submission challenging
- Difficult to identify incorrect parameters
- Responses too lengthy to read efficiently
The frontend team rushed implementation based on the provided documentation to meet a deadline. However, once the application went live, numerous API errors caused crashes due to missing parameters, incorrect parameter types, and nonexistent interfaces, leading to heated debates between frontend and backend teams.
Root Causes
The CTO intervened, calmly analyzing the situation and identifying key causes:
- Backend negligence: Some API documentation was incorrectly written and poorly debugged.
- Time constraints: Frontend developers didn't have enough time to thoroughly test the API.
The CTO noted these issues stemmed from inadequate tools and a lack of sufficient testing time. The team then sought an API documentation tool with the following capabilities:
- Debugging Features: Allow frontend developers to easily debug APIs directly from the documentation.
- Code Generation: Reduce the need for manual code writing, improving efficiency and accuracy.
- Real-time Sync: Ensure documentation always reflects the latest code information.
Final Solution for the Team – Advanced Free Tools
The team eventually chose EchoAPI, a comprehensive API development tool integrating features from Postman, Swagger, Mock, and JMeter. With EchoAPI, the following capabilities are available:
- Online Debugging: Facilitates real-time API debugging.
- Code Generation: Automatically generates API request and response codes.
- Cloud Mock: Creates virtual servers for unlimited free API requests during testing.
Best Practices for Creating API Documentation with EchoAPI
API documentation is crucial for API usability and success. Well-documented APIs significantly enhance the developer experience, accelerating adoption and fostering a strong developer community. Conversely, poor documentation can lead to confusion, frustration, and errors, resulting in low adoption rates. This section explores advanced best practices, providing examples for creating more understandable API documentation.
1. Clearly Define the API’s Purpose
Before writing the documentation, clearly understand the API’s purpose. What problem does it solve? Who is the target audience? What are the main use cases?
- Target Audience: Tailor the documentation to the specific needs of your users. Are you writing for experienced developers, content creators, or both?
- Use Cases: Clearly illustrate common use cases to give meaning to the API’s functionality.
- Example: Documenting a weather API aims to provide real-time weather data to various applications, targeting developers building weather apps or integrating weather data into existing services.
2. Organize the Documentation for Easy Navigation
Well-structured documentation is easy to navigate and understand. Use logical sections and subsections.
- Introduction: Provide an overview of what the API does and why it’s useful.
- Getting Started: Offer a quick-start guide with setup steps, authentication processes, and simple examples to get users up and running quickly.
Example:
## Introduction
The WeatherAPI provides real-time weather information for any location in the world. It can be used to get current weather conditions, forecasts, and historical weather data.
## Getting Started
To start using WeatherAPI, you need to sign up at our [website](#) to obtain an API key.
- Endpoints Overview: Present a summary table or list of all available endpoints.
- Detailed Endpoint Documentation: Include the following information for each endpoint:
Example:
## Endpoints Overview
| Endpoint | Method | Description |
|----------------|--------|---------------------------------|
| `/current` | GET | Get current weather data |
| `/forecast` | GET | Get weather forecast |
| `/historical` | GET | Get historical weather data |
## Current Weather Data
### Endpoint URL
`/current`
### HTTP Method
`GET`
### Summary
Retrieve current weather conditions for a specific location.
### Parameters
- **location** (string, required): The location for which to retrieve weather data.
- **units** (string, optional): Units of measurement (metric or imperial).
### Example Request
```sh
curl -X GET "https://api.weather.com/current?location=London&units=metric&apikey=your_api_key"
Example Response
{
"location": "London",
"temperature": 15,
"units": "metric",
"description": "Partly cloudy"
}
Error Codes
- 400: Invalid location
- 401: Unauthorized (Invalid API key)
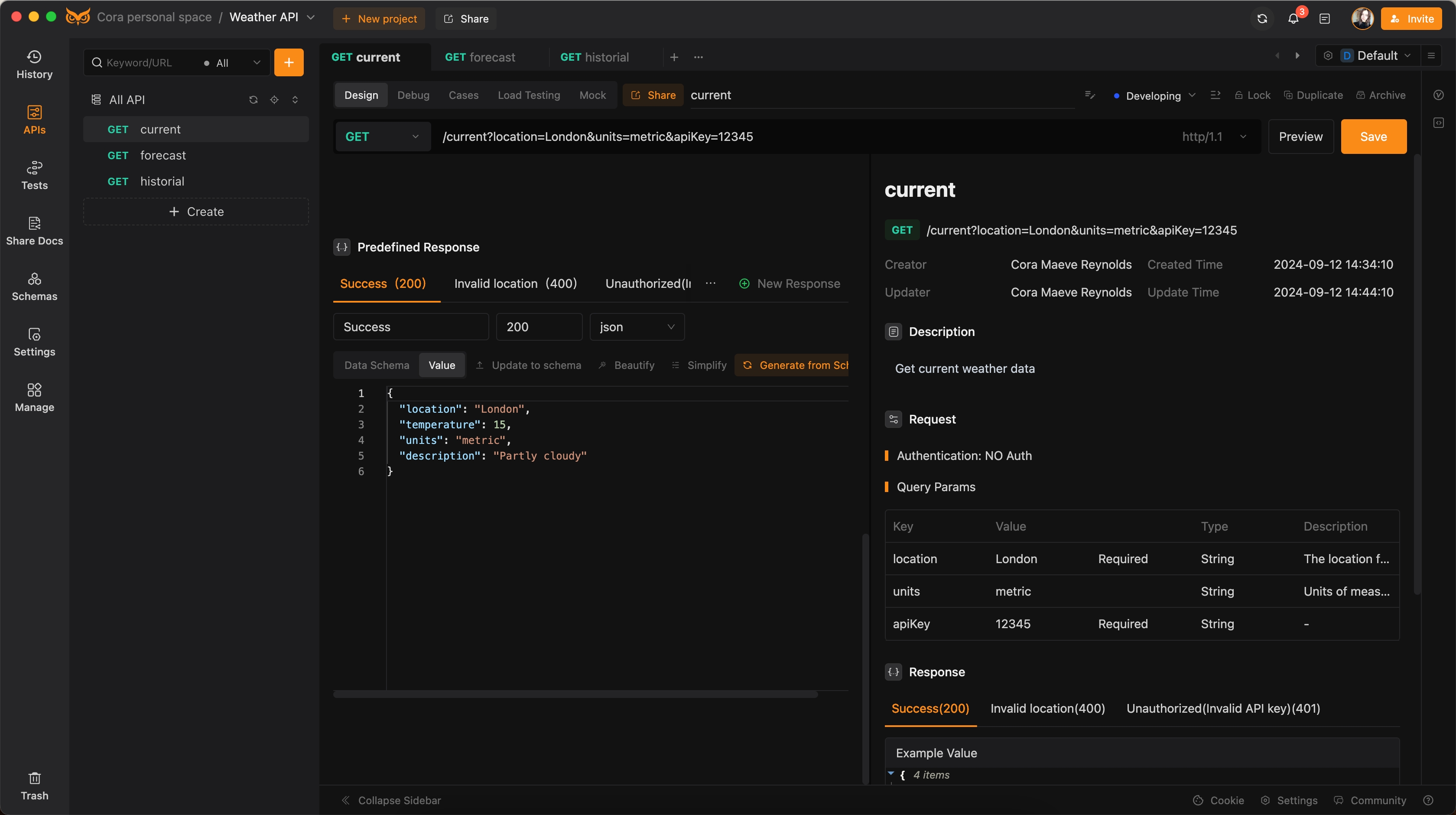
3. Provide Detailed Explanations and Use Examples
General explanations are important, but providing specific examples greatly enhances understanding of the API.
- Scenario-based Examples: Show examples based on common scenarios, contextualizing the information for easier understanding.
Example:
## Use Cases
### Scenario: Displaying Current Weather in a Mobile App
To display current weather conditions in your mobile app, you can use the `/current` endpoint to fetch the latest data based on the user's location.
#### Step-by-Step Guide
1. Obtain the user's location coordinates.
2. Make a request to the `/current` endpoint with the location parameter.
3. Parse the JSON response to extract temperature and weather conditions.
4. Display the data in the app’s weather widget.
```sh
curl -X GET "https://api.weather.com/current?location=40.7128,-74.0060&units=metric&apikey=your_api_key"
{
"location": "New York",
"temperature": 71.6,
"units": "imperial",
"description": "Clear sky"
}
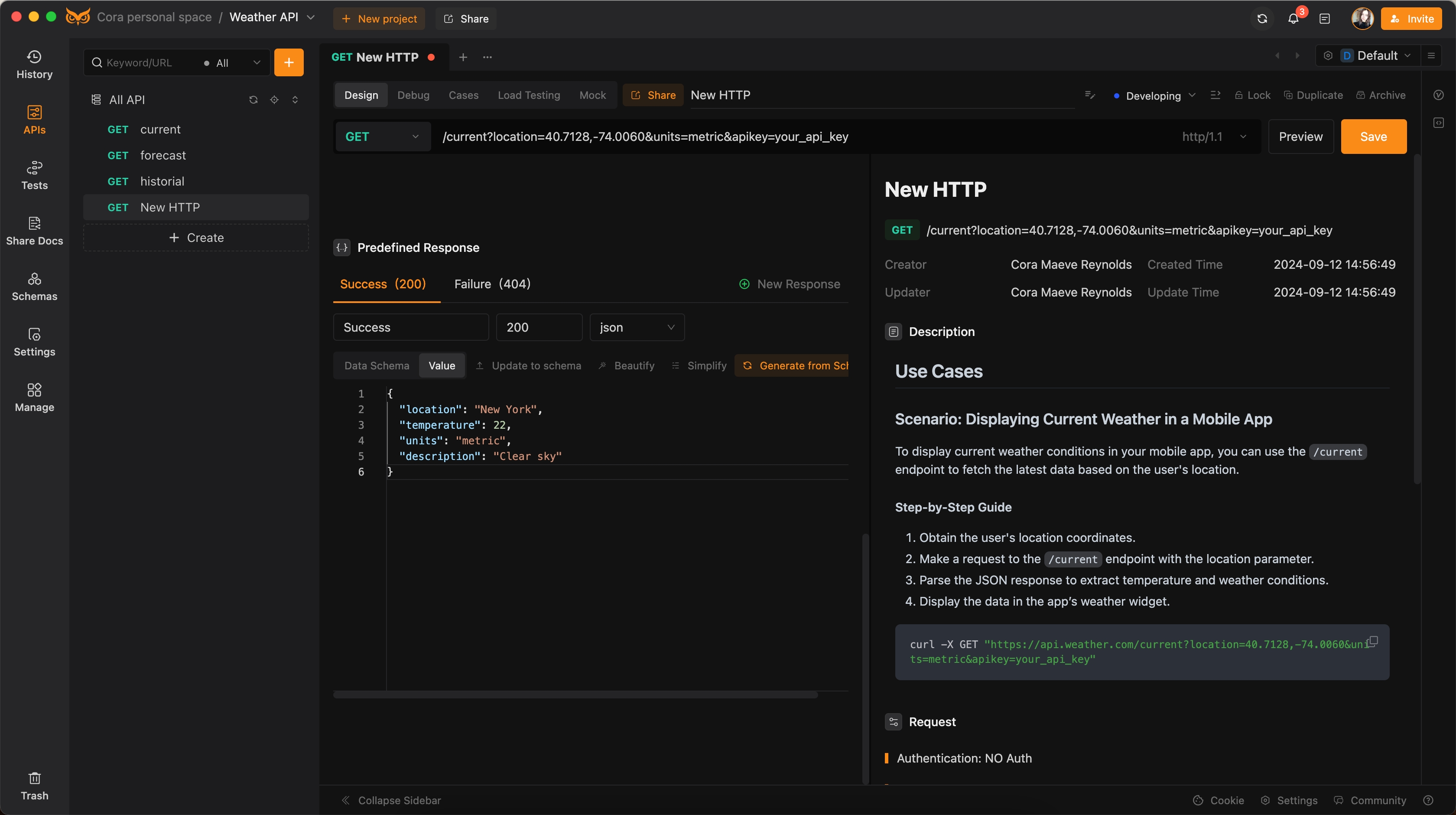
4. Use Consistent Style and Clear Language
Consistency and clarity are key to good documentation.
- Terminology: Use consistent terminology throughout the documentation.
- Language: Avoid jargon unless it's standard in the users’ field. Write concisely and directly.
- Formatting: Use consistent formatting for headings, code snippets, and emphasis.
5. Document Authentication and Authorization in Detail
Security is often a major concern for new API users. Cover authentication and authorization in detail.
- API Keys and Tokens: Explain how to obtain and use API keys, OAuth tokens, and other authentication methods.
Example:
## Authentication
WeatherAPI uses API keys to authenticate requests. You can obtain an API key by signing up on our website.
### How to Use an API Key
Pass your API key as a query parameter in your requests:
```sh
curl -X GET "https://api.weather.com/current?location=London&apikey=your_api_key"
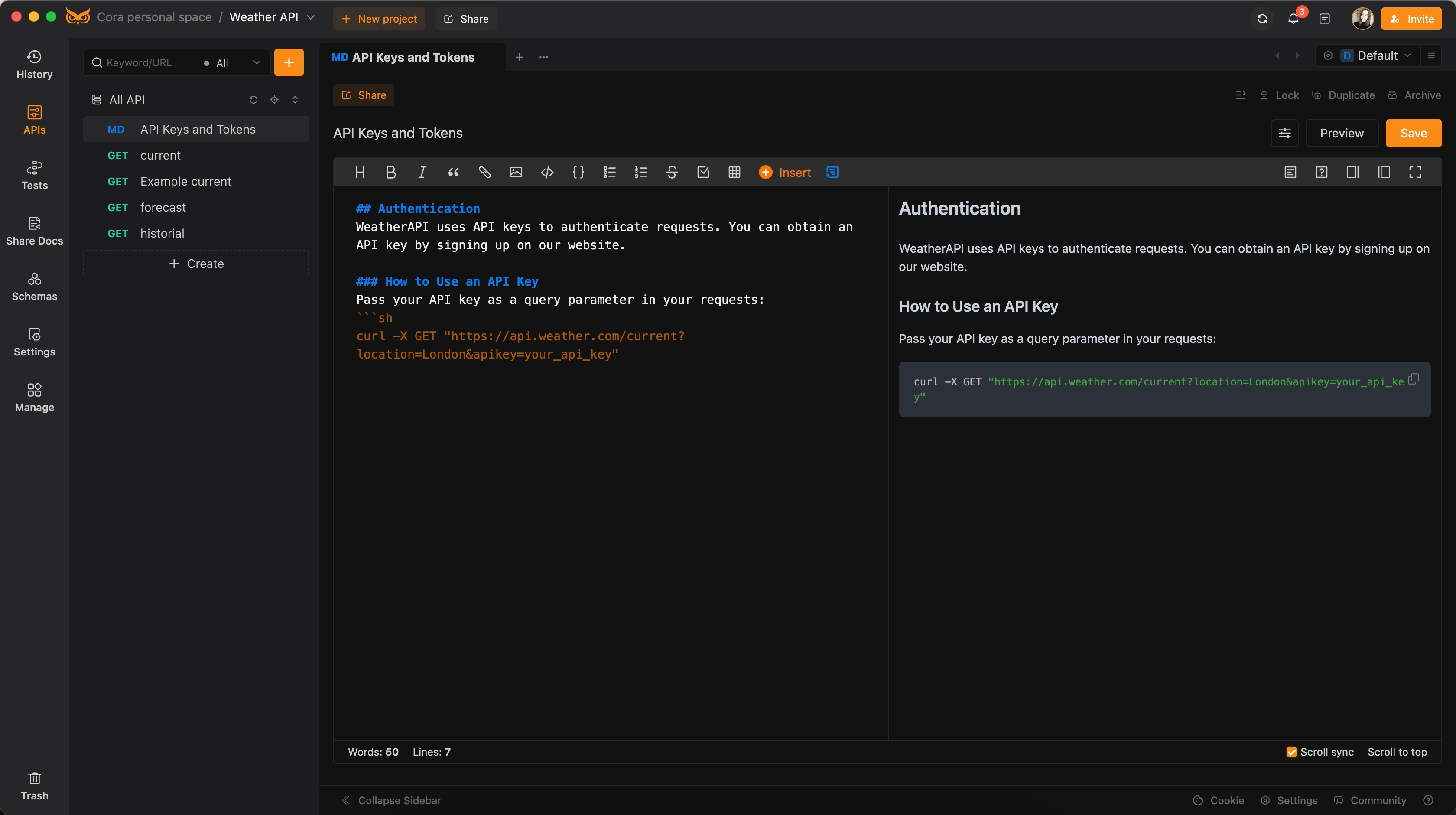
6. Include Code Samples and SDKs
Code samples help bridge the gap between abstract concepts and actual implementation.
- Language-specific Examples: Provide examples in multiple popular programming languages.
- SDKs: If SDKs are available, document their installation and usage.
- Copy & Paste: Offer examples that users can easily copy and try out.
Example:
## Code Samples
### Python Example
```python
import requests
response = requests.get(
"https://api.weather.com/current",
params={"location": "London", "apikey": "your_api_key"}
)
data = response.json()
print(f"Temperature: {data['temperature']}°C, Description: {data['description']}")
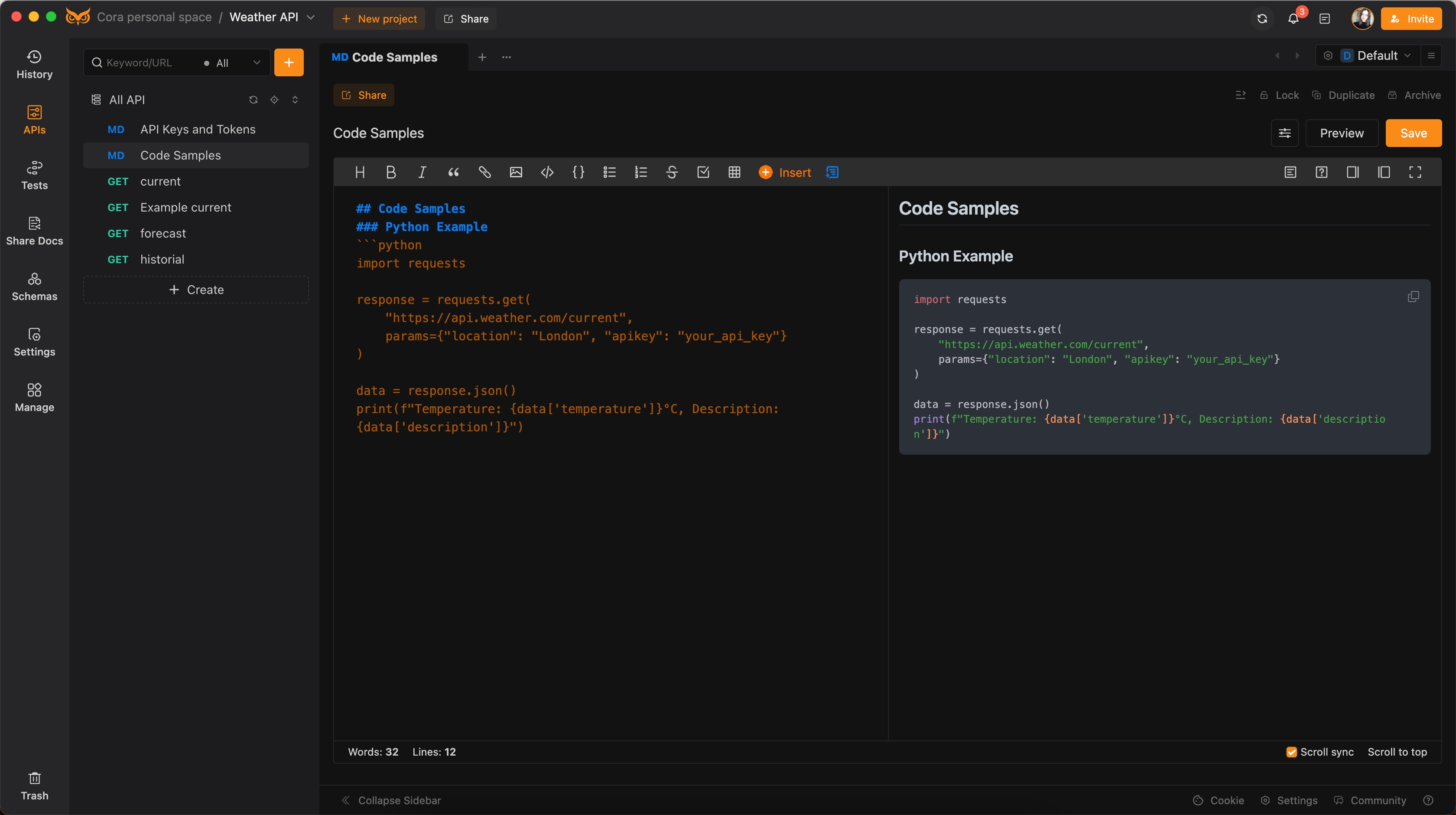
JavaScript Example
const fetch = require('node-fetch');
fetch("https://api.weather.com/current?location=London&apikey=your_api_key")
.then(response => response.json())
.then(data => {
console.log(`Temperature: ${data.temperature}°C, Description: ${data.description}`);
});
7. Provide Interactive Documentation
Interactive documentation significantly improves usability.
- EchoAPI: Use tools like EchoAPI to create interactive, self-documenting APIs.
- API Explorer: Implement an API explorer that allows users to test endpoints directly within the documentation.
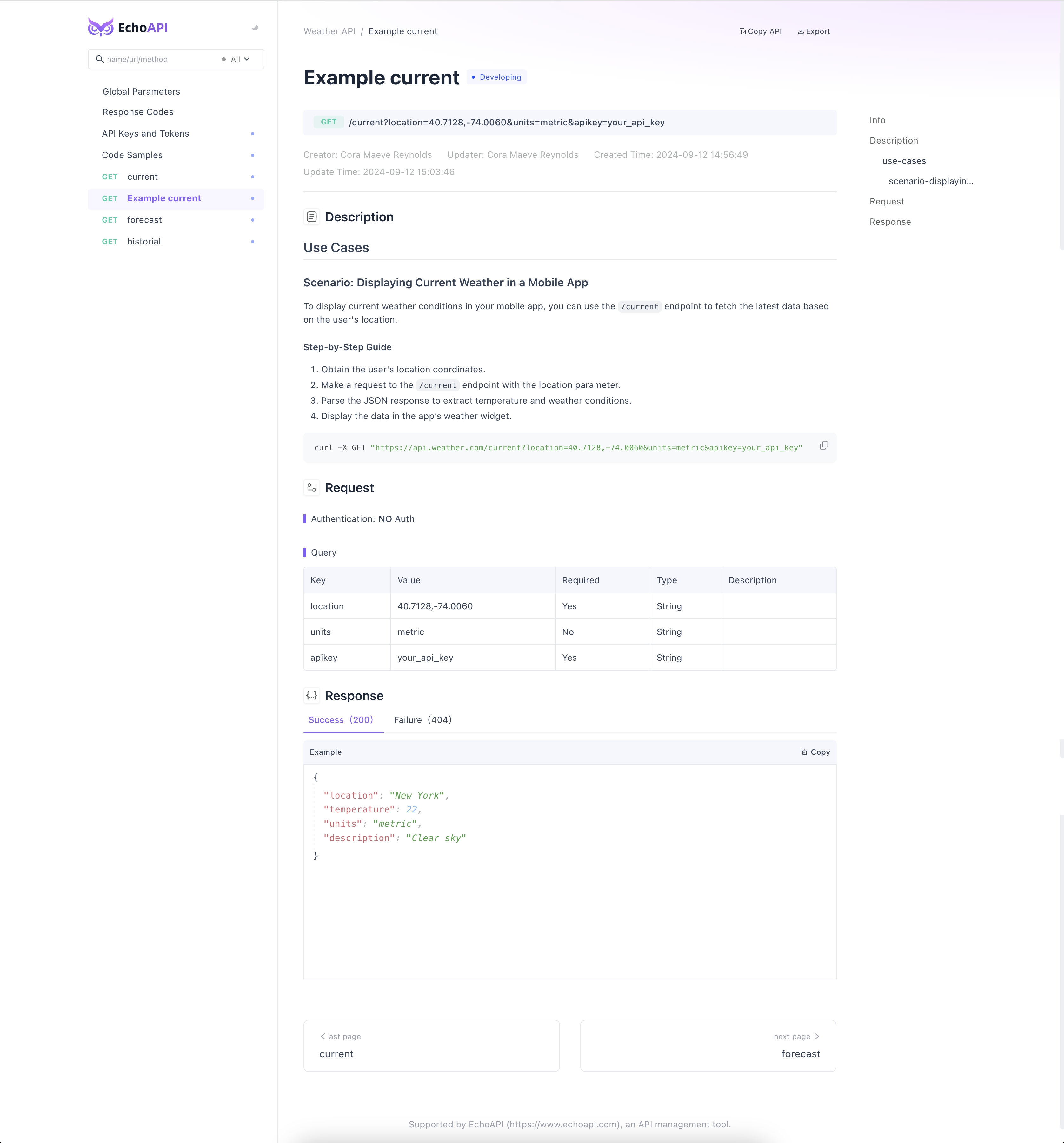
8. Include Error Handling and Troubleshooting Sections
Help users understand what might go wrong and how to resolve it.
- Common Errors: Document common errors and provide troubleshooting tips.
- Error Responses: Clearly define what each error code means and possible solutions.
Example:
## Error Handling
### Common Errors
- **400 Bad Request**: The location parameter is missing or invalid.
- **401 Unauthorized**: Invalid API key.
### Example Error Response
```json
{
"error": {
"code": 401,
"message": "Invalid API key"
}
}
Troubleshooting Tips
- Ensure the API key is active and correctly included in the request.
- Confirm the location parameter is properly formatted.
9. Versioning and Change Logs
API versions and updates can significantly impact users.
- Versioning: Clearly explain how versioning is handled and guide how to specify versions in API requests.
- Change Logs: Maintain detailed change logs to inform users of updates, deprecations, and newly added features.
Example:
## Versioning
To specify API versions, include the version number in the URL:
```sh
curl -X GET "https://api.weather.com/v1/current?location=London&apikey=your_api_key"
Change Logv1.1.0 - 2024-09-12
- Added support for metric and imperial units.
- Improved error messages for missing parameters.
v1.0.0 - 2023-01-01
- Initial release with endpoints for current weather, forecasts, and historical data.
10. Comprehensive Testing and Validation
Ensure the accuracy and completeness of the documentation before it goes live.
- Review Process: Conduct reviews by multiple team members.
- External Feedback: Collect feedback from beta users and the developer community to ensure the documentation meets their needs.
- Automated Testing: Use automation tools to verify all documented endpoints function as described.
11. Accessibility and Localization
Make the documentation accessible and understandable for a global audience.
- Accessibility: Ensure the documentation meets accessibility standards (e.g., WCAG).
- Localization: Consider translating the documentation into multiple languages for a global audience, enhancing accessibility.
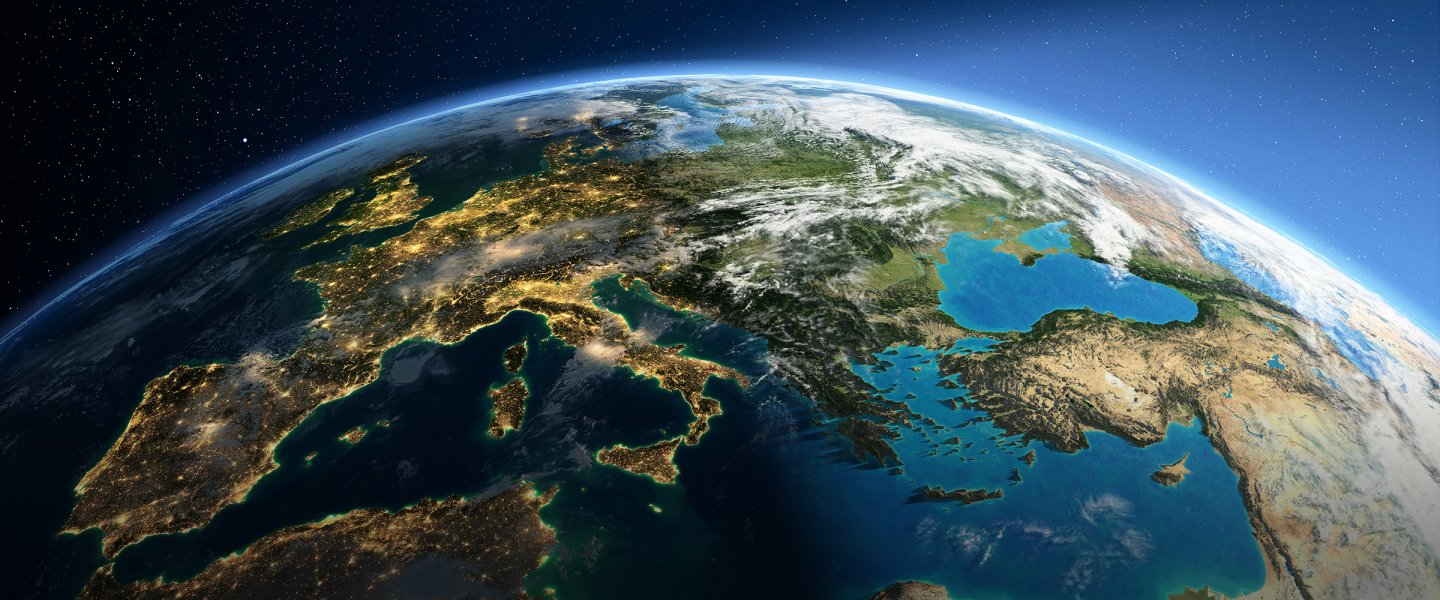
Conclusion
Effective API documentation is crucial for the successful adoption and utilization of APIs. By providing clear, comprehensive, and up-to-date information, you enable developers to integrate your APIs quickly and efficiently, reducing support costs and promoting API adoption. Utilizing appropriate tools, adhering to best practices, and understanding your target audience are essential steps to creating great API documentation. Whether automatically generating documentation with tools like EchoAPI or manually crafting it, well-documented APIs are invaluable resources for developers, enhancing the overall user experience.