Spy Agency 2.0: How to Design Real-Time operations with WebSockets + DTOs
In today's fast-paced tech landscape, designing efficient and secure real-time operations is crucial for applications. This article explores how utilizing WebSockets and Data Transfer Objects (DTOs) can enhance performance and security for your applications.
Previously, we explored why DTOs are the secret weapon for API efficiency and security. Now we are in a different situation, and we're diving into real-time operations with WebSockets + DTOs!
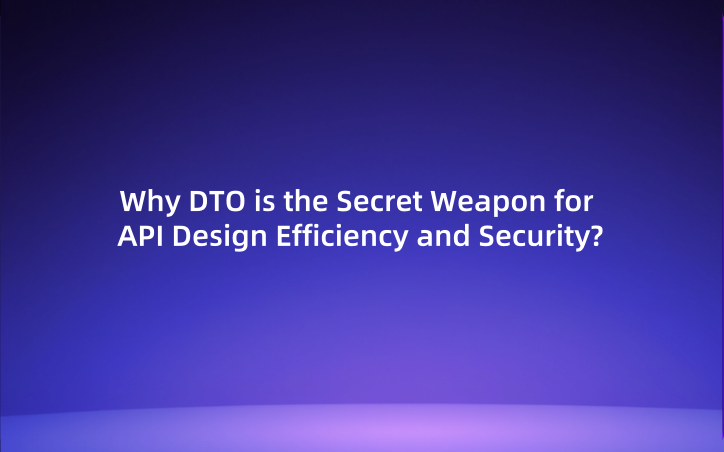
Agent X is deep undercover in a high-stakes mission. Suddenly, HQ updates his objective. If he doesn’t get the update instantly, he could be compromised. Refreshing an API? Too slow. Polling the server? Inefficient.
Mission failure is NOT an option.
1️⃣ Mission: Real-Time Intel with WebSockets + DTOs
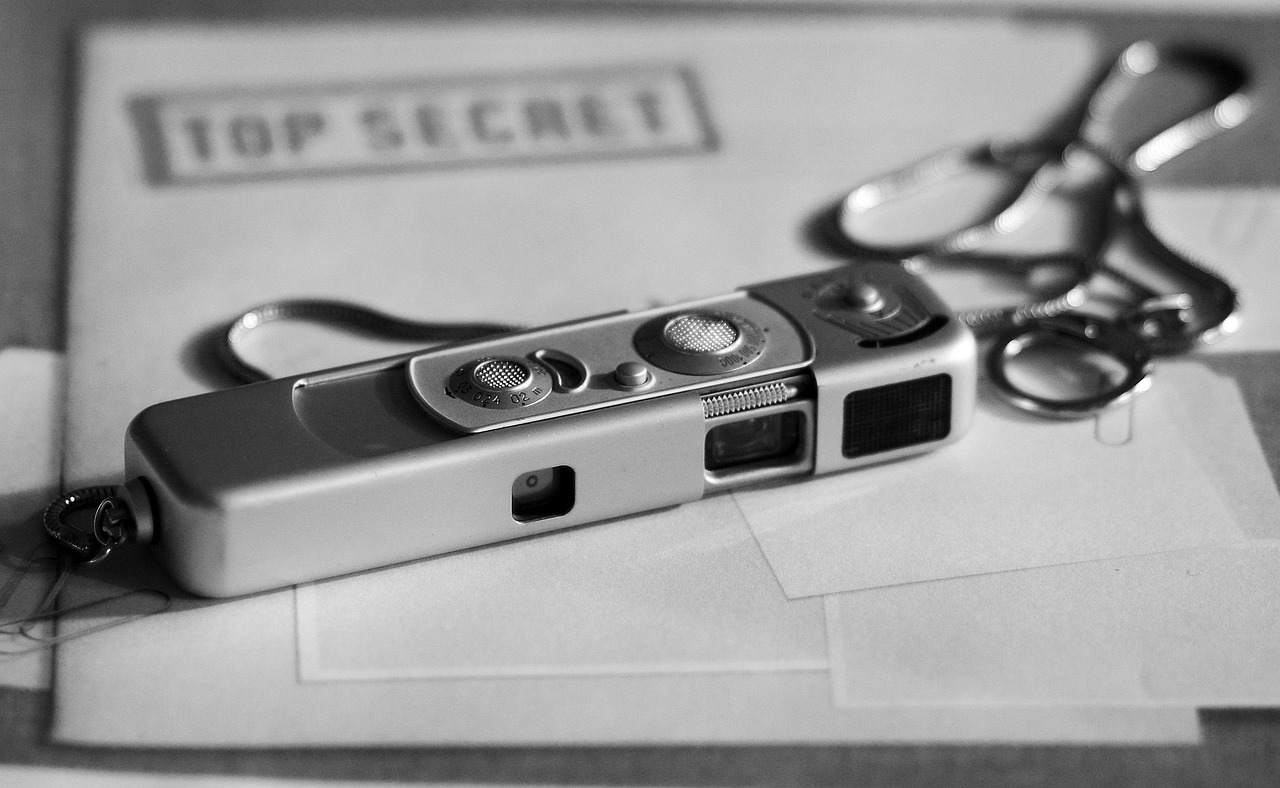
Live Agent Status Updates
- A field agent is deployed in hostile territory.
- HQ updates his mission details in the database.
- WebSocket broadcasts the change to all connected agents.
- Only relevant DTO data is sent (no classified leaks).
- Agent’s UI updates in real-time.
Why WebSockets Beat Traditional API Calls?
Method | Speed | Efficiency | Best For |
---|---|---|---|
REST API | Slow | High server load | One-time data retrieval |
Polling API | Slower | Wastes resources | Legacy systems |
WebSockets | **Instant ** | Efficient & Scalable | Live updates! |
✅ No more waiting for updates!
✅ No unnecessary API calls clogging your server.
✅ Better user experience – data updates instantly!
WebSockets + DTOs ensures instant synchronization between HQ and field agents without overwhelming the server.
2️⃣ Setting Up WebSockets + DTOs
Tech Stack:
✅ Node.js (Express) + Socket.io (WebSockets)
✅ PostgreSQL + Sequelize (Database)
✅ Redis (for scaling WebSockets across multiple servers)
Step 1: Install Dependencies
npm install express socket.io sequelize pg
Step 2: Create WebSocket Server
const express = require("express");
const http = require("http");
const { Server } = require("socket.io");
const app = express();
const server = http.createServer(app);
const io = new Server(server);
io.on("connection", (socket) => {
console.log(`Agent Connected: ${socket.id}`);
socket.on("disconnect", () => {
console.log(`Agent Disconnected: ${socket.id}`);
});
});
server.listen(3000, () => console.log("WebSocket server running on port 3000"));
✅ Now, agents can connect to the WebSocket and receive real-time updates!
3️⃣ Mission Update DTO: Secure & Efficient Data Transfer
DTO (Data Transfer Object) ensures that only necessary mission data is sent to agents, avoiding leaks.
function missionUpdateDTO(mission) {
return {
id: mission.id,
codename: mission.codename,
status: mission.status,
location: mission.location,
};
}
✅ No unnecessary or classified info—only mission-critical data! 🔥
4️⃣ Sending Real-Time Updates on Mission Changes
const { Mission } = require("./models");
app.put("/missions/:id", async (req, res) => {
const { id } = req.params;
const { status, location } = req.body;
const mission = await Mission.findByPk(id);
if (!mission) return res.status(404).json({ error: "Mission not found" });
mission.status = status;
mission.location = location;
await mission.save();
const updateDTO = missionUpdateDTO(mission);
io.emit("mission-update", updateDTO);
res.json(updateDTO);
});
✅ Any update in the database is immediately pushed to all connected agents!
5️⃣ Agents Receiving Mission Updates Instantly!
Step 1: Install WebSocket Client
npm install socket.io-client
Step 2: Connect & Listen for Updates
import { io } from "socket.io-client";
const socket = io("http://localhost:3000");
socket.on("mission-update", (update) => {
console.log("Mission Updated:", update);
// Update UI dynamically
});
✅ Now, agents see mission updates in real-time without refreshing!
6️⃣ Scaling WebSockets with Redis Pub/Sub
For large-scale operations with multiple API servers, we use Redis Pub/Sub to synchronize WebSocket updates.
Install Redis for WebSocket Scaling
npm install ioredis
Redis-Powered WebSocket Broadcasting
const Redis = require("ioredis");
const pub = new Redis();
const sub = new Redis();
sub.subscribe("mission-updates");
sub.on("message", (channel, message) => {
if (channel === "mission-updates") {
io.emit("mission-update", JSON.parse(message));
}
});
app.put("/missions/:id", async (req, res) => {
const { id } = req.params;
const { status, location } = req.body;
const mission = await Mission.findByPk(id);
if (!mission) return res.status(404).json({ error: "Mission not found" });
mission.status = status;
mission.location = location;
await mission.save();
const updateDTO = missionUpdateDTO(mission);
await pub.publish("mission-updates", JSON.stringify(updateDTO));
res.json(updateDTO);
});
✅ Now, updates are synchronized across all WebSocket servers!
7️⃣ How EchoAPI Helps
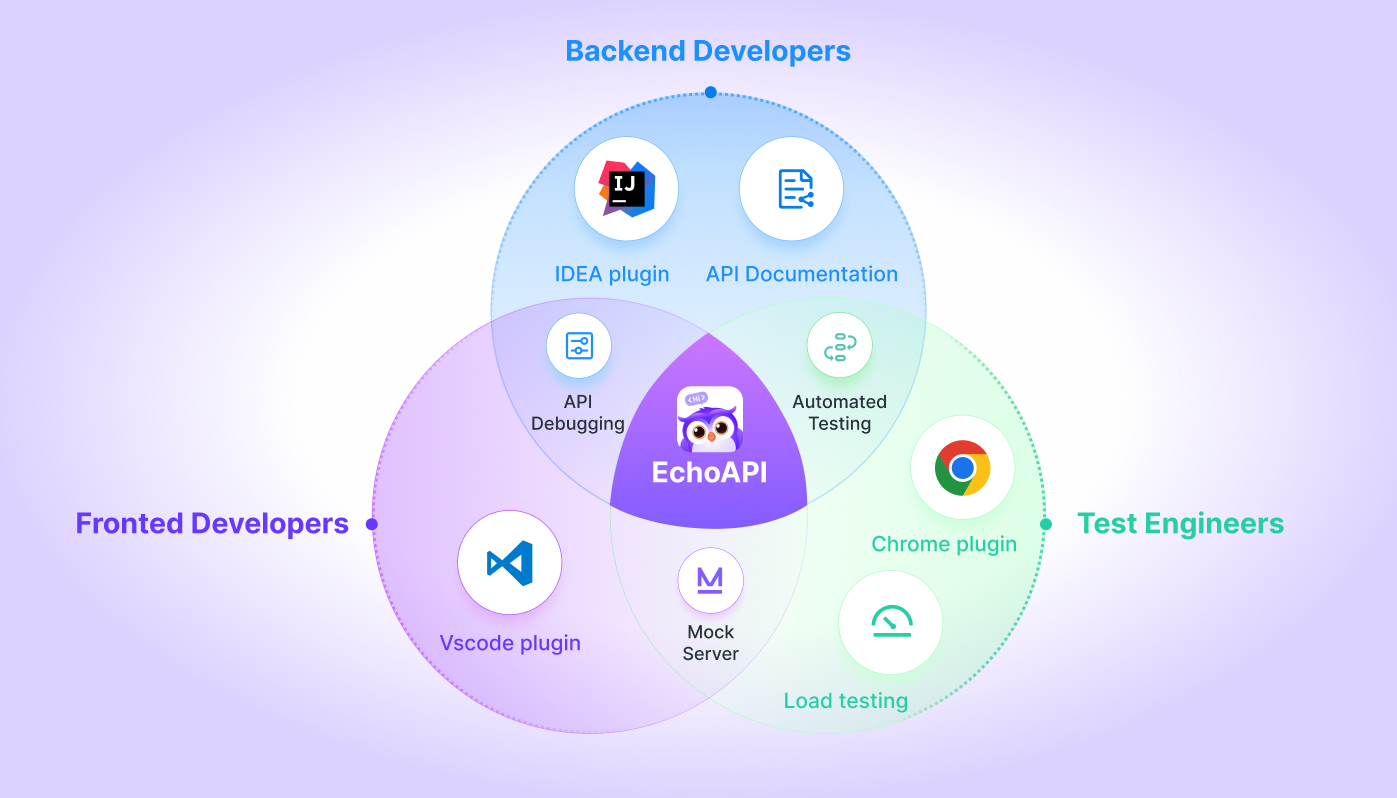
EchoAPI is a powerful tool that allows you to test, debug, and monitor WebSocket communications in real-time.
Benefits of EchoAPI:
✅ Simulate WebSocket connections to test live updates.
✅ Monitor API responses without writing extra logging code.
✅ Easily debug API
✅ You can verify API updates effortlessly!
Final Debrief: Why WebSockets + DTOs?
Feature | Benefit |
---|---|
Instant updates | Agents get real-time mission updates |
No polling required | Saves server resources |
DTOs secure data | No leaks—only necessary info is sent |
Scales with Redis | Works across multiple servers |
Your Spy Agency just got a major upgrade!
No more outdated mission details. No more unnecessary API calls. Just pure real-time intelligence.
Ready to deploy? Try EchoAPI today!