The Essential Guide to Socket.IO: Enhancing Real-Time Communication in Web Development
Socket.IO revolutionizes real-time communication in web applications, offering developers a robust framework for building interactive features.
As a developer, understanding the intricacies of Socket.IO is not just beneficial; it’s necessary. This technology has transformed the way modern web applications communicate in real time, making it a critical tool in the developer's arsenal. In this article, we'll explore what Socket.IO is, its key features, common uses, practical implementations, and how you can effectively debug Socket.IO using EchoAPI.
What is Socket.IO?
Socket.IO is a powerful JavaScript library that enables real-time, bidirectional and event-based communication between web clients and servers. It's built on top of the WebSocket protocol but provides additional features including reliability, automatic reconnection, and the ability to broadcast to multiple sockets.
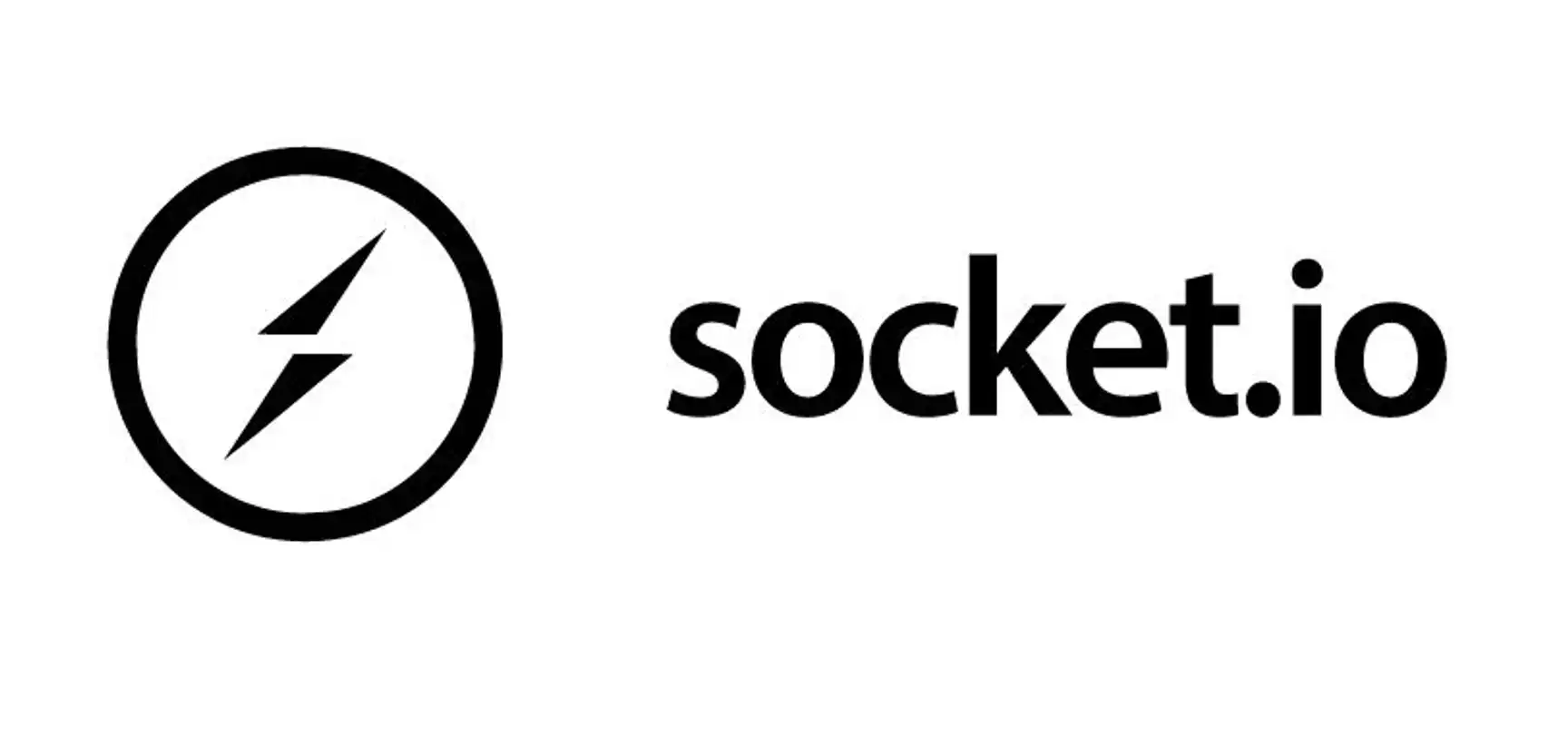
Key Features of Socket.IO
Socket.IO stands out due to its robust set of features which include:
- Real-time bidirectional event-based communication: Allows both the server and the client to initiate communication, sending messages at will.
- Fallback to HTTP long polling: Ensures reliability by using WebSocket where available and HTTP long polling as a fallback.
- Automatic reconnection support: Enhances user experience by automatically attempting to reconnect following a dropped connection.
- Multiplexing support: Supports multiple namespaces and rooms within a single connection, enabling more structured and complex data exchange.
- Built-in binary support: Transmits binary data seamlessly, which is essential for applications dealing with multimedia content.
Common Use Cases for Socket.IO
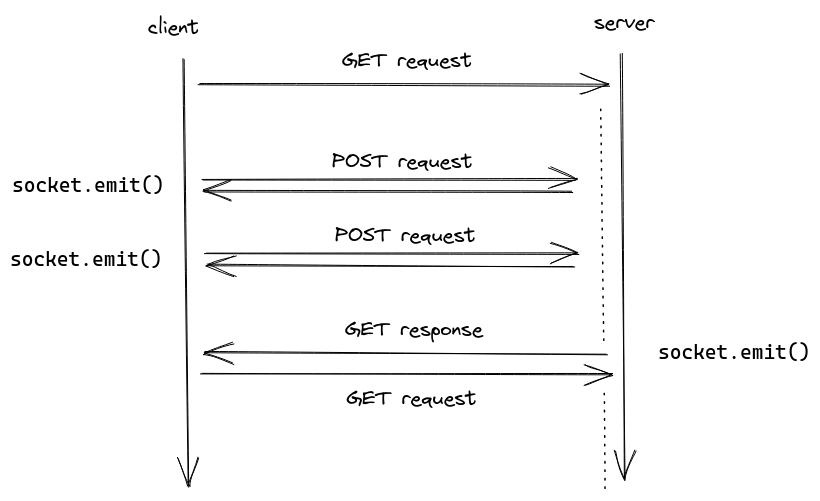
Socket.IO is versatile, suitable for a variety of real-time applications, such as:
- Chat applications: Enables users to send and receive messages instantly without needing to refresh the web page.
- Live notifications: Sends updates to clients instantly, useful in social media apps, news feeds, and trading platforms.
- Collaborative tools: Allows multiple users to edit documents or code in real-time, enhancing productivity tools.
- Real-time analytics: Streams data to clients in real-time, perfect for dashboards monitoring user activity or financial transactions.
Practical Implementation: Building an Advanced Real-Time Chat Application Using Socket.IO
Scenario
Imagine creating an advanced real-time chat application, comparable to industry standards like Slack or Discord, which supports features such as private messaging, groups, user authentication, and data encryption. This app will provide an engaging user interface where participants can join different chat rooms or create private conversations.
Benefits of Using Socket.IO
- Real-time Communication: Delivers and displays messages without any perceptible delay.
- Flexibility: Seamlessly integrates with various databases and authentication services.
- Scalability: Handles numerous simultaneous connections, essential for a growing user base.
Technical Implementation
Let’s detail the components of this more complex chat application using Socket.IO.
Server Setup
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
app.use(cors());
app.use(bodyParser.json());
const server = http.createServer(app);
const io = socketIo(server, {
cors: {
origin: "*",
methods: ["GET", "POST"]
}
});
io.on('connection', (socket) => {
console.log(`New user connected: ${socket.id}`);
socket.on('joinRoom', ({username, room}) => {
socket.join(room);
socket.to(room).emit('message', {user: 'Admin', text: `${username} has joined the room.`});
});
socket.on('sendMessage', (message, room) => {
io.to(room).emit('message', {user: message.sender, text: message.text, time: new Date().toISOString()});
});
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
Key Functionalities
- User Authentication and Rooms: Integrates user validation and manages chat rooms, enabling private and group messaging options.
- Message Broadcasting: Uses rooms for broadcasting messages only to users who are part of a particular conversation.
- Error Handling and Feedback: Sends administrative messages for user activities like joining or leaving a chat room.
Enhancements with Socket.IO
To improve the user experience and application efficiency, consider implementing:
- Read receipts, to inform senders when the receiver has seen the message.
- Typing indicators, to show when someone is typing a response in the chat.
- Message encryption, to ensure that all communications are secure and private.
Advanced Error Handling and Security
- Implement automatic retries for sending messages when the server detects a drop in the connection.
- Use SSL/TLS encryption over connections to enhance security.
- Ensure all data is validated both client-side and server-side to prevent common security threats like XSS (Cross-Site Scripting).
This example sets up an initial framework for an advanced chat application, demonstrating Socket.IO’s capabilities in handling complex functionalities such as real-time communication, error handling, and user room management. Moreover, this structure paves the way for incorporating additional features that enhance scalability and security. By following this guide, developers can engineer robust applications that not only meet but exceed modern expectations.
Advanced Error Handling and Security
In a complex chat application, dealing with errors and ensuring the security of the communications is pivotal. Here are specific strategies you could implement:
1. Automatic retries for message sending: Useful particularly in fluctuating network conditions.
- Implement a queue system on the client side to store messages temporarily if they fail to send. Retry the sending process a fixed number of times before notifying the user of a failure.
- This ensures that short disconnections or server issues do not disrupt the user experience significantly.
2. SSL/TLS encryption over connections: Crucial for protecting data in transit.
- By deploying SSL/TLS, all data transmitted between clients and servers is encrypted. This prevents attackers from intercepting sensitive data and keeps the communication secure.
- It is advisable to use certificates from a recognized certificate authority to avoid issues with untrusted notifications.
3. Validation of data both client-side and server-side: Essential to protect your application from common security threats like XSS and injection attacks.
- Client-side validation can provide quick feedback to users but should not be relied upon for security.
- Server-side validation acts as a second layer of defense, ensuring that tainted data does not affect the backend processes or get stored in the database.
Incorporating these advanced security measures and error handling strategies will significantly boost the robustness and reliability of a real-time chat application built using Socket.IO. Each step ensures an extra layer of protection against possible failures or security breaches, leading to a trustworthy platform for users. Implementing such comprehensive features from the outset sets a strong foundation for scalability and user trust in long-term application growth.
EchoAPI: A Recommended API Debugging Tool
EchoAPI is a versatile API debugging tool that supports multiple protocols including HTTP, WebSocket, TCP, SSE, and GraphQL. It’s particularly useful for debugging real-time applications that use Socket.IO.
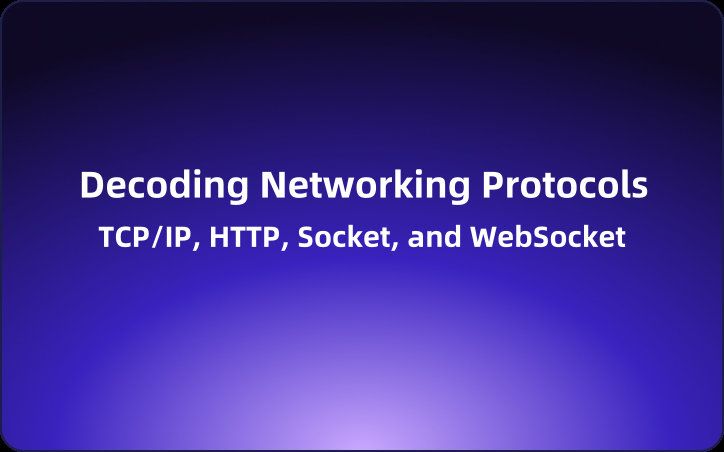
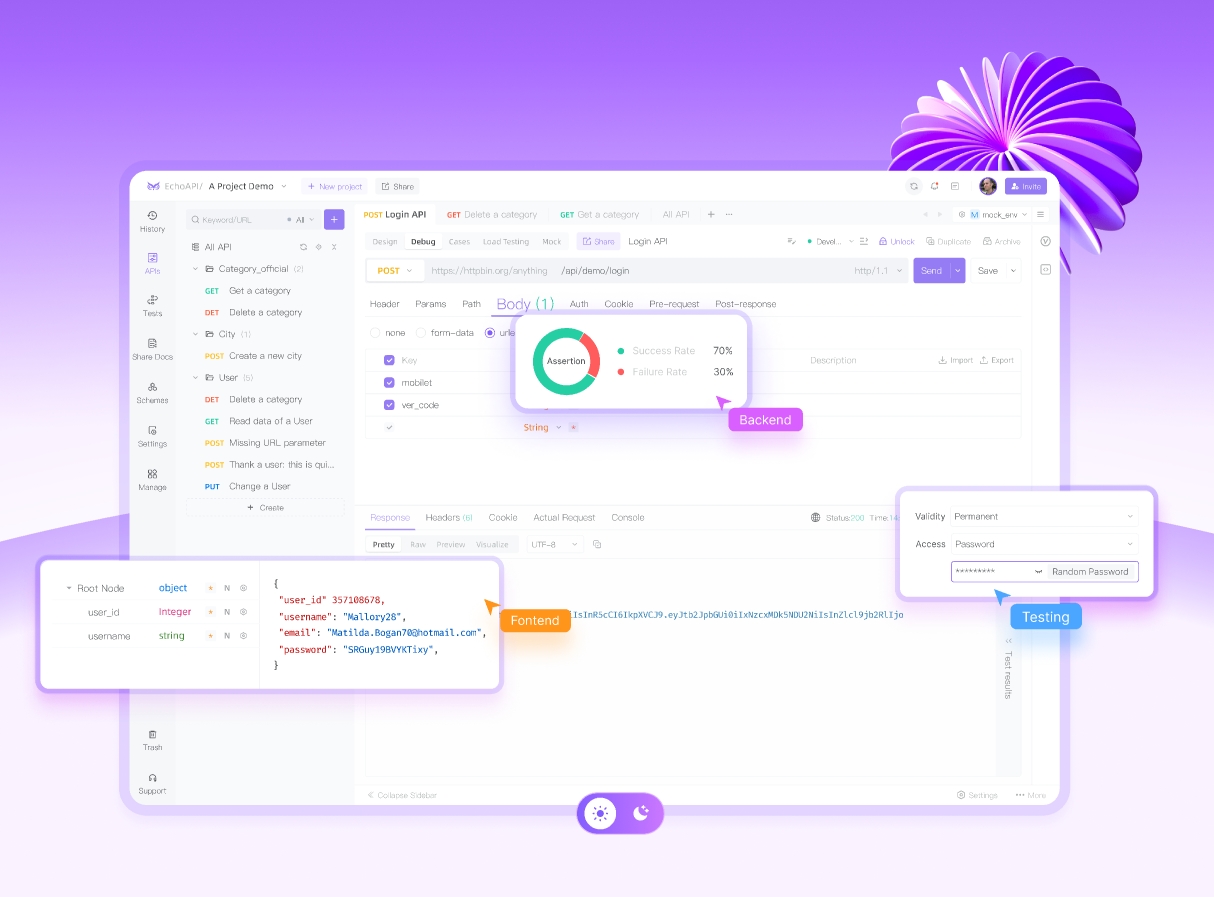
Debugging Socket.IO with EchoAPI
Step 1: Create a New Request
Open EchoAPI and click the “+” button in the left menu to create a new request.
In the request type dropdown menu, select Socket.IO.
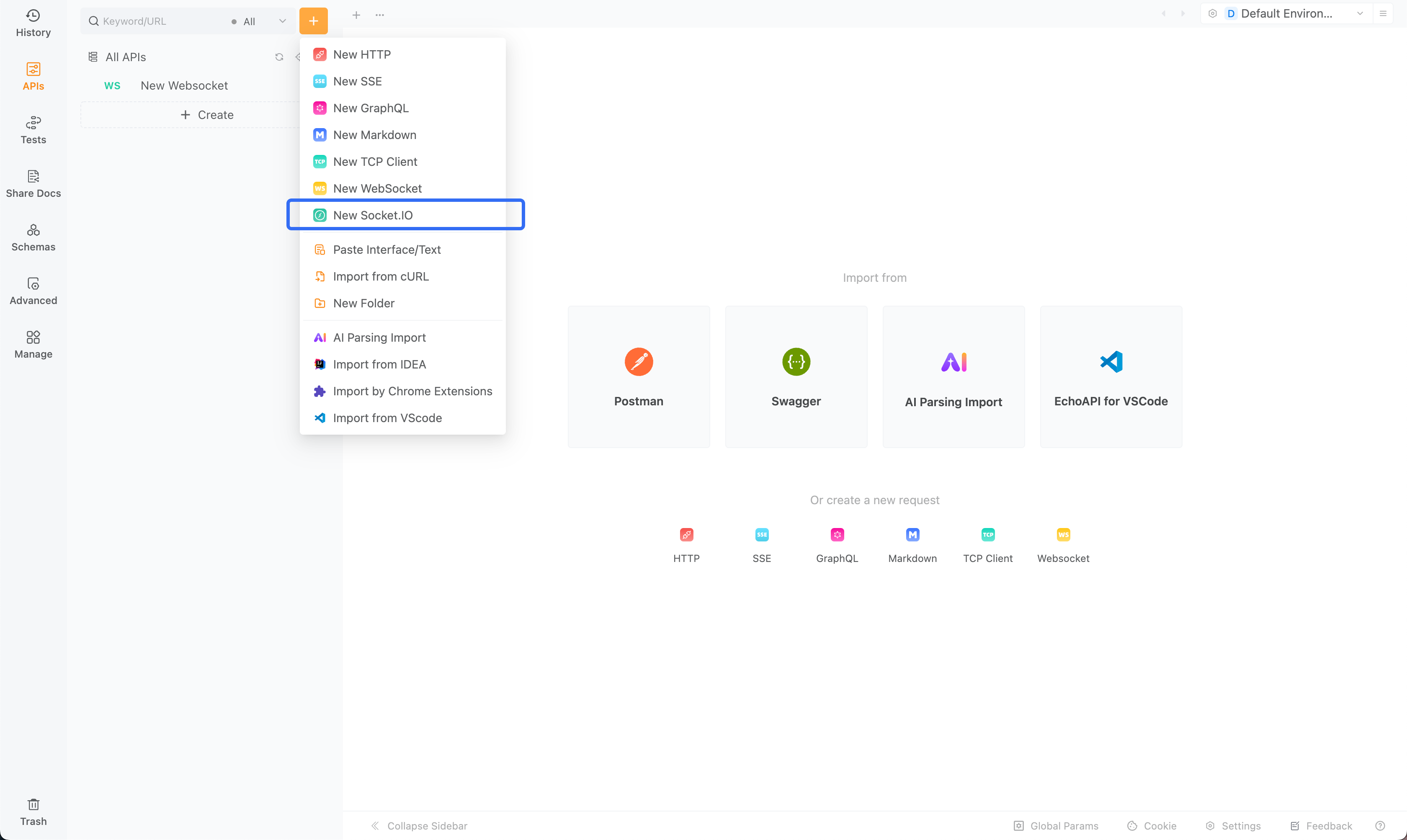
Step 2: Configure Socket.IO Connection
Ensure you are on the Debug page.
In the URL box, enter the Socket.IO server URL.
You can also configure request headers or request parameters at the indicated location below.
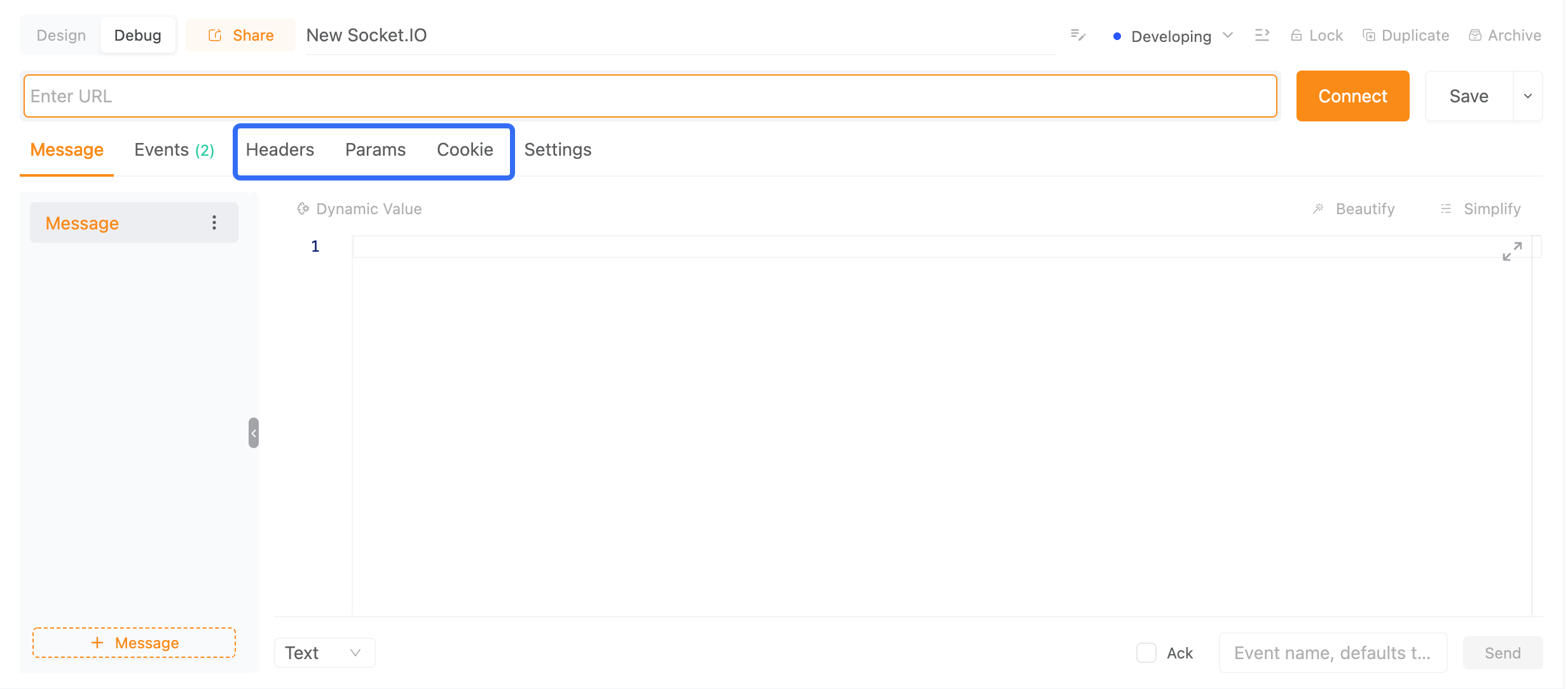
You can also set timeouts and other configurations in the settings tab.
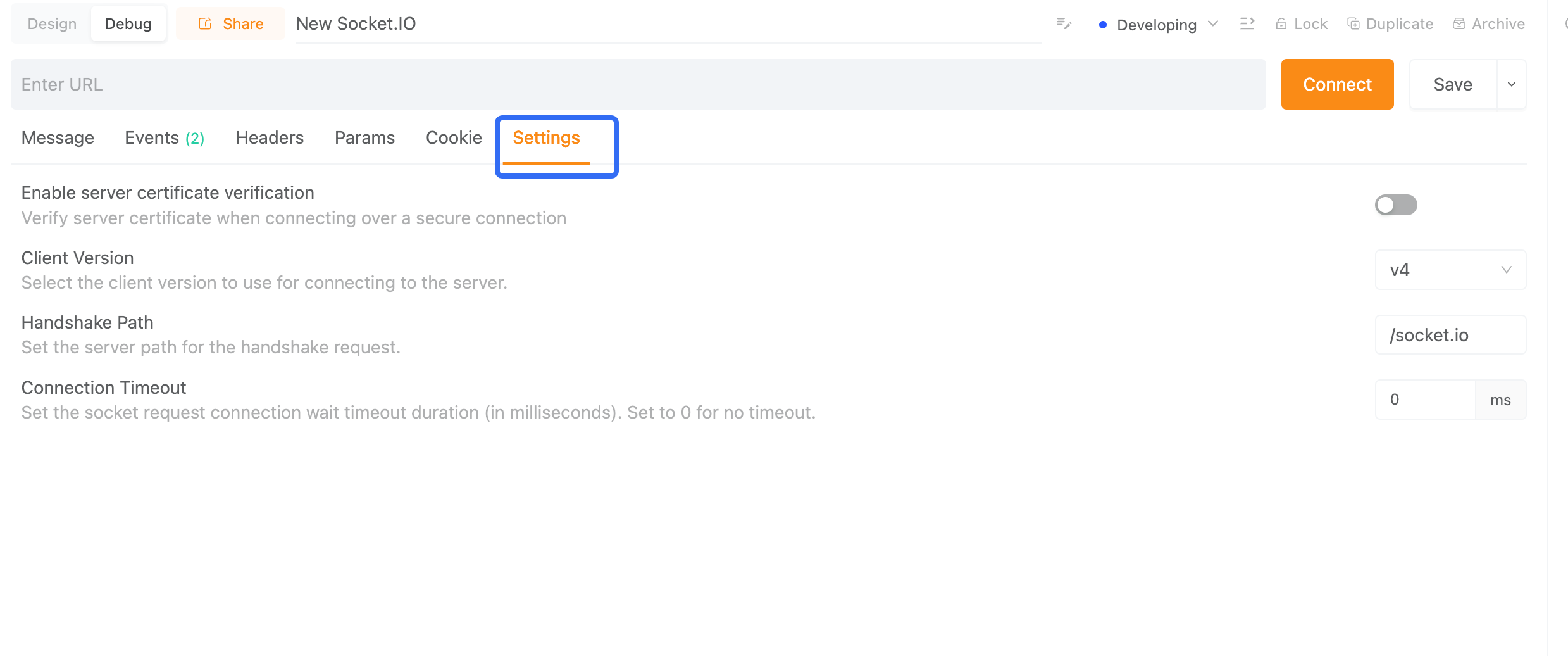
Click the “Connect” button to establish a connection.
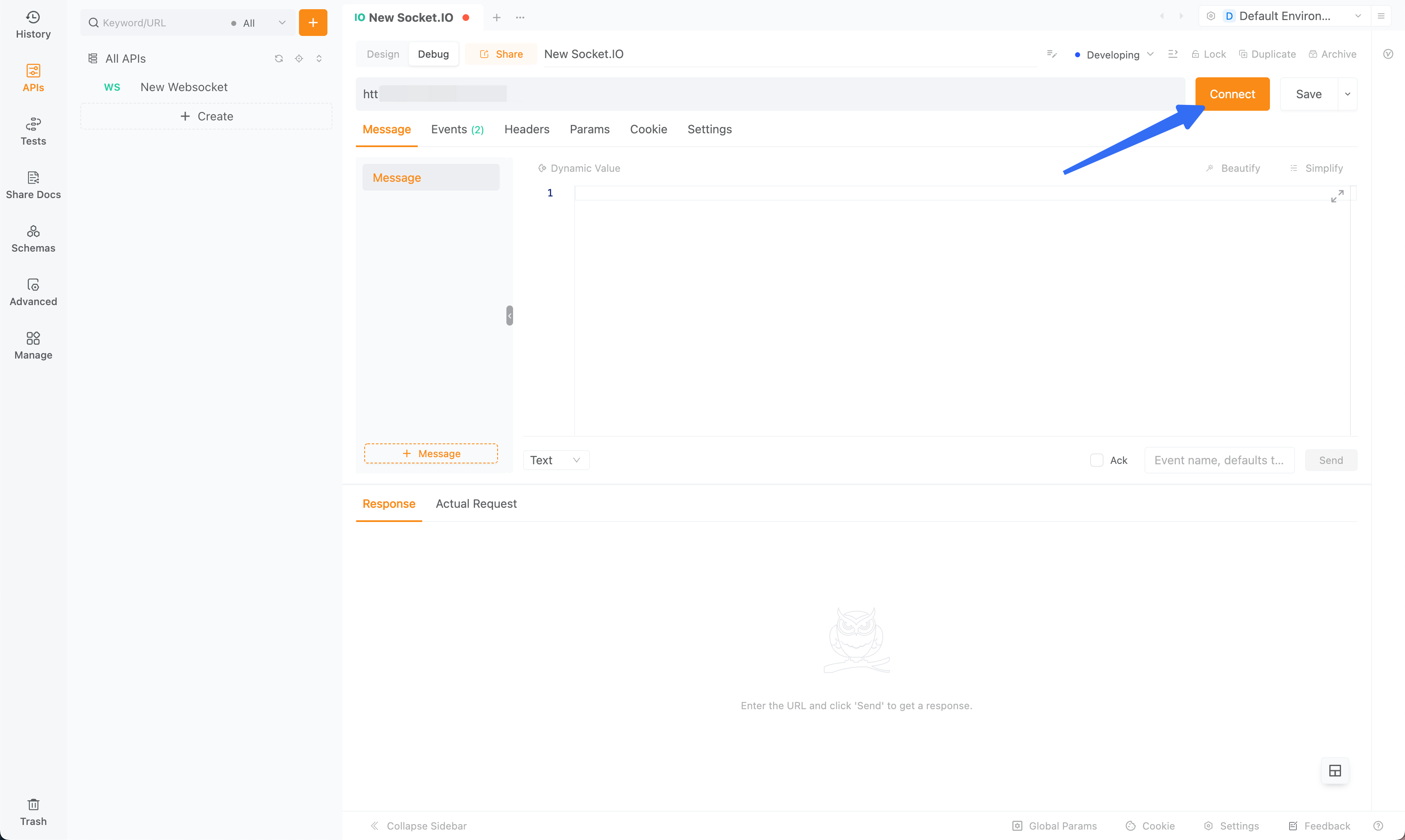
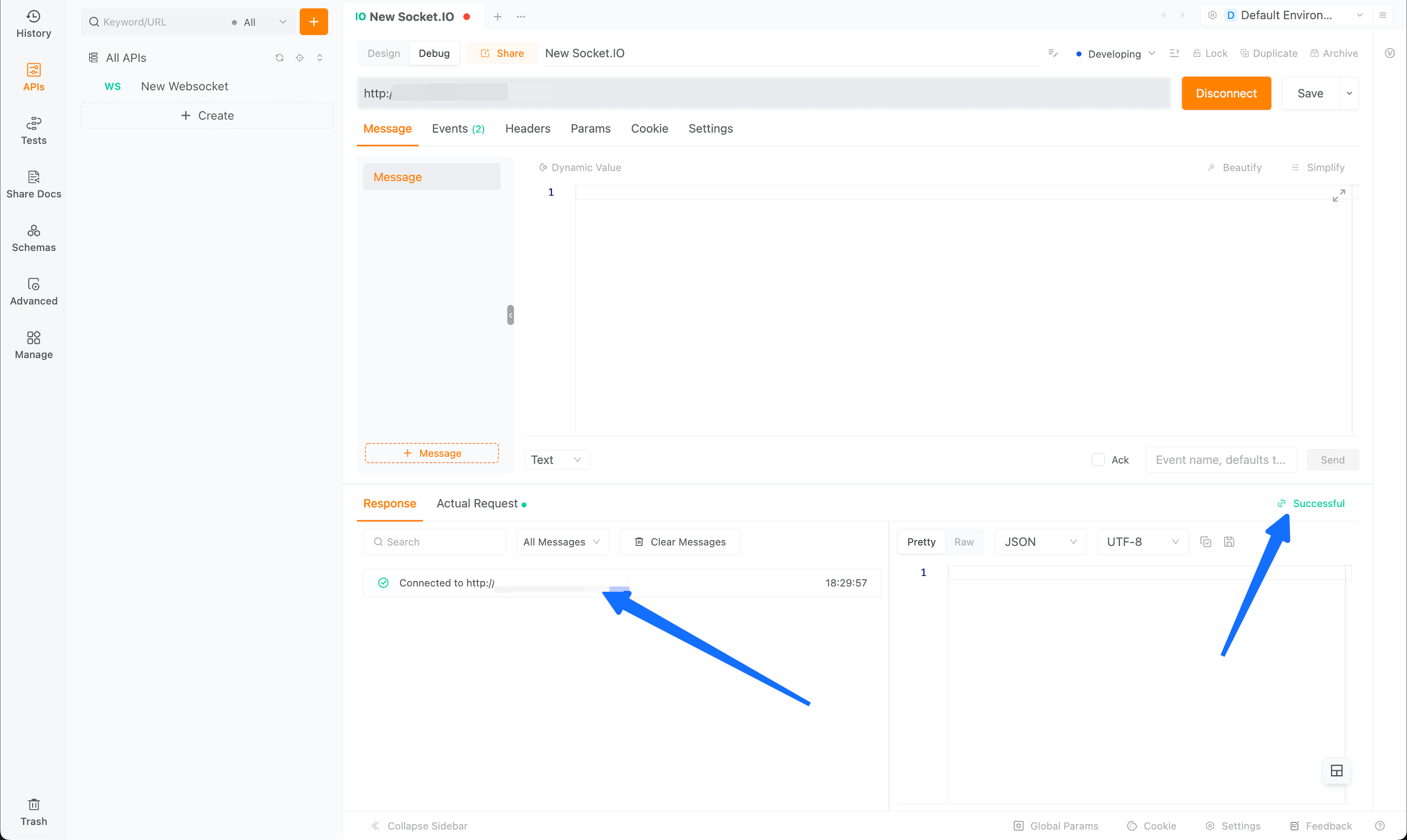
Step 3: Event Listening
Add the event name and turn on listening. When the server returns that event, you will be able to see the event message in the response area. If you turn it off, you will not receive messages.
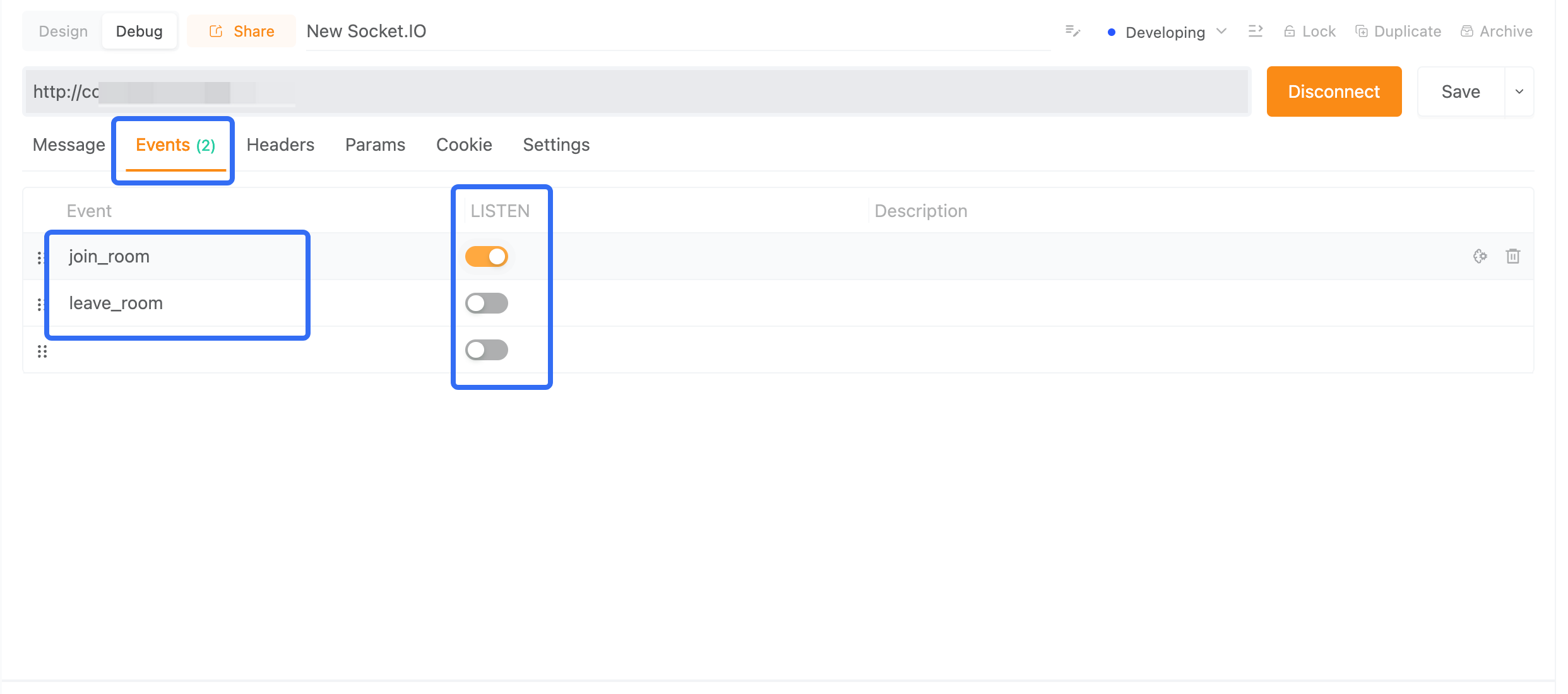
Step 4: Sending Messages
Once connected, you can send Socket.IO messages.
Enter the message content in the input box, select the content type, and optionally enter an event name; if not provided, it will default to message
.
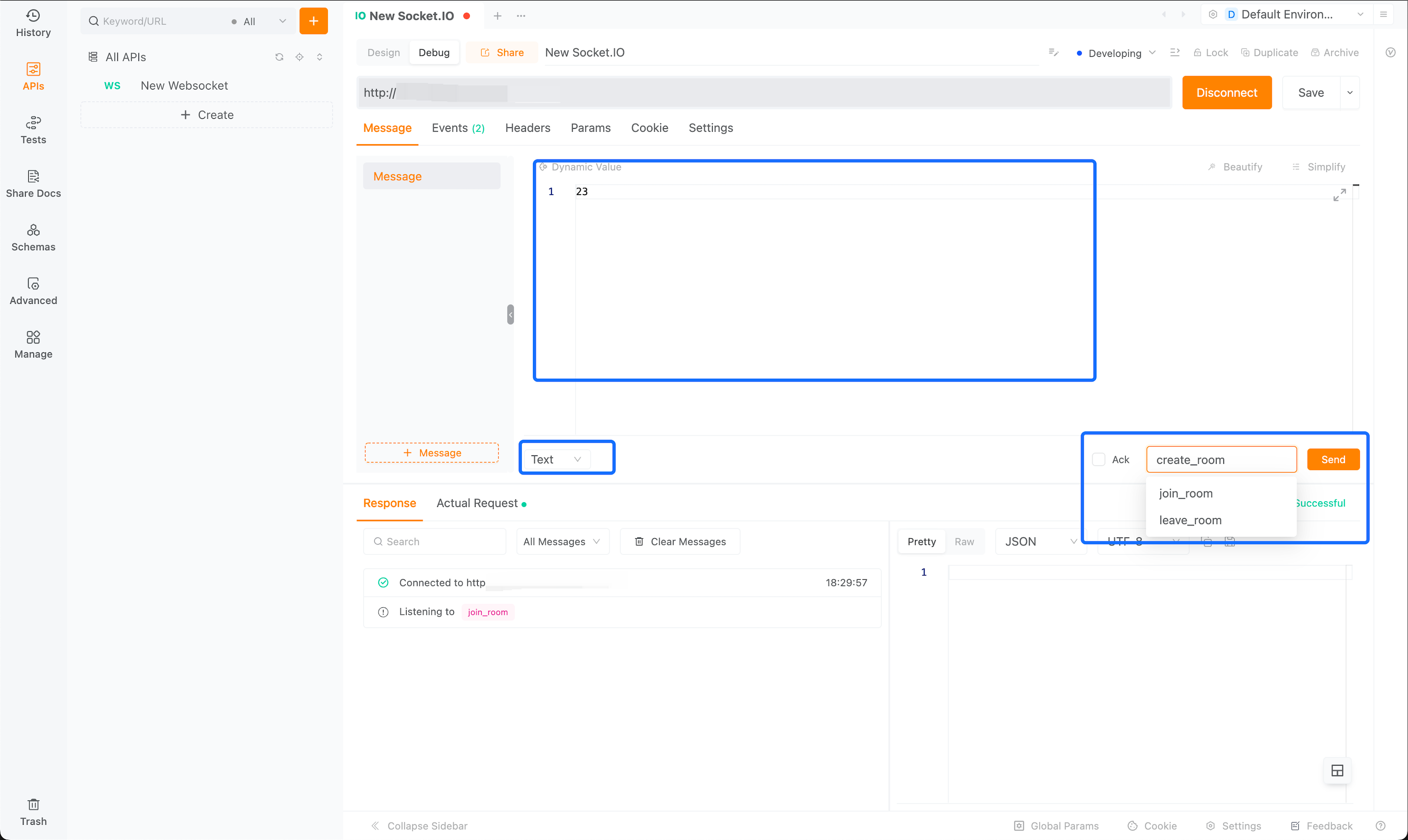
Tips
On the left, you can quickly create multiple messages for debugging without having to create multiple Socket.IO connections for different message types. In the same Socket.IO connection, you can add various types of message requests for archiving, allowing for quicker debugging later on. This flexibility makes debugging more efficient and convenient!
You can also double-click the name to rename it.
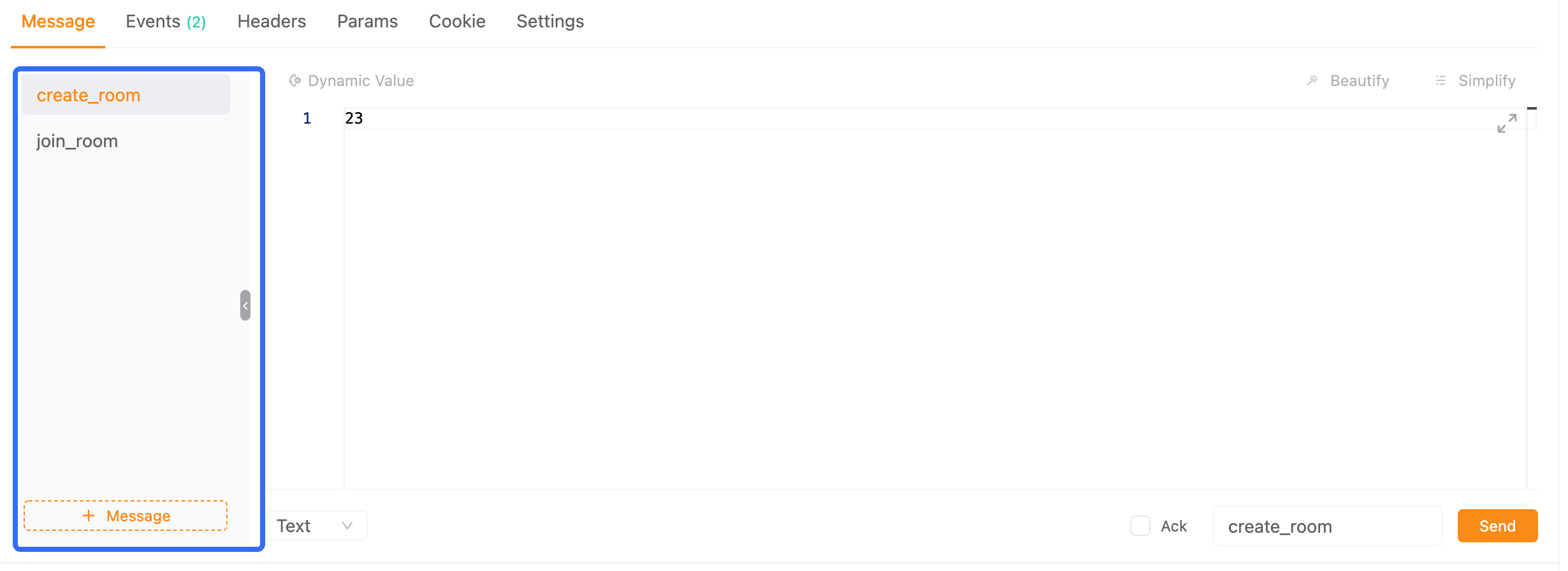
Step 5: Receiving Messages
After successfully sending a message and if you have opened event listening, you can receive messages returned by the server.
All received messages will be displayed in the EchoAPI “Response” section.
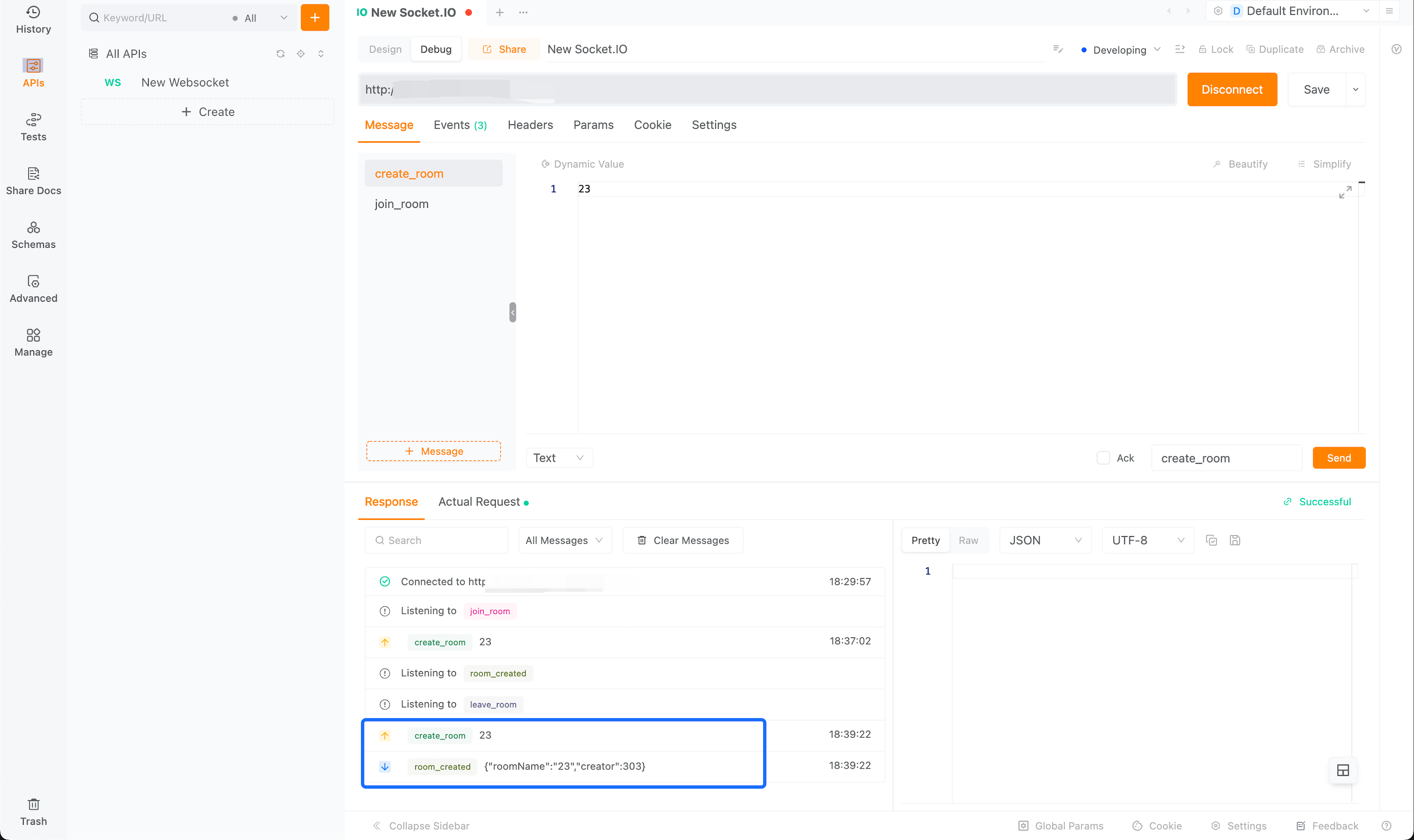
Step 6: Save Received Messages as Response
In the response area, you can quickly save the current response content as a response example for a particular message, which will help generate a more complete API documentation later.
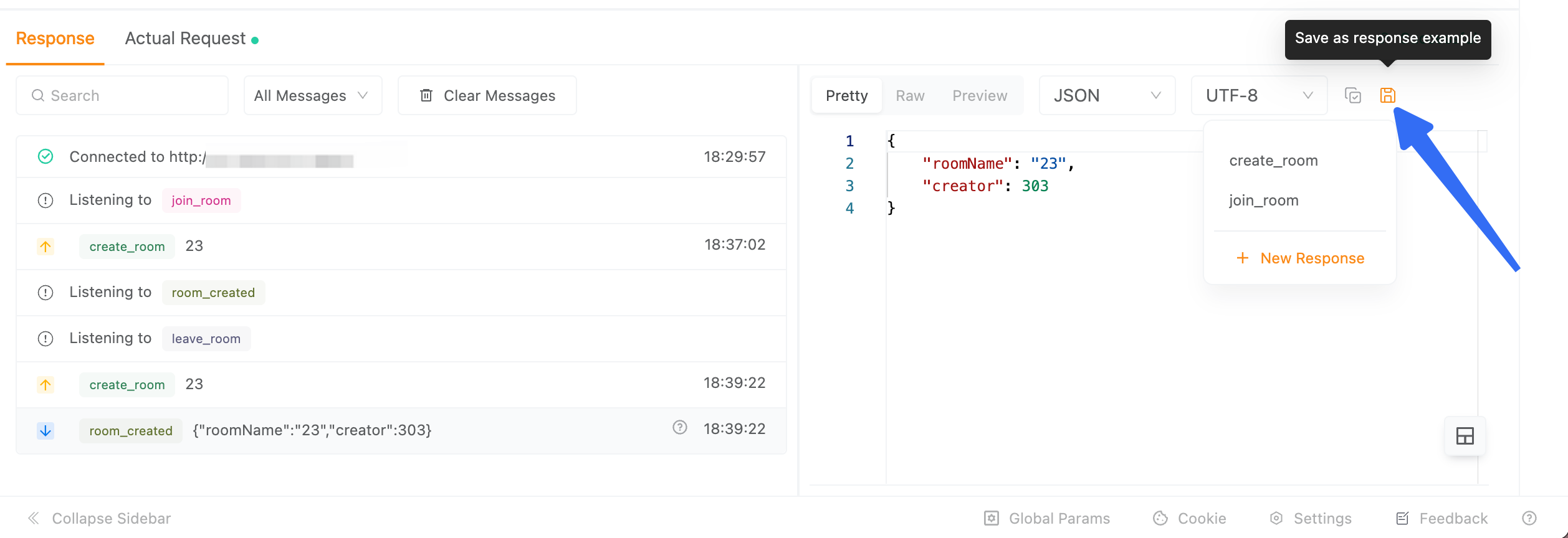
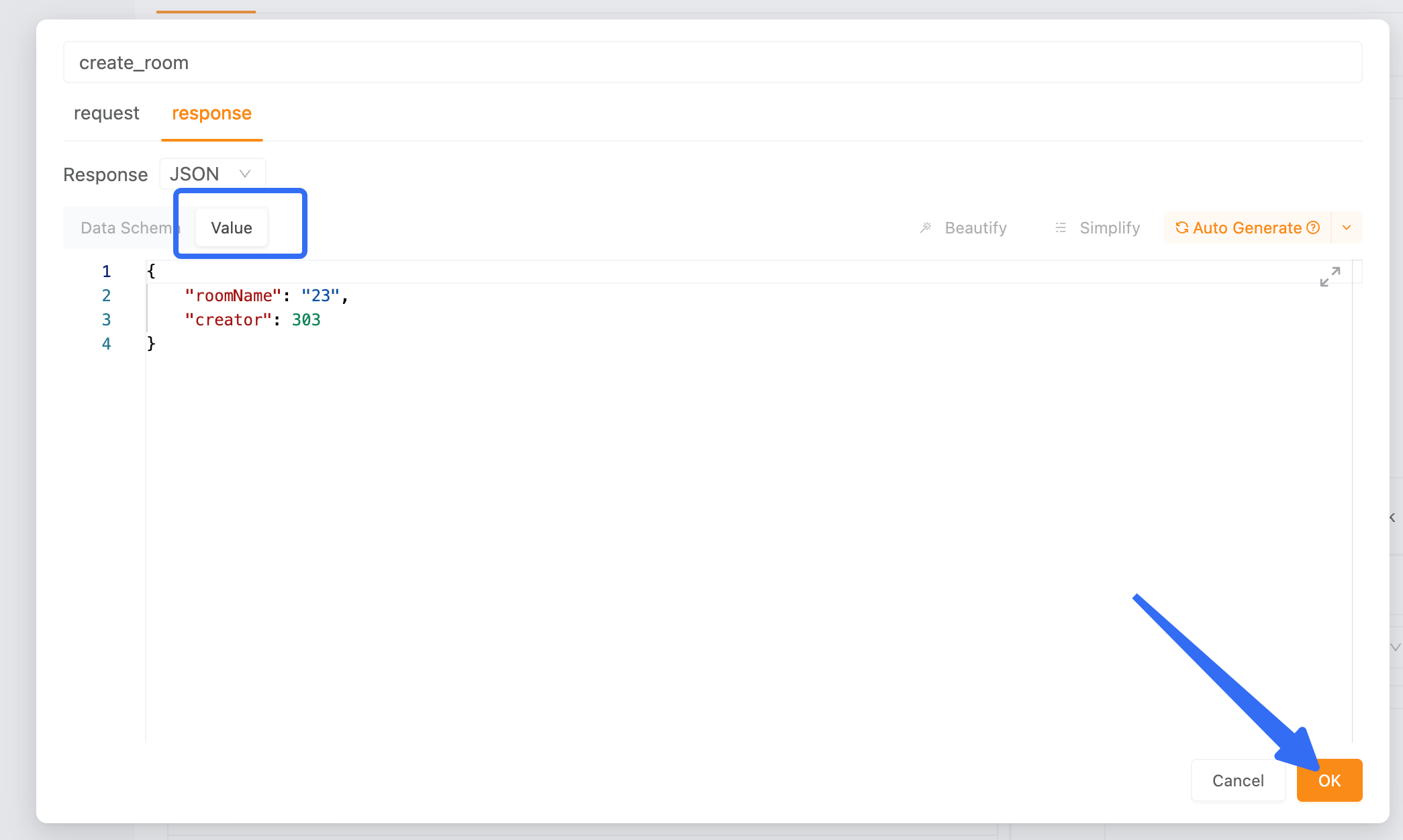
The generated documentation after saving looks like this. You can check the specific details at Socket.IO Design/Documentation.
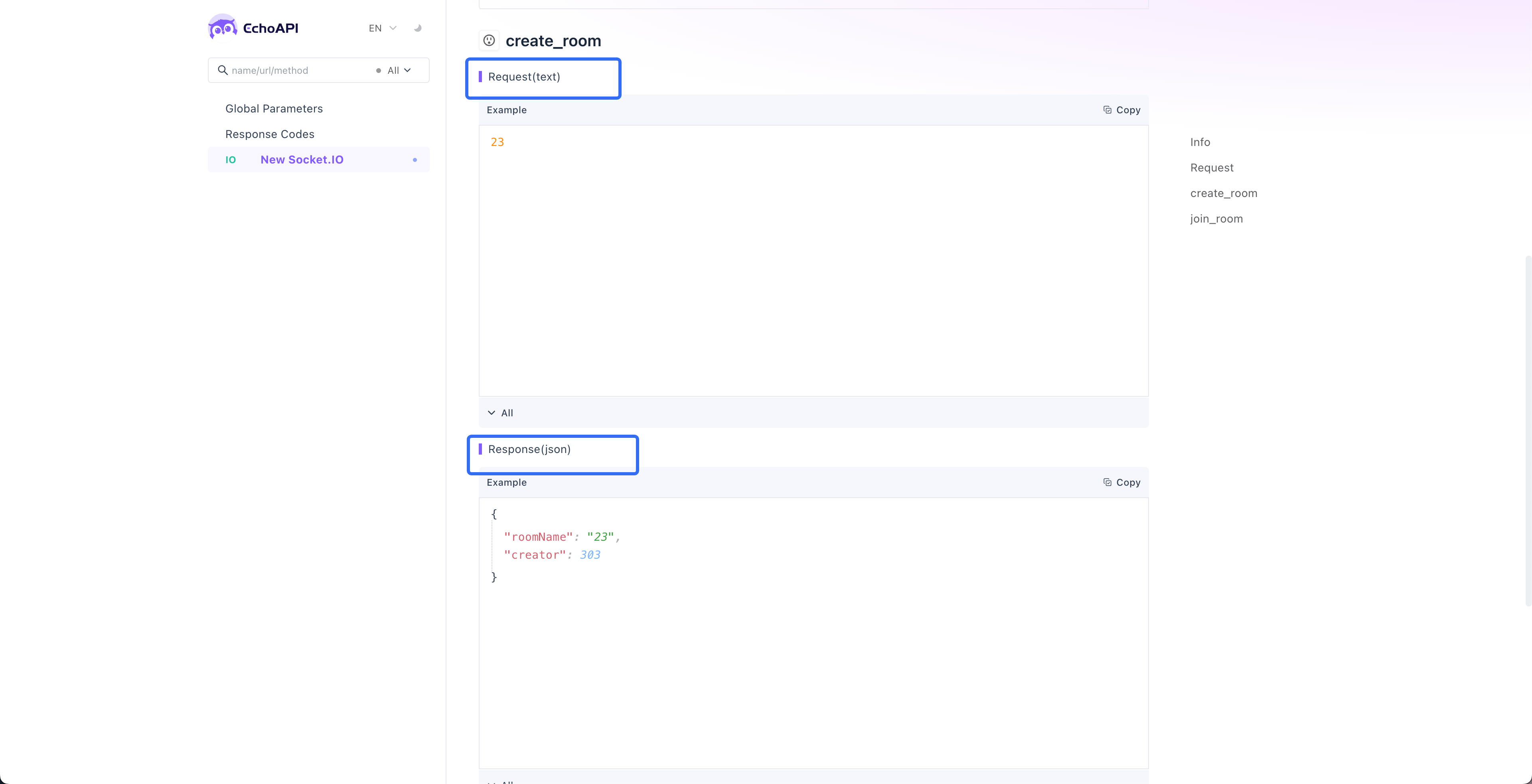
The Necessity of Socket.IO
In the world of modern web development, real-time interaction is not just a luxury; it's often a requirement. Socket.IO provides developers with the tools necessary to build these interactive experiences efficiently and effectively.
Conclusion
Socket.IO is an indispensable part of creating interactive, real-time web applications. Its comprehensive features and adaptability make it ideal for a wide range of applications, from simple chats to complex collaborative solutions. By leveraging tools like EchoAPI, developers can streamline their workflow, ensuring their implementations are both robust and reliable. Understanding and utilizing Socket.IO effectively is not just an enhancement to your skillset—it is essential to staying competitive in the ever-evolving field of web development.