The Essential Guide to WebSockets for Developers
WebSocket is an essential technology in the development of modern web applications. It enables real-time data exchange and seamless communication between users and servers. This protocol is widely used in various fields such as online gaming and chat applications.
Understanding WebSockets is crucial for developers aiming to build real-time, interactive applications. This protocol facilitates not only seamless interactions between user and server but also supports the real-time data exchange crucial for today's dynamic web environments.
What is WebSocket?
WebSocket is a communication protocol that provides a bi-directional, full-duplex communication channel over a single, long-lived connection. It was designed to be implemented in web browsers and web servers but can be used by any client or server application. The WebSocket protocol makes more interactive experiences possible, directly through the basic structure of the HTTP connection.
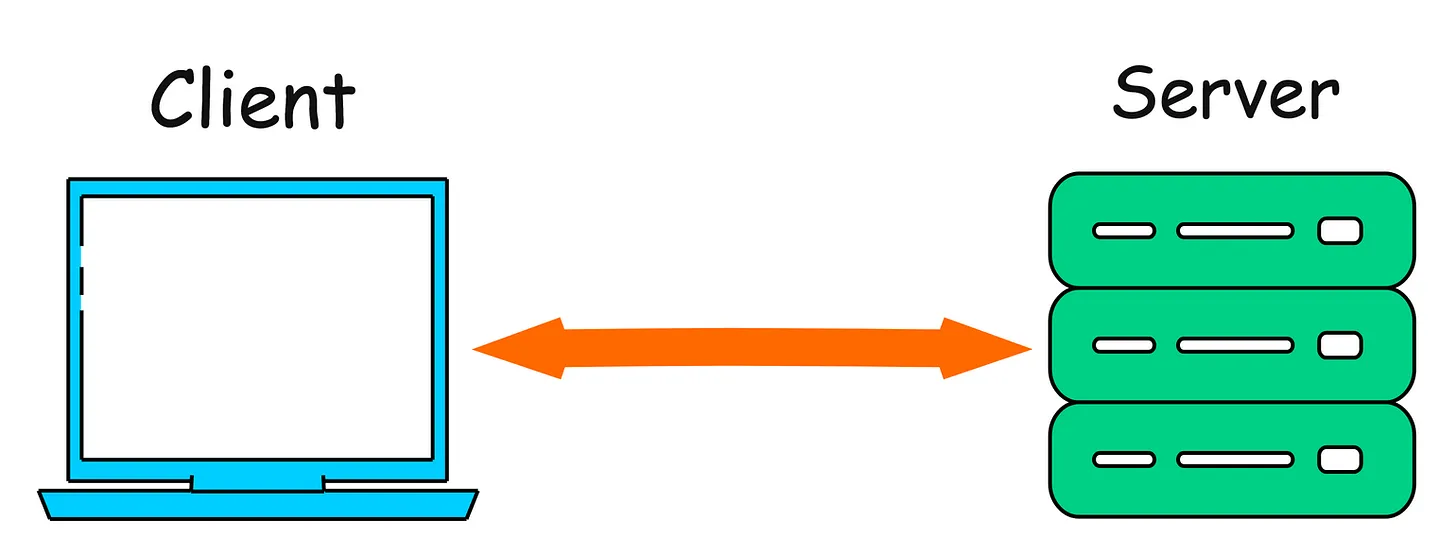
Key Features of WebSocket
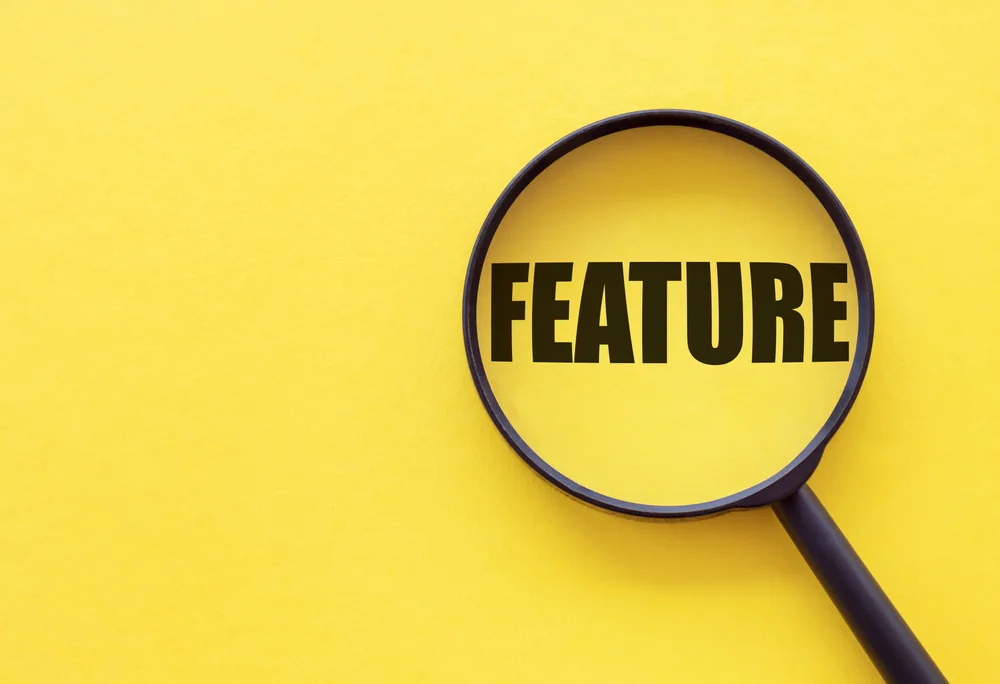
- Bi-directional: Unlike the traditional request/response paradigm, WebSocket provides a two-way communication avenue between the client and server.
- Full Duplex: It allows messages to be sent and received simultaneously.
- Low Overhead: WebSockets maintain a persistent connection, reducing both latency and overhead by minimizing the data exchanged between the client and server.
- Backward Compatibility: It is designed to work over HTTP ports 80 and 443 as well as to support HTTP proxies and intermediaries.
Common Use Cases
WebSockets are typically used in applications that require real-time data flows such as:
- Online gaming:
WebSockets are pivotal in online gaming environments where player actions must be immediately reflected on all other players' devices to ensure a synchronized and fair gameplay experience. This protocol supports rapid message exchanges, essential for multiplayer games like MMORPGs (Massively Multiplayer Online Role-Playing Games) and real-time strategy games. For instance, when a player moves or attacks, the action is broadcasted without delay to all participants, maintaining the game's pace and integrity. - Chat applications
In chat applications, WebSockets enable users to send and receive messages with minimal latency. Popular apps like WhatsApp and Facebook Messenger rely on WebSocket technology to deliver messages instantaneously, significantly improving the flow of conversation. This technology also supports features such as "user is typing" indicators and real-time read receipts, enhancing the user's interactive experience. - Live sports updates
For live updates during sports events, WebSockets ensure that fans receive real-time information such as scores, player stats, and in-game events directly on their devices. This continuous stream of updated data allows fans to feel more engaged and connected to the live action, even if they are only following the event through an app or website. - Real-time notifications
WebSockets are extensively used to send real-time alerts and notifications to users. This is crucial in applications like trading platforms, where a few seconds' delay can impact a user's financial decisions, and in service monitoring tools, where it is essential to instantly alert users about system outages or performance issues. - Financial trading platforms
In stock or forex trading platforms, where market conditions can change in milliseconds, WebSockets play a vital role in transmitting real-time price updates, trade execution confirmations, and market news without delay. The protocol's ability to maintain a stable, low-latency connection ensures that traders receive swift updates, which is fundamental in making timely, informed trading decisions.
Each of these use cases demonstrates the core strengths of WebSockets in delivering efficient, real-time, bi-directional communication, making it an indispensable technology in modern software and application development. By using WebSockets, developers can ensure that their applications are not just functional but also deliver a seamless and responsive user experience.
Comparison with Other Protocols
Feature | WebSocket | HTTP | Long Polling |
---|---|---|---|
Connection | Persistent | Non-persistent | Non-persistent |
Latency | Low | High | Moderate |
Throughput | High | Moderate | Low |
Complexity | Moderate | Low | High |
Real-Time | Yes | No | Partially |
WebSockets provide an optimized solution for scenarios where real-time data is a necessity rather than a luxury.
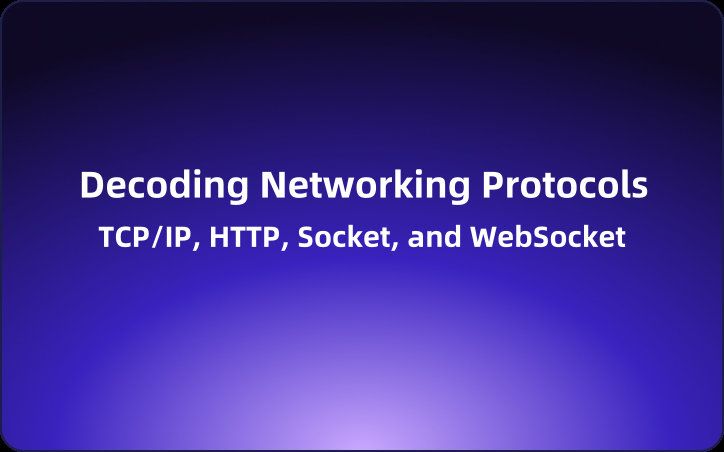
Detailed Example: Real-Time Stock Ticker and Trading Application
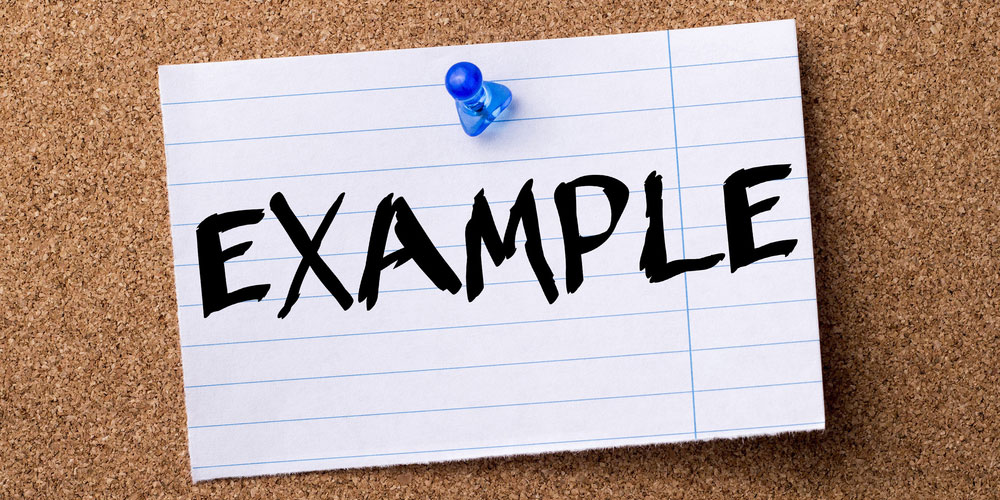
Let's explore a more sophisticated example of using WebSocket to build a real-time stock ticker and trading application. Such applications need to update stock prices instantly and continuously, and also allow users to execute trades in real time.
Scenario
An online trading platform where users can view real-time stock quotes and execute trades.
Benefits of Using WebSocket
- Real-time Data Transmission: Users can see updates to stock prices instantly without needing to refresh the page.
- Reduced Resource Consumption: WebSocket technology uses less server resources and bandwidth than traditional polling.
- Enhanced Interactivity: Provides instantaneous updates to the user interface, enhancing the user experience.
Technical Implementation
Server-Side Code (using Node.js and ws
library)
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
// Simulate stock data
const stocks = {
AAPL: { price: 150 },
GOOGL: { price: 1200 },
MSFT: { price: 200 }
};
// Periodically update stock prices and broadcast them
setInterval(() => {
for (let stock in stocks) {
// Randomly update prices
const change = Math.floor(Math.random() * 10) - 5;
stocks[stock].price += change;
}
wss.clients.forEach((client) => {
if (client.readyState === WebSocket.OPEN) {
client.send(JSON.stringify(stocks));
}
});
}, 1000);
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
const { action, stock, amount } = JSON.parse(message);
if (action === 'BUY' || action === 'SELL') {
// Handle trading actions
console.log(`Received order: ${action} ${amount} shares of ${stock}`);
// Confirm trades with a response
ws.send(`Order confirmed: ${action} ${amount} shares of ${stock}`);
}
});
// Send initial stock prices when a client connects
ws.send(JSON.stringify(stocks));
});
Client-Side Code
const socket = new WebSocket('ws://localhost:8080');
socket.onmessage = function(event) {
// Update display with the latest stock prices
const stocks = JSON.parse(event.data);
console.log('Updated stock prices:', stocks);
};
// Send a trading order
function placeOrder(stock, amount, action) {
socket.send(JSON.stringify({ stock, amount, action }));
}
// Example: Buy 100 shares of Apple
placeOrder('AAPL', 100, 'BUY');
User Interface (HTML and JavaScript)
<button onclick="placeOrder('AAPL', 100, 'BUY')">Buy 100 AAPL</button>
<div id="stockPrices"></div>
<script>
const socket = new WebSocket('ws://localhost:8080');
socket.onmessage = function(event) {
const stocks = JSON.parse(event.data);
document.getElementById('stockPrices').innerHTML = '';
for (let stock in stocks) {
document.getElementById('stockPrices').innerHTML += `<p>${stock}: $${stocks[stock].price}</p>`;
}
};
function placeOrder(stock, amount, action) {
socket.send(JSON.stringify({ stock, amount, action }));
}
</script>
Optimization and Testing
- Performance Testing: Simulate high-load scenarios with multiple users sending trade orders and receiving stock updates simultaneously.
- Fault Tolerance: Handle unexpected disconnections or erroneous user inputs on the server side.
- Security Measures: Implement encrypted WebSocket connections and authentication to protect user data.
With WebSocket technology, this real-time stock ticker and trading application can efficiently synchronize data between users and the server, providing an effective and dynamic online trading experience.
Debugging with EchoAPI
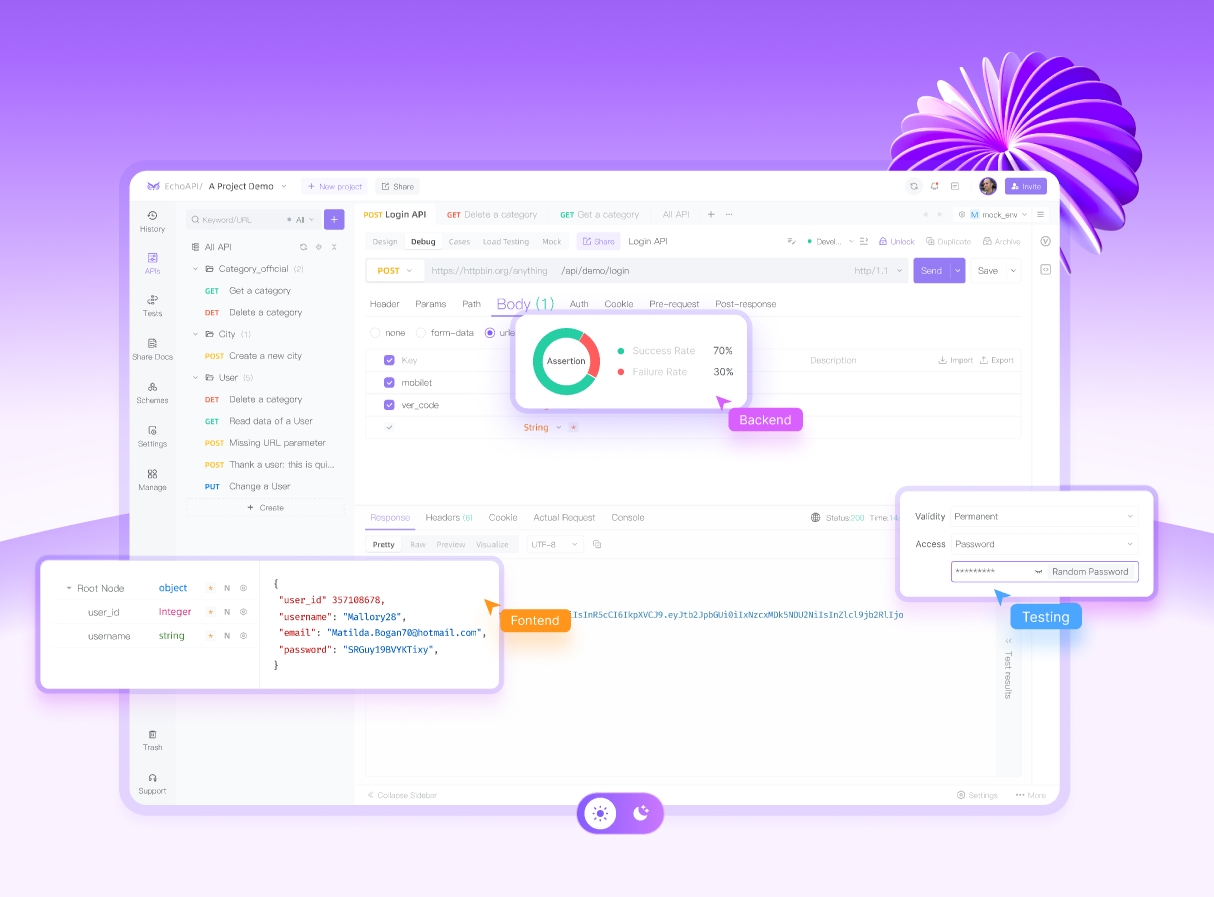
EchoAPI is a versatile tool that supports protocols including HTTP, WebSocket, TCP, SSE, and GraphQL.
We can click the following link to download and use:https://www.echoapi.com/download
For WebSocket debugging:
Step 1: Create a New Request
Open EchoAPI and click the “+” button in the left menu to create a new request. In the request type dropdown menu, select WebSocket.
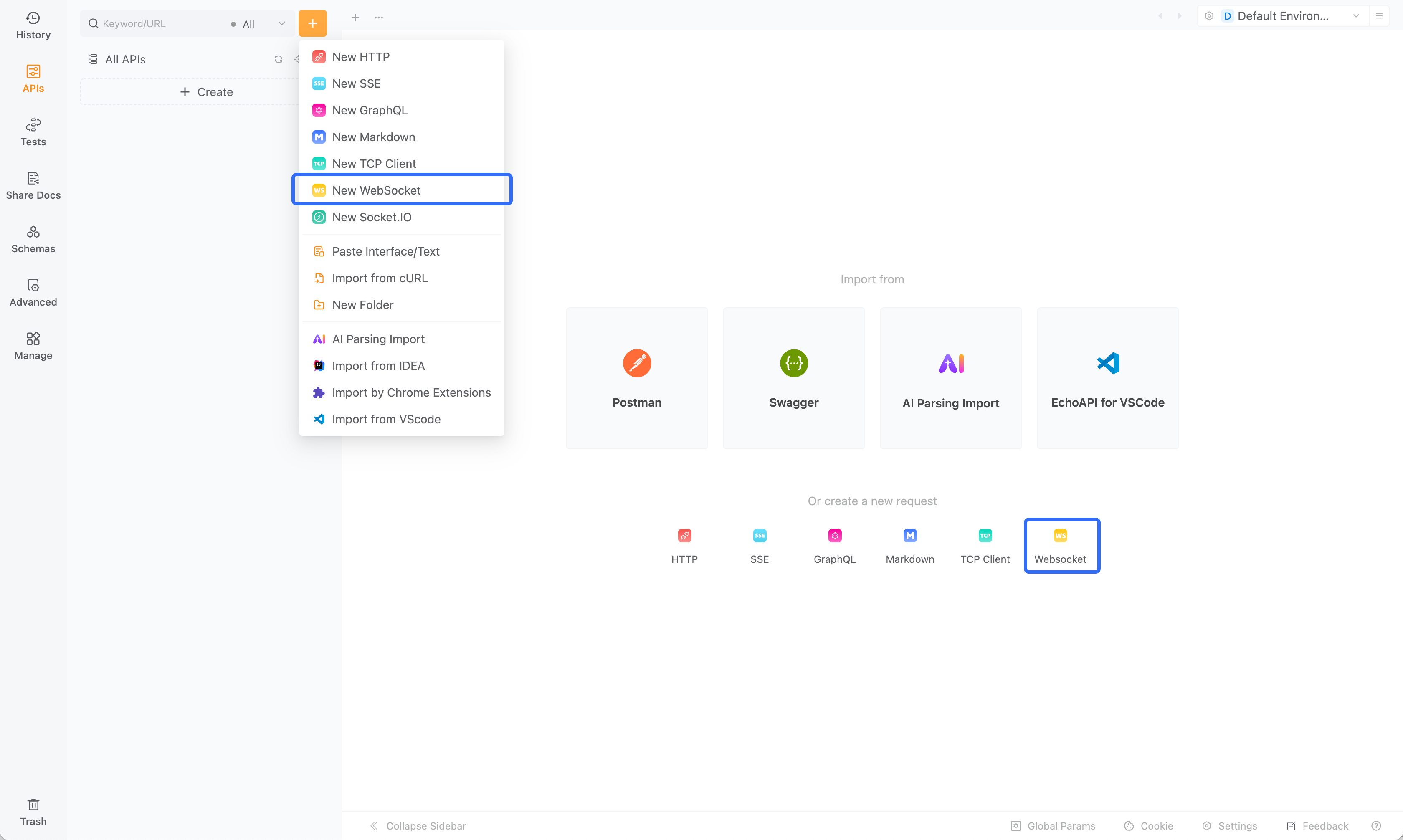
Step 2: Configure the WebSocket Connection
Ensure you are on the Debug
page. In the URL box, enter the WebSocket server URL. For example, wss://echo.websocket.org
. Make sure to replace it with your actual WebSocket server address and port. Note that the address should start with ws://
or wss://
.
If you need to enter request headers or parameters, you can configure them in the position shown in the image below.
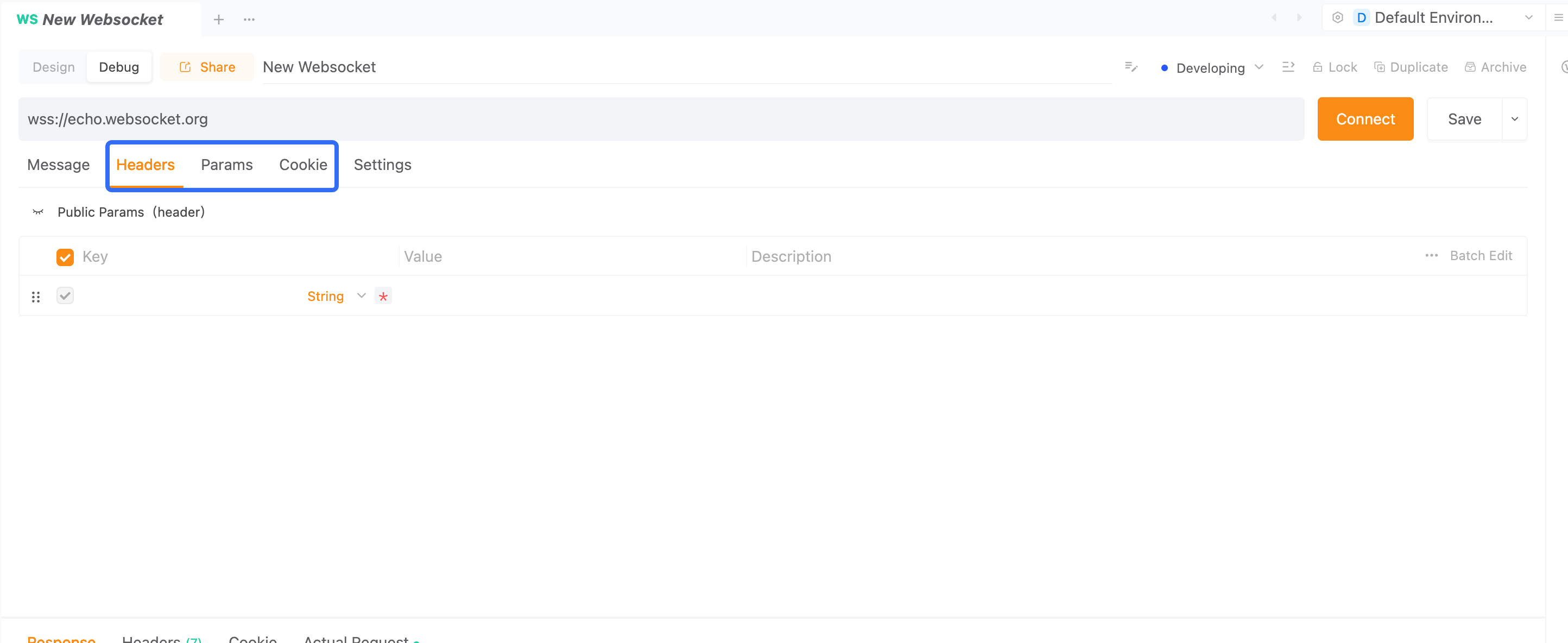
In the settings tab, you can also configure timeout and other settings.
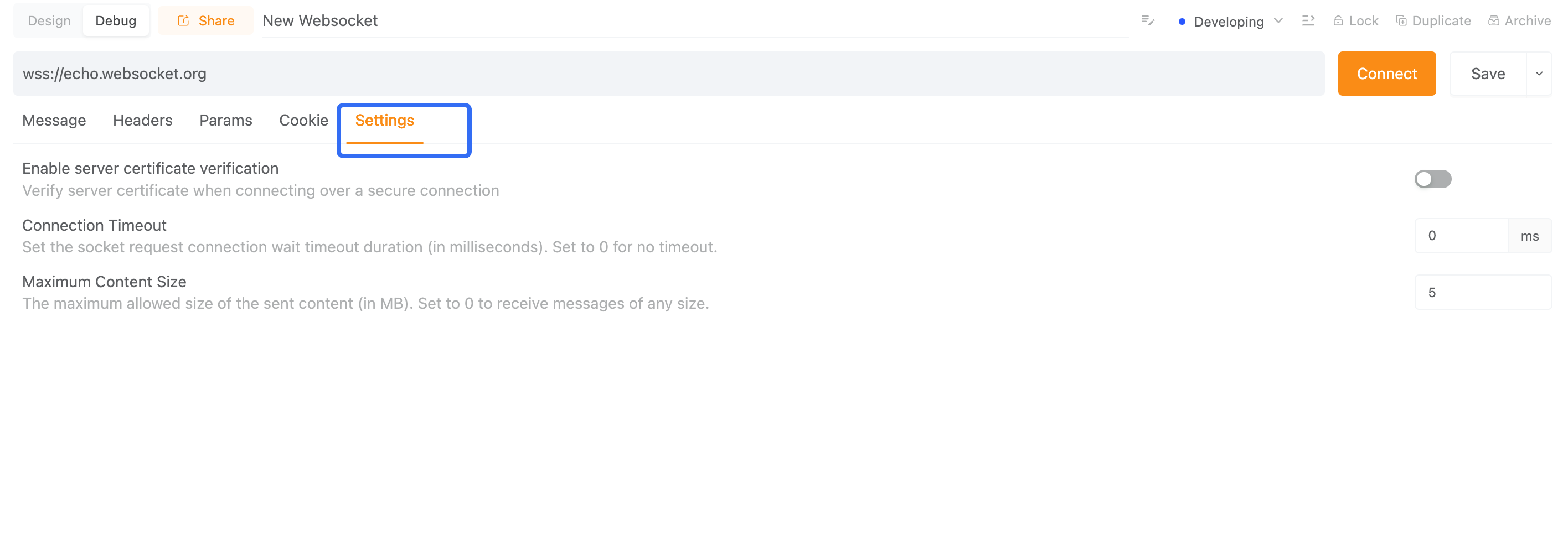
Click the “Connect” button to establish the connection.
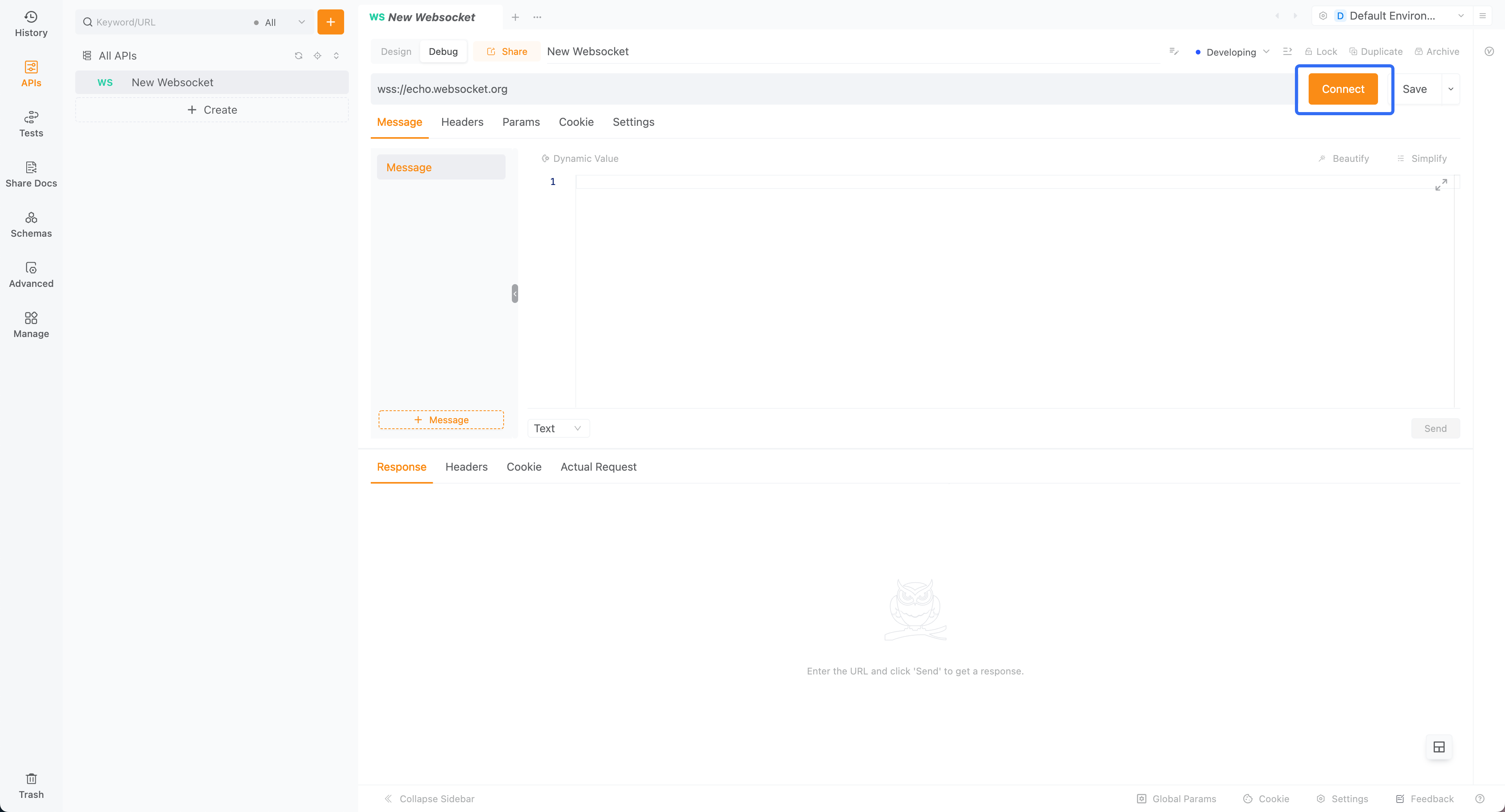
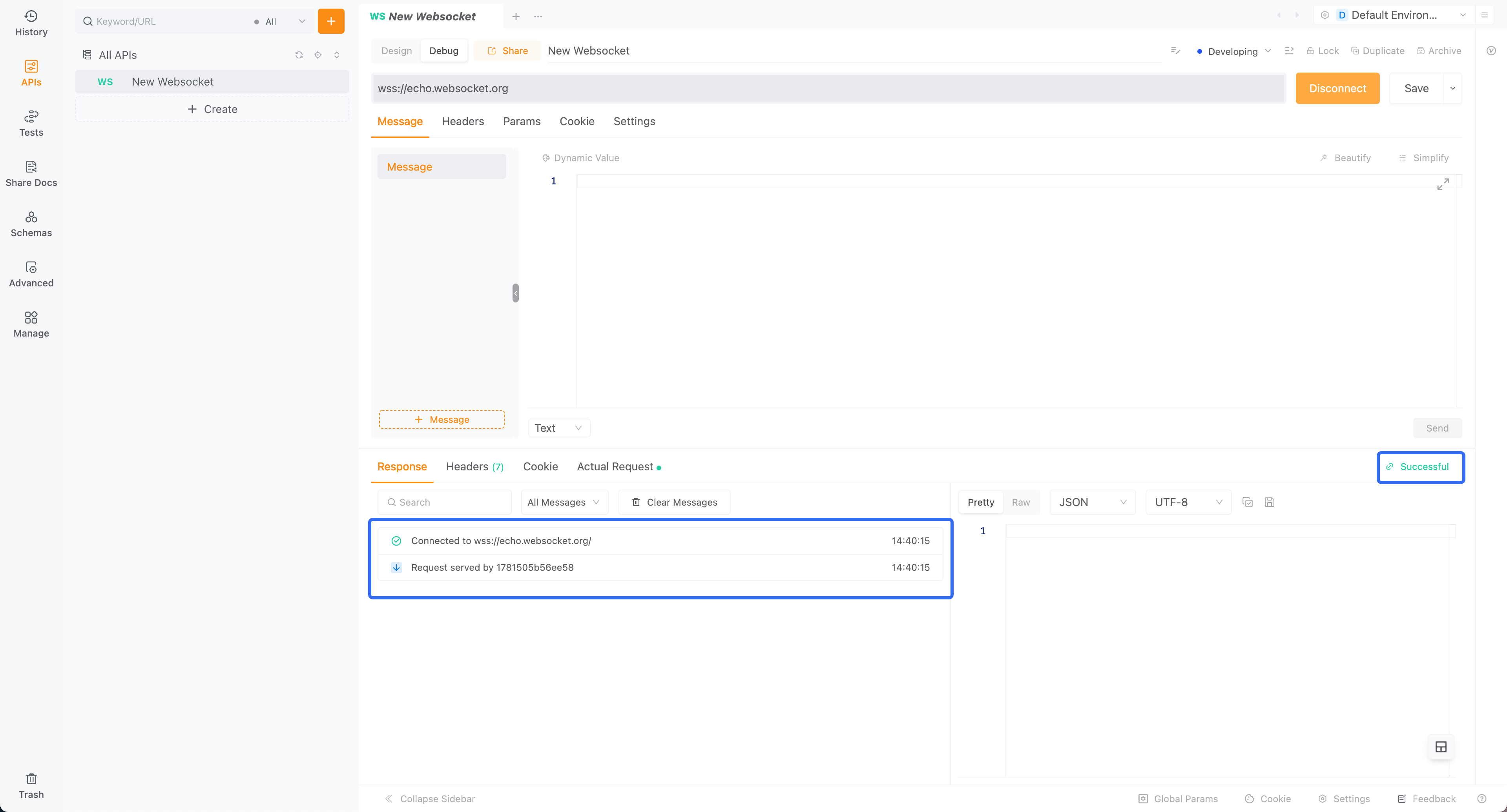
Step 3: Send a Message
Once connected successfully, you can send WebSocket messages. WebSocket messages typically have a type, and you can send them as a JSON object. In the input box, enter the message you want to send. For example:
{
"message": "content",
"data": "Hello, WebSocket!"
}
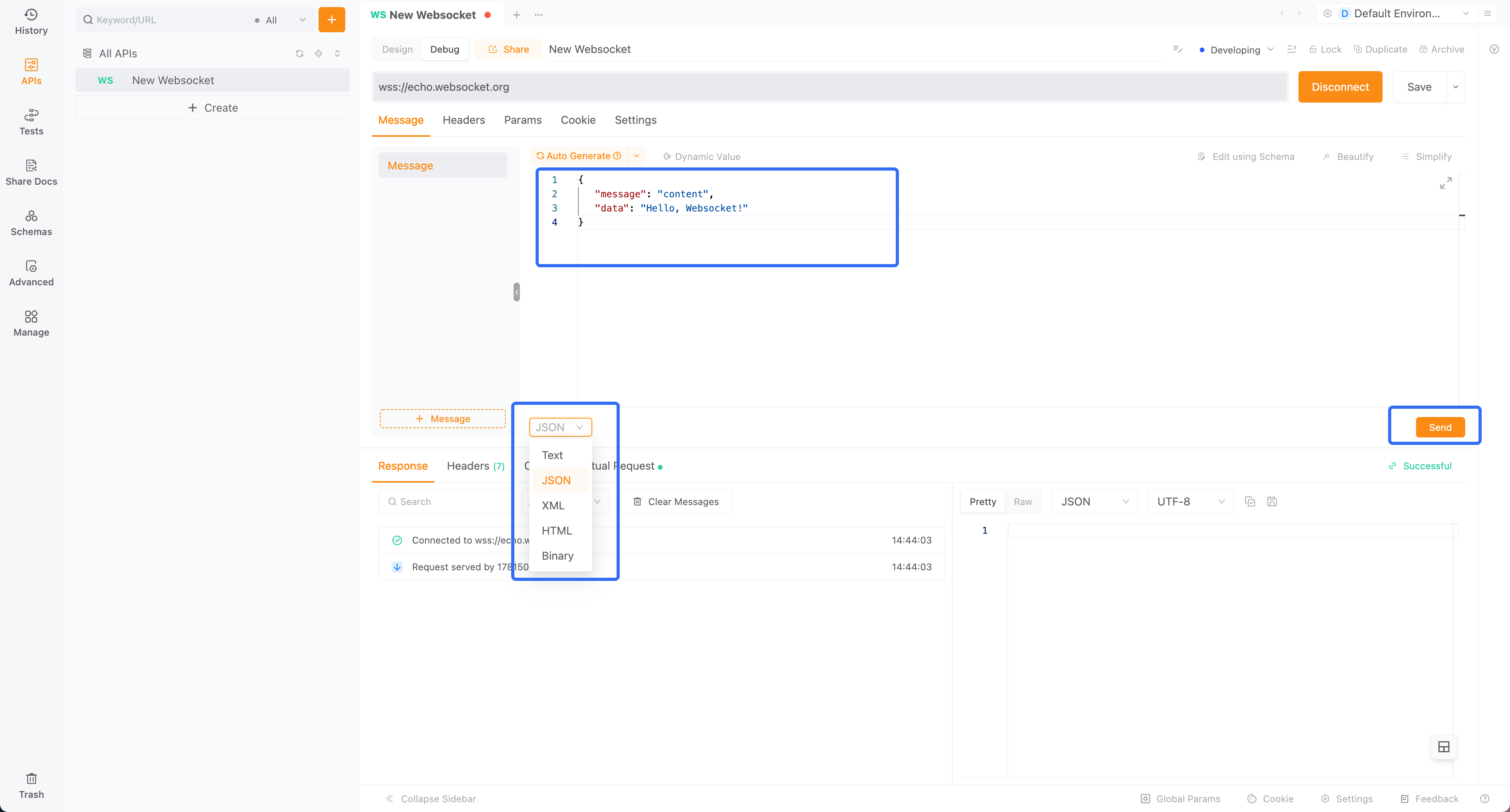
tips
On the left side, you can quickly create multiple messages for debugging, eliminating the need to create multiple WebSocket connections for each different message type. You can add various types of message requests in the same WebSocket connection for archiving, making subsequent debugging faster and more efficient!
You can also double-click the name to rename it.
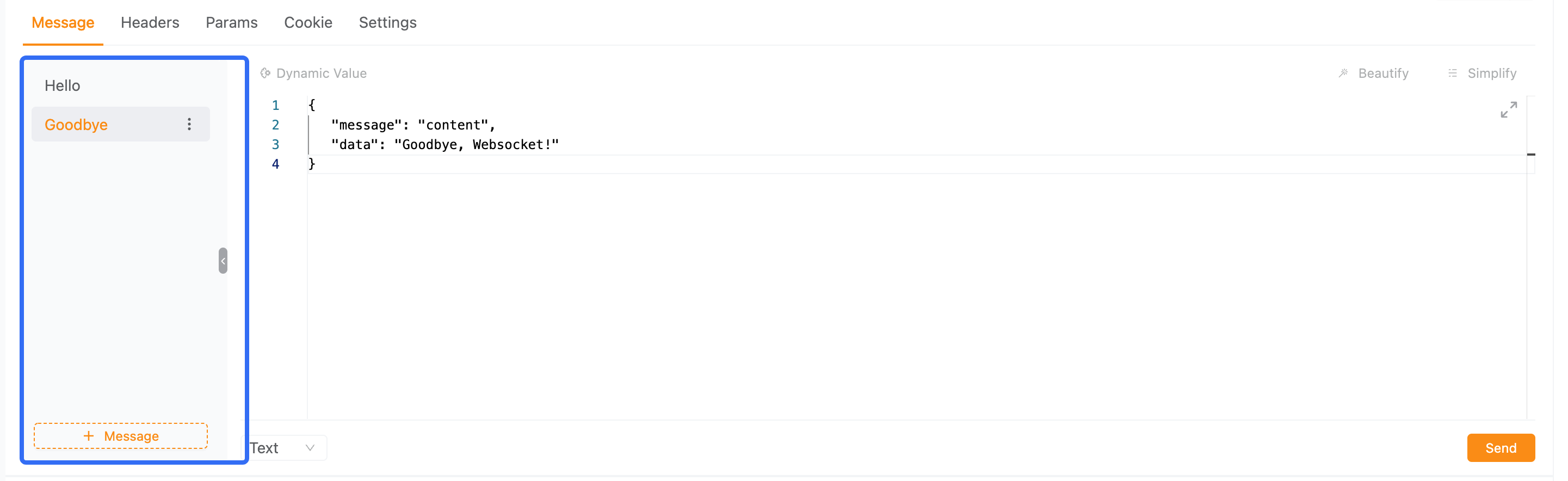
Step 4: Receive Messages
After successfully sending a message, you can start receiving messages from the server. All received messages will be displayed in the “Response” section of EchoAPI.
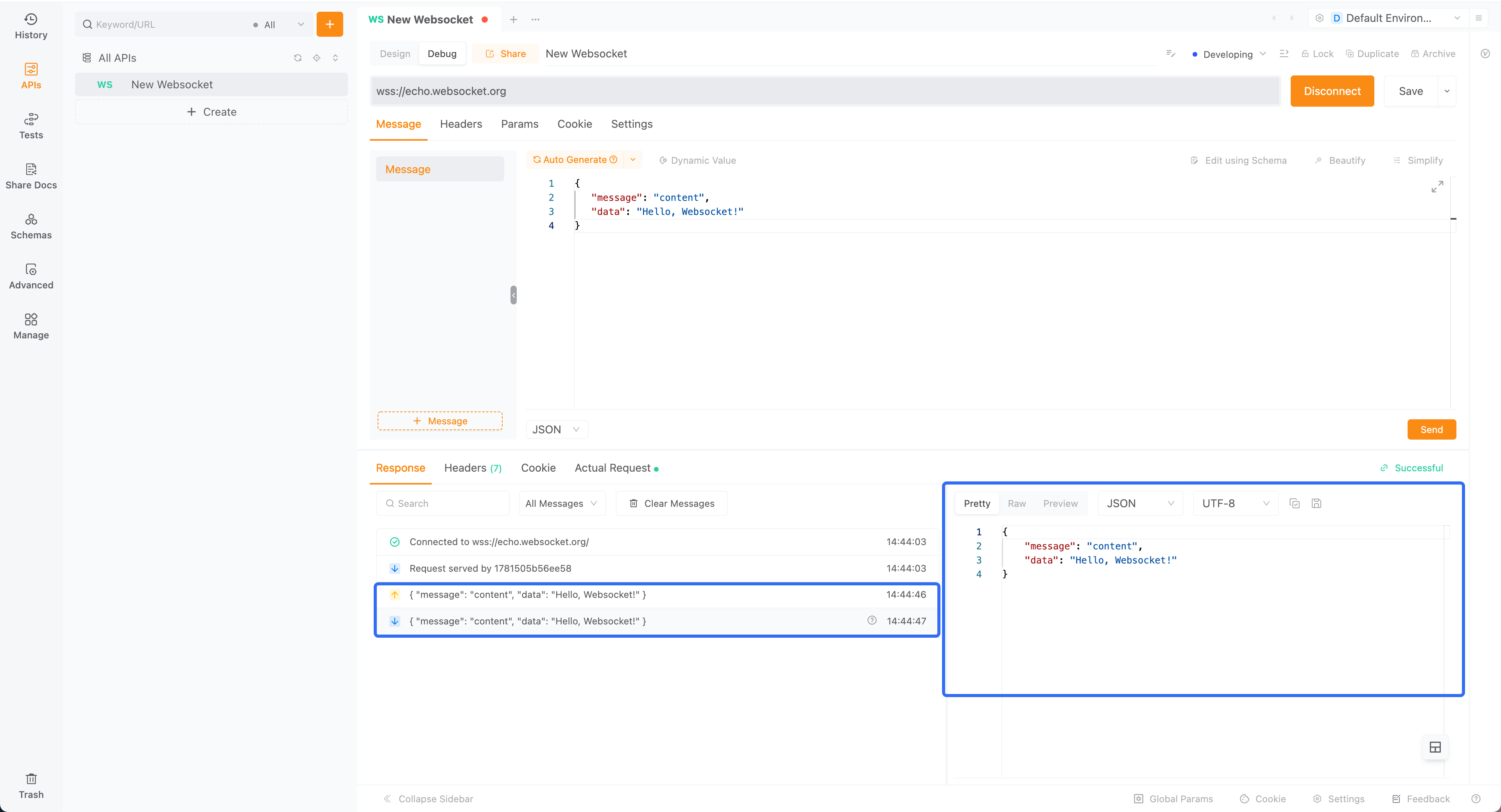
Step 5: Save Received Messages as Responses
In the response area, you can quickly save the current response content as an example response for a particular message, allowing you to generate a more complete API documentation later.
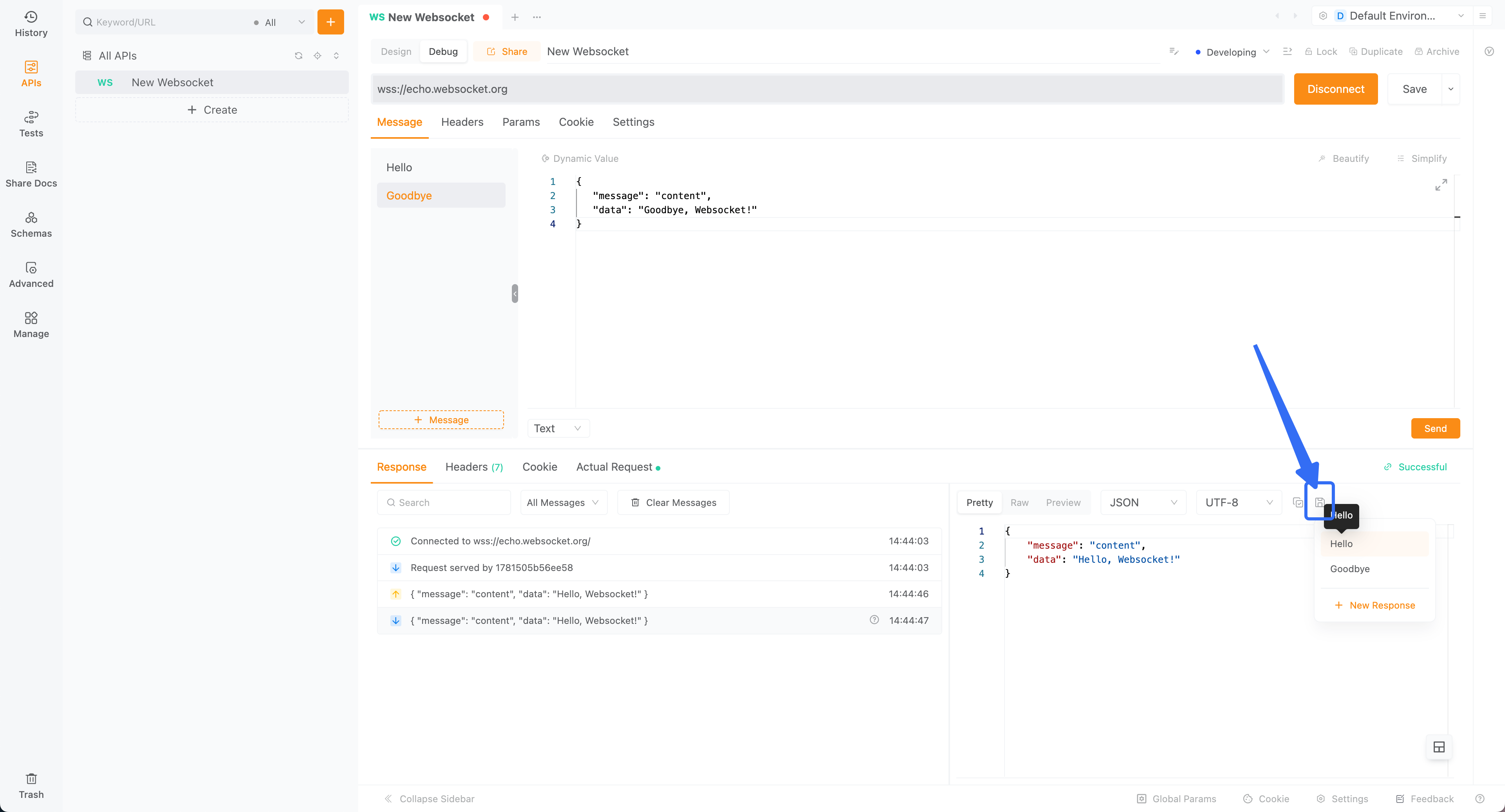
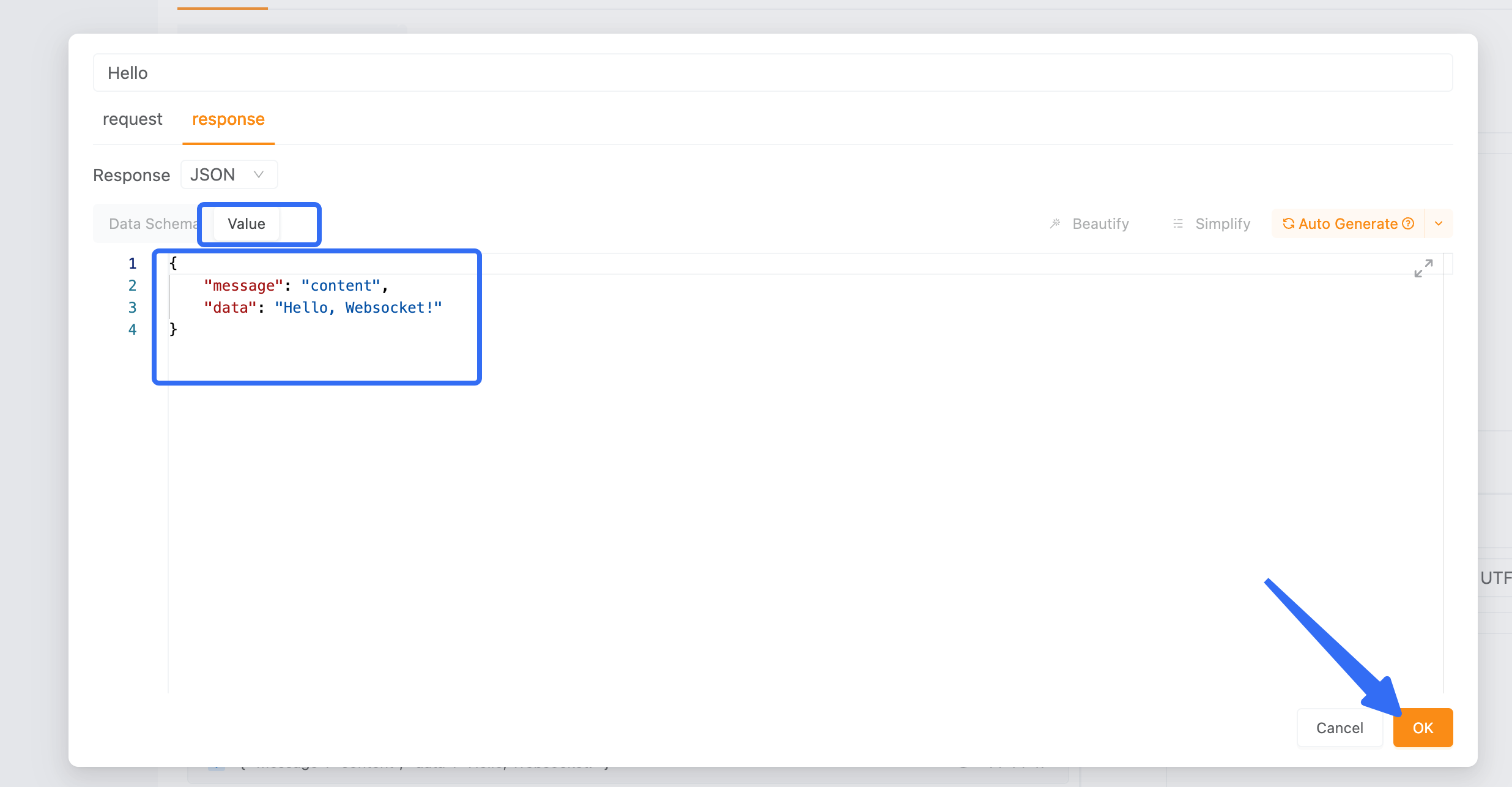
After saving, the generated documentation looks like this. For details, you can check the WebSocket Design/Documentation.
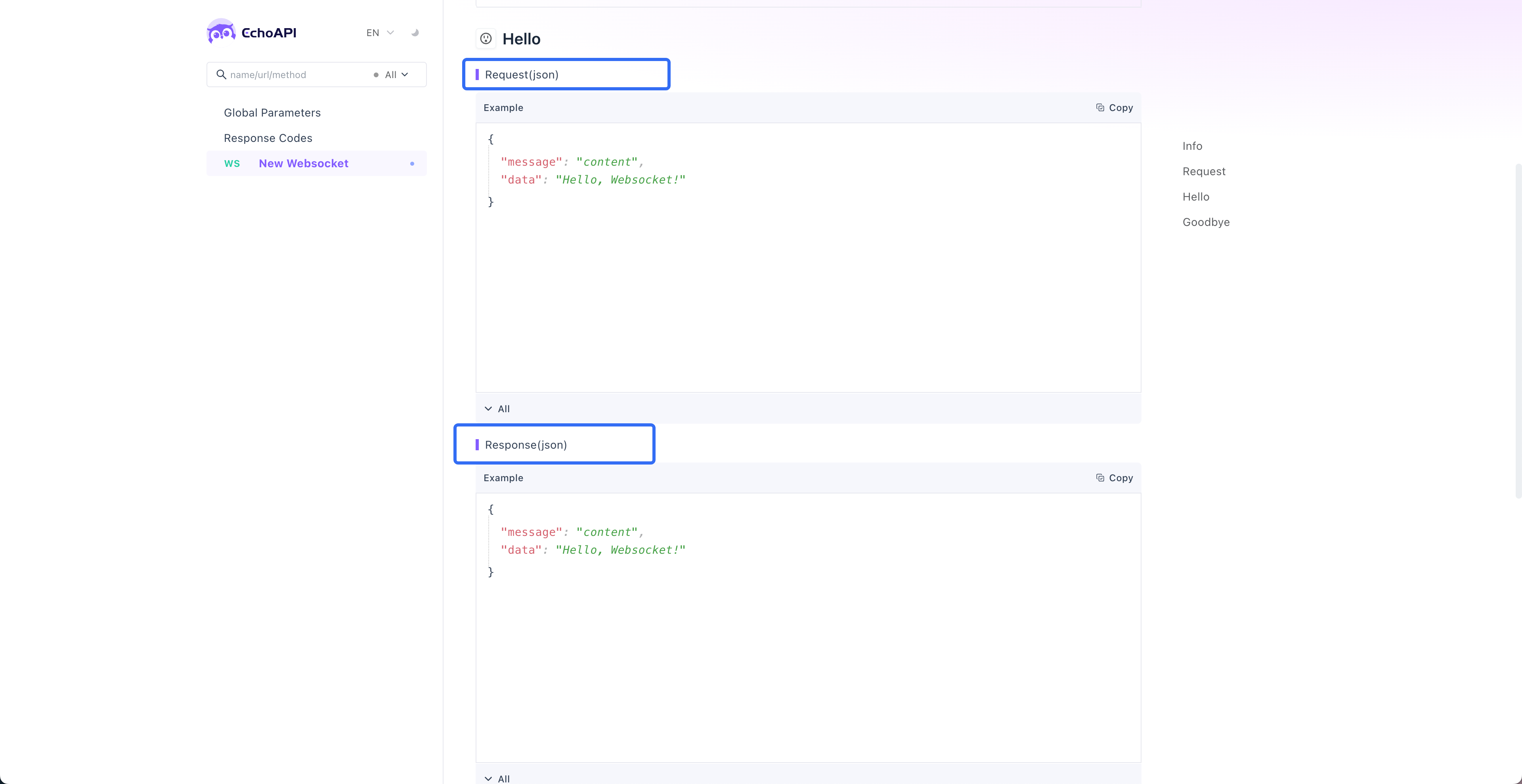
Necessity of WebSocket
In modern web applications, where the timing of data reception can be critical, WebSocket offers a robust solution. Instead of polling the server for a complete response, as seen with traditional HTTP requests, WebSockets maintain an open line where data can flow freely as soon as it's available, reducing latency and increasing the efficiency of data transmission.
Conclusion
WebSockets revolutionize the way we interact with web technologies, breaking down the barriers set by conventional request-response paradigms. The protocol is an essential tool underpinning the creation of dynamic, responsive, and seamless applications across various domains. As real-time interaction becomes more prevalent, understanding and implementing WebSockets is more important than ever for developers looking to stay on the cutting edge of technology.