The Essential Role of Server-Sent Events (SSE) in Modern Web Development
Server-Sent Events (SSE) are a powerful, yet underutilized technology in modern web applications. By leveraging SSE, developers can enhance their applications' responsiveness and real-time capabilities in an efficient and straightforward manner.
In the fast-paced world of web development, embracing cutting-edge technologies that enhance user experience and streamline data flow is crucial for developers. Server-Sent Events (SSE) represent one such technology. This guide aims to shed light on what SSE is, its key features, beneficial use cases, a practical implementation example, and how to debug these events using a tool such as EchoAPI.
What is SSE?
Server-Sent Events (SSE) is a standard describing how servers can initiate data transmission towards browser clients once an initial client connection has been established. It is part of HTML5 and provides a way to send real-time updates to the client. Unlike Websockets, SSE is designed specifically for handling unidirectional communication—from server to client—which simplifies many applications where full duplex communication is not necessary.
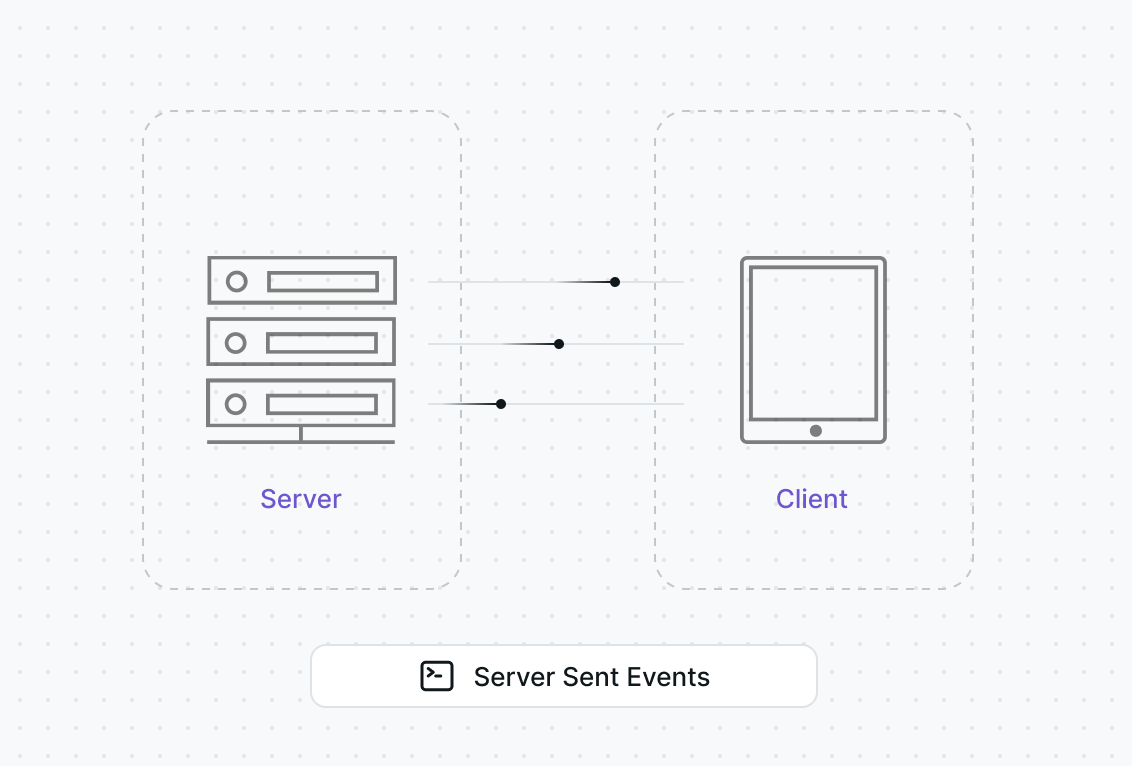
Features of SSE
- Simple Implementation: SSE is easy to use with standard web technologies as it operates over HTTP.
- Built-in Reconnection: Automatic reconnection is an integral feature, with the browser automatically attempting to reconnect to the server in case of connection loss.
- Event-driven Communication: It allows servers to push updates in real time, which are event-driven and can be targeted to specific events in a web application.
- Lightweight Protocol: SSE is designed to be lightweight and simple, using standard HTTP connections for data push.
Common Use Cases for SSE
Server-Sent Events (SSE) are used to enhance user experiences in various online platforms by providing real-time communication from the server to the client. Here are some enriched and detailed scenarios where SSE is particularly beneficial:
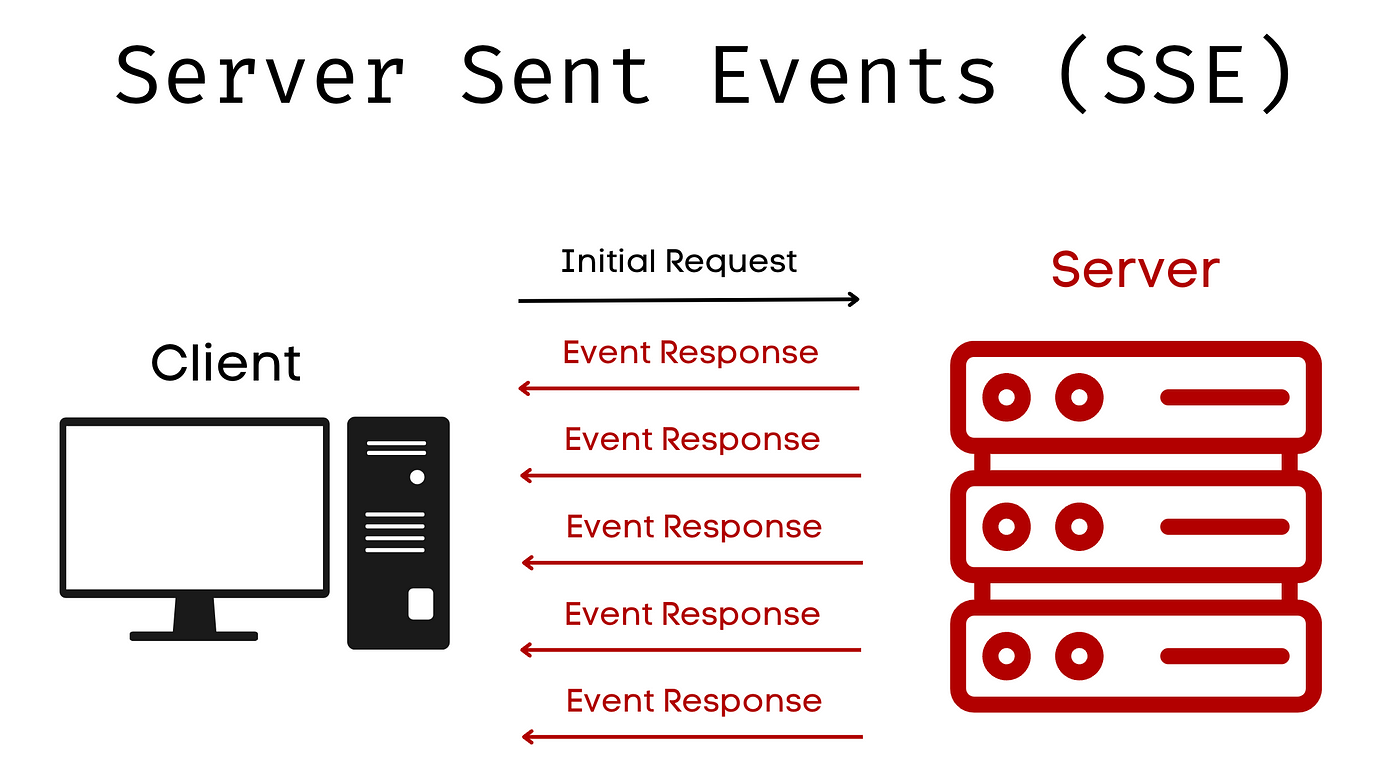
- Live Notifications: Ideal for sending alerts or notifications directly to users' browsers. This can include updates on ticket availability, system status notifications, or urgent messages that require immediate attention without polling the server.
- News Feeds: SSE is perfect for situations where information updates frequently, such as news sites or social media platforms where new posts and interactions need to be pushed to users as they happen.
- Real-Time Analytics: For dashboards monitoring data like traffic flow, server health, or financial markets, SSE allows administrators or relevant users to view changes and metrics updated in real-time without manual refreshes.
- Sport Event Updates: During live sporting events, SSE can be used to push the latest scores, statistics, and play-by-play actions to enthusiasts following online, ensuring fans have the most current information without delay.
- Multiplayer Online Games: In gaming scenarios where events need to be broadcast to all players (like changes in the game world or actions performed by other players), SSE can be effectively utilized to manage these updates efficiently.
- Auction Sites: In online auctions, bidding information needs to be updated in real time for all viewers. SSE can push these updates to all connected clients instantly as new bids are placed, ensuring a fair and timely bidding process.
- Transportation Updates: For service disruptions, schedule changes, or vehicle tracking in public transportation networks, SSE is useful for sending this critical information to commuters or logistic managers in real-time.
- Live Blogging and Commentary: During live events such as product launches or conferences, editors might use SSE to push updates to readers to follow the event in real time, enhancing the reach and engagement of their content.
Each of these use cases demonstrates the flexibility and efficiency of SSE in different contexts—any application scenario where information must be sent from server to client seamlessly and efficiently may benefit from implementing Server-Sent Events, making it a versatile technology in the toolkit of modern web developers.
Practical Business Example: Live Notification System for E-commerce
Scenario
An e-commerce platform wants to implement a notification system to alert users about flash sales and newly available products instantly.
Benefits of Using SSE
- Reduced Load Times: With SSE, new data (such as notifications of a sale starting) can be pushed immediately to users who are online, without them needing to refresh the browser.
- Simplified Architecture: Since SSE works over standard HTTP, the need for a more complex protocol stack is reduced, simplifying the backend complexity.
Technical Implementation
Server-Side Code (using Node.js)
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {
'Content-Type': 'text/event-stream',
'Cache-Control': 'no-cache',
'Connection': 'keep-alive'
});
const sendEvent = setInterval(() => {
const data = `data: ${JSON.stringify({ message: 'Flash Sale Starts Now!' })}\n\n`;
res.write(data);
}, 10000);
req.on('close', () => {
clearInterval(sendEvent);
res.end();
});
});
server.listen(3000, () => {
console.log('SSE server running on port 3000');
});
Debugging with EchoAPI
EchoAPI, which supports HTTP, Websocket, TCP, SSE, and GraphQL, offers a robust environment for API testing.
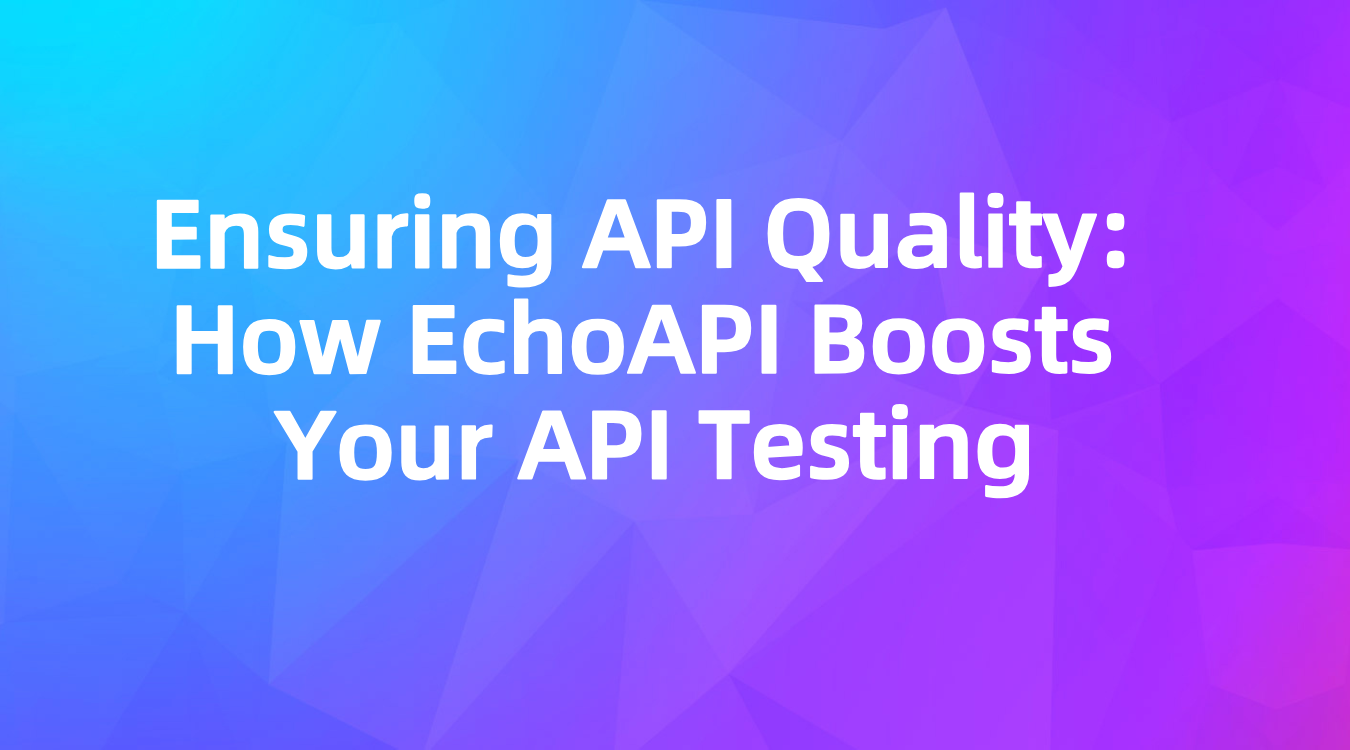
For SSE:
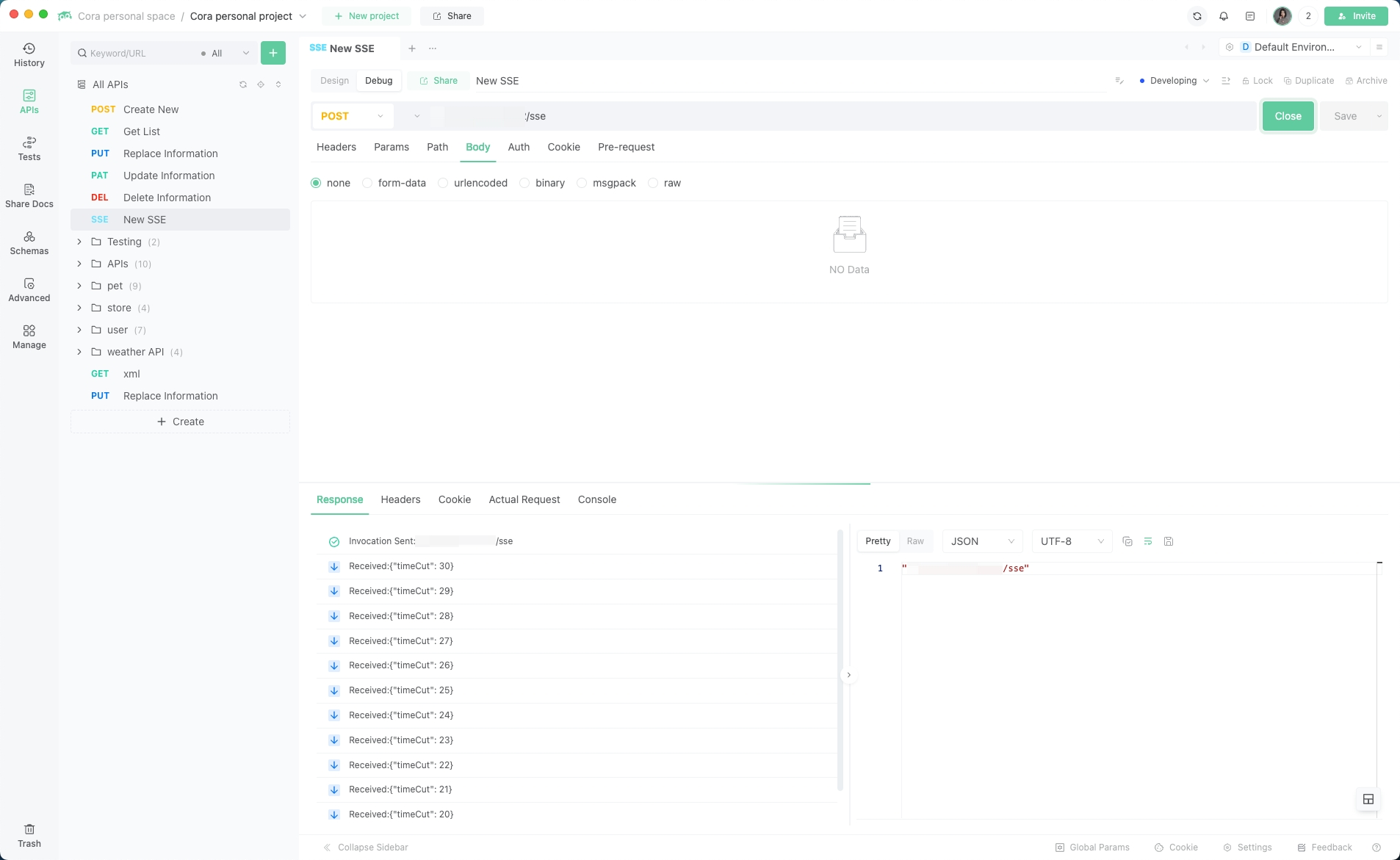
The Importance of SSE
Understanding the use and implementations of SSE is critical for developers who are looking to build responsive and efficient applications. While technologies like WebSocket offer robust two-way communication, the simplicity and specificity of SSE make it an excellent choice for cases where only server-to-client communication is required without the need for a client response.
Conclusion
Server-Sent Events (SSE) are a powerful, yet underutilized technology in modern web applications. By leveraging SSE, developers can enhance their applications' responsiveness and real-time capabilities in an efficient and straightforward manner. With tools like EchoAPI to help debug and optimize SSE implementations, adopting this technology becomes even more approachable and beneficial.