Thunder Client Guide: How to Use the `tc` Object in Scripting
Thunder Client holds a powerful secret in its scripting capabilities, available in its paid version. Let’s break down how to use the tc object for various advanced use cases, making your API testing smooth and efficient.
Looking to up your API testing game? Thunder Client holds a powerful secret in its scripting capabilities, available in its paid version. Let’s break down how to use the tc
object for various advanced use cases, making your API testing smooth and efficient.
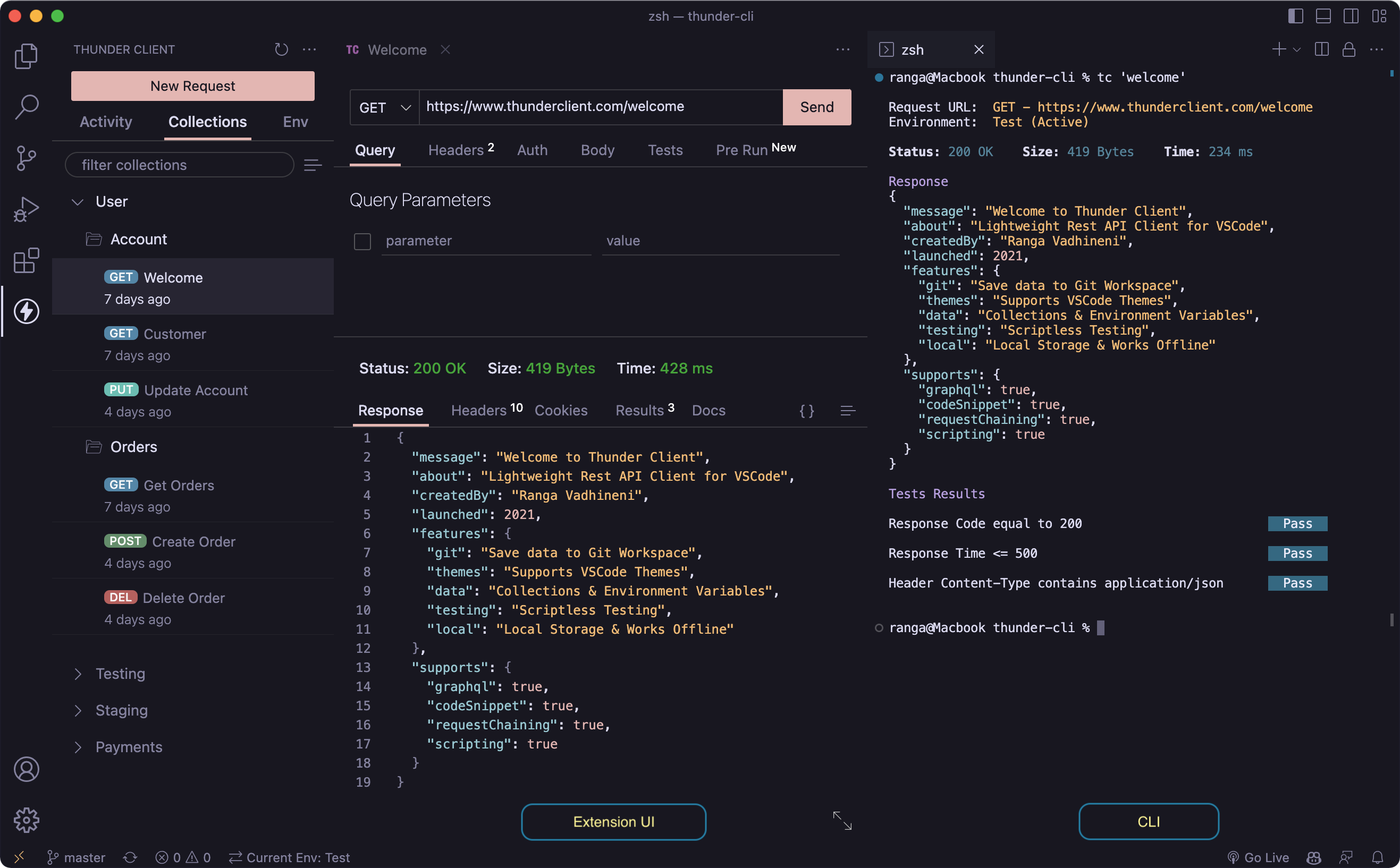
Introduction to Thunder Client
Thunder Client is a lightweight REST API client for Visual Studio Code, designed to simplify API testing. It allows you to set environment variables, extract data from responses, execute test assertions, and more. For these advanced tasks, the scripting feature in the paid version is indispensable.
Using the tc
Object in Scripting
Global Variables
The tc
object is your primary hub for API access in Thunder Client. Alongside tc
, you also get access to other useful global variables like expect
and assert
from the Chai assertion library, and functions like btoa
for Base64 encoding and atob
for Base64 decoding.
Built-in Libraries
Thunder Client provides several built-in libraries for various tasks:
- axios: Promise-based HTTP client
- chai: Assertion library
- crypto-js: Cryptographic operations
- fs: File system module
- http/https: HTTP and HTTPS modules
- uuid: UUID generator
Using axios
const axios = require('axios');
const response = await axios.get("https://www.thunderclient.com/welcome");
console.log(response);
Managing Requests
Request Object
You can access and modify the request object properties:
tc.request.id
tc.request.url
tc.request.method
tc.request.headers
tc.request.body
Modifying Headers
tc.request.setHeader('Content-Type', 'application/json');
const contentType = tc.request.getHeader('Content-Type');
console.log(contentType);
Modifying Body
tc.request.setBody({ key: "value" });
console.log(tc.request.body);
Managing Responses
Response Object
This object is read-only and contains properties like:
tc.response.status
tc.response.time
tc.response.contentType
tc.response.json
tc.response.text
Accessing Headers and Cookies
const responseHeader = tc.response.getHeader('Set-Cookie');
console.log(responseHeader);
const binaryResponse = tc.response.getBinary();
console.log(binaryResponse);
Advanced Methods
Handling Variables
tc.setVar('myVar', 'value');
const myVar = tc.getVar('myVar');
console.log(myVar);
Running and Retrying Requests
await tc.runRequest('reqId');
await tc.retryRequest();
Skipping Requests
tc.skipRequest('reqId');
Why EchoAPI is a Great Alternative
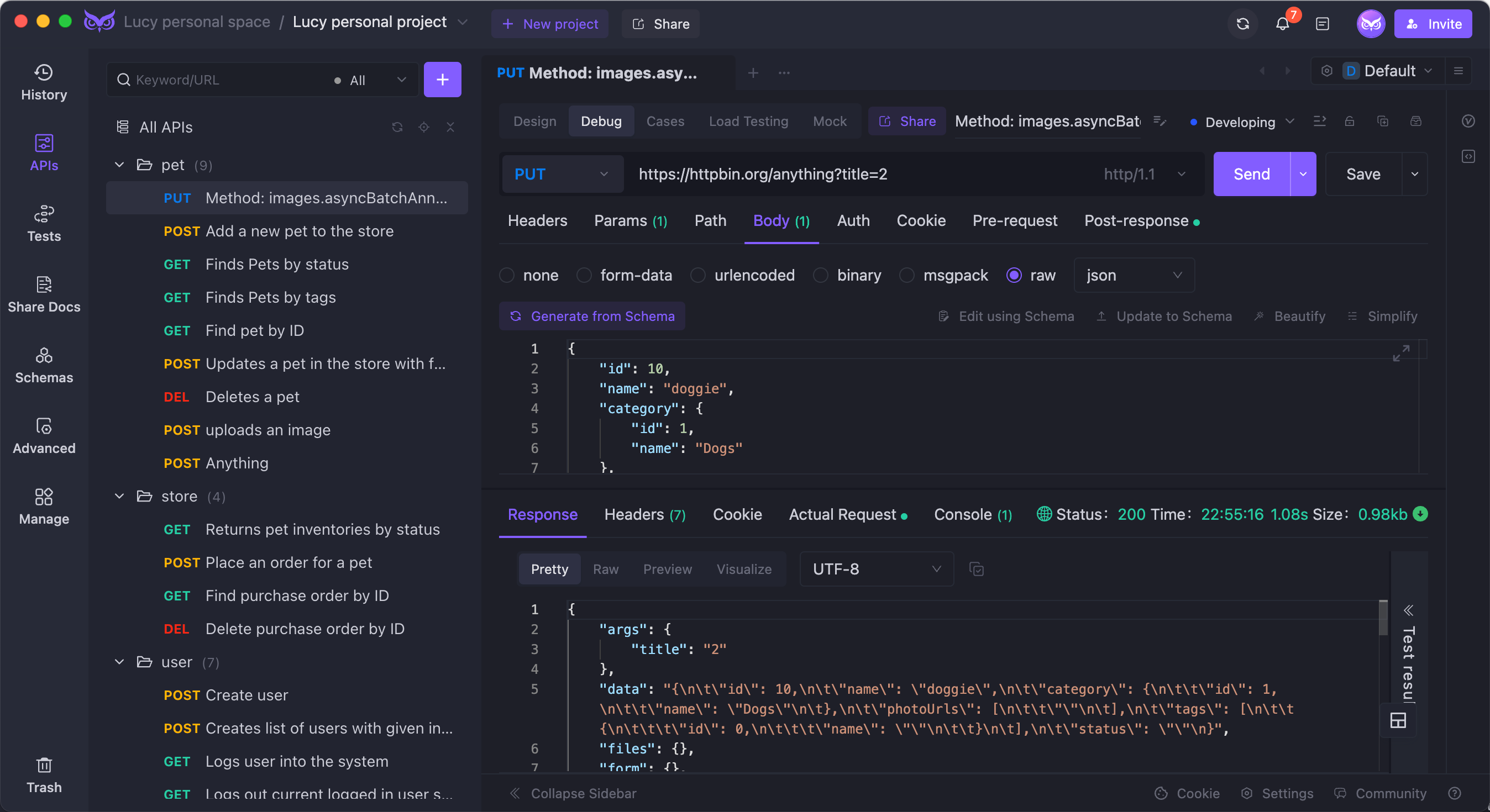
If you’re considering alternatives, EchoAPI for VS Code is a powerful, ultra-lightweight collaboration tool perfect for API development. It supports features like API design, debugging, automated testing, load testing, and more.
Key Features of EchoAPI:
- No login required: Start immediately without the hassle of signing up.
- Supports Scratch Pad: Quickly jot down notes or temporary code.
- Ultra lightweight: Designed to be fast and efficient.
- 100% compatible with Postman script syntax: Easily migrate or use your existing scripts.
EchoAPI seamlessly integrates with IntelliJ IDEA, VS Code, and Chrome request capture extension, enhancing your API development workflow significantly.
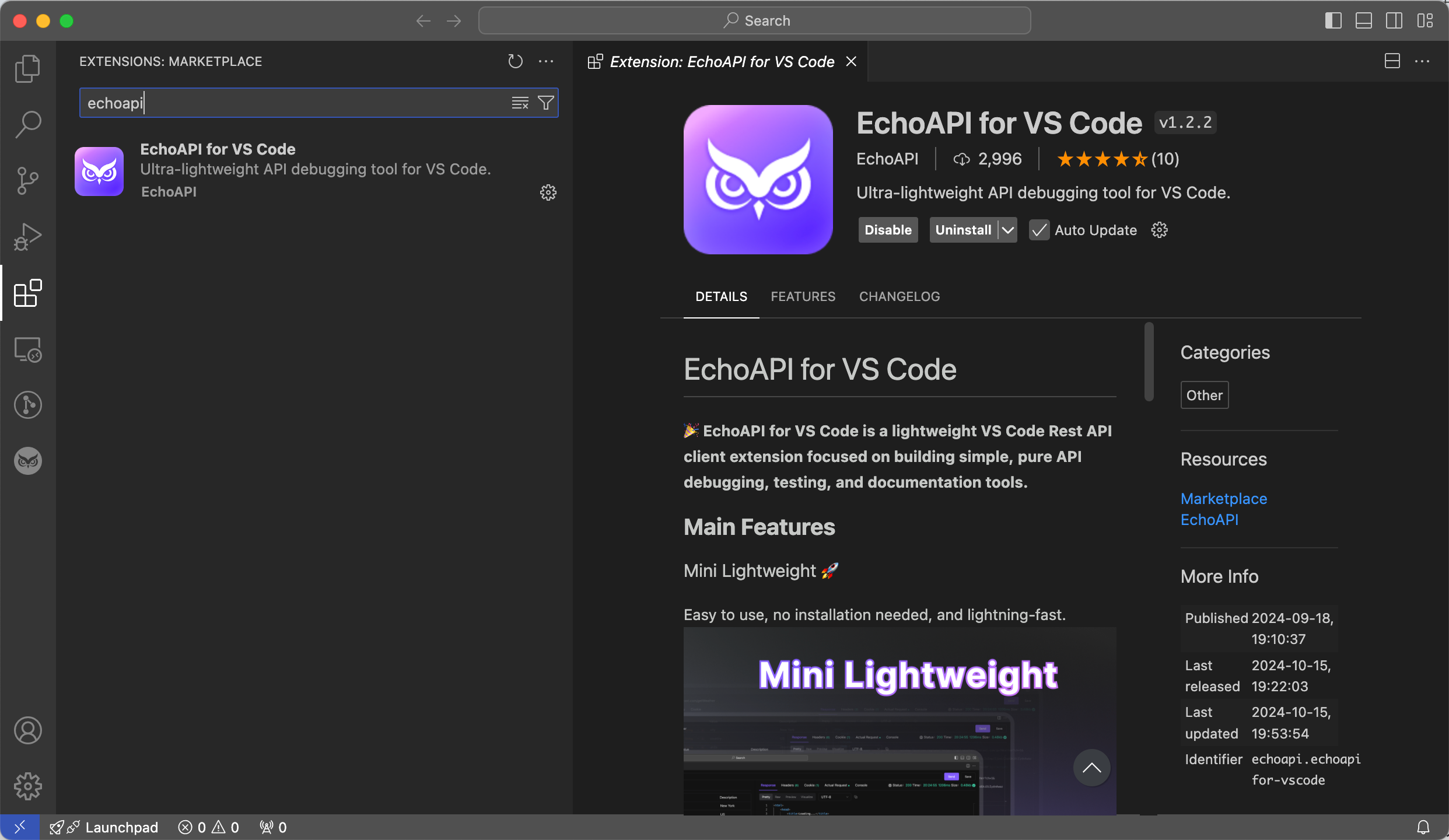
By leveraging the full power of the tc
object, you can perform highly efficient and advanced API testing with Thunder Client. Whether you stick with Thunder Client or try out EchoAPI, both tools offer robust features to streamline your API workflows. Happy testing!