Utilizing Pre-execution and Post-execution Scripts in EchoAPI
Pre-execution scripts in EchoAPI are designed to execute specific tasks before an HTTP request is sent.
Introduction to Pre-execution Scripts
Pre-execution scripts in EchoAPI are designed to execute specific tasks before an HTTP request is sent. These scripts can be used for a variety of purposes, such as defining global variables, manipulating request headers, and performing complex calculations with JavaScript.
1. Complex Calculations with JS
You can create functions to perform complex calculations or generate values dynamically. For example, to concatenate a string with a random number and assign it to a global variable:
function _random(){
return 'Hello, Sky ' + Math.random();
}
pm.globals.set("random_var", _random());
2. Logging Information
Simply log any data by using
console.log("Message")`.
3. Managing Environment Variables
pm.environment.set("key", "value"); // Set an environment variable 'key' with the value 'value'.
pm.environment.get("key"); // Get the value of the environment variable 'key'.
pm.environment.delete("key"); // Delete the environment variable 'key'.
pm.environment.clear(); // Clear all defined environment variables.
4. Managing Global Variables
pm.globals.set("key", "value"); // Set a global variable 'key' with a value 'value'
pm.globals.get("key"); // Get the value of the global variable 'key'
pm.globals.delete("key"); // Delete the global variable 'key'
pm.globals.clear(); // Clear all defined global variables
5. Request Parameters
Utilize the request object to log and manipulate request parameters.
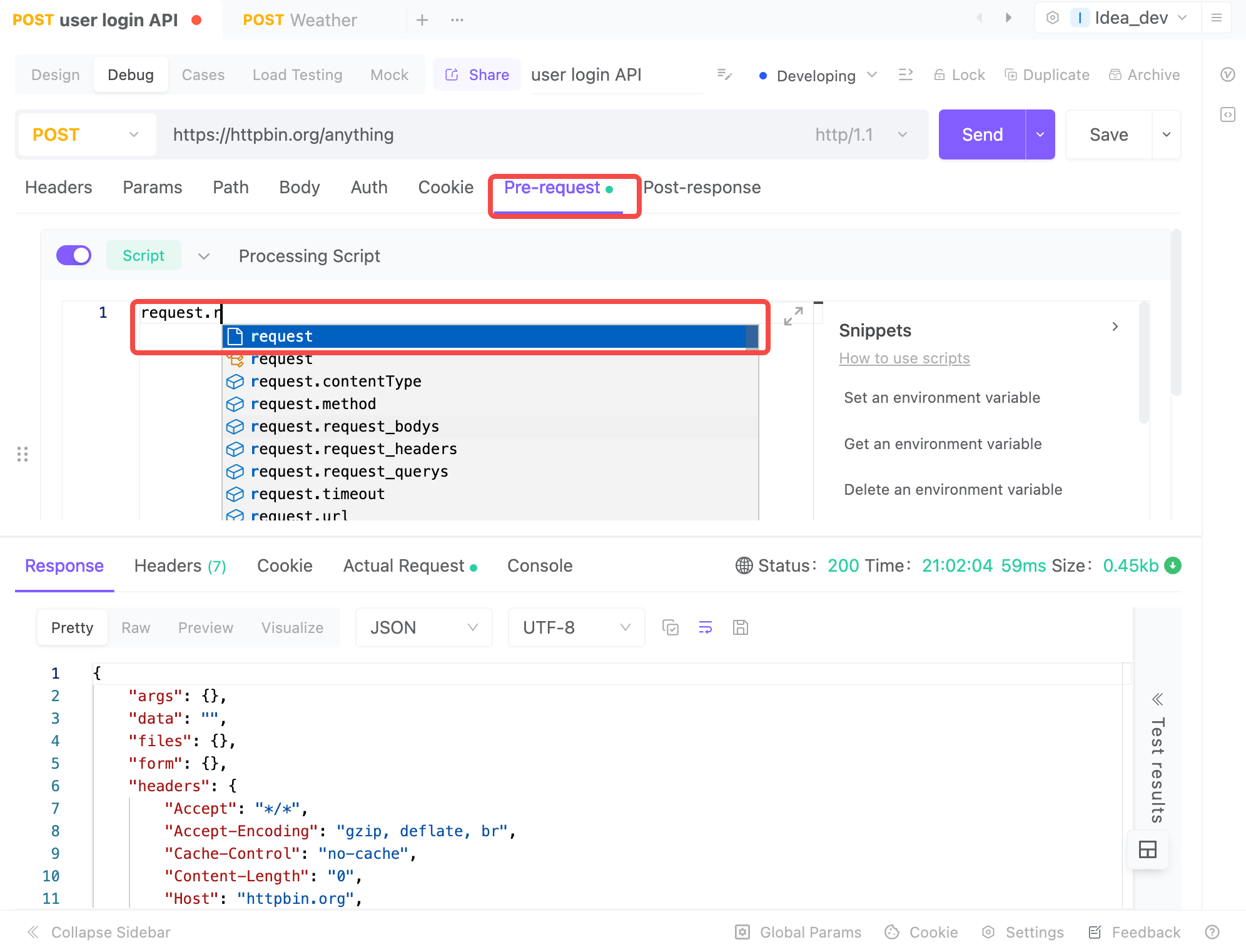
6. Dynamic Header Management
pm.setRequestHeader("key", "value"); // Dynamically add a header parameter with key as the key and value as the value
pm.removeRequestHeader("key"); // Remove the parameter with key as the key from the header parameters
7. Query Parameter Management
pm.setRequestQuery("key", "value"); // Dynamically add a query parameter with key as "key" and value as "value"
pm.removeRequestQuery("key"); // Remove the query parameter with key "key"
8. Body Parameter Management
apt.setRequestBody("key", "value"); // Dynamically add a body parameter with key as "key" and value as "value"
apt.removeRequestBody("key"); // Remove the parameter with key as "key" from the body
9. Sending HTTP Requests
Send asynchronous HTTP requests and handle responses accordingly. Example:
$.ajax({
url: "https://echoapi.sky.com/get.php",
method: "POST",
headers: {
"content-type": "application/json"
},
timeout: "10000",
data: JSON.stringify({"email": "xxx@xxx.com", "password": "123456"}),
success: function (response) {
pm.globals.set("bigint", response.bigint);
}
});
Synchronously send HTTP requests using:
await $.ajax({
url: "https://echoapi.sky.com/get.php",
method: "POST",
headers: {
"content-type": "application/json"
},
timeout: "10000",
data: JSON.stringify({"email": "xxx@xxx.com", "password": "123456"}),
success: function (response) {
pm.globals.set("bigint", response.bigint);
}
});
Post-Execution Script Functions (Scripts Executed After Request Sending)
Post-execution scripts run after an HTTP request is completed, allowing developers to process the response data.
1. Write JS functions, etc., to perform complex calculations
Same as pre-execution scripts.
2. Define, get, delete, and clear environment variables
Same as pre-execution scripts.
3. Define, get, delete, and clear global variables
Same as pre-execution scripts.
4. Get request parameters (request object)
Same as pre-execution scripts.
5. Get response parameters (response object)
An object composed of all the response parameters of a request. It can only be used in post-execution scripts (as there are responses only after sending). We can print, view, and use this variable in the post-execution script.
response.raw: Raw response data
response.raw.status // Response status code (200, 301, 404, etc.)
response.raw.responseTime // Response time (milliseconds)
response.raw.type // Response type (json, etc.)
response.raw.responseText // Response text
response.headers.server // Also response.headers["server"] Response headers
response.cookies.PHPSESSION // Also response.cookies["PHPSESSION"]` Response cookies
response.json: Response data in JSON format
`response.json.data.token` // Also `response.json.data["token"]`
6. Send HTTP requests
Same as pre-execution scripts.
7. Test (assert) the correctness of the request's return results
Define Global Variables via Post-Execution Scripts
Global variables added in the global parameters need manual updates each time they change. However, global variables defined via post-execution scripts are updated automatically whenever the script is executed with the corresponding interface.
In the post-execution script of the login interface, define or update the global token variable using the response data.Other interfaces that need to use the token can directly call it.
set globals variables:
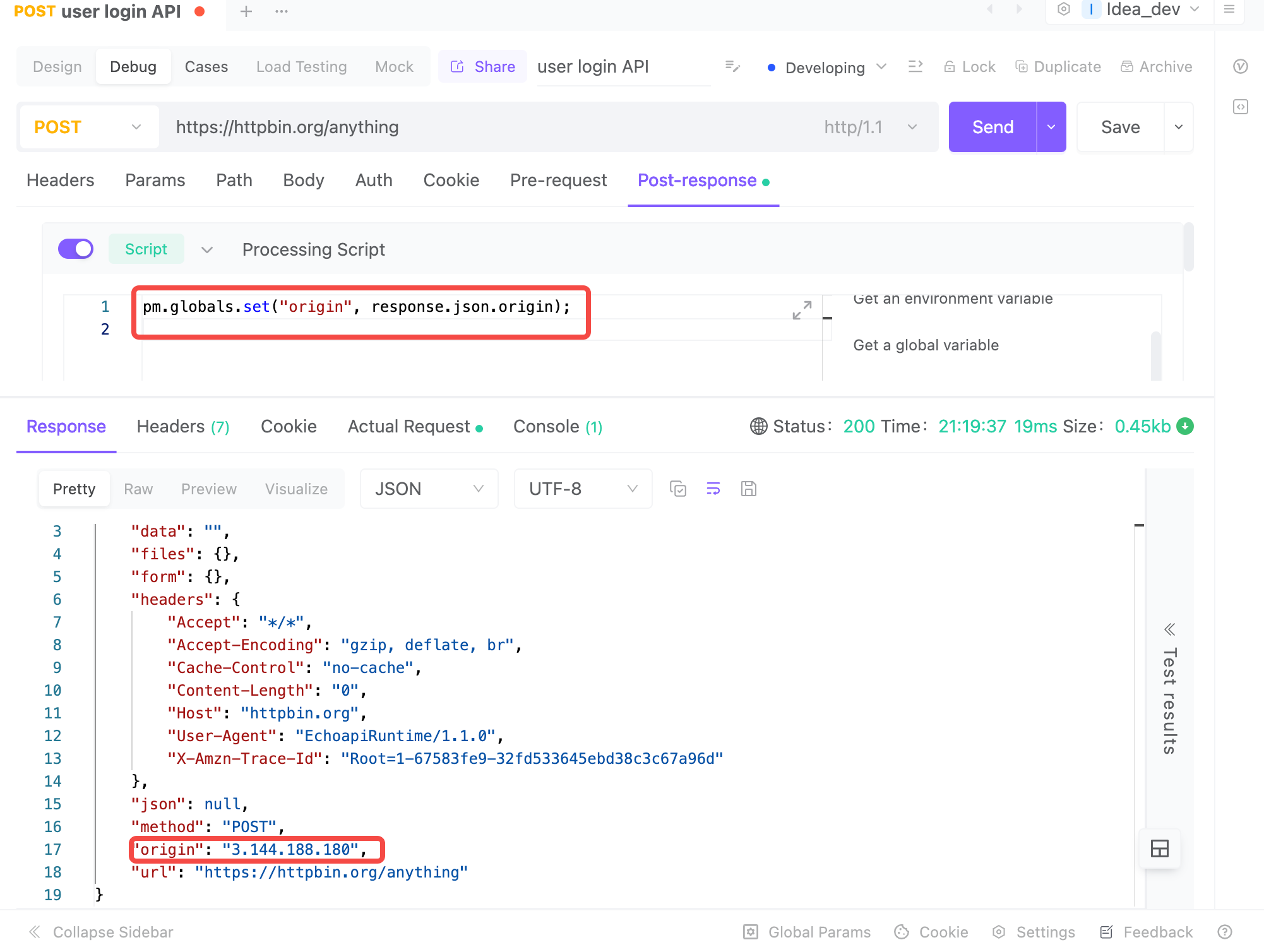
use globals variables:
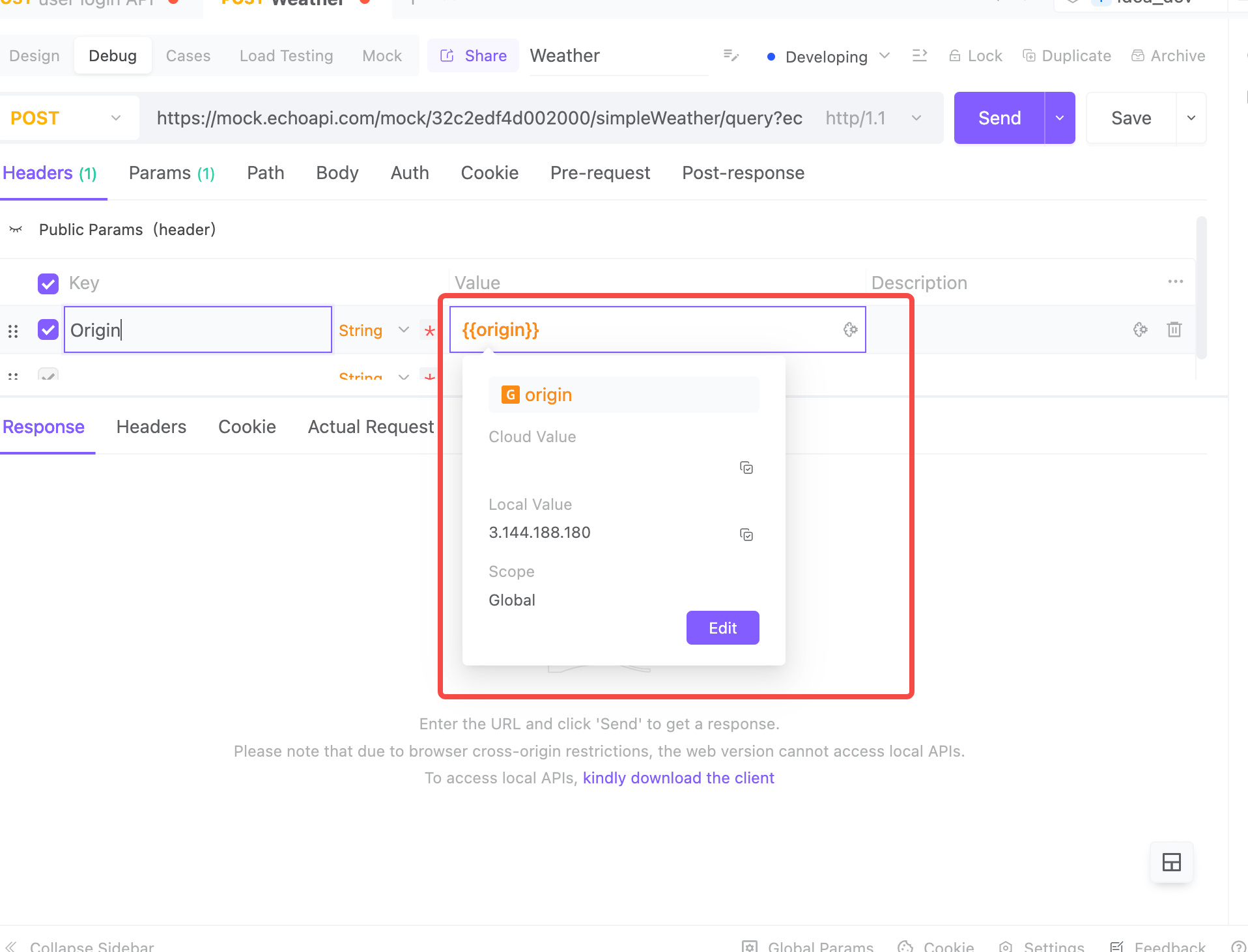
Pre-Execution Script Business Process
- Register an account
- Log in using the registered account
- Update the token variable upon login
let username = "test007";
let email = pm.environment.get("email");
let password = "123456";
let password_confirmation = "123456";
pm.setRequestHeader("Content-Type","application/json");
pm.setRequestBody("name",username);
pm.setRequestBody("email",email);
pm.setRequestBody("password",password);
pm.setRequestBody("password_confirmation",password_confirmation);
sleep(3000)
let host = pm.environment.get("host");
await $.ajax({
"url":"https://"+host+"/api/auth/login",
// "url":"https://api.shop.eduwork.cn/api/auth/login",
"method":"POST",
"content-type":"appication/json",
"data":JSON.stringify({
"email":email,
"password":password
}),
"success":function(response){
response = typeof response == "object" ? response : JSON.parse(response);
console.log(response);
// pm.variables.set("token", response.token);
pm.globals.set("access_token", response.access_token);
}
});
By mastering these EchoAPI scripts, developers can automate complex workflows, manage data across requests efficiently, and enhance API testing processes.