What Is Basic Auth for REST APIs and How to Debug It With Code & Tools
EchoAPI is a powerful tool for API testing. Here's how to use it for Basic Auth.
Why Use Authentication for REST APIs? ๐
In the evolving landscape of web services, REST APIs play a crucial role in enabling communication between various software systems. However, with great power comes great responsibility. It is paramount to ensure that sensitive data remains secure and communication is reliable. This is where authentication comes into play. By using authentication, we can:
- Verify the identity of users or systems accessing the API.
- Control access to resources, ensuring only authorized users can perform certain actions.
- Protect data from unauthorized access and potential breaches.
- Log and monitor API usage, helping with compliance and tracing unauthorized attempts.
In essence, authentication fortifies the integrity and security of REST APIs.
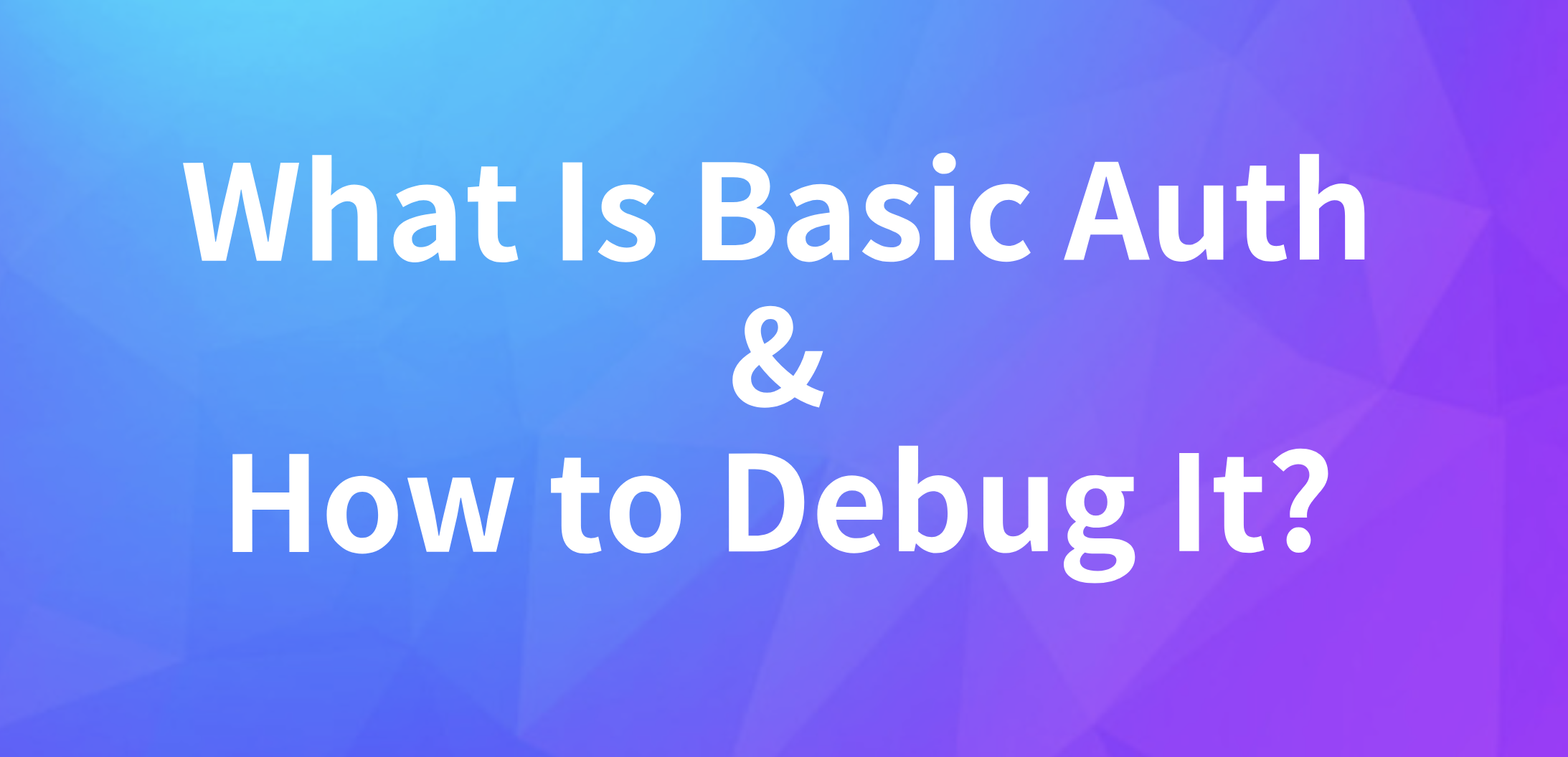
What is Basic Auth? ๐ก๏ธ
Basic Auth (Basic Authentication) is a simple yet effective method to secure REST APIs. It requires the client to send the username and password encoded in Base64 format in the HTTP request header. This method involves:
- Client: Sends a request with the Authorization header containing
Basic <Base64Encoded(username:password)>
. - Server: Decodes the Base64 string, verifies the credentials, and responds accordingly.
Despite its simplicity, Basic Auth has certain limitations. The credentials are encoded, not encrypted, making it vulnerable if used over non-secure connections. Thus, it is advisable to use Basic Auth over HTTPS.
Implementing Basic Auth in Java and Go Code ๐ป
Java Implementation:
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Base64;
public class BasicAuthExample {
public static void main(String[] args) throws IOException {
String user = "username";
String password = "password";
String auth = user + ":" + password;
String encodedAuth = Base64.getEncoder().encodeToString(auth.getBytes());
URL url = new URL("https://api.example.com/resource");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Authorization", "Basic " + encodedAuth);
int responseCode = connection.getResponseCode();
System.out.println("Response Code : " + responseCode);
}
}
Go Implementation:
package main
import (
"encoding/base64"
"fmt"
"net/http"
"io/ioutil"
)
func main() {
username := "username"
password := "password"
auth := username + ":" + password
encodedAuth := base64.StdEncoding.EncodeToString([]byte(auth))
client := &http.Client{}
req, _ := http.NewRequest("GET", "https://api.example.com/resource", nil)
req.Header.Add("Authorization", "Basic "+encodedAuth)
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error:", err)
return
}
defer resp.Body.Close()
body, _ := ioutil.ReadAll(resp.Body)
fmt.Println("Response Body:", string(body))
}
Tools to Test Basic Auth ๐งช
Several tools can help test REST APIs secured with Basic Auth. Here's a guide for using some popular options:
1. EchoAPI
EchoAPI is a powerful tool for API testing. Here's how to use it for Basic Auth:
- Open EchoAPI and create a new request.
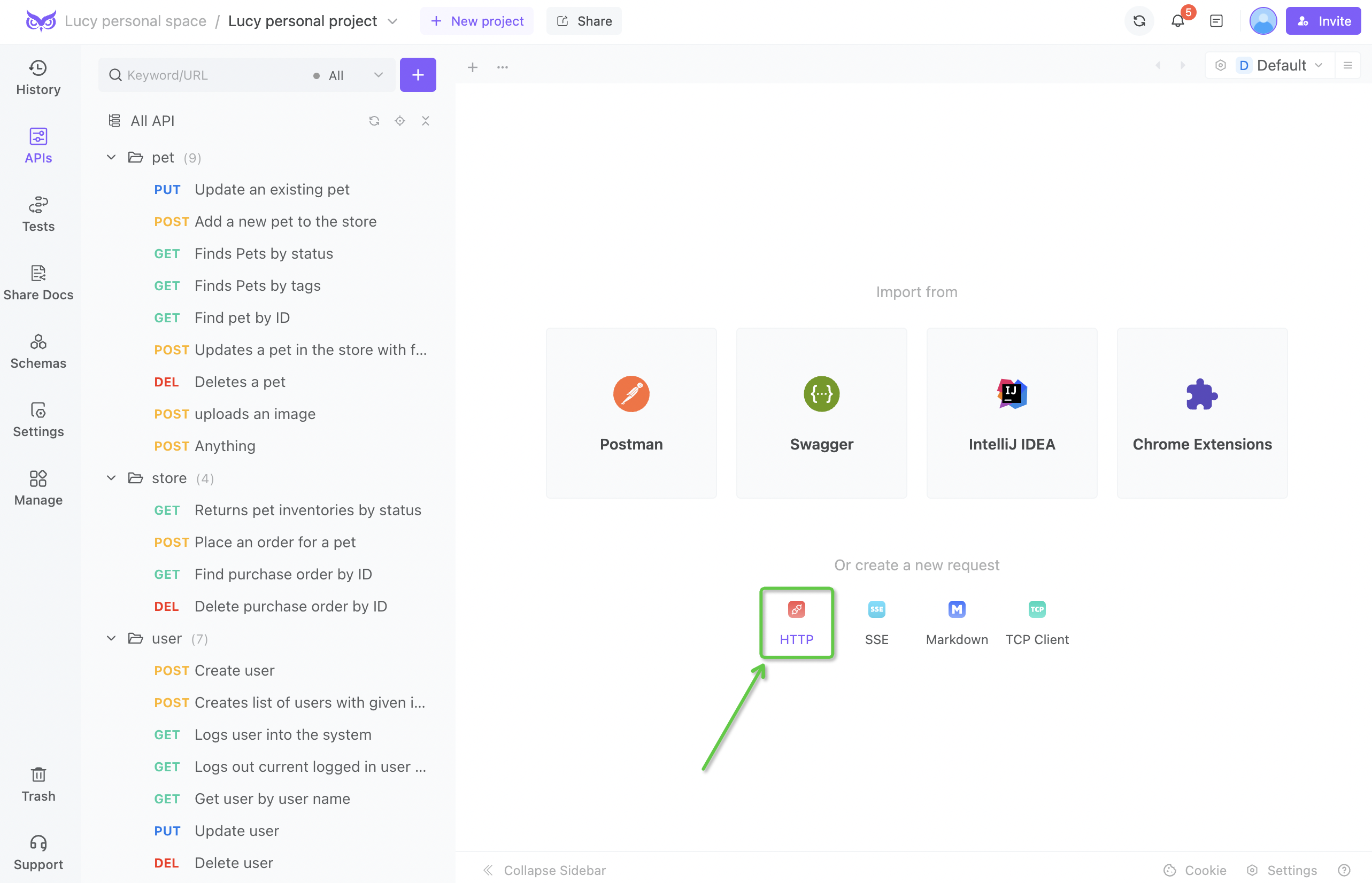
- Select the Authorization tab.
- Choose Basic Auth from the dropdown.
- Enter your username and password.
- Send the request and inspect the response.
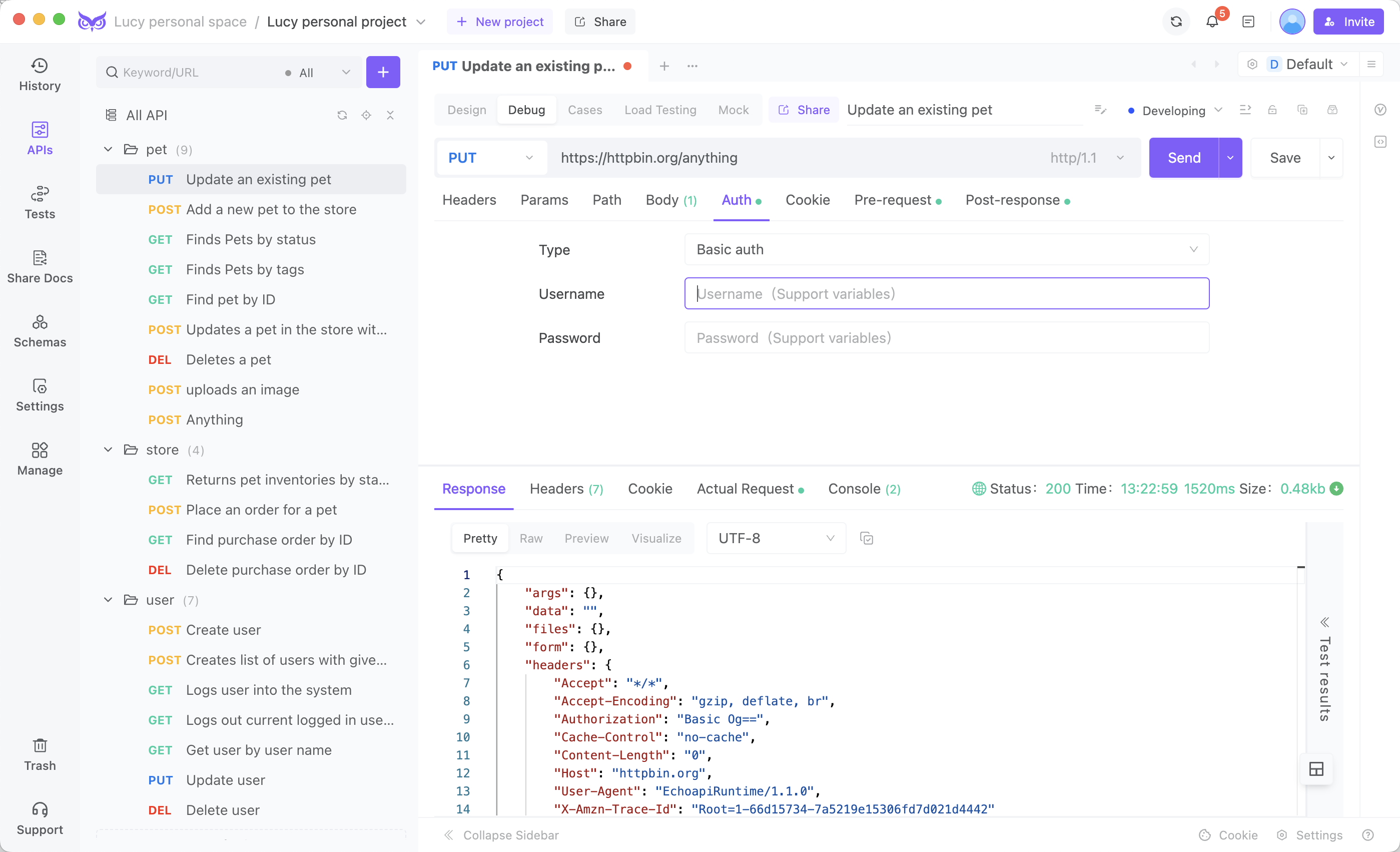
2. Curl
Curl is a versatile command-line tool for making network requests. Here's an example command:
curl -u username:password https://api.example.com/resource
3. Postman
Here's how to use Postman for Basic Auth:
- Open Postman and create a new request.
- Select the Authorization tab.
- Choose Basic Auth from the dropdown.
- Enter your username and password.
- Send the request and inspect the response.
Conclusion ๐
Authentication is a critical aspect of securing REST APIs. Basic Auth provides a straightforward method to add a layer of security, although it should be coupled with HTTPS to ensure data safety. Implementing Basic Auth in Java and Go is relatively simple, and various testing tools like Postman, Curl, and Insomnia make it easy to verify the setup. By understanding and applying these principles, developers can ensure their APIs are both functional and secure. ๐
Happy Securing! ๐๐