Why DTO is the Secret Weapon for API Design Efficiency and Security?
In the rapidly evolving world of software development, ensuring the security and efficiency of APIs is crucial. This article explores how employing Data Transfer Objects (DTOs) can serve as a secret weapon for achieving these goals.
You're running a super-secret spy agency, and you need to communicate with your field agents. The problem? You can’t send too much information—you don’t want enemy spies intercepting sensitive data!
That’s where DTO (Data Transfer Object) comes in. It’s a class or object that you define yourself to format your data.
Think of it as a classified spy report—only sending the necessary details while keeping secret stuff hidden.
What is a DTO (Data Transfer Object)?
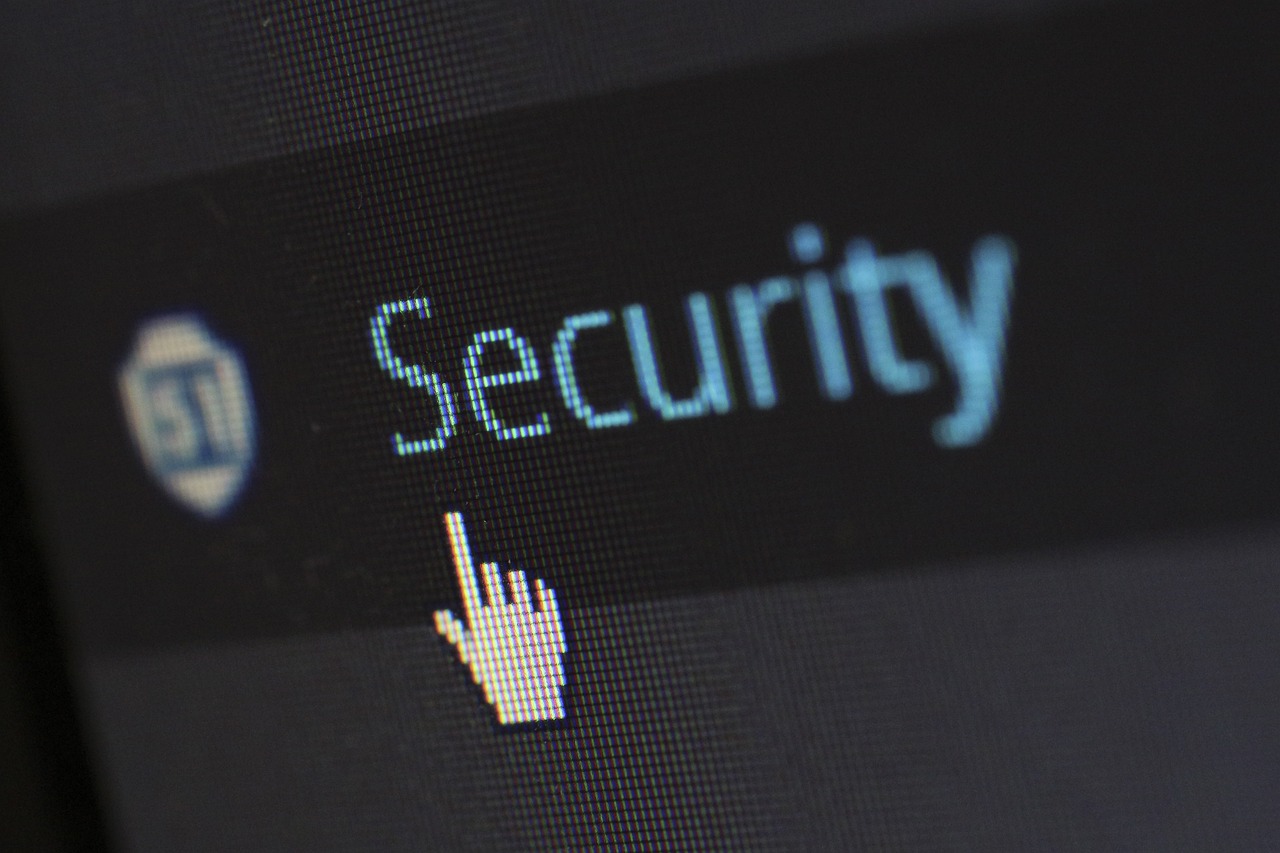
A DTO (Data Transfer Object) is a design pattern used to carry data between processes.
In simpler terms, it's an object that transports data without any business logic. You use it mainly to avoid directly exposing your database entities to external systems or clients (e.g., web apps, mobile apps). Instead, you define a clean, simple, and secure version of the data (just the information you want to expose).
Think of DTOs like mission reports for spies —you only send the essential info, leaving out anything unnecessary or sensitive.
DTOs help by:
âś… Keeping responses clean (only the required data)
âś… Improving security (hiding internal details)
âś… Boosting performance (reducing payload size)
âś… Making frontend-backend communication easier
API Implementation with DTOs
We’ve created a basic Spy Agency API with DTOs (Data Transfer Objects) for both requests and responses. Let’s go step-by-step to understand the input, processing, and output for both GET and POST requests.
1. Full Agent Model (Database
An in-memory database (just a JavaScript array) called agents
, where each agent has sensitive information like their realName, password, missionDetails, and clearanceLevel. Here's what a typical agent looks like in the database:
const agents = [
{
id: 1,
codename: "Shadow Wolf",
realName: "John Doe",
email: "shadowwolf@spyagency.com",
password: "SuperSecret123",
missionDetails: "Undercover operation in Europe",
clearanceLevel: "Top Secret"
}
];
Problem:
- We want to avoid sending sensitive data (like
realName
,password
, ormissionDetails
) back to the client when someone fetches agent details.
2. DTOs for Requests & Responses
We’ve created two DTOs:
agentResponseDTO
: This is used to format the response that goes back to the client. It only includes safe data likecodename
andclearanceLevel
.agentRequestDTO
: This is used to parse the incoming request when creating a new agent. It accepts thecodename
,email
, andpassword
from the request body.
Response DTO (agentResponseDTO
)
function agentResponseDTO(agent) {
return {
codename: agent.codename,
clearanceLevel: agent.clearanceLevel
};
}
- Purpose: Converts the agent object to only send safe fields in the API response.
Request DTO (agentRequestDTO
)
function agentRequestDTO(requestBody) {
return {
codename: requestBody.codename,
email: requestBody.email,
password: requestBody.password
};
}
- Purpose: Converts the incoming request body into an object with just the necessary fields.
3. API Endpoints
GET /agents
- Get Secure List of Agents
When you hit the GET /agents
endpoint, the API will return a list of agents, but only the safe data that has been processed through the agentResponseDTO
. This ensures that sensitive details (like passwords or mission information) are not exposed.
Example Request:
GET http://localhost:3000/agents
Example Response:
[
{
"codename": "Shadow Wolf",
"clearanceLevel": "Top Secret"
}
]
Explanation:
- The response includes the codename, clearance level of each agent, but NOT their password, real name, email, or mission details.
- This keeps the sensitive data hidden and only exposes what’s necessary to the client.
POST /agents
- Create a New Agent
When you send a POST
request to /agents
, the API will create a new agent. The request body will contain the codename, email, and password (which will be saved, but not returned). The clearance level will be set to "Classified" by default.
Example Request:
POST http://localhost:3000/agents
Content-Type: application/json
{
"codename": "Night Hawk",
"email": "nighthawk@spyagency.com",
"password": "superSecret123"
}
Example Response:
{
"codename": "Night Hawk",
"email": "nighthawk@spyagency.com",
"clearanceLevel": "Classified"
}
Explanation:
- The request body contains the data sent by the client:
codename
,email
, andpassword
. - The server processes the request and creates a new agent, with the clearance level automatically set to "Classified".
- The response includes only safe data (
codename
,email
,clearanceLevel
), not the password. - Password is stored securely (although for real applications, passwords should be hashed before storage).
What Would Other Users See?
Scenario 1: Getting the List of Agents (GET /agents)
A client calls the GET /agents
API and receives only safe information:
[
{
"codename": "Shadow Wolf",
"clearanceLevel": "Top Secret"
}
]
Scenario 2: Creating a New Agent (POST /agents)
When creating a new agent, the client sends a request with sensitive data (password
), but the API responds with safe information:
Request:
{
"codename": "Night Hawk",
"email": "nighthawk@spyagency.com",
"password": "superSecret123"
}
Response:
{
"codename": "Night Hawk",
"email": "nighthawk@spyagency.com",
"clearanceLevel": "Classified"
}
Notice that even though the client submitted a password, the response doesn’t include it. The server ensures that only safe details are sent back.
In this example, the client will see a clean and secure API response—and never the sensitive data like passwords or mission details—thanks to the use of DTOs.
Why Use DTOs in API Design and How to Build a Secure API?
Method | Purpose |
---|---|
AgentDTO | Returns only safe information to API responses |
AgentRequestDTO | Accepts only necessary fields for creating/updating agents |
Service Layer Converts Data | Prevents direct exposure of the database model |
Controller Uses DTOs | Ensures API is clean, secure, and efficient |
Designing a secure API that works effectively requires a thoughtful approach to structure, data flow, and efficiency.
But with EchoAPI, you can efficiently manage API design without unnecessary complexity.
Why EchoAPI for MCP & RAG API Design?
- All-in-One API Platform – Design, test, debug, and document your API in one place.
- No Login Required – Instantly start working, no account setup needed.
- Smart Authentication – Support for OAuth 2.0, JWT, AWS Signature, and more.
- Multiple Protocols – HTTP, GraphQL, WebSocket, SSE, TCP—you name it!
- Cross-Tool Compatibility – Seamlessly import/export from Postman, Swagger, and Insomnia.
đź“– Learn More:
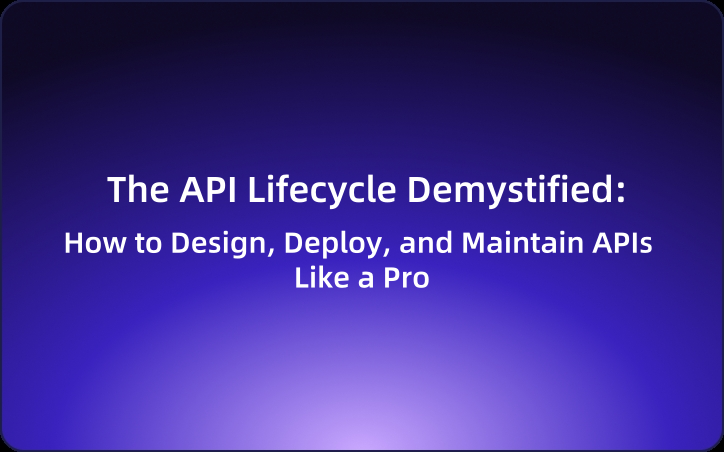
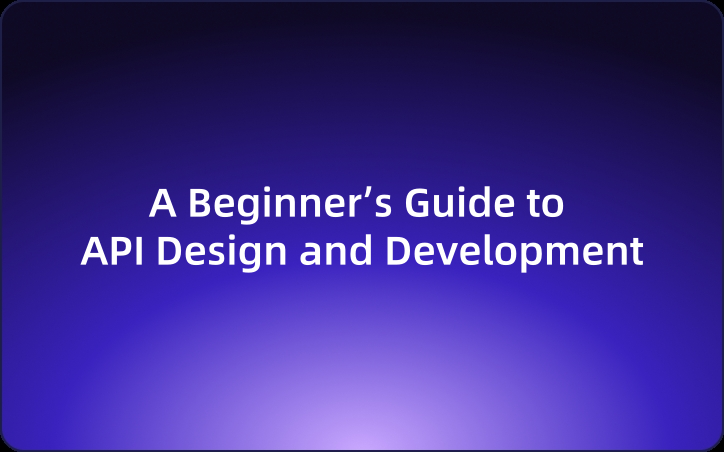
Now, your API is secure, efficient, and structured!
By using DTOs (Data Transfer Objects), you've taken a crucial step in ensuring that your API not only provides the necessary data but also protects sensitive information.
With DTOs in place, you’ve effectively organized your API design, improving security, performance, and clarity, making it easier for both developers and users to interact with.