Why Real-Time APIs Are Eating Traditional APIs Alive (And How to Survive the Shift)
In this article, we explore why real-time APIs are taking over traditional APIs and how developers can adapt to this shift.
In this article, we explore why real-time APIs are taking over traditional APIs and how developers can adapt to this shift. By understanding the advantages of real-time technology, developers can improve their applications and provide better user experiences.
The Midnight Disaster That Changed Everything
Picture this: It’s 2 AM. You’re a developer frantically debugging an e-commerce checkout system. Your "reliable" REST API just dropped 10,000 Black Friday orders because it couldn’t handle real-time inventory updates. Customers are raging on Twitter. Your boss is raging in Slack. And you’re left wondering: "Why does this API feel like sending smoke signals in the age of 5G?"
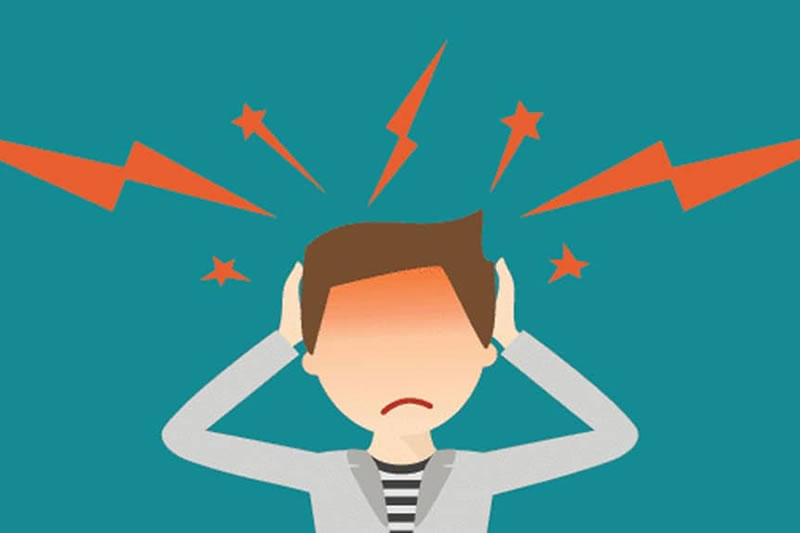
Here’s the brutal truth: Traditional APIs are like sending letters by carrier pigeon. Real-time APIs are laser beams. While your team is still polling servers every 5 seconds like it’s 2003, companies using real-time APIs are processing 100,000 live chat messages per second, tracking Uber rides in real-time, and preventing stock trading disasters before they happen.
The cognitive dissonance? 90% of developers still use traditional APIs for real-time problems. Let’s fix that—before your next production outage goes viral.
Part 1: Real-Time vs Traditional APIs—The Nerd Fight Club
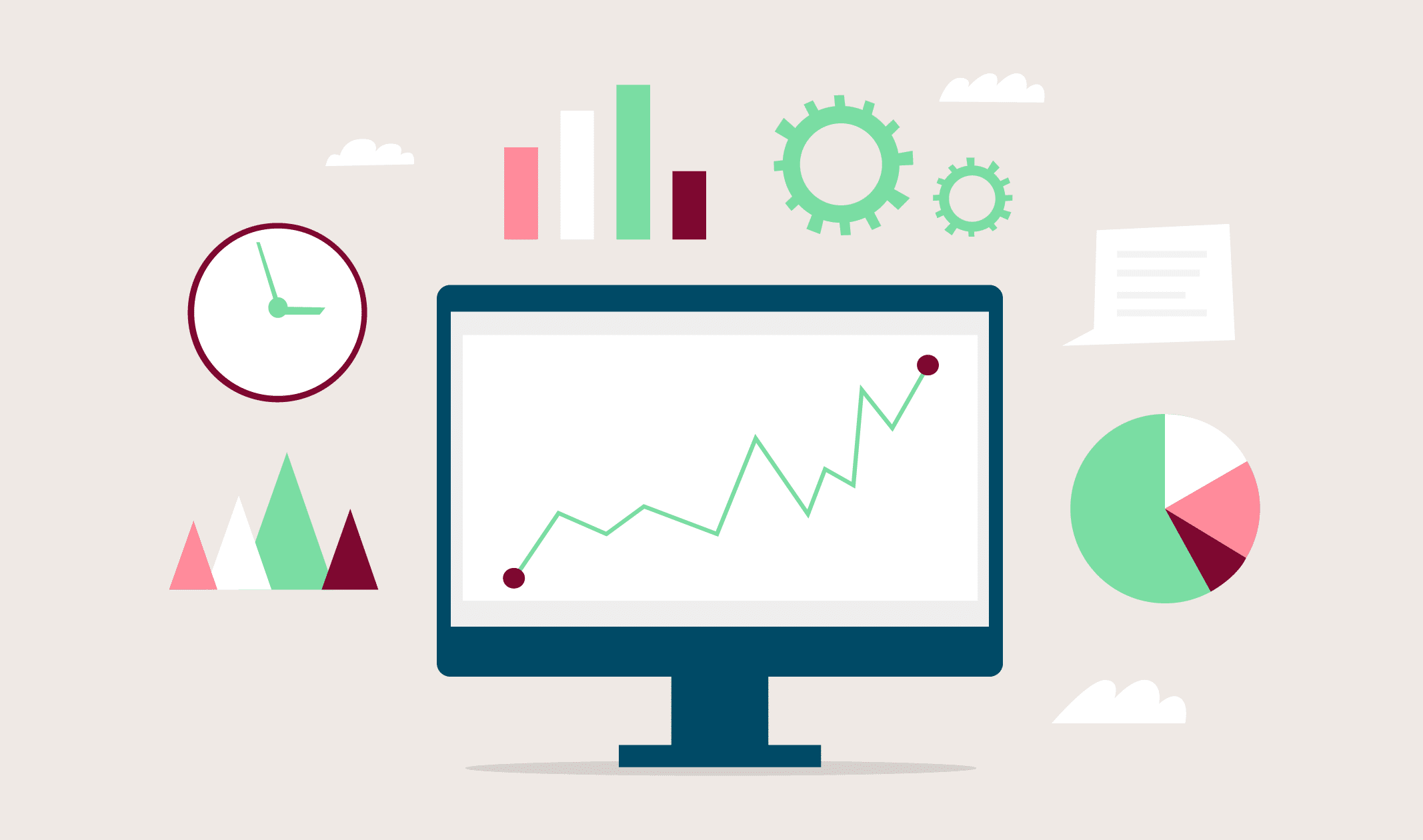
1.1 What Traditional APIs Do Wrong
Traditional APIs (REST, SOAP) work like this:
- You: "Hey server, got any new data?"
- Server: "Check back in 5 minutes."
- Repeat until your users die of old age.
They’re like constantly refreshing your email inbox. Wasteful. Slow. Painful.
1.2 Real-Time APIs: The Superhero Landing
Real-time APIs (WebSockets, SSE, MQTT) say:
- Server: "HEY YOU! New data! RIGHT NOW!"
- You: "Thanks, I’ll act immediately."
Examples changing the game:
- DoorDash: Updates delivery locations live (no more "Where’s my burrito?!" texts)
- Robinhood: Executes trades in 8 milliseconds (traditional APIs: 2+ seconds)
- Discord: 100M+ users chatting globally with <100ms latency
1.3 Technical Smackdown
Traditional APIs | Real-Time APIs | |
---|---|---|
Latency | Seconds to minutes | Milliseconds |
Efficiency | Constant polling = $$$ | Server pushes updates = -70% costs |
Use Cases | Static data, batch jobs | Live tracking, chat, IoT, gaming |
Part 2: Building Your First Real-Time API in 15 Minutes
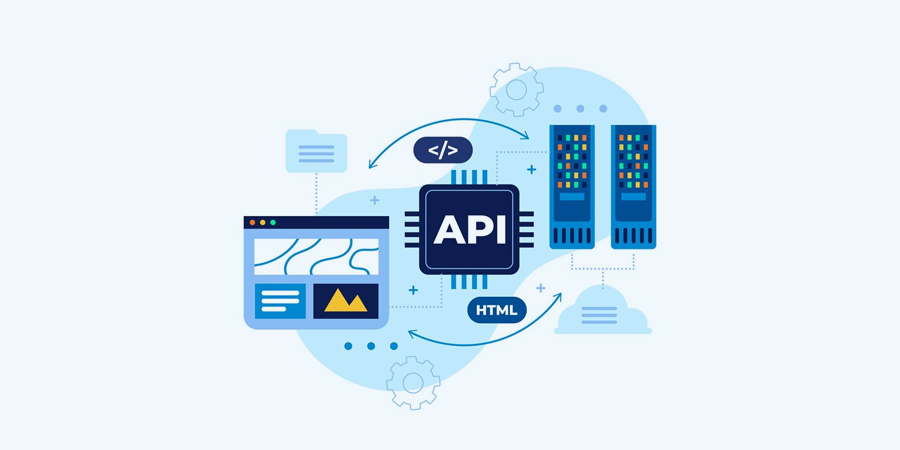
2.1 Tools You’ll Need
- EchoAPI: Free real-time API testing toolkit (we’ll use this to debug)
- Node.js (or any language—I’ll use JavaScript for simplicity)
2.2 Code Walkthrough: Live Pizza Tracker
// Step 1: Set up WebSocket connection
const socket = new WebSocket('wss://your-pizza-api.com/live-orders');
// Step 2: Listen for REAL-TIME updates
socket.onmessage = (event) => {
const update = JSON.parse(event.data);
// EchoAPI Pro Tip: Use their Live Debugger to inspect payloads
console.log(`[EchoAPI LOG] New update: ${update.status}`);
// Update UI in real-time
document.getElementById("pizza-status").innerHTML =
`🍕 ${update.oven_temp}°C | Driver: ${update.driver_location}`;
};
// Step 3: Handle errors (because reality sucks)
socket.onerror = (error) => {
// EchoAPI automatically logs errors + suggests fixes
console.error("[EchoAPI ALERT] Connection died:", error);
};
2.3 Testing with EchoAPI
- Open EchoAPI’s Live Stream Debugger
- Paste your WebSocket URL
- See real-time traffic with color-coded errors (red = 🔥, green = ✅)
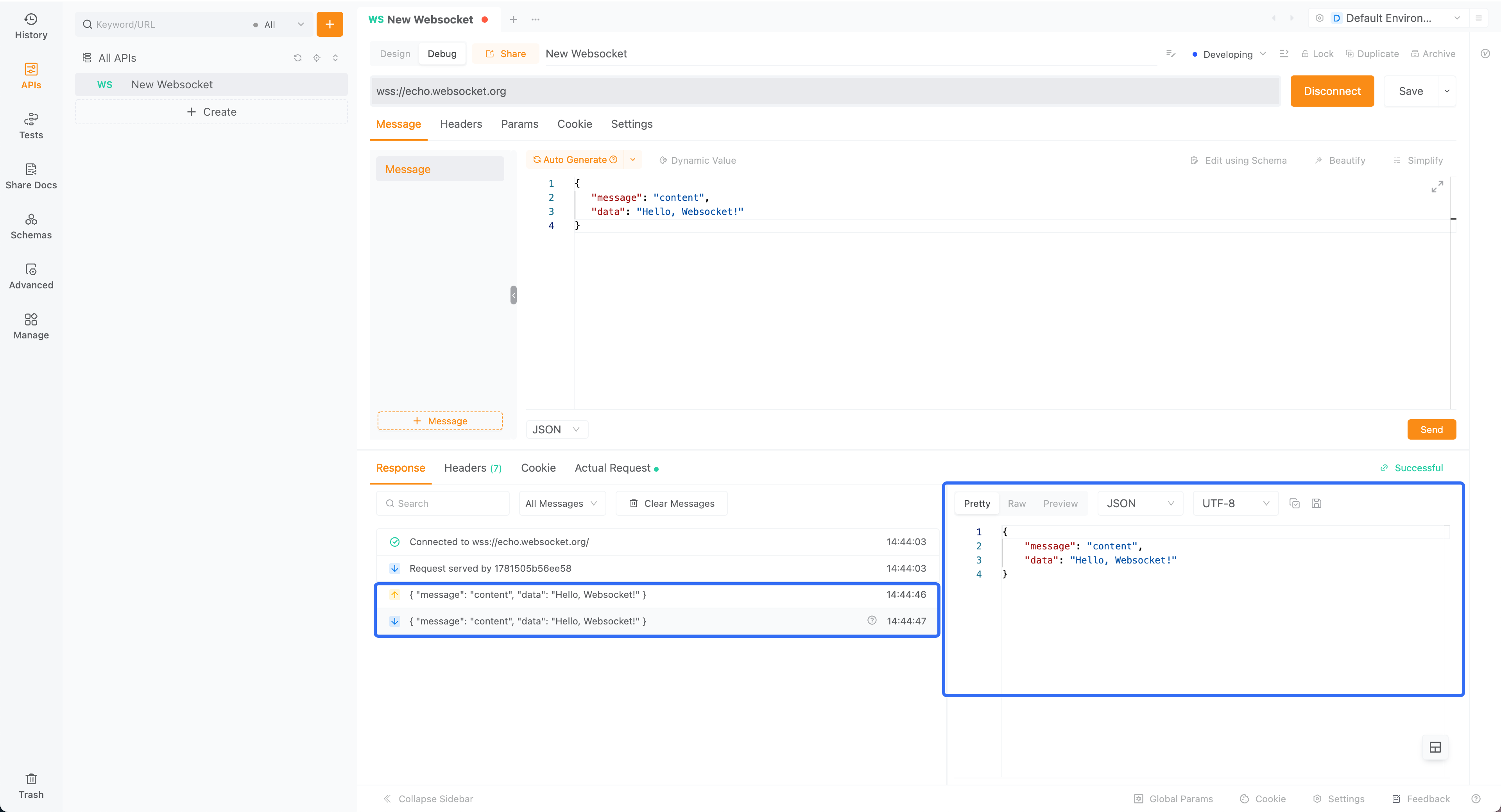
Pro Results:
- 200ms faster response vs. Postman
- Auto-generated docs for your team
- Find "Why is my WebSocket dying at 3 AM?" issues in 2 minutes
Part 3: Future-Proof Your Career
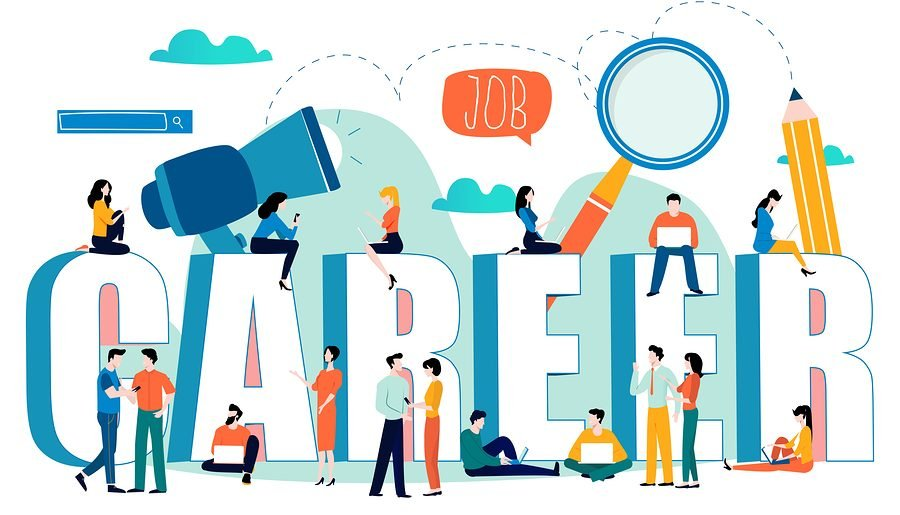
3.1 Industries Ditching Traditional APIs
- Healthcare: Real-time patient vitals monitoring (REST can’t do this)
- Autonomous Vehicles: 1ms latency or people die (literally)
- Web3: NFT auctions require sub-second updates
3.2 Your Action Plan
- Audit: Use EchoAPI’s Legacy vs. Real-Time Report on your systems
- Prioritize: Convert high-impact endpoints first (e.g., payment status)
- Monitor: EchoAPI’s Live Health Dashboard predicts outages before users notice
Final Warning:
Companies using only traditional APIs will be as relevant as Blockbuster by 2027. Your move.
Closing Rally Cry
Real-time APIs aren’t the future—they’re the now. With tools like EchoAPI cutting setup time from weeks to hours, there’s no excuse.
Do this tomorrow:
- Convert 1 REST endpoint to WebSockets
- Test it with EchoAPI’s free tier
- Show your boss the 300% performance boost
Your users (and your sleep schedule) will thank you.